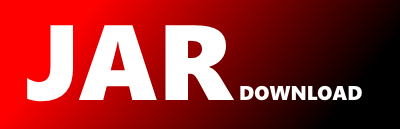
org.gpel.client.GcAtomResource Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gpel-client Show documentation
Show all versions of gpel-client Show documentation
Grid BPEL Engine Client developed by Extreme Computing Lab, Indiana University
The newest version!
/* -*- mode: Java; c-basic-offset: 4; indent-tabs-mode: nil; -*- //------100-columns-wide------>|*/
/* Copyright (c) 2005 Extreme! Lab, Indiana University. All rights reserved.
* This software is open source. See the bottom of this file for the license.
* $Id: GcAtomResource.java,v 1.5 2006/12/07 04:55:28 aslom Exp $ */
package org.gpel.client;
import java.net.URI;
import org.gpel.GpelConstants;
import org.xmlpull.infoset.XmlElement;
import org.xmlpull.infoset.XmlInfosetBuilder;
import org.xmlpull.infoset.XmlNamespace;
import org.xmlpull.infoset.view.TypedXmlElementView;
import org.xmlpull.infoset.view.XmlValidationException;
public abstract class GcAtomResource extends TypedXmlElementView implements GcWebResource {
private final static XmlInfosetBuilder builder = GpelConstants.BUILDER;
private URI location;
private String rel;
public GcAtomResource(String title, String mimeType) {
//TODO: use ATOMIXMISER?
super(builder.newFragment(GcUtil.ATOM_NS, GcUtil.ATOM_ENTRY_EL));
if (title == null) throw new IllegalArgumentException();
setTitle(title);
setMimeType(mimeType);
// XmlElement contentEl = xml().addElement(GcUtil.ATOM_NS, GcUtil.ATOM_CONTENT_EL);
// contentEl.setAttributeValue("type", GcUtil.XML_MIMETYPE);
// contentEl.addElement(GpelConstants.GPEL_NS, GcTemplate.TYPE_NAME);
//loadResourcesFromLinks();
//super(wrapped);
}
public GcAtomResource(XmlElement atomRes) {
super(atomRes);
if (!atomRes.getNamespace().equals(GcUtil.ATOM_NS)) {
throw new XmlValidationException("must be in ATOM namespace");
}
if (!atomRes.getName().equals(GcUtil.ATOM_ENTRY_EL)) {
throw new XmlValidationException("must be atom:entry");
}
if (getTitle(false) == null) {
throw new XmlValidationException("required atom:title");
}
if (getContentEl(false) == null) {
throw new XmlValidationException("required atom:content");
}
}
public XmlNamespace xmlTypeNs() {
return xml().getNamespace();
}
public String xmlTypeName() {
return xml().getName();
}
public void xmlSet(XmlElement xmlEl) {
this.xml = xmlEl;
}
protected XmlElement getContentEl(boolean create) {
return xml().element(GcUtil.ATOM_NS, GcUtil.ATOM_CONTENT_EL, create);
// XmlElement topEl = xml();
// if (topEl.getName().equals(GcUtil.ATOM_ENTRY_EL)) { //&& topEl.getNamespace(GcUtil.ATOM_NS)) {
// XmlElement contentEl = topEl.element(GcUtil.ATOM_NS, GcUtil.ATOM_CONTENT_EL);
// return contentEl;
// }
// return null;
}
public URI getId() throws GcException {
//if(xml().getName().equals(TYPE_NAME)) {
XmlElement idEl = xml().element(GpelConstants.ATOM_NS, "id");
if (idEl == null) {
// if (getLocation() != null) {
// URI id = client.mapLocationToId(getLocation());
// return id;
// }
throw new GcException("resource must be first deployed to server have id");
} else {
String id = idEl.requiredText();
return URI.create(id);
}
}
public String getMimeType() {
XmlElement contentEl = getContentEl(true);
String mimeType = contentEl.attributeValue(GcUtil.ATOM_CONTENT_TYPE_ATTR);
return mimeType;
}
public void setMimeType(String mimeType) {
if (mimeType == null) throw new IllegalArgumentException();
// if (!GcWebResourceType.XML.equals(GcUtil.categorizeContentType(mimeType))) {
// throw new IllegalArgumentException("content type must be xml and not " + mimeType);
// }
XmlElement contentEl = getContentEl(true);
contentEl.setAttributeValue(GcUtil.ATOM_CONTENT_TYPE_ATTR, mimeType);
}
public String getTitle(boolean require) throws GcException {
XmlElement titleEl;
if (require) {
titleEl = xml().requiredElement(GcUtil.ATOM_NS, GcUtil.ATOM_TITLE_EL);
} else {
titleEl = xml().element(GcUtil.ATOM_NS, GcUtil.ATOM_TITLE_EL);
if (titleEl == null) {
return null;
}
}
String title = titleEl.requiredText();
return title;
}
public String getTitle() throws GcException {
return getTitle(true);
}
public void setTitle(String title) throws GcException {
XmlElement titleEl = xml().element(GpelConstants.ATOM_NS, "title", true);
titleEl.setText(title);
}
// public DcDate getUpdated() throws GcException {
// XmlElement updatedEl = xml().requiredElement(GcUtil.ATOM_NS, GcUtil.ATOM_UPDATED_EL);
// String updatedText = updatedEl.requiredText();
// DcDate updated = DcDate.create(updatedText);
// return updated;
// //return updated.getTimeInMillis();
// }
public URI getLocation() {
return location;
}
public void setLocation(URI location) {
//this.oldLocation = this.location;
this.location = location;
}
public String getRel() {
return this.rel;
}
public void setRel(String rel) {
this.rel = rel;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy