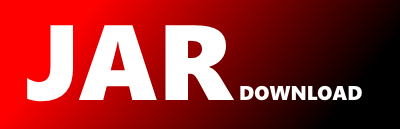
org.gpel.client.GcInstance Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gpel-client Show documentation
Show all versions of gpel-client Show documentation
Grid BPEL Engine Client developed by Extreme Computing Lab, Indiana University
The newest version!
/* -*- mode: Java; c-basic-offset: 4; indent-tabs-mode: nil; -*- //------100-columns-wide------>|*/
/* Copyright (c) 2005 Extreme! Lab, Indiana University. All rights reserved.
* This software is open source. See the bottom of this file for the licence.
* $Id: GcInstance.java,v 1.9 2007/01/17 21:42:36 aslom Exp $ */
package org.gpel.client;
import java.net.URI;
import org.gpel.GpelConstants;
import org.gpel.client.http.GcHttpRequest;
import org.gpel.client.http.GcHttpResponse;
import org.gpel.logger.GLogger;
import org.xmlpull.infoset.XmlElement;
import org.xmlpull.infoset.XmlInfosetBuilder;
public class GcInstance extends GcLinksResource {
private final static GLogger logger = GLogger.getLogger();
private final static XmlInfosetBuilder builder = GpelConstants.BUILDER;
public final static String MIME_TYPE = "application/x-gpel-instance+xml";
public final static String TYPE_NAME = "instance";
public final static String START_ACTION = "gpel-instance-start";
public final static String STEP_ACTION = "gpel-instance-step";
public final static String PAUSE_ACTION = "gpel-instance-pause";
public final static String RESUME_ACTION = "gpel-instance-resume";
public final static String STOP_ACTION = "gpel-instance-stop";
//final String STOP_ACTION = "gpel-instance-resume";
private final static String PRIORITY_EL = "priority";
protected GcInstance(GpelClient client, String title, GcLinkFilter filter)
throws GcException {
super(client, title, builder.newFragment(GpelConstants.GPEL_NS, TYPE_NAME),
filter, MIME_TYPE);
setPriority( GcPriority.SILVER );
}
public GcInstance(XmlElement atomRes) {
super(atomRes);
}
public void store() {
getClient().storeInstance(this);
}
// protected GcInstance(GpelClient client, GcXmlWebResource atomRes, GcLinkFilter filter)
// throws GcException {
// super(client, atomRes, filter);
// }
public URI getInstanceId() throws GcException {
return getId();
}
// public URI getTemplateId() throws GcException {
// GcWebResource template = getLinkWithRel(GpelConstants.REL_TEMPLATE);
// return template.getId();
// }
public GcPriority getPriority() {
XmlElement priorityEl = getXmlContent().requiredElement(PRIORITY_EL);
String s = priorityEl.requiredText();
return GcPriority.valueOf(s);
}
public void setPriority(GcPriority status) {
XmlElement priority = getXmlContent().element(null, PRIORITY_EL, true);
priority.setText(status.toString());
}
public GcState retrieveCurrentState() {
GcState oldState = (GcState) getLinkWithRel(GcState.REL);
if(null == oldState) throw new IllegalStateException("internal");
String href = oldState.getId().toString();
String rel = oldState.getRel();
GcState newState = (GcState) loadXmlResourceLink(href, rel);
replaceLinkWithRel(newState);
return newState;
}
// extract special link with template relation
//public URI getTemplateId() throws GcException {
// return getId();
//}
public GcState start() {
return executeInstanceAction(START_ACTION);
}
public GcState step() {
return executeInstanceAction(STEP_ACTION);
}
public GcState pause() {
return executeInstanceAction(PAUSE_ACTION);
}
public GcState resume() {
return executeInstanceAction(RESUME_ACTION);
}
private GcState executeInstanceAction(String action) {
URI tloc = getClient().mapIdToLocation(getInstanceId());
URI loc = URI.create(tloc.toString()+"?action="+action);
logger.finest("loc="+loc);
GcHttpRequest.Method httpMethod = GcHttpRequest.Method.POST;
// TODO: parameters ...
GcHttpRequest req = new GcHttpRequest(httpMethod, loc, GcUtil.CONTENT_TYPE_ATOM,
builder.newFragment(GcUtil.ATOM_NS, "entry"));
GcHttpResponse resp = getClient().getTransport().perform(req);
if (!resp.hasContent()) {
throw new GcException("expected XML result when POSTing to " + loc);
}
//TODO: this needs to be implemented and synced with instance link?!
GcState state = (GcState) getClient().getResourceFromHttpFactory()
.createResourceFromHttp(resp, null);
state.setLocation(resp.getLocation());
replaceLinkWithRel(state);
return state;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy