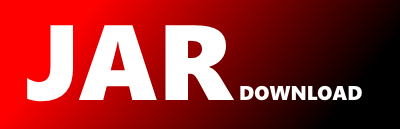
org.obolibrary.robot.RelatedObjectsHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of robot-core Show documentation
Show all versions of robot-core Show documentation
Core library for ROBOT: Library for working with OWL ontologies, especially Open Biological and Biomedical Ontologes (OBO).
The newest version!
package org.obolibrary.robot;
import com.google.common.collect.Lists;
import com.google.common.collect.Sets;
import java.util.*;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import java.util.stream.Collectors;
import org.semanticweb.owlapi.apibinding.OWLManager;
import org.semanticweb.owlapi.model.*;
import org.semanticweb.owlapi.model.parameters.Imports;
import org.semanticweb.owlapi.reasoner.OWLReasoner;
import org.semanticweb.owlapi.search.EntitySearcher;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Convenience methods to get related entities for a set of IRIs. Allowed relation options are: -
* ancestors - descendants - disjoints - domains - equivalents - inverses - ranges - types
*
* @author Becky Tauber
*/
public class RelatedObjectsHelper {
/** Logger. */
private static final Logger logger = LoggerFactory.getLogger(RelatedObjectsHelper.class);
/** Shared data factory. */
private static final OWLDataFactory df = OWLManager.getOWLDataFactory();
/** Namespace for error messages. */
private static final String NS = "errors#";
/** Error message when --axioms is not a valid AxiomType. Expects: input string. */
private static final String axiomTypeError = NS + "AXIOM TYPE ERROR %s is not a valid axiom type";
/**
* Error message when a datatype is given for an annotation, but the annotation value does not
* match the datatype.
*/
private static final String literalValueError = NS + "LITERAL VALUE ERROR %s is not a %s value";
/** Error message when an invalid IRI is provided. Expects the entry field and term. */
private static final String invalidIRIError =
NS + "INVALID IRI ERROR %1$s \"%2$s\" is not a valid CURIE or IRI";
/** Error message when an IRI pattern to match does not have a wildcard character. */
private static final String invalidIRIPatternError =
NS
+ "INVALID IRI PATTERN ERROR the pattern '%s' must contain at least one wildcard character.";
/** String for subclass/property mapping */
private static final String SUB = "sub";
/** String for superclass/property mapping */
private static final String SUPER = "super";
/**
* Given an ontology and a set of objects, return the annotation axioms for all objects.
*
* @param ontology OWLOntology to get annotations from
* @param objects OWLObjects to get annotations of
* @return set of OWLAxioms
*/
public static Set getAnnotationAxioms(OWLOntology ontology, Set objects) {
Set axioms = new HashSet<>();
for (OWLObject object : objects) {
if (object instanceof OWLClass) {
OWLClass cls = (OWLClass) object;
axioms.addAll(ontology.getAnnotationAssertionAxioms(cls.getIRI()));
} else if (object instanceof OWLObjectProperty) {
OWLObjectProperty prop = (OWLObjectProperty) object;
axioms.addAll(ontology.getAnnotationAssertionAxioms(prop.getIRI()));
} else if (object instanceof OWLDataProperty) {
OWLDataProperty prop = (OWLDataProperty) object;
axioms.addAll(ontology.getAnnotationAssertionAxioms(prop.getIRI()));
} else if (object instanceof OWLAnnotationProperty) {
OWLAnnotationProperty prop = (OWLAnnotationProperty) object;
axioms.addAll(ontology.getAnnotationAssertionAxioms(prop.getIRI()));
} else if (object instanceof OWLDatatype) {
OWLDatatype dt = (OWLDatatype) object;
axioms.addAll(ontology.getAnnotationAssertionAxioms(dt.getIRI()));
} else if (object instanceof OWLNamedIndividual) {
OWLNamedIndividual indiv = (OWLNamedIndividual) object;
axioms.addAll(ontology.getAnnotationAssertionAxioms(indiv.getIRI()));
}
}
return axioms;
}
/**
* Filter a set of OWLAxioms based on the provided arguments.
*
* @param inputAxioms Set of OWLAxioms to filter
* @param objects Set of OWLObjects to get axioms for
* @param axiomSelectors List of string selectors for types of axioms
* @param baseNamespaces List of string base namespaces used for internal/external selections
* @param partial if true, get any axiom containing at least one OWLObject from objects
* @param namedOnly if true, ignore anonymous OWLObjects in axioms
* @return set of filtered OWLAxioms
* @throws OWLOntologyCreationException on issue creating empty ontology for tautology checker
*/
public static Set filterAxioms(
Set inputAxioms,
Set objects,
List axiomSelectors,
List baseNamespaces,
boolean partial,
boolean namedOnly)
throws OWLOntologyCreationException {
// Go through the axiom selectors in order and process selections
boolean internal = false;
boolean external = false;
Set filteredAxioms = new HashSet<>();
for (String axiomSelector : axiomSelectors) {
if (axiomSelector.equalsIgnoreCase("internal")) {
if (external) {
logger.error(
"ignoring 'internal' axiom selector - 'internal' and 'external' together will remove all axioms");
continue;
}
filteredAxioms.addAll(
RelatedObjectsHelper.filterInternalAxioms(inputAxioms, baseNamespaces));
internal = true;
} else if (axiomSelector.equalsIgnoreCase("external")) {
if (internal) {
logger.error(
"ignoring 'external' axiom selector - 'internal' and 'external' together will remove all axioms");
}
filteredAxioms.addAll(
RelatedObjectsHelper.filterExternalAxioms(inputAxioms, baseNamespaces));
external = true;
} else if (axiomSelector.equalsIgnoreCase("tautologies")) {
filteredAxioms.addAll(RelatedObjectsHelper.filterTautologicalAxioms(inputAxioms, false));
} else if (axiomSelector.equalsIgnoreCase("structural-tautologies")) {
filteredAxioms.addAll(RelatedObjectsHelper.filterTautologicalAxioms(inputAxioms, true));
} else {
// Assume this is a normal OWLAxiom type
Set> axiomTypes =
RelatedObjectsHelper.getAxiomValues(axiomSelector);
filteredAxioms.addAll(
filterAxiomsByAxiomType(inputAxioms, objects, axiomTypes, partial, namedOnly));
}
}
return filteredAxioms;
}
/**
* Given a set of OWLAxioms, a set of OWLObjects, a set of OWLAxiom Classes, a partial boolean,
* and a named-only boolean, return a set of OWLAxioms based on OWLAxiom type. The axiom is added
* to the set if it is an instance of an OWLAxiom Class in the axiomTypes set. If partial, return
* any axioms that use at least one object from the objects set. Otherwise, only return axioms
* that use objects in the set. If namedOnly, only consider named OWLObjects in the axioms and
* ignore any anonymous objects when selecting the axioms.
*
* @param axioms set of OWLAxioms to filter
* @param objects set of OWLObjects to get axioms for
* @param axiomTypes set of OWLAxiom Classes that determines what type of axioms will be selected
* @param partial if true, include all axioms that use at least one OWLObject from objects
* @param namedOnly if true, ignore anonymous OWLObjects used in axioms
* @return set of filtered axioms
*/
public static Set filterAxiomsByAxiomType(
Set axioms,
Set objects,
Set> axiomTypes,
boolean partial,
boolean namedOnly) {
if (partial) {
return RelatedObjectsHelper.filterPartialAxioms(axioms, objects, axiomTypes, namedOnly);
} else {
return RelatedObjectsHelper.filterCompleteAxioms(axioms, objects, axiomTypes, namedOnly);
}
}
/**
* Given a st of axioms, a set of objects, and a set of axiom types, return a set of axioms where
* all the objects in those axioms are in the set of objects.
*
* @param inputAxioms Set of OWLAxioms to filter
* @param objects Set of objects to match in axioms
* @param axiomTypes OWLAxiom types to return
* @param namedOnly when true, consider only named OWLObjects
* @return Set of OWLAxioms containing only the OWLObjects
*/
public static Set filterCompleteAxioms(
Set inputAxioms,
Set objects,
Set> axiomTypes,
boolean namedOnly) {
axiomTypes = setDefaultAxiomType(axiomTypes);
if (namedOnly) {
return getCompleteAxiomsNamedOnly(inputAxioms, objects, axiomTypes);
} else {
return getCompleteAxiomsIncludeAnonymous(inputAxioms, objects, axiomTypes);
}
}
/**
* Given a list of base namespaces and a set of axioms, return only the axioms that DO NOT have a
* subject in the base namespaces.
*
* @param axioms set of OWLAxioms
* @param baseNamespaces list of base namespaces
* @return external OWLAxioms
*/
public static Set filterExternalAxioms(
Set axioms, List baseNamespaces) {
Set externalAxioms = new HashSet<>();
for (OWLAxiom axiom : axioms) {
Set subjects = getAxiomSubjects(axiom);
if (!isBase(baseNamespaces, subjects)) {
externalAxioms.add(axiom);
}
}
return externalAxioms;
}
/**
* Given a list of base namespaces and a set of axioms, return only the axioms that have a subject
* in the base namespaces.
*
* @param axioms set of OWLAxioms
* @param baseNamespaces list of base namespaces
* @return internal OWLAxioms
*/
public static Set filterInternalAxioms(
Set axioms, List baseNamespaces) {
Set internalAxioms = new HashSet<>();
for (OWLAxiom axiom : axioms) {
Set subjects = getAxiomSubjects(axiom);
if (isBase(baseNamespaces, subjects)) {
internalAxioms.add(axiom);
}
}
return internalAxioms;
}
/**
* Given a set of axioms, a set of objects, and a set of axiom types, return a set of axioms where
* at least one object in those axioms is also in the set of objects.
*
* @param inputAxioms Set of OWLAxioms to filter
* @param objects Set of OWLObjects to match in axioms
* @param axiomTypes OWLAxiom types to return
* @param namedOnly when true, only consider named OWLObjects
* @return Set of OWLAxioms containing at least one of the OWLObjects
*/
public static Set filterPartialAxioms(
Set inputAxioms,
Set objects,
Set> axiomTypes,
boolean namedOnly) {
axiomTypes = setDefaultAxiomType(axiomTypes);
if (namedOnly) {
return getPartialAxiomsNamedOnly(inputAxioms, objects, axiomTypes);
} else {
return getPartialAxiomsIncludeAnonymous(inputAxioms, objects, axiomTypes);
}
}
/**
* Given a set of OWLAxioms and a structural boolean, filter for tautological axioms, i.e., those
* that would be true in any ontology.
*
* @param axioms set of OWLAxioms to filter
* @param structural if true, only filter for structural tautological axioms based on a hard-coded
* set of patterns (e.g., X subClassOf owl:Thing, owl:Nothing subClassOf X, X subClassOf X)
* @return tautological OWLAxioms from original set
* @throws OWLOntologyCreationException on issue creating empty ontology for tautology checker
*/
public static Set filterTautologicalAxioms(Set axioms, boolean structural)
throws OWLOntologyCreationException {
// If structural, checker will be null
OWLReasoner tautologyChecker = ReasonOperation.getTautologyChecker(structural);
Set tautologies = new HashSet<>();
for (OWLAxiom a : axioms) {
if (ReasonOperation.isTautological(a, tautologyChecker, structural)) {
tautologies.add(a);
}
}
return tautologies;
}
/**
* @deprecated replaced by {@link #filterAxiomsByAxiomType(Set, Set, Set, boolean, boolean)}
* @param ontology OWLOntology to get axioms from
* @param objects OWLObjects to get axioms about
* @param axiomTypes Set of OWLAxiom Classes that are the types of OWLAxioms to return
* @param partial if true, return any OWLAxiom that has at least one OWLObject from objects
* @param signature if true, ignore anonymous OWLObjects in axioms
* @return axioms from ontology based on options
*/
@Deprecated
public static Set getAxioms(
OWLOntology ontology,
Set objects,
Set> axiomTypes,
boolean partial,
boolean signature) {
if (partial) {
return RelatedObjectsHelper.getPartialAxioms(ontology, objects, axiomTypes, signature);
} else {
return RelatedObjectsHelper.getCompleteAxioms(ontology, objects, axiomTypes, signature);
}
}
/**
* Given a string axiom selector, return the OWLAxiom Class type(s) or null. The selector is
* either a special keyword that represents a set of axiom classes or the name of an OWLAxiom
* Class.
*
* @param axiomSelector string option to get the OWLAxiom Class type or types for
* @return set of OWLAxiom Class types based on the string option
*/
public static Set> getAxiomValues(String axiomSelector) {
Set ignore =
new HashSet<>(
Arrays.asList("internal", "external", "tautologies", "structural-tautologies"));
if (ignore.contains(axiomSelector)) {
// Ignore special axiom options
return null;
}
Set> axiomTypes = new HashSet<>();
if (axiomSelector.equalsIgnoreCase("all")) {
axiomTypes.add(OWLAxiom.class);
} else if (axiomSelector.equalsIgnoreCase("logical")) {
axiomTypes.add(OWLLogicalAxiom.class);
} else if (axiomSelector.equalsIgnoreCase("annotation")) {
axiomTypes.add(OWLAnnotationAxiom.class);
} else if (axiomSelector.equalsIgnoreCase("subclass")) {
axiomTypes.add(OWLSubClassOfAxiom.class);
} else if (axiomSelector.equalsIgnoreCase("subproperty")) {
axiomTypes.add(OWLSubObjectPropertyOfAxiom.class);
axiomTypes.add(OWLSubDataPropertyOfAxiom.class);
axiomTypes.add(OWLSubAnnotationPropertyOfAxiom.class);
} else if (axiomSelector.equalsIgnoreCase("equivalent")) {
axiomTypes.add(OWLEquivalentClassesAxiom.class);
axiomTypes.add(OWLEquivalentObjectPropertiesAxiom.class);
axiomTypes.add(OWLEquivalentDataPropertiesAxiom.class);
} else if (axiomSelector.equalsIgnoreCase("disjoint")) {
axiomTypes.add(OWLDisjointClassesAxiom.class);
axiomTypes.add(OWLDisjointObjectPropertiesAxiom.class);
axiomTypes.add(OWLDisjointDataPropertiesAxiom.class);
axiomTypes.add(OWLDisjointUnionAxiom.class);
} else if (axiomSelector.equalsIgnoreCase("type")) {
axiomTypes.add(OWLClassAssertionAxiom.class);
} else if (axiomSelector.equalsIgnoreCase("abox")) {
axiomTypes.addAll(
AxiomType.ABoxAxiomTypes.stream()
.map(AxiomType::getActualClass)
.collect(Collectors.toSet()));
} else if (axiomSelector.equalsIgnoreCase("tbox")) {
axiomTypes.addAll(
AxiomType.TBoxAxiomTypes.stream()
.map(AxiomType::getActualClass)
.collect(Collectors.toSet()));
} else if (axiomSelector.equalsIgnoreCase("rbox")) {
axiomTypes.addAll(
AxiomType.RBoxAxiomTypes.stream()
.map(AxiomType::getActualClass)
.collect(Collectors.toSet()));
} else if (axiomSelector.equalsIgnoreCase("declaration")) {
axiomTypes.add(OWLDeclarationAxiom.class);
} else {
AxiomType> at = AxiomType.getAxiomType(axiomSelector);
if (at != null) {
// Attempt to get the axiom type based on AxiomType names
axiomTypes.add(at.getActualClass());
} else {
throw new IllegalArgumentException(String.format(axiomTypeError, axiomSelector));
}
}
return axiomTypes;
}
/**
* Given an ontology, a set of objects, and a set of axiom types, return a set of axioms where all
* the objects in those axioms are in the set of objects. Prefer {@link #filterCompleteAxioms(Set,
* Set, Set, boolean)}.
*
* @param ontology OWLOntology to get axioms from
* @param objects Set of objects to match in axioms
* @param axiomTypes OWLAxiom types to return
* @return Set of OWLAxioms containing only the OWLObjects
*/
public static Set getCompleteAxioms(
OWLOntology ontology, Set objects, Set> axiomTypes) {
return filterCompleteAxioms(ontology.getAxioms(), objects, axiomTypes, false);
}
/**
* Given an ontology, a set of objects, and a set of axiom types, return a set of axioms where all
* the objects in those axioms are in the set of objects. Prefer {@link #filterCompleteAxioms(Set,
* Set, Set, boolean)}.
*
* @param ontology OWLOntology to get axioms from
* @param objects Set of objects to match in axioms
* @param axiomTypes OWLAxiom types to return
* @param namedOnly when true, consider only named OWLObjects
* @return Set of OWLAxioms containing only the OWLObjects
*/
public static Set getCompleteAxioms(
OWLOntology ontology,
Set objects,
Set> axiomTypes,
boolean namedOnly) {
return filterCompleteAxioms(ontology.getAxioms(), objects, axiomTypes, namedOnly);
}
/**
* Given an ontology, a set of objects, and a set of axiom types, return a set of axioms where at
* least one object in those axioms is also in the set of objects. Prefer {@link
* #filterPartialAxioms(Set, Set, Set, boolean)}.
*
* @param ontology OWLOntology to get axioms from
* @param objects Set of objects to match in axioms
* @param axiomTypes OWLAxiom types to return
* @return Set of OWLAxioms containing at least one of the OWLObjects
*/
public static Set getPartialAxioms(
OWLOntology ontology, Set objects, Set> axiomTypes) {
return filterPartialAxioms(ontology.getAxioms(), objects, axiomTypes, false);
}
/**
* Given an ontology, a set of objects, and a set of axiom types, return a set of axioms where at
* least one object in those axioms is also in the set of objects. Prefer {@link
* #filterPartialAxioms(Set, Set, Set, boolean)}.
*
* @param ontology OWLOntology to get axioms from
* @param objects Set of objects to match in axioms
* @param axiomTypes OWLAxiom types to return
* @param namedOnly when true, only consider named OWLObjects
* @return Set of OWLAxioms containing at least one of the OWLObjects
*/
public static Set getPartialAxioms(
OWLOntology ontology,
Set objects,
Set> axiomTypes,
boolean namedOnly) {
return filterPartialAxioms(ontology.getAxioms(), objects, axiomTypes, namedOnly);
}
/**
* Given an ontology, a set of objects, and a list of select groups (as lists), return the objects
* related to the set of OWLObjects based on each set of selectors. Each selector group will build
* on the previous group.
*
* @param ontology OWLOntology to get related objects from
* @param ioHelper IOHelper to use for prefixes
* @param objects Set of objects to start with
* @param selectorGroups types of related objects to return
* @return Set of related objects
* @throws Exception on annotation pattern issue
*/
public static Set selectGroups(
OWLOntology ontology,
IOHelper ioHelper,
Set objects,
List> selectorGroups)
throws Exception {
for (List selectors : selectorGroups) {
objects = select(ontology, ioHelper, objects, selectors);
}
return objects;
}
/**
* Given an ontology, a set of objects, and a list of selector strings, return the objects related
* to the set of OWLObjects based on the set of selectors.
*
* @param ontology OWLOntology to get related objects from
* @param ioHelper IOHelper to use for prefixes
* @param objects Set of objects to start with
* @param selectors types of related objects to return
* @return Set of related objects
* @throws Exception on annotation pattern issue
*/
public static Set select(
OWLOntology ontology, IOHelper ioHelper, Set objects, List selectors)
throws Exception {
// An empty list is essentially "self"
if (selectors.isEmpty()) {
return objects;
}
Set union = new HashSet<>();
for (String selector : selectors) {
union.addAll(select(ontology, ioHelper, objects, selector));
}
return union;
}
/**
* Given an ontology, a set of objects, and a selector, return the related objects related to the
* set of OWL objects based on the selector.
*
* @param ontology OWLOntology to get related objects from
* @param ioHelper IOHelper to use for prefixes
* @param objects Set of objects to start with
* @param selector type of related objects to return
* @return Set of related objects
* @throws Exception on annotation pattern issue
*/
public static Set select(
OWLOntology ontology, IOHelper ioHelper, Set objects, String selector)
throws Exception {
if (selector.equals("ancestors")) {
return selectAncestors(ontology, objects);
} else if (selector.equals("anonymous")) {
return selectAnonymous(objects);
} else if (selector.equals("annotation-properties")) {
return selectAnnotationProperties(objects);
} else if (selector.equals("children")) {
return selectChildren(ontology, objects);
} else if (selector.equals("classes")) {
return selectClasses(objects);
} else if (selector.equals("complement")) {
return selectComplement(ontology, objects);
} else if (selector.equals("data-properties")) {
return selectDataProperties(objects);
} else if (selector.equals("descendants")) {
return selectDescendants(ontology, objects);
} else if (selector.equals("equivalents")) {
return selectEquivalents(ontology, objects);
} else if (selector.equals("individuals")) {
return selectIndividuals(objects);
} else if (selector.equals("instances")) {
return selectInstances(ontology, objects);
} else if (selector.equals("named")) {
return selectNamed(objects);
} else if (selector.equals("object-properties")) {
return selectObjectProperties(objects);
} else if (selector.equals("parents")) {
return selectParents(ontology, objects);
} else if (selector.equals("properties")) {
return selectProperties(objects);
} else if (selector.equals("self")) {
return objects;
} else if (selector.equals("types")) {
return selectTypes(ontology, objects);
} else if (selector.equals("ranges")) {
return selectRanges(ontology, objects);
} else if (selector.equals("domains")) {
return selectDomains(ontology, objects);
} else if (selector.contains("=")) {
return selectPattern(ontology, ioHelper, objects, selector);
} else if (Pattern.compile("<.*>").matcher(selector).find()) {
return selectIRI(objects, selector);
} else if (selector.contains(":")) {
return selectCURIE(ioHelper, objects, selector);
} else {
logger.error(String.format("%s is not a valid selector and will be ignored", selector));
return new HashSet<>();
}
}
/**
* Given an ontology and a set of objects, return all ancestors (recursively) of those objects.
*
* @param ontology OWLOntology to retrieve ancestors from
* @param objects OWLObjects to retrieve ancestors of
* @return set of ancestors of the starting set
*/
public static Set selectAncestors(OWLOntology ontology, Set objects) {
Set relatedObjects = new HashSet<>();
for (OWLObject object : objects) {
if (object instanceof OWLClass) {
selectClassAncestors(ontology, (OWLClass) object, relatedObjects);
} else if (object instanceof OWLAnnotationProperty) {
selectAnnotationPropertyAncestors(ontology, (OWLAnnotationProperty) object, relatedObjects);
} else if (object instanceof OWLDataProperty) {
selectDataPropertyAncestors(ontology, (OWLDataProperty) object, relatedObjects);
} else if (object instanceof OWLObjectProperty) {
selectObjectPropertyAncestors(ontology, (OWLObjectProperty) object, relatedObjects);
}
}
return relatedObjects;
}
/**
* Given a set of objects, return a set of annotation properties from the starting set.
*
* @param objects Set of OWLObjects to filter
* @return subset of OWLAnnotationProperties from the starting set
*/
public static Set selectAnnotationProperties(Set objects) {
Set relatedObjects = new HashSet<>();
for (OWLObject object : objects) {
if (object instanceof OWLAnnotationProperty) {
relatedObjects.add(object);
}
}
return relatedObjects;
}
/**
* Given a set of objects, return a set of anonymous objects from the starting set. Valid for
* classes and individuals.
*
* @param objects Set of OWLObjects to filter
* @return subset of anonymous objects from the starting set
*/
public static Set selectAnonymous(Set objects) {
Set relatedObjects = new HashSet<>();
for (OWLObject object : objects) {
if ((object instanceof OWLAnonymousClassExpression)
|| (object instanceof OWLAnonymousIndividual)) {
relatedObjects.add(object);
}
}
return relatedObjects;
}
/**
* Given an ontology and a set of objects, return the children of the starting set.
*
* @param ontology OWLOntology to retrieve children from
* @param objects Set of OWLObjects to get children of
* @return set of children of the starting set
*/
public static Set selectChildren(OWLOntology ontology, Set objects) {
Set relatedObjects = new HashSet<>();
for (OWLObject object : objects) {
if (object instanceof OWLClass) {
relatedObjects.addAll(EntitySearcher.getSubClasses((OWLClass) object, ontology));
} else if (object instanceof OWLObjectProperty) {
relatedObjects.addAll(
EntitySearcher.getSubProperties((OWLObjectProperty) object, ontology));
} else if (object instanceof OWLDataProperty) {
relatedObjects.addAll(EntitySearcher.getSubProperties((OWLDataProperty) object, ontology));
} else if (object instanceof OWLAnnotation) {
relatedObjects.addAll(
EntitySearcher.getSubProperties((OWLAnnotationProperty) object, ontology));
}
}
return relatedObjects;
}
/**
* Given a set of objects, return a set of OWLClasses from the starting set.
*
* @param objects Set of OWLObjects to filter
* @return subset of OWLClasses from the starting set
*/
public static Set selectClasses(Set objects) {
Set relatedObjects = new HashSet<>();
for (OWLObject object : objects) {
if (object instanceof OWLClass) {
relatedObjects.add(object);
}
}
return relatedObjects;
}
/**
* Given an ontology and a set of objects, return all objects contained in the ontology that are
* NOT in the starting set.
*
* @param ontology OWLOntology to retrieve complement objects from
* @param objects Set of OWLObjects to get the complement of
* @return complement set of OWLObjects
*/
public static Set selectComplement(OWLOntology ontology, Set objects) {
Set relatedObjects = new HashSet<>();
for (OWLAxiom axiom : ontology.getAxioms()) {
relatedObjects.addAll(OntologyHelper.getObjects(axiom));
}
relatedObjects.removeAll(objects);
return relatedObjects;
}
/**
* Given an IOHelper, a set of objects, and a selector string for CURIE pattern, return a set of
* objects matching that CURIE pattern.
*
* @param ioHelper the IOHelper to resolve names
* @param objects the set of objects to filter
* @param selector the CURIE selector string
* @return the subset of objects that match the CURIE selector
*/
public static Set selectCURIE(
IOHelper ioHelper, Set objects, String selector) {
String prefix = selector.split(":")[0];
String pattern = selector.split(":")[1];
Map prefixes = ioHelper.getPrefixes();
String namespace = prefixes.getOrDefault(prefix, null);
if (namespace == null) {
logger.error(String.format("Prefix '%s' is not a loaded prefix and will be ignored", prefix));
return objects;
}
String iriPattern = namespace + pattern;
return selectIRI(objects, iriPattern);
}
/**
* Given a set of objects, return a set of OWLDataProperties from the starting set.
*
* @param objects Set of OWLObjects to filter
* @return subset of OWLDataProperties from the starting set
*/
public static Set selectDataProperties(Set objects) {
Set relatedObjects = new HashSet<>();
for (OWLObject object : objects) {
if (object instanceof OWLDataProperty) {
relatedObjects.add(object);
}
}
return relatedObjects;
}
/**
* Given an ontology and a set of objects, return all descendants (recursively) of those objects.
*
* @param ontology OWLOntology to retrieve descendants from
* @param objects Set of OWLObjects to get descendants of
* @return set of descendants of the starting set
*/
public static Set selectDescendants(OWLOntology ontology, Set objects) {
Set relatedObjects = new HashSet<>();
for (OWLObject object : objects) {
if (object instanceof OWLClass) {
selectClassDescendants(ontology, (OWLClass) object, relatedObjects);
} else if (object instanceof OWLAnnotationProperty) {
selectAnnotationPropertyDescendants(
ontology, (OWLAnnotationProperty) object, relatedObjects);
} else if (object instanceof OWLDataProperty) {
selectDataPropertyDescendants(ontology, (OWLDataProperty) object, relatedObjects);
} else if (object instanceof OWLObjectProperty) {
selectObjectPropertyDescendants(ontology, (OWLObjectProperty) object, relatedObjects);
}
}
return relatedObjects;
}
/**
* Given an ontology and a set of objects, return all domains of any properties in the set.
*
* @param ontology OWLOntology to retrieve domains from
* @param objects OWLObjects to retrieve domains of
* @return set of domains of the starting set
*/
public static Set selectDomains(OWLOntology ontology, Set objects) {
Set relatedObjects = new HashSet<>();
for (OWLObject object : objects) {
if (object instanceof OWLAnnotationProperty) {
relatedObjects.addAll(EntitySearcher.getDomains((OWLAnnotationProperty) object, ontology));
} else if (object instanceof OWLDataProperty) {
relatedObjects.addAll(EntitySearcher.getDomains((OWLDataProperty) object, ontology));
} else if (object instanceof OWLObjectProperty) {
relatedObjects.addAll(EntitySearcher.getDomains((OWLObjectProperty) object, ontology));
}
}
return relatedObjects;
}
/**
* Given an ontology and a set of objects, return all equivalent objects of the starting set.
*
* @param ontology OWLOntology to retrieve equivalents from
* @param objects Set of OWLObjects to get equivalents of
* @return set of equivalent OWLObjects
*/
public static Set selectEquivalents(OWLOntology ontology, Set objects) {
Set relatedObjects = new HashSet<>();
for (OWLObject object : objects) {
if (object instanceof OWLClass) {
relatedObjects.addAll(EntitySearcher.getEquivalentClasses((OWLClass) object, ontology));
} else if (object instanceof OWLDataProperty) {
relatedObjects.addAll(
EntitySearcher.getEquivalentProperties((OWLDataProperty) object, ontology));
} else if (object instanceof OWLObjectProperty) {
relatedObjects.addAll(
EntitySearcher.getEquivalentProperties((OWLObjectProperty) object, ontology));
}
}
return relatedObjects;
}
/**
* Given a set of objects, return a set of OWLIndividuals from the starting set.
*
* @param objects Set of OWLObjects to filter
* @return subset of OWLIndividuals from the starting set
*/
public static Set selectIndividuals(Set objects) {
Set relatedObjects = new HashSet<>();
for (OWLObject object : objects) {
if (object instanceof OWLIndividual) {
relatedObjects.add(object);
}
}
return relatedObjects;
}
/**
* Given an ontology and a set of objects, select all the instances of the classes in the set of
* objects.
*
* @param ontology OWLOntology to retrieve instances from
* @param objects Set of OWLObjects to get instances of
* @return set of instances as OWLObjects
*/
public static Set selectInstances(OWLOntology ontology, Set objects) {
Set relatedObjects = new HashSet<>();
for (OWLObject object : objects) {
if (object instanceof OWLClass) {
relatedObjects.addAll(EntitySearcher.getIndividuals((OWLClass) object, ontology));
}
}
return relatedObjects;
}
/**
* Given a set of objects, and a selector string (IRI pattern in angle brackets), select all the
* objects that have an IRI matching that pattern.
*
* @param objects Set of OWLObjects to look through
* @param selector IRI pattern to match (enclosed in angle brackets, using ? or * wildcards)
* @return set of IRIs that match the selector pattern
*/
public static Set selectIRI(Set objects, String selector) {
Set relatedObjects = new HashSet<>();
// Check that we're matching a pattern here
// --term should be used for full IRIs
if (!selector.contains("~") && !selector.contains("*") && !selector.contains("?")) {
throw new IllegalArgumentException(String.format(invalidIRIPatternError, selector));
}
// Get rid of brackets
if (selector.startsWith("<") && selector.endsWith(">")) {
selector = selector.substring(1, selector.length() - 1);
}
if (!selector.startsWith("~")) {
// Transform wildcards into regex patterns and escape periods
selector = selector.replace(".", "\\.").replace("?", ".?").replace("*", ".*");
} else {
// Otherwise, it is already a regex pattern
// Just remove the tilde
selector = selector.substring(1);
}
Pattern pattern = Pattern.compile(selector);
for (OWLObject object : objects) {
if (object instanceof OWLEntity) {
OWLEntity entity = (OWLEntity) object;
// Determine if IRI matches the pattern
String iri = entity.getIRI().toString();
if (pattern.matcher(iri).matches()) {
relatedObjects.add(object);
}
}
}
return relatedObjects;
}
/**
* Given a set of objects, return a set of named objects from the starting set.
*
* @param objects Set of OWLObjects to filter
* @return subset of named objects from the starting set
*/
public static Set selectNamed(Set objects) {
Set relatedObjects = new HashSet<>();
for (OWLObject object : objects) {
if (object instanceof OWLNamedObject) {
relatedObjects.add(object);
}
}
return relatedObjects;
}
/**
* Given a set of objects, return a set of OWLObjectProperties from the starting set.
*
* @param objects Set of OWLObjects to filter
* @return subset of OWLObjectProperties from the starting set
*/
public static Set selectObjectProperties(Set objects) {
Set relatedObjects = new HashSet<>();
for (OWLObject object : objects) {
if (object instanceof OWLObjectProperty) {
relatedObjects.add(object);
}
}
return relatedObjects;
}
/**
* Given an ontology and a set of objects, return the parent objects of all objects in the set.
*
* @param ontology OWLOntology to retrieve parents from
* @param objects set of OWLObjects to get parents of
* @return set of parent objects
*/
public static Set selectParents(OWLOntology ontology, Set objects) {
Set relatedObjects = new HashSet<>();
for (OWLObject object : objects) {
if (object instanceof OWLClass) {
relatedObjects.addAll(EntitySearcher.getSuperClasses((OWLClass) object, ontology));
} else if (object instanceof OWLObjectProperty) {
relatedObjects.addAll(
EntitySearcher.getSuperProperties((OWLObjectProperty) object, ontology));
} else if (object instanceof OWLDataProperty) {
relatedObjects.addAll(
EntitySearcher.getSuperProperties((OWLDataProperty) object, ontology));
} else if (object instanceof OWLAnnotation) {
relatedObjects.addAll(
EntitySearcher.getSuperProperties((OWLAnnotationProperty) object, ontology));
}
}
return relatedObjects;
}
/**
* Given an ontology, a set of objects, and a pattern to match annotations to, return a set of
* objects that are annotated with the matching annotation.
*
* @param ontology OWLOntology to get annotations from
* @param ioHelper IOHelper to use for prefixes
* @param objects Set of OWLObjects to filter
* @param annotationPattern annotation to filter OWLObjects on
* @return subset of OWLObjects matching the annotation pattern
* @throws Exception on issue getting literal annotations
*/
public static Set selectPattern(
OWLOntology ontology, IOHelper ioHelper, Set objects, String annotationPattern)
throws Exception {
Set annotations = getAnnotations(ontology, ioHelper, annotationPattern);
return selectAnnotated(ontology, objects, annotations);
}
/**
* Given a set of objects, return a set of all OWLProperties from the starting set.
*
* @param objects Set of OWLObjects to filter OWLProperties of
* @return subset of OWLProperties from the starting set
*/
public static Set selectProperties(Set objects) {
Set relatedObjects = new HashSet<>();
for (OWLObject object : objects) {
if (object instanceof OWLProperty) {
relatedObjects.add(object);
}
}
return relatedObjects;
}
/**
* Given an ontology and a set of objects, return all ranges of any properties in the set.
*
* @param ontology OWLOntology to retrieve ranges from
* @param objects OWLObjects to retrieve ranges of
* @return set of ranges of the starting set
*/
public static Set selectRanges(OWLOntology ontology, Set objects) {
Set relatedObjects = new HashSet<>();
for (OWLObject object : objects) {
if (object instanceof OWLAnnotationProperty) {
relatedObjects.addAll(EntitySearcher.getRanges((OWLAnnotationProperty) object, ontology));
} else if (object instanceof OWLDataProperty) {
relatedObjects.addAll(EntitySearcher.getRanges((OWLDataProperty) object, ontology));
} else if (object instanceof OWLObjectProperty) {
relatedObjects.addAll(EntitySearcher.getRanges((OWLObjectProperty) object, ontology));
}
}
return relatedObjects;
}
/**
* Given an ontology and a set of objects, return a set of all the types of the individuals in
* that set.
*
* @param ontology OWLOntology to retrieve types from
* @param objects Set of OWLObjects to get the types of
* @return set of types (OWLClass or OWLClassExpression)
*/
public static Set selectTypes(OWLOntology ontology, Set objects) {
Set relatedObjects = new HashSet<>();
for (OWLObject object : objects) {
if (object instanceof OWLIndividual) {
relatedObjects.addAll(EntitySearcher.getTypes((OWLIndividual) object, ontology));
}
}
return relatedObjects;
}
/**
* Given an ontology and a set of objects, construct a set of subClassOf axioms that span the gaps
* between classes to maintain a hierarchy.
*
* @param ontology input OWLOntology
* @param objects set of Objects to build hierarchy
* @return set of OWLAxioms to maintain hierarchy
*/
public static Set spanGaps(OWLOntology ontology, Set objects) {
return spanGaps(ontology, objects, false);
}
/**
* Given an ontology and a set of objects, construct a set of subClassOf axioms that span the gaps
* between classes to maintain a hierarchy.
*
* @param ontology input OWLOntology
* @param objects set of Objects to build hierarchy
* @param excludeAnonymous when true, span across anonymous nodes
* @return set of OWLAxioms to maintain hierarchy
*/
public static Set spanGaps(
OWLOntology ontology, Set objects, boolean excludeAnonymous) {
return spanGaps(ontology, Lists.newArrayList(), objects, excludeAnonymous, false, false);
}
/**
* Given an ontology and a set of objects, construct a set of subClassOf axioms that span the gaps
* between classes to maintain a hierarchy.
*
* @param ontology input OWLOntology
* @param baseNamespaces list of base namespaces as strings
* @param objects set of Objects to build hierarchy
* @param excludeAnonymous when true, span across anonymous nodes
* @param internal when true, only preserve structure between internal nodes defined by base
* namespaces
* @param external when true, only preserve structure between external nodes defined by base
* namespaces
* @return set of OWLAxioms to maintain hierarchy
*/
public static Set spanGaps(
OWLOntology ontology,
List baseNamespaces,
Set objects,
boolean excludeAnonymous,
boolean internal,
boolean external) {
Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy