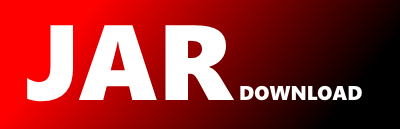
org.gpel.GpelUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gpel-client Show documentation
Show all versions of gpel-client Show documentation
Grid BPEL Engine Client developed by Extreme Computing Lab, Indiana University
The newest version!
/* -*- mode: Java; c-basic-offset: 4; indent-tabs-mode: nil; -*- //------100-columns-wide------>|*/
/*
* Copyright (c) 2004-2005 Extreme! Lab, Indiana University. All rights reserved.
*
* This software is open source. See the bottom of this file for the licence.
*
* $Id: GpelUtil.java,v 1.3 2006/11/20 05:50:42 aslom Exp $
*/
package org.gpel;
import org.gpel.GpelConstants;
import org.gpel.logger.GLogger;
import org.xmlpull.infoset.XmlInfosetBuilder;
public class GpelUtil {
private final static GLogger logger = GLogger.getLogger();
private final static XmlInfosetBuilder builder = GpelConstants.BUILDER;
// /**
// * Will serialize XML and call toString() on unrecognized objects
// */
// public static String safeXmlToString(List xmlContainers) { //JDK15 List<>
// StringBuffer sb = new StringBuffer();
// for (Iterator i = xmlContainers.iterator(); i.hasNext(); )
// {
// Object child = i.next();
// // if(child instanceof XmlContainer) {
// // XmlContainer xc = (XmlContainer) child;
// // String xml = safeXmlToString(xc);
// // }
// String xml = safeSerializeXmlItem(child);
// sb.append(xml);
// }
// return sb.toString();
// }
//
// public static String safeXmlToString(XmlContainer xc) {
// return safeSerializeXmlItem(xc);
// }
//
// public static String safeXmlToString(XmlElement el) {
// return safeSerializeXmlItem(el);
// }
//
// public static String safeSerializeXmlItem(Object item) {
// StringWriter sw = new StringWriter();
// XmlSerializer ser = preapareSerializer(sw);
// try {
// safeSerializeXmlItem(item, ser);
// } catch (IOException e) {
// throw new XmlBuilderException("could not serialize output", e);
// }
// try {
// ser.flush();
// } catch (IOException e) {
// throw new XmlBuilderException("could not flush output", e);
// }
// return sw.toString();
// }
//
// private static XmlSerializer preapareSerializer(StringWriter sw)
// throws XmlBuilderException
// {
// XmlSerializer ser;
// try {
// ser = builder.getFactory().newSerializer();
// ser.setOutput(sw);
// } catch (Exception e) {
// throw new XmlBuilderException("could not serialize node to writer", e);
// }
// return ser;
// }
//
// public static void safeSerializeXmlElement(XmlElement el, XmlSerializer ser) throws IOException {
// builder.serializeStartTag(el, ser);
// //now do children recurson - bit only if they are recognized safe to serialize
// if(el.hasChildren()) {
// Iterator iter = el.children();
// while (iter.hasNext())
// {
// Object child = iter.next();
// safeSerializeXmlItem(child, ser);
// }
// }
// builder.serializeEndTag(el, ser);
// }
//
// /**
// * It is safe serialization in sense that non XML serializable content is represnted as toString()
// * and no exception is thrown (so one can even print Thread to XML).
// *
NOTE: use this and related methods for debuggin purposes only!!!
// *
NOTE: this method is not suitable to generate correct XML
// * (output from toString() may be hard or impossible to deserialize !!!!)
// */
// public static void safeSerializeXmlItem(Object child, XmlSerializer ser)
// throws IllegalArgumentException, IllegalStateException, IOException, XmlBuilderException
// {
// if(child instanceof XmlSerializable) {
// //((XmlSerializable)child).serialize(ser);
// try {
// ((XmlSerializable)child).serialize(ser);
// } catch (IOException e) {
// throw new XmlBuilderException("could not serialize item "+child+": "+e, e);
// }
//
// } else if(child instanceof XmlElement) {
// safeSerializeXmlElement((XmlElement)child, ser);
// } else if(child instanceof XmlDocument) {
// // ignore prolog
// XmlElement el = ((XmlDocument) child).getDocumentElement();
// safeSerializeXmlElement(el, ser);
// } else if(child instanceof String) {
// ser.text(child.toString());
// } else if(child instanceof XmlComment) {
// ser.comment(((XmlComment)child).getContent());
// } else {
// //throw new IllegalArgumentException("could not serialize "+child.getClass());
// ser.text(child != null ? child.toString() : "null"); //TODO revisit
// }
// }
}
/*
* Indiana University Extreme! Lab Software License, Version 1.2
*
* Copyright (c) 2004-2005 The Trustees of Indiana University.
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are
* met:
*
* 1) All redistributions of source code must retain the above
* copyright notice, the list of authors in the original source
* code, this list of conditions and the disclaimer listed in this
* license;
*
* 2) All redistributions in binary form must reproduce the above
* copyright notice, this list of conditions and the disclaimer
* listed in this license in the documentation and/or other
* materials provided with the distribution;
*
* 3) Any documentation included with all redistributions must include
* the following acknowledgement:
*
* "This product includes software developed by the Indiana
* University Extreme! Lab. For further information please visit
* http://www.extreme.indiana.edu/"
*
* Alternatively, this acknowledgment may appear in the software
* itself, and wherever such third-party acknowledgments normally
* appear.
*
* 4) The name "Indiana University" or "Indiana University
* Extreme! Lab" shall not be used to endorse or promote
* products derived from this software without prior written
* permission from Indiana University. For written permission,
* please contact http://www.extreme.indiana.edu/.
*
* 5) Products derived from this software may not use "Indiana
* University" name nor may "Indiana University" appear in their name,
* without prior written permission of the Indiana University.
*
* Indiana University provides no reassurances that the source code
* provided does not infringe the patent or any other intellectual
* property rights of any other entity. Indiana University disclaims any
* liability to any recipient for claims brought by any other entity
* based on infringement of intellectual property rights or otherwise.
*
* LICENSEE UNDERSTANDS THAT SOFTWARE IS PROVIDED "AS IS" FOR WHICH
* NO WARRANTIES AS TO CAPABILITIES OR ACCURACY ARE MADE. INDIANA
* UNIVERSITY GIVES NO WARRANTIES AND MAKES NO REPRESENTATION THAT
* SOFTWARE IS FREE OF INFRINGEMENT OF THIRD PARTY PATENT, COPYRIGHT, OR
* OTHER PROPRIETARY RIGHTS. INDIANA UNIVERSITY MAKES NO WARRANTIES THAT
* SOFTWARE IS FREE FROM "BUGS", "VIRUSES", "TROJAN HORSES", "TRAP
* DOORS", "WORMS", OR OTHER HARMFUL CODE. LICENSEE ASSUMES THE ENTIRE
* RISK AS TO THE PERFORMANCE OF SOFTWARE AND/OR ASSOCIATED MATERIALS,
* AND TO THE PERFORMANCE AND VALIDITY OF INFORMATION GENERATED USING
* SOFTWARE.
*/
© 2015 - 2024 Weber Informatics LLC | Privacy Policy