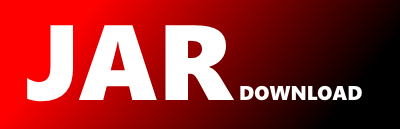
org.odpi.openmetadata.accessservices.governanceprogram.api.RelatedElementsManagementInterface Maven / Gradle / Ivy
/* SPDX-License-Identifier: Apache-2.0 */
/* Copyright Contributors to the ODPi Egeria project. */
package org.odpi.openmetadata.accessservices.governanceprogram.api;
import org.odpi.openmetadata.accessservices.governanceprogram.metadataelements.GovernanceDefinitionElement;
import org.odpi.openmetadata.accessservices.governanceprogram.metadataelements.GovernanceRoleElement;
import org.odpi.openmetadata.accessservices.governanceprogram.metadataelements.RelatedElement;
import org.odpi.openmetadata.accessservices.governanceprogram.properties.AssignmentScopeProperties;
import org.odpi.openmetadata.accessservices.governanceprogram.properties.RelationshipProperties;
import org.odpi.openmetadata.accessservices.governanceprogram.properties.ResourceListProperties;
import org.odpi.openmetadata.accessservices.governanceprogram.properties.StakeholderProperties;
import org.odpi.openmetadata.frameworks.connectors.ffdc.InvalidParameterException;
import org.odpi.openmetadata.frameworks.connectors.ffdc.PropertyServerException;
import org.odpi.openmetadata.frameworks.connectors.ffdc.UserNotAuthorizedException;
import java.util.List;
/**
* Defines the interface that is common to multiple element types
*/
public interface RelatedElementsManagementInterface
{
/**
* Create a "MoreInformation" relationship between an element that is descriptive and one that is providing the detail.
*
* @param userId calling user
* @param elementGUID unique identifier of the element that is descriptive
* @param properties properties of the relationship
* @param detailGUID unique identifier of the element that provides the detail
*
* @throws InvalidParameterException one of the parameters is invalid
* @throws UserNotAuthorizedException the user is not authorized to issue this request
* @throws PropertyServerException there is a problem reported in the open metadata server(s)
*/
void setupMoreInformation(String userId,
String elementGUID,
RelationshipProperties properties,
String detailGUID) throws InvalidParameterException,
UserNotAuthorizedException,
PropertyServerException;
/**
* Remove a "MoreInformation" relationship between two referenceables.
*
* @param userId calling user
* @param elementGUID unique identifier of the element that is descriptive
* @param detailGUID unique identifier of the element that provides the detail
*
* @throws InvalidParameterException one of the parameters is invalid
* @throws UserNotAuthorizedException the user is not authorized to issue this request
* @throws PropertyServerException there is a problem reported in the open metadata server(s)
*/
void clearMoreInformation(String userId,
String elementGUID,
String detailGUID) throws InvalidParameterException,
UserNotAuthorizedException,
PropertyServerException;
/**
* Retrieve the detail elements linked via a "MoreInformation" relationship between two referenceables.
*
* @param userId calling user
* @param elementGUID unique identifier of the element that is descriptive
* @param startFrom index of the list to start from (0 for start)
* @param pageSize maximum number of elements to return.
*
* @return list of related elements
* @throws InvalidParameterException one of the parameters is invalid
* @throws UserNotAuthorizedException the user is not authorized to issue this request
* @throws PropertyServerException there is a problem reported in the open metadata server(s)
*/
List getMoreInformation(String userId,
String elementGUID,
int startFrom,
int pageSize) throws InvalidParameterException,
UserNotAuthorizedException,
PropertyServerException;
/**
* Retrieve the descriptive elements linked via a "MoreInformation" relationship between two referenceables.
*
* @param userId calling user
* @param detailGUID unique identifier of the element that provides the detail
* @param startFrom index of the list to start from (0 for start)
* @param pageSize maximum number of elements to return.
*
* @return list of related elements
* @throws InvalidParameterException one of the parameters is invalid
* @throws UserNotAuthorizedException the user is not authorized to issue this request
* @throws PropertyServerException there is a problem reported in the open metadata server(s)
*/
List getDescriptiveElements(String userId,
String detailGUID,
int startFrom,
int pageSize) throws InvalidParameterException,
UserNotAuthorizedException,
PropertyServerException;
/**
* Create a "GovernedBy" relationship between an element and a governance definition.
*
* @param userId calling user
* @param elementGUID unique identifier of the element that is governed
* @param properties properties of the relationship
* @param governanceDefinitionGUID unique identifier of the element that provides the detail
*
* @throws InvalidParameterException one of the parameters is invalid
* @throws UserNotAuthorizedException the user is not authorized to issue this request
* @throws PropertyServerException there is a problem reported in the open metadata server(s)
*/
void setupGovernedBy(String userId,
String elementGUID,
RelationshipProperties properties,
String governanceDefinitionGUID) throws InvalidParameterException,
UserNotAuthorizedException,
PropertyServerException;
/**
* Remove a "GovernedBy" relationship between two elements.
*
* @param userId calling user
* @param elementGUID unique identifier of the element that is governed
* @param governanceDefinitionGUID unique identifier of the governance definition
*
* @throws InvalidParameterException one of the parameters is invalid
* @throws UserNotAuthorizedException the user is not authorized to issue this request
* @throws PropertyServerException there is a problem reported in the open metadata server(s)
*/
void clearGovernedBy(String userId,
String elementGUID,
String governanceDefinitionGUID) throws InvalidParameterException,
UserNotAuthorizedException,
PropertyServerException;
/**
* Retrieve the governance definitions linked via a "GovernedBy" relationship to an element.
*
* @param userId calling user
* @param elementGUID unique identifier of the element that is descriptive
* @param startFrom index of the list to start from (0 for start)
* @param pageSize maximum number of elements to return.
*
* @return list of related governance definitions
* @throws InvalidParameterException one of the parameters is invalid
* @throws UserNotAuthorizedException the user is not authorized to issue this request
* @throws PropertyServerException there is a problem reported in the open metadata server(s)
*/
List getGovernanceDefinitionsForElement(String userId,
String elementGUID,
int startFrom,
int pageSize) throws InvalidParameterException,
UserNotAuthorizedException,
PropertyServerException;
/**
* Retrieve the governed elements linked via a "GovernedBy" relationship to a governance definition.
*
* @param userId calling user
* @param governanceDefinitionGUID unique identifier of the element that provides the detail
* @param startFrom index of the list to start from (0 for start)
* @param pageSize maximum number of elements to return.
*
* @return list of related elements
* @throws InvalidParameterException one of the parameters is invalid
* @throws UserNotAuthorizedException the user is not authorized to issue this request
* @throws PropertyServerException there is a problem reported in the open metadata server(s)
*/
List getGovernedElements(String userId,
String governanceDefinitionGUID,
int startFrom,
int pageSize) throws InvalidParameterException,
UserNotAuthorizedException,
PropertyServerException;
/**
* Create an "GovernanceDefinitionScope" relationship between a governance definition and its scope element.
*
* @param userId calling user
* @param governanceDefinitionGUID unique identifier of the governance definition
* @param scopeGUID unique identifier of the scope
*
* @throws InvalidParameterException one of the parameters is invalid
* @throws UserNotAuthorizedException the user is not authorized to issue this request
* @throws PropertyServerException there is a problem reported in the open metadata server(s)
*/
void setupGovernanceDefinitionScope(String userId,
String governanceDefinitionGUID,
String scopeGUID) throws InvalidParameterException,
UserNotAuthorizedException,
PropertyServerException;
/**
* Remove an "GovernanceDefinitionScope" relationship between two referenceables.
*
* @param userId calling user
* @param governanceDefinitionGUID unique identifier of the governance definition
* @param scopeGUID unique identifier of the scope
*
* @throws InvalidParameterException one of the parameters is invalid
* @throws UserNotAuthorizedException the user is not authorized to issue this request
* @throws PropertyServerException there is a problem reported in the open metadata server(s)
*/
void clearGovernanceDefinitionScope(String userId,
String governanceDefinitionGUID,
String scopeGUID) throws InvalidParameterException,
UserNotAuthorizedException,
PropertyServerException;
/**
* Retrieve the assigned scopes linked by the "GovernanceDefinitionScope" relationship between two referenceables.
*
* @param userId calling user
* @param governanceDefinitionGUID unique identifier of the governance definition
* @param startFrom index of the list to start from (0 for start)
* @param pageSize maximum number of elements to return.
*
* @return list of related elements
* @throws InvalidParameterException one of the parameters is invalid
* @throws UserNotAuthorizedException the user is not authorized to issue this request
* @throws PropertyServerException there is a problem reported in the open metadata server(s)
*/
List getGovernanceDefinitionScopes(String userId,
String governanceDefinitionGUID,
int startFrom,
int pageSize) throws InvalidParameterException,
UserNotAuthorizedException,
PropertyServerException;
/**
* Retrieve the governance definitions linked by the "GovernanceDefinitionScope" relationship to a scope element.
*
* @param userId calling user
* @param scopeGUID unique identifier of the scope
* @param startFrom index of the list to start from (0 for start)
* @param pageSize maximum number of elements to return.
*
* @return list of related elements
* @throws InvalidParameterException one of the parameters is invalid
* @throws UserNotAuthorizedException the user is not authorized to issue this request
* @throws PropertyServerException there is a problem reported in the open metadata server(s)
*/
List getScopedGovernanceDefinitions(String userId,
String scopeGUID,
int startFrom,
int pageSize) throws InvalidParameterException,
UserNotAuthorizedException,
PropertyServerException;
/**
* Create a "GovernanceResponsibilityAssignment" relationship between a governance responsibility and a person role.
*
* @param userId calling user
* @param governanceResponsibilityGUID unique identifier of the governance responsibility (type of governance definition)
* @param personRoleGUID unique identifier of the person role element
*
* @throws InvalidParameterException one of the parameters is invalid
* @throws UserNotAuthorizedException the user is not authorized to issue this request
* @throws PropertyServerException there is a problem reported in the open metadata server(s)
*/
void setupGovernanceResponsibilityAssignment(String userId,
String governanceResponsibilityGUID,
String personRoleGUID) throws InvalidParameterException,
UserNotAuthorizedException,
PropertyServerException;
/**
* Remove a "GovernanceResponsibilityAssignment" relationship between a governance responsibility and a person role.
*
* @param userId calling user
* @param governanceResponsibilityGUID unique identifier of the governance responsibility (type of governance definition)
* @param personRoleGUID unique identifier of the person role element
*
* @throws InvalidParameterException one of the parameters is invalid
* @throws UserNotAuthorizedException the user is not authorized to issue this request
* @throws PropertyServerException there is a problem reported in the open metadata server(s)
*/
void clearGovernanceResponsibilityAssignment(String userId,
String governanceResponsibilityGUID,
String personRoleGUID) throws InvalidParameterException,
UserNotAuthorizedException,
PropertyServerException;
/**
* Retrieve the person roles linked via a "GovernanceResponsibilityAssignment" relationship to a governance responsibility.
*
* @param userId calling user
* @param governanceResponsibilityGUID unique identifier of the governance responsibility (type of governance definition)
* @param startFrom index of the list to start from (0 for start)
* @param pageSize maximum number of elements to return.
*
* @return list of related elements
* @throws InvalidParameterException one of the parameters is invalid
* @throws UserNotAuthorizedException the user is not authorized to issue this request
* @throws PropertyServerException there is a problem reported in the open metadata server(s)
*/
List getResponsibleRoles(String userId,
String governanceResponsibilityGUID,
int startFrom,
int pageSize) throws InvalidParameterException,
UserNotAuthorizedException,
PropertyServerException;
/**
* Retrieve the governance responsibilities linked via a "GovernanceResponsibilityAssignment" relationship to a person role.
*
* @param userId calling user
* @param personRoleGUID unique identifier of the role
* @param startFrom index of the list to start from (0 for start)
* @param pageSize maximum number of elements to return.
*
* @return list of related elements
* @throws InvalidParameterException one of the parameters is invalid
* @throws UserNotAuthorizedException the user is not authorized to issue this request
* @throws PropertyServerException there is a problem reported in the open metadata server(s)
*/
List getRoleResponsibilities(String userId,
String personRoleGUID,
int startFrom,
int pageSize) throws InvalidParameterException,
UserNotAuthorizedException,
PropertyServerException;
/**
* Create a "Stakeholder" relationship between an element and its stakeholder.
*
* @param userId calling user
* @param elementGUID unique identifier of the element
* @param properties properties of the relationship
* @param stakeholderGUID unique identifier of the stakeholder
*
* @throws InvalidParameterException one of the parameters is invalid
* @throws UserNotAuthorizedException the user is not authorized to issue this request
* @throws PropertyServerException there is a problem reported in the open metadata server(s)
*/
void setupStakeholder(String userId,
String elementGUID,
StakeholderProperties properties,
String stakeholderGUID) throws InvalidParameterException,
UserNotAuthorizedException,
PropertyServerException;
/**
* Remove a "Stakeholder" relationship between two referenceables.
*
* @param userId calling user
* @param elementGUID unique identifier of the element
* @param stakeholderGUID unique identifier of the stakeholder
*
* @throws InvalidParameterException one of the parameters is invalid
* @throws UserNotAuthorizedException the user is not authorized to issue this request
* @throws PropertyServerException there is a problem reported in the open metadata server(s)
*/
void clearStakeholder(String userId,
String elementGUID,
String stakeholderGUID) throws InvalidParameterException,
UserNotAuthorizedException,
PropertyServerException;
/**
* Retrieve the stakeholder elements linked via the "Stakeholder" relationship between two referenceables.
*
* @param userId calling user
* @param elementGUID unique identifier of the element
* @param startFrom index of the list to start from (0 for start)
* @param pageSize maximum number of elements to return.
*
* @return list of related elements
* @throws InvalidParameterException one of the parameters is invalid
* @throws UserNotAuthorizedException the user is not authorized to issue this request
* @throws PropertyServerException there is a problem reported in the open metadata server(s)
*/
List getStakeholders(String userId,
String elementGUID,
int startFrom,
int pageSize) throws InvalidParameterException,
UserNotAuthorizedException,
PropertyServerException;
/**
* Retrieve the elements commissioned by a stakeholder, linked via the "Stakeholder" relationship between two referenceables.
*
* @param userId calling user
* @param stakeholderGUID unique identifier of the stakeholder
* @param startFrom index of the list to start from (0 for start)
* @param pageSize maximum number of elements to return.
*
* @return list of related elements
* @throws InvalidParameterException one of the parameters is invalid
* @throws UserNotAuthorizedException the user is not authorized to issue this request
* @throws PropertyServerException there is a problem reported in the open metadata server(s)
*/
List getStakeholderCommissionedElements(String userId,
String stakeholderGUID,
int startFrom,
int pageSize) throws InvalidParameterException,
UserNotAuthorizedException,
PropertyServerException;
/**
* Create an "AssignmentScope" relationship between an element and its scope.
*
* @param userId calling user
* @param elementGUID unique identifier of the element
* @param properties properties of the relationship
* @param scopeGUID unique identifier of the scope
*
* @throws InvalidParameterException one of the parameters is invalid
* @throws UserNotAuthorizedException the user is not authorized to issue this request
* @throws PropertyServerException there is a problem reported in the open metadata server(s)
*/
void setupAssignmentScope(String userId,
String elementGUID,
AssignmentScopeProperties properties,
String scopeGUID) throws InvalidParameterException,
UserNotAuthorizedException,
PropertyServerException;
/**
* Remove an "AssignmentScope" relationship between two referenceables.
*
* @param userId calling user
* @param elementGUID unique identifier of the element
* @param scopeGUID unique identifier of the scope
*
* @throws InvalidParameterException one of the parameters is invalid
* @throws UserNotAuthorizedException the user is not authorized to issue this request
* @throws PropertyServerException there is a problem reported in the open metadata server(s)
*/
void clearAssignmentScope(String userId,
String elementGUID,
String scopeGUID) throws InvalidParameterException,
UserNotAuthorizedException,
PropertyServerException;
/**
* Retrieve the assigned scopes linked by the "AssignmentScope" relationship between two referenceables.
*
* @param userId calling user
* @param elementGUID unique identifier of the element
* @param startFrom index of the list to start from (0 for start)
* @param pageSize maximum number of elements to return.
*
* @return list of related elements
* @throws InvalidParameterException one of the parameters is invalid
* @throws UserNotAuthorizedException the user is not authorized to issue this request
* @throws PropertyServerException there is a problem reported in the open metadata server(s)
*/
List getAssignedScopes(String userId,
String elementGUID,
int startFrom,
int pageSize) throws InvalidParameterException,
UserNotAuthorizedException,
PropertyServerException;
/**
* Retrieve the assigned actors linked by the "AssignmentScope" relationship between two referenceables.
*
* @param userId calling user
* @param scopeGUID unique identifier of the scope
* @param startFrom index of the list to start from (0 for start)
* @param pageSize maximum number of elements to return.
*
* @return list of related elements
* @throws InvalidParameterException one of the parameters is invalid
* @throws UserNotAuthorizedException the user is not authorized to issue this request
* @throws PropertyServerException there is a problem reported in the open metadata server(s)
*/
List getAssignedActors(String userId,
String scopeGUID,
int startFrom,
int pageSize) throws InvalidParameterException,
UserNotAuthorizedException,
PropertyServerException;
/**
* Create a "ResourceList" relationship between a consuming element and an element that represents resources.
*
* @param userId calling user
* @param elementGUID unique identifier of the element
* @param properties properties of the relationship
* @param resourceGUID unique identifier of the resource
*
* @throws InvalidParameterException one of the parameters is invalid
* @throws UserNotAuthorizedException the user is not authorized to issue this request
* @throws PropertyServerException there is a problem reported in the open metadata server(s)
*/
void setupResource(String userId,
String elementGUID,
ResourceListProperties properties,
String resourceGUID) throws InvalidParameterException,
UserNotAuthorizedException,
PropertyServerException;
/**
* Remove a "ResourceList" relationship between two referenceables.
*
* @param userId calling user
* @param elementGUID unique identifier of the element
* @param resourceGUID unique identifier of the resource
*
* @throws InvalidParameterException one of the parameters is invalid
* @throws UserNotAuthorizedException the user is not authorized to issue this request
* @throws PropertyServerException there is a problem reported in the open metadata server(s)
*/
void clearResource(String userId,
String elementGUID,
String resourceGUID) throws InvalidParameterException,
UserNotAuthorizedException,
PropertyServerException;
/**
* Retrieve the list of resources assigned to an element via the "ResourceList" relationship between two referenceables.
*
* @param userId calling user
* @param elementGUID unique identifier of the element
* @param startFrom index of the list to start from (0 for start)
* @param pageSize maximum number of elements to return.
*
* @return list of related elements
* @throws InvalidParameterException one of the parameters is invalid
* @throws UserNotAuthorizedException the user is not authorized to issue this request
* @throws PropertyServerException there is a problem reported in the open metadata server(s)
*/
List getResourceList(String userId,
String elementGUID,
int startFrom,
int pageSize) throws InvalidParameterException,
UserNotAuthorizedException,
PropertyServerException;
/**
* Retrieve the list of elements assigned to a resource via the "ResourceList" relationship between two referenceables.
*
* @param userId calling user
* @param resourceGUID unique identifier of the resource
* @param startFrom index of the list to start from (0 for start)
* @param pageSize maximum number of elements to return.
*
* @return list of related elements
* @throws InvalidParameterException one of the parameters is invalid
* @throws UserNotAuthorizedException the user is not authorized to issue this request
* @throws PropertyServerException there is a problem reported in the open metadata server(s)
*/
List getSupportedByResource(String userId,
String resourceGUID,
int startFrom,
int pageSize) throws InvalidParameterException,
UserNotAuthorizedException,
PropertyServerException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy