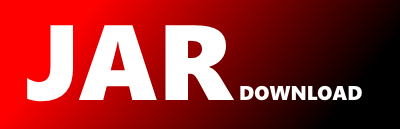
org.objectweb.petals.ant.task.InstallComponentTask Maven / Gradle / Ivy
The newest version!
/**
* PETALS: PETALS Services Platform
* Copyright (C) 2005 EBM WebSourcing
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA.
*
* Initial developer(s): EBM WebSourcing
*
* --------------------------------------------------------------------------
* $Id$
* --------------------------------------------------------------------------
*/
package org.objectweb.petals.ant.task;
import java.io.FileInputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import javax.management.Attribute;
import javax.management.MBeanServerConnection;
import javax.management.ObjectName;
import org.objectweb.petals.ant.AbstractInstallerAntTask;
/**
* Ant task : jbi-install-component. This task install a JBI component (service
* engine of binding component) into the JBI environment.
*
* @author ddesjardins chamerling - eBMWebsourcing
*/
public class InstallComponentTask extends AbstractInstallerAntTask {
/**
* The nested elements
*/
private List nestedParams = new ArrayList();
/**
* Location of the nestedParams file that contains MBean properties
*/
private String params;
/**
* Creates a new instance of {@link InstallComponentTask}
*
*/
public InstallComponentTask() {
super();
}
/**
* Execute the ant task
*
* @throws IOException
*/
public void doTask() throws Exception {
MBeanServerConnection connection = connector.getMBeanServerConnection();
Object result = performAction(connection,
AbstractInstallerAntTask.LOAD_NEW_INSTALLED);
if (result instanceof ObjectName) {
ObjectName installerName = (ObjectName) result;
// Get the installer configuration
Object result1 = connection.getAttribute(installerName,
"InstallerConfigurationMBean");
if (result1 instanceof ObjectName) {
// set the properties and parameters
ObjectName configurationMBean = (ObjectName) result1;
setConnectionAttributeFromPropertiesFile(connection,
configurationMBean);
setConnectionAttributeFromNestedParam(connection,
configurationMBean);
}
// Install the component
Object[] objects = new Object[0];
String[] strings = new String[0];
connection.invoke(installerName, "install", objects, strings);
try {
log("The component '" + installerName.getKeyProperty("name")
+ "' has been installed");
} catch (NullPointerException e) {
}
}
}
/**
* Get the properties from the params file.
*
* @return
* @throws IOException
*/
private Properties loadProperties() throws IOException {
Properties props = new Properties();
if (params != null)
props.load(new FileInputStream(params));
return props;
}
/**
* Set the connection attributes from the properties file
*
* @nestedParams connection
* @nestedParams configurationMBean
* @throws Exception
*/
private void setConnectionAttributeFromPropertiesFile(
MBeanServerConnection connection, ObjectName configurationMBean)
throws Exception {
Properties props = loadProperties();
for (Map.Entry entry : props.entrySet()) {
Attribute attribute = new Attribute((String) entry.getKey(),
(String) entry.getValue());
connection.setAttribute(configurationMBean, attribute);
}
}
/**
* Set the connection attribute from the nested params
*
* @nestedParams connection
* @nestedParams configurationMBean
* @throws Exception
*/
private void setConnectionAttributeFromNestedParam(
MBeanServerConnection connection, ObjectName configurationMBean)
throws Exception {
// Set the properties of the element
for (Param p : nestedParams) {
Attribute attribute = new Attribute(p.getName(), p.getValue());
connection.setAttribute(configurationMBean, attribute);
}
}
/**
* @return the params
*/
public String getParams() {
return params;
}
/**
* @nestedParams params
* the params to set
*/
public void setParams(String params) {
this.params = params;
}
/**
*
* @return
*/
public Param createParam() {
Param param = new Param();
nestedParams.add(param);
return param;
}
/**
* Parameter class for nested elements
* @author chamerling
*
*/
public static class Param {
/**
* Name attribute
*/
private String name;
/**
* Value attribute
*/
private String value;
/**
* Creates a new instance of {@link Param}
*
*/
public Param() {
this ("", "");
}
/**
* Creates a new instance of {@link Param}
*
* @param name
* @param value
*/
public Param(String name, String value) {
this.name = name;
this.value = value;
}
/**
* @return the name
*/
public String getName() {
return name;
}
/**
* @param name the name to set
*/
public void setName(String name) {
this.name = name;
}
/**
* @return the value
*/
public String getValue() {
return value;
}
/**
* @param value the value to set
*/
public void setValue(String value) {
this.value = value;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy