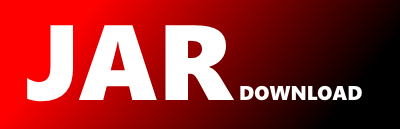
org.gpel.client.GcLinksResource Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gpel-client Show documentation
Show all versions of gpel-client Show documentation
Grid BPEL Engine Client developed by Extreme Computing Lab, Indiana University
The newest version!
/* -*- mode: Java; c-basic-offset: 4; indent-tabs-mode: nil; -*- //------100-columns-wide------>|*/
/* Copyright (c) 2005 Extreme! Lab, Indiana University. All rights reserved.
* This software is open source. See the bottom of this file for the license.
* $Id: GcLinksResource.java,v 1.9 2006/12/07 04:55:28 aslom Exp $ */
package org.gpel.client;
import java.net.URI;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import org.gpel.GpelConstants;
import org.xmlpull.infoset.XmlElement;
import org.xmlpull.infoset.XmlInfosetBuilder;
public abstract class GcLinksResource extends GcXmlWebResource { //AtomEntry/title/etc
public final static GcLinkFilter DEFAULT_LINKS_FILTER = new GcDefaultSupportedLinksFilter();
private final static XmlInfosetBuilder builder = GpelConstants.BUILDER;
private List linkedResources = new ArrayList();
private GcLinkFilter filter;
private GpelClient client;
protected GcLinksResource(GpelClient client, String title, XmlElement contentEl,
GcLinkFilter filter, String mimeType)
throws GcException {
super(title, contentEl, mimeType);
this.client = client;
this.filter = filter;
}
// protected GcLinksResource(GpelClient client, GcXmlWebResource atomRes, GcLinkFilter filter)
// throws GcException {
// super(atomRes.xml());
// setMimeType(atomRes.getMimeType());
// setLocation(atomRes.getLocation());
// setRel(atomRes.getRel());
// this.client = client;
// this.filter = filter;
// loadResourcesFromLinks();
// }
public GcLinksResource(XmlElement atomRes) {
super(atomRes);
filter = DEFAULT_LINKS_FILTER;
// client and filter will be null ...
}
public void setFilter(GcLinkFilter filter) {
this.filter = filter;
}
public GcLinkFilter getFilter() {
return filter;
}
public void setClient(GpelClient client) {
this.client = client;
}
public GpelClient getClient() {
return client;
}
public List getLinks() {
return linkedResources;
}
public GcWebResource getLinkWithId(URI id) {
for (GcWebResource gwr: getLinks()) {
if (gwr.getId().equals(id)) {
return gwr;
}
}
return null;
}
public GcWebResource getLinkWithRel(String rel) {
if (rel == null) throw new IllegalArgumentException();
for (GcWebResource gwr: getLinks()) {
if (rel.equals(gwr.getRel())) {
return gwr;
}
}
return null;
}
public GcWebResource getLinkWithTitleAndRel(String title, String rel) {
if (title == null) throw new IllegalArgumentException();
if (rel == null) throw new IllegalArgumentException();
for (GcWebResource gwr: getLinks()) {
if (title.equals(gwr.getTitle()) && rel.equals(gwr.getRel())) {
return gwr;
}
}
return null;
}
public void setLinks(List links) {
this.linkedResources = links;
}
public void setLinks(GcWebResource[] linkArr) {
linkedResources = new ArrayList(linkArr.length);
for (GcWebResource linkedRes : linkArr) {
if (linkedRes.getRel() == null) {
throw new GcException(
"every linked resource must have rel missing for " + linkedRes.getLocation());
}
linkedResources.add(linkedRes);
}
removeAllSupportedLinks();
}
public void replaceLinkWithRel(GcWebResource res) {
if (res.getRel() == null) {
throw new GcException(
"every linked resource must have rel but missing for " + res);
}
//if (!filter.accept(res.getRel())) {
//}
removeLinksWithRel(res.getRel());
linkedResources.add(res);
}
private void removeLinksWithRel(String rel) {
if(rel == null) throw new IllegalArgumentException();
ArrayList forRemoval = new ArrayList();
for (GcWebResource res : linkedResources) {
String linkRel = res.getRel();
if(rel.equals(linkRel)) {
forRemoval.add(res);
}
}
for (GcWebResource res : forRemoval) {
linkedResources.remove(res);
}
}
// ---
protected void saveLinkedResources() throws GcException {
if (client == null) {
throw new GcException(
"this template is contained as a link and its linked resources can not be saved");
}
for (GcWebResource linkedRes : linkedResources) {
try {
client.storeResource(linkedRes);
} catch (Exception e) {
String loc = "";
if (linkedRes.getLocation() != null) {
loc = " to location=" + linkedRes.getLocation();
}
String title = linkedRes.getTitle();
throw new GcException("failed to store resource title=" + title + loc, e);
}
}
recomputeLinks();
client.storeResource(this);
}
public void loadResourcesFromLinks() throws GcException {
linkedResources.clear();
for (GcLink link : supportedLinks()) {
String rel = link.getRel();
String href = link.getHref();
linkedResources.add(loadXmlResourceLink(href, rel));
}
//recomputeLinks();
}
protected GcWebResource loadXmlResourceLink(String href, String rel) throws GcException {
URI loc = URI.create(href);
GcWebResource res = client.loadResource(loc, rel);
res.setRel(rel);
return res;
}
private void recomputeLinks() {
removeAllSupportedLinks();
// recreate list of links
for (GcWebResource linkedRes : linkedResources) {
URI href = linkedRes.getLocation();
URI id = client.mapLocationToId(href);
String rel = linkedRes.getRel();
GcLink link = new GcLink(id, rel);
xml().addElement(link.xml());
}
}
private void removeAllSupportedLinks() {
List linksToRemove = new ArrayList(linkedResources.size());
for (GcLink link : supportedLinks()) {
linksToRemove.add(link);
}
//TODO: could it be in one-stope instead of two steps???
for (GcLink link : linksToRemove) {
xml().removeChild(link.xml());
}
}
private Iterable supportedLinks() {
final Iterator allLinksIter = xml().elements(GcUtil.ATOM_NS, "link").iterator();
final GcLinkFilter f = filter;
return new Iterable() {
public Iterator iterator() {
return new Iterator() {
private XmlElement nextLink = findNextLink();
private XmlElement findNextLink() {
XmlElement e = null;
while (allLinksIter.hasNext()) {
XmlElement link = allLinksIter.next();
GcLink glink = link.viewAs(GcLink.class);
if (filter.accept(glink)) {
e = link;
break;
}
}
return e;
}
public boolean hasNext() {
return nextLink != null;
}
public GcLink next() {
if (nextLink == null) throw new IllegalStateException();
XmlElement oldNextLink = nextLink;
nextLink = findNextLink();
return oldNextLink.viewAs(GcLink.class);
}
public void remove() {
throw new UnsupportedOperationException();
}
};
}
};
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy