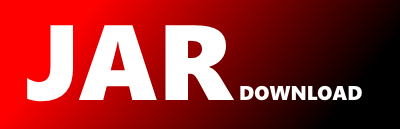
org.ocpsoft.rewrite.util.CompositeMap Maven / Gradle / Ivy
/*
* Copyright 2011 Lincoln Baxter, III
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.ocpsoft.rewrite.util;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.LinkedHashMap;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
* {@link Map} implementation supporting delegation to other {@link Map} instances configured with
* {@link CompositeMap#addDelegate(Map)}.
*
* @author Lincoln Baxter, III
*
* @param
* @param
*/
public class CompositeMap implements Map
{
@SuppressWarnings("unchecked")
private Map[] delegates = new Map[] {};
@SuppressWarnings("unchecked")
public CompositeMap addDelegate(Map delegate)
{
/*
* Highly optimized for performance reasons. Think before you change this and profile after!
*/
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy