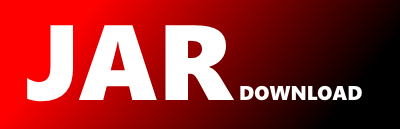
org.objectweb.petals.plugin.jbiplugin.JBIStopMojo Maven / Gradle / Ivy
/**
* PETALS - PETALS Services Platform.
* Copyright (c) 2005 EBM Websourcing, http://www.ebmwebsourcing.com/
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* -------------------------------------------------------------------------
* $Id: PackageAssemblyMojo.java 16:56:43 ddesjardins $
* -------------------------------------------------------------------------
*/
package org.objectweb.petals.plugin.jbiplugin;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.objectweb.petals.jbi.descriptor.JBIDescriptor;
import org.objectweb.petals.tools.jmx.api.PetalsComponentComponentClient;
import org.objectweb.petals.tools.jmx.api.PetalsDeploymentServiceClient;
import org.objectweb.petals.tools.jmx.api.PetalsJMXClient;
import org.objectweb.petals.tools.jmx.api.exception.AttributeErrorException;
import org.objectweb.petals.tools.jmx.api.exception.ComponentComponentDoesNotExistException;
import org.objectweb.petals.tools.jmx.api.exception.ComponentErrorException;
import org.objectweb.petals.tools.jmx.api.exception.ConnectionErrorException;
import org.objectweb.petals.tools.jmx.api.exception.DeploymentServiceDoesNotExistException;
import org.objectweb.petals.tools.jmx.api.exception.DeploymentServiceErrorException;
import org.objectweb.petals.tools.jmx.api.exception.PerformActionErrorException;
/**
* Stop a JBI Artefact (Component / Service Assembly)
*
* @goal jbi-stop
* @description Stop a JBI Artefact (Component / Service Assembly)
*
* @author cdeneux - Capgemini Sud
*/
public class JBIStopMojo extends JBIAbstractJMXMojo {
/**
* Flag to force the service-assembly uninstallation when shutdowning a JBI
* component.
*
* @parameter expression="false"
* @editable true
* @description Flag to force the service-assembly uninstallation when
* shutdowning a JBI component.
*/
protected Boolean undeploySAs;
/**
* Execute the plugin
*/
@Override
public void executeMojo() throws MojoExecutionException, MojoFailureException {
try {
JBIDescriptor jbiDescriptor = this.readJbiDescriptor(project
.getArtifact().getFile());
this.getLog().info(
"Using JMX Petals server located at: " + this.host + ":"
+ this.port);
PetalsJMXClient petalsJMXClient = new PetalsJMXClient(this.host,
this.port, this.username, this.password);
if (PACKAGING_COMPONENT.equals(project.getPackaging())) {
this.stopComponent(petalsJMXClient, jbiDescriptor);
} else if (PACKAGING_SA.equals(project.getPackaging())) {
this.stopServiceAssembly(petalsJMXClient, jbiDescriptor);
} else if (PACKAGING_SU.equals(project.getPackaging())) {
/*
* NOP because an SU is not startable, see its service assembly.
*
* No error is returned to be able to use the same plugin
* configuration for the different JBI artefacts.
*/
this
.info("Start a service-unit has no sens. This goal is skipped.");
} else if (PACKAGING_SL.equals(project.getPackaging())) {
/*
* NOP because an SL is not startable.
*
* No error is returned to be able to use the same plugin
* configuration for the different JBI artefacts.
*/
this
.info("Start a shared-libarary has no sens. This goal is skipped.");
}
} catch (ConnectionErrorException e) {
throw new MojoExecutionException("Error", e);
}
}
private void stopComponent(PetalsJMXClient petalsJMXClient,
final JBIDescriptor jbiDescriptor) throws MojoExecutionException {
try {
String componentName = this
.getComponentNameFromJBIDescriptor(jbiDescriptor);
PetalsComponentComponentClient pccc = null;
// We check if the component exists
pccc = petalsJMXClient.getComponentComponentClient(componentName);
if (PetalsComponentComponentClient.State.STARTED.equals(pccc
.getState())
|| PetalsComponentComponentClient.State.STOPPED.equals(pccc
.getState())) {
// Check and shutdown SA if needed
this.checkAndUndeployServiceAssemblies(petalsJMXClient, componentName, "\t");
if (PetalsComponentComponentClient.State.STARTED.equals(pccc
.getState())) {
this.info("The component is started. Stopping it ...");
pccc.stop();
this.info("Component stopped.");
}
if (PetalsComponentComponentClient.State.STOPPED.equals(pccc
.getState())) {
this.info("The component is stopped. Shutdowning it ...");
pccc.shutdown();
this.info("Component shutdowned.");
}
} else {
throw new MojoExecutionException("Error, the component "
+ componentName + " is in the following state: "
+ pccc.getState());
}
} catch (ComponentComponentDoesNotExistException e1) {
// The component is not installed
this.warn("The component is not installed. No error is raised.");
} catch (ComponentErrorException e) {
throw new MojoExecutionException("Error", e);
} catch (ConnectionErrorException e) {
throw new MojoExecutionException("Error", e);
} catch (AttributeErrorException e) {
throw new MojoExecutionException("Error", e);
} catch (PerformActionErrorException e) {
throw new MojoExecutionException("Error", e);
}
}
private void checkAndUndeployServiceAssemblies(PetalsJMXClient petalsJMXClient,
final String componentName, final String prefixLog)
throws MojoExecutionException {
if (this.force) {
this.info("Force mode activated.");
try {
PetalsDeploymentServiceClient pdsc = petalsJMXClient
.getDeploymentServiceClient();
String[] serviceAssemblies = pdsc
.getDeployedServiceAssembliesForComponent(componentName);
for (int i = 0; i < serviceAssemblies.length; i++) {
this.info(prefixLog + "Shutdowning the service-assembly: "
+ serviceAssemblies[i] + " ...");
this.shutdownDeployedSA(serviceAssemblies[i], pdsc,
prefixLog + "\t");
this.info(prefixLog + "service-assembly shutdowned.");
if (this.undeploySAs) {
this.info(prefixLog
+ "Undeploying the service-assembly: "
+ serviceAssemblies[i] + " ...");
pdsc.undeploy(serviceAssemblies[i]);
this.info(prefixLog + "service-assembly undeployed.");
}
}
} catch (DeploymentServiceErrorException e) {
throw new MojoExecutionException("Error", e);
} catch (DeploymentServiceDoesNotExistException e) {
throw new MojoExecutionException(
"Unable to find the deployment service ", e);
} catch (ConnectionErrorException e) {
throw new MojoExecutionException("Error", e);
} catch (PerformActionErrorException e) {
throw new MojoExecutionException("Error", e);
}
}
}
private void stopServiceAssembly(PetalsJMXClient petalsJMXClient,
final JBIDescriptor jbiDescriptor) throws MojoExecutionException {
try {
String serviceAssemblyName = this
.getServiceAssemblyNameFromJBIDescriptor(jbiDescriptor);
PetalsDeploymentServiceClient pdsc = petalsJMXClient
.getDeploymentServiceClient();
// We check if the SA is already deployed
this.info("Checking service assembly state.");
if (pdsc.isServiceAssemblyDeployed(serviceAssemblyName)) {
this.info("The service assembly is deployed.");
this.shutdownDeployedSA(serviceAssemblyName, pdsc, "\t");
} else {
this.warn("The service-assembly " + serviceAssemblyName
+ " is not deployed.");
}
} catch (DeploymentServiceDoesNotExistException e) {
throw new MojoExecutionException("Error", e);
} catch (DeploymentServiceErrorException e) {
throw new MojoExecutionException("Error", e);
} catch (ConnectionErrorException e) {
throw new MojoExecutionException("Error", e);
} catch (PerformActionErrorException e) {
throw new MojoExecutionException("Error", e);
}
}
private void shutdownDeployedSA(final String serviceAssemblyName,
PetalsDeploymentServiceClient pdsc, final String prefixLog)
throws MojoExecutionException {
try {
// We check its state to know if we can stop it
if (PetalsDeploymentServiceClient.State.STARTED.equals(pdsc
.getState(serviceAssemblyName))) {
// We can stop it
this.info(prefixLog + "Stopping the service assembly.");
String result = pdsc.stop(serviceAssemblyName);
this.info(prefixLog + "The service assembly is stopped: "
+ result);
}
if (PetalsDeploymentServiceClient.State.STOPPED.equals(pdsc
.getState(serviceAssemblyName))) {
// We can shutdown it
this.info(prefixLog + "Shutdowning the service assembly.");
String result = pdsc.shutdown(serviceAssemblyName);
this.info(prefixLog + "The service assembly is shutdowned: "
+ result);
}
if (!PetalsDeploymentServiceClient.State.SHUTDOWN.equals(pdsc
.getState(serviceAssemblyName))) {
throw new MojoExecutionException("Error, the service assembly "
+ serviceAssemblyName + " is in the following state: "
+ pdsc.getState(serviceAssemblyName));
}
} catch (PerformActionErrorException e) {
throw new MojoExecutionException("Error", e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy