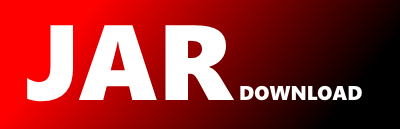
org.ocpsoft.rewrite.param.RegexParameterizedPatternBuilder Maven / Gradle / Ivy
/*
* Copyright 2011 Lincoln Baxter, III
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.ocpsoft.rewrite.param;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashSet;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.regex.Pattern;
import org.ocpsoft.common.util.Assert;
import org.ocpsoft.rewrite.bind.Binding;
import org.ocpsoft.rewrite.context.EvaluationContext;
import org.ocpsoft.rewrite.event.Rewrite;
import org.ocpsoft.rewrite.exception.ParameterizationException;
import org.ocpsoft.rewrite.util.ParameterUtils;
import org.ocpsoft.rewrite.util.ParseTools;
import org.ocpsoft.rewrite.util.ParseTools.CaptureType;
import org.ocpsoft.rewrite.util.ParseTools.CapturingGroup;
/**
* An {@link org.ocpsoft.rewrite.param.Parameterized} regular expression {@link Pattern}.
*
* @author Lincoln Baxter, III
*/
public class RegexParameterizedPatternBuilder implements ParameterizedPatternBuilder
{
private static final String DEFAULT_PARAMETER_PATTERN = ".*";
private final String pattern;
private final char[] chars;
private final List groups = new ArrayList();
private final String defaultParameterPattern;
private ParameterStore parameters;
private RegexParameterizedPatternParser parser = null;
RegexParameterizedPatternBuilder(String pattern, RegexParameterizedPatternParser parser)
{
this(pattern);
this.parser = parser;
}
/**
* Create a new {@link RegexParameterizedPatternBuilder} instance with the default
* {@link org.ocpsoft.rewrite.bind.parse.CaptureType#BRACE} and parameter compiledPattern of ".*".
*/
public RegexParameterizedPatternBuilder(final String pattern)
{
this(CaptureType.BRACE, DEFAULT_PARAMETER_PATTERN, pattern);
}
/**
* Create a new {@link RegexParameterizedPatternBuilder} instance with the default {@link CaptureType#BRACE}.
*/
public RegexParameterizedPatternBuilder(final String parameterPattern, final String pattern)
{
this(CaptureType.BRACE, parameterPattern, pattern);
}
/**
* Create a new {@link RegexParameterizedPatternBuilder} instance with the default parameter regex of ".*".
*/
public RegexParameterizedPatternBuilder(final CaptureType type, final String pattern)
{
this(type, DEFAULT_PARAMETER_PATTERN, pattern);
}
/**
* Create a new {@link RegexParameterizedPatternBuilder} instance.
*/
public RegexParameterizedPatternBuilder(final CaptureType type, final String defaultParameterPattern,
final String pattern)
{
Assert.notNull(pattern, "Pattern must not be null");
this.defaultParameterPattern = defaultParameterPattern;
this.pattern = pattern;
chars = pattern.toCharArray();
if (chars.length > 0)
{
int parameterIndex = 0;
int cursor = 0;
while (cursor < chars.length)
{
switch (chars[cursor])
{
case '{':
int startPos = cursor;
CapturingGroup group = ParseTools.balancedCapture(chars, startPos, chars.length - 1, type);
cursor = group.getEnd();
groups.add(new RegexGroup(group, parameterIndex++));
break;
default:
break;
}
cursor++;
}
}
}
@Override
public String build(final Rewrite event, final EvaluationContext context, final Transposition transposition)
throws ParameterizationException
{
return build(extractBoundValues(event, context, transposition));
}
@Override
public String build(final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy