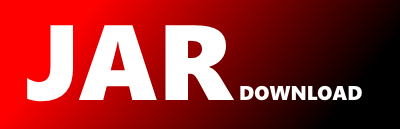
org.objectweb.petals.plugin.jbiplugin.JBIStartMojo Maven / Gradle / Ivy
/**
* PETALS - PETALS Services Platform.
* Copyright (c) 2005 EBM Websourcing, http://www.ebmwebsourcing.com/
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* -------------------------------------------------------------------------
* $Id: PackageAssemblyMojo.java 16:56:43 ddesjardins $
* -------------------------------------------------------------------------
*/
package org.objectweb.petals.plugin.jbiplugin;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.objectweb.petals.jbi.descriptor.JBIDescriptor;
import org.objectweb.petals.tools.jmx.api.PetalsComponentComponentClient;
import org.objectweb.petals.tools.jmx.api.PetalsDeploymentServiceClient;
import org.objectweb.petals.tools.jmx.api.PetalsJMXClient;
import org.objectweb.petals.tools.jmx.api.exception.AttributeErrorException;
import org.objectweb.petals.tools.jmx.api.exception.ComponentComponentDoesNotExistException;
import org.objectweb.petals.tools.jmx.api.exception.ComponentErrorException;
import org.objectweb.petals.tools.jmx.api.exception.ConnectionErrorException;
import org.objectweb.petals.tools.jmx.api.exception.DeploymentServiceDoesNotExistException;
import org.objectweb.petals.tools.jmx.api.exception.DeploymentServiceErrorException;
import org.objectweb.petals.tools.jmx.api.exception.PerformActionErrorException;
/**
* Start a JBI Artefact (Component / Service Assembly)
*
* @goal jbi-start
* @description Start a JBI Artefact (Component / Service Assembly)
*
* @author cdeneux - Capgemini Sud
*/
public class JBIStartMojo extends JBIAbstractJMXMojo {
/**
* Execute the plugin
*/
@Override
public void executeMojo() throws MojoExecutionException, MojoFailureException {
try {
JBIDescriptor jbiDescriptor = this.readJbiDescriptor(project
.getArtifact().getFile());
this.getLog().info(
"Using JMX Petals server located at: " + this.host + ":"
+ this.port);
PetalsJMXClient petalsJMXClient = new PetalsJMXClient(this.host,
this.port, this.username, this.password);
if (PACKAGING_COMPONENT.equals(project.getPackaging())) {
this.startComponent(petalsJMXClient, jbiDescriptor);
} else if (PACKAGING_SA.equals(project.getPackaging())) {
this.startServiceAssembly(petalsJMXClient, jbiDescriptor);
} else if (PACKAGING_SU.equals(project.getPackaging())) {
/*
* NOP because an SU is not startable, see its service assembly.
*
* No error is returned to be able to use the same plugin
* configuration for the different JBI artefacts.
*/
this.info("Start a service-unit has no sens. This goal is skipped.");
} else if (PACKAGING_SL.equals(project.getPackaging())) {
/*
* NOP because an SL is not startable.
*
* No error is returned to be able to use the same plugin
* configuration for the different JBI artefacts.
*/
this.info("Start a shared-libarary has no sens. This goal is skipped.");
}
} catch (ConnectionErrorException e) {
throw new MojoExecutionException("Error", e);
}
}
private void startComponent(PetalsJMXClient petalsJMXClient,
final JBIDescriptor jbiDescriptor) throws MojoExecutionException {
try {
String componentName = this.getComponentNameFromJBIDescriptor(jbiDescriptor);
PetalsComponentComponentClient pccc = null;
// We check if the component exists
pccc = petalsJMXClient.getComponentComponentClient(componentName);
if (PetalsComponentComponentClient.State.STARTED.equals(pccc
.getState())) {
// The component is already started
throw new MojoExecutionException(
"The component is already started.");
}
if ((PetalsComponentComponentClient.State.SHUTDOWN.equals(pccc
.getState()))
|| (PetalsComponentComponentClient.State.STOPPED
.equals(pccc.getState()))) {
// We shutdown the component
this.info("Starting the component.");
pccc.start();
this.info("The component is started.");
}
} catch (ComponentComponentDoesNotExistException e1) {
// The component is not installed
throw new MojoExecutionException("The component is not installed.");
} catch (ComponentErrorException e) {
throw new MojoExecutionException("Error", e);
} catch (ConnectionErrorException e) {
throw new MojoExecutionException("Error", e);
} catch (AttributeErrorException e) {
throw new MojoExecutionException("Error", e);
} catch (PerformActionErrorException e) {
throw new MojoExecutionException("Error", e);
}
}
private void startServiceAssembly(PetalsJMXClient petalsJMXClient,
final JBIDescriptor jbiDescriptor) throws MojoExecutionException {
try {
String serviceAssemblyName = this
.getServiceAssemblyNameFromJBIDescriptor(jbiDescriptor);
PetalsDeploymentServiceClient pdsc = petalsJMXClient
.getDeploymentServiceClient();
// We check if the SA is already deployed
this.info("Checking service assembly state.");
if (pdsc.isServiceAssemblyDeployed(serviceAssemblyName)) {
this.info("The service assembly is deployed.");
// We check its state to know if we can start it
if (PetalsDeploymentServiceClient.State.SHUTDOWN.equals(pdsc
.getState(serviceAssemblyName))
|| PetalsDeploymentServiceClient.State.STOPPED
.equals(pdsc.getState(serviceAssemblyName))) {
// We can start it
this.info("Starting the service assembly.");
// TODO: Add a forced mode: when activated, check if
// needed components are started and start them
String result = pdsc.start(serviceAssemblyName);
this.info("The service assembly is started: " + result);
} else if (PetalsDeploymentServiceClient.State.STARTED
.equals(pdsc.getState(serviceAssemblyName))) {
this.info("The service assembly is already started.");
} else {
throw new MojoExecutionException(
"Error, the service assembly "
+ serviceAssemblyName
+ " is in the following state: "
+ pdsc.getState(serviceAssemblyName));
}
} else {
throw new MojoExecutionException("The service-assembly "
+ serviceAssemblyName + " is not deployed.");
}
} catch (DeploymentServiceDoesNotExistException e) {
throw new MojoExecutionException("Error", e);
} catch (DeploymentServiceErrorException e) {
throw new MojoExecutionException("Error", e);
} catch (ConnectionErrorException e) {
throw new MojoExecutionException("Error", e);
} catch (PerformActionErrorException e) {
throw new MojoExecutionException("Error", e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy