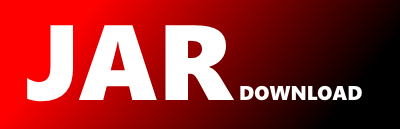
org.gpel.client.GpelClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gpel-client Show documentation
Show all versions of gpel-client Show documentation
Grid BPEL Engine Client developed by Extreme Computing Lab, Indiana University
The newest version!
/* -*- mode: Java; c-basic-offset: 4; indent-tabs-mode: nil; -*- //------100-columns-wide------>|*/
/* Copyright (c) 2005 Extreme! Lab, Indiana University. All rights reserved.
* This software is open source. See the bottom of this file for the license.
* $Id: GpelClient.java,v 1.26 2007/02/08 19:31:36 aslom Exp $ */
package org.gpel.client;
import java.net.URI;
import java.util.ArrayList;
import java.util.List;
import org.gpel.GpelConstants;
import org.gpel.client.GcSearchResult;
import org.gpel.client.http.GcDefaultWebResourceFromHttp;
import org.gpel.client.http.GcDefaultWebResourceToHttp;
import org.gpel.client.http.GcHttpRequest;
import org.gpel.client.http.GcHttpResponse;
import org.gpel.client.http.GcHttpTransport;
import org.gpel.client.http.GcWebResourceFromHttp;
import org.gpel.client.http.GcWebResourceToHttp;
import org.gpel.logger.GLogger;
import org.xmlpull.infoset.XmlElement;
import org.xmlpull.infoset.XmlInfosetBuilder;
/**
* This is a proxy class that can be used to access and manipulate GPEL workflow space.
*/
public class GpelClient {
private final static XmlInfosetBuilder builder = GpelConstants.BUILDER;
private final static GLogger logger = GLogger.getLogger();
private GcHttpTransport transport;
// private GcHttpTransport secureTransport;
private GcWebResourceFromHttp resFromHttp = GcDefaultWebResourceFromHttp.getInstance();
private GcWebResourceToHttp resToHttp = GcDefaultWebResourceToHttp.getInstance();
private GcLinkFilter filter = new GcDefaultSupportedLinksFilter();
private URI gpelNewPostLocation;
private String gpelSpaceGuid;
private URI gpelSpaceLoc; // this will work both for secure and "unsecure"
//private URI gpelSecureSpaceLoc;
//private URI gpelSecureNewPostLocation;
public GpelClient(URI autoDicoveryLocation, GcHttpTransport transport) throws GcException {
if (autoDicoveryLocation == null) throw new IllegalArgumentException();
if (transport == null) throw new IllegalArgumentException();
this.transport = transport;
//transport.setCredentials(credentials)
introspectLocation(autoDicoveryLocation);
}
// public void setTransport(GcHttpTransport transport) {
// this.transport = transport;
// }
public GcHttpTransport getTransport() {
// use secure transport if available
// if(secureTransport != null) {
// return secureTransport;
// } else {
return transport;
// }
}
public GcHttpTransport requireSecureTransport() {
// //if(secureTransport == null) throw new IllegalArgumentException(
// // "secure transport was not set");
// //return secureTransport;
return getTransport();
}
// public GcHttpTransport getSecureTransport() {
// return secureTransport;
// }
//
// public void setSecureTransport(GcHttpTransport secureTransport) {
// this.secureTransport = secureTransport;
// }
public void setFilter(GcLinkFilter filter) {
this.filter = filter;
}
public GcLinkFilter getFilter() {
return filter;
}
public URI getApiPostLocation() throws GcException {
return gpelNewPostLocation;
}
public URI mapIdToLocation(URI maybeId) {
// if(getSecureTransport() != null) {
// return mapIdToSecureLocation(maybeId);
// }
return mapIdToKnownLocation(maybeId, gpelSpaceLoc);
}
// public URI mapIdToSecureLocation(URI maybeId) {
// return mapIdToKnownLocation(maybeId, gpelSecureSpaceLoc);
// }
public URI mapIdToKnownLocation(URI maybeId, URI spaceLoc) {
URI loc = maybeId;
//tag:gpel.leadproject.org,2006:2005/07/21/entry3
if (maybeId.getScheme().equals("tag")) {
String id = maybeId.toString();
if (id.startsWith(gpelSpaceGuid)) {
String path = id.substring(gpelSpaceGuid.length());
if (!path.endsWith(".atom")) {
path += ".atom";
}
loc = URI.create(spaceLoc + path);
}
}
return loc;
}
public URI mapLocationToId(URI uri) {
URI id;
String uriAsString = uri.toString();
String spaceLoc = gpelSpaceLoc.toString();
//String secureSpaceLoc = gpelSecureSpaceLoc.toString();
if(uriAsString.startsWith(spaceLoc)){
// || uriAsString.startsWith(secureSpaceLoc)
// || uriAsString.startsWith(
// "http://tyr13.cs.indiana.edu:7443/gpel/")) { //FIXME HACK ALEK
String path = uriAsString.substring(spaceLoc.length());
// String path;
// if(uriAsString.startsWith(spaceLoc)
// || uriAsString.startsWith(
// "http://tyr13.cs.indiana.edu:7443/gpel/")) { //FIXME HACK ALEK
// path = uriAsString.substring(spaceLoc.length());
// } else {
// assert uriAsString.startsWith(secureSpaceLoc);
// path = uriAsString.substring(secureSpaceLoc.length());
// }
if (path.endsWith(".atom")) {
path = path.substring(0, path.length() - ".atom".length());
}
id = URI.create(gpelSpaceGuid + path);
} else if (uriAsString.startsWith(gpelSpaceGuid)) {
id = uri;
} else {
throw new GcException("could not map " + uri + " to id"+
" (known locations "+spaceLoc+" ) "); //" and "+secureSpaceLoc+" )");
}
return id;
}
// --- templates
public GcTemplate createTemplate(String title) throws GcException {
return new GcTemplate(this, title, filter);
}
/**
* Return the newest first.
*/
public GcSearchList findTemplates(int num) throws GcException {
GcSearchRequest req = new GcSearchRequest(num);
return findTemplates(req);
}
public GcSearchList findTemplates(int num, GcSearchRequest.SearchType st) throws GcException {
GcSearchRequest req = new GcSearchRequest(num);
req.setSearchType(st);
return findTemplates(req);
}
public GcSearchList findTemplates(GcSearchRequest req) throws GcException {
if (req.size() <= 0) throw new IllegalArgumentException();
String queryLoc = getApiPostLocation() + "/?action=search&type=gpel-template&num=" + req.size();
//parse collection
//GcHttpRequest.Method httpMethod = GcHttpRequest.Method.POST;
//GcHttpRequest req = new GcHttpRequest(GcHttpRequest.Method.GET, location);
XmlElement feed = getTransport().getXml(URI.create(queryLoc));
GcSearchListImpl results = new GcSearchListImpl();
//scan feed for results
for (XmlElement entry : feed.elements(GpelConstants.ATOM_NS, "entry")) {
String title = null;
URI id = null;
long lastModfied = -1;
XmlElement idEl = entry.element(GpelConstants.ATOM_NS, "id");
if (idEl != null) {
id = URI.create(idEl.requiredText());
}
XmlElement titleEl = entry.element(GpelConstants.ATOM_NS, "title");
if (titleEl != null) {
title = titleEl.requiredText(); //TODO: allow type="xhtml" in title
}
//TODO: process updated as lasModified
GcSearchResult hit = new GcSearchResultImpl(id, title, lastModfied);
results.addResult(hit);
}
return results;
}
public GcTemplate retrieveTemplate(URI location) throws GcException {
//GcXmlWebResource xmlRes = (GcXmlWebResource) loadResource(location);
//GcTemplate t = new GcTemplate(this, xmlRes, filter);
GcTemplate t = (GcTemplate) loadResource(location);
t.setClient(this);
t.setFilter(filter);
t.loadResourcesFromLinks();
return t;
}
public void deployTemplate(GcTemplate t) throws GcException {
t.saveLinkedResources();
}
public void storeInstance(GcInstance wfi) {
wfi.saveLinkedResources();
}
public GcInstance createInstance(GcTemplate template) {
URI tloc = mapIdToLocation(template.getTemplateId());
URI loc = URI.create(tloc.toString()+"?action=gpel-create-instance");
logger.finest("loc="+loc);
GcHttpRequest.Method httpMethod = GcHttpRequest.Method.POST;
GcHttpRequest req = new GcHttpRequest(httpMethod, loc, GcUtil.CONTENT_TYPE_ATOM,
builder.newFragment(GcUtil.ATOM_NS, "entry"));
GcHttpResponse resp = requireSecureTransport().perform(req);
if (!resp.hasContent()) {
throw new GcException("expected XML result when POSTing to " + loc);
}
GcInstance instance = (GcInstance) resFromHttp.createResourceFromHttp(resp, null);
instance.setLocation(resp.getLocation());
instance.setClient(this);
instance.setFilter(filter);
instance.loadResourcesFromLinks();
return instance;
}
public GcInstance retrieveInstance(URI location) {
GcInstance instance = (GcInstance) loadResource(location);
instance.setClient(this);
instance.setFilter(filter);
instance.loadResourcesFromLinks();
return instance;
}
//TODO: findInstance of given template or all instances of one user
//public findInstance(GcSearchRequest req)
//public findInstancesForTemplateId(URI templateId)
//public findInstancesOfTemplateId(GcTemplate template)
// --- generic resources
public void storeResource(GcWebResource resource) throws GcException {
if (resource == null) throw new IllegalArgumentException();
GcHttpRequest.Method httpMethod;
URI loc = resource.getLocation();
if (loc == null) {
// if location is null then POST and update location
httpMethod = GcHttpRequest.Method.POST;
loc = getApiPostLocation();
} else {
// if location not null then PUT location new value
httpMethod = GcHttpRequest.Method.PUT;
}
assert loc != null;
GcHttpRequest req = resToHttp.transformResourceToHttpRequest(httpMethod, loc, resource);
//actually do it
GcHttpResponse resp = requireSecureTransport().perform(req);
//if (resp.hasContent()) throw new IllegalStateException();
if (resp.hasContent()) {
throw new GcException("Unexpected content when storing resource to " + loc);
} else {
if (httpMethod == GcHttpRequest.Method.POST) {
//newly created resources need to be GET to retrieve atom:id
URI newLoc = resp.getLocation();
GcWebResource newRes = loadResource(newLoc);
//ALERT!!! now do some magic to transfer content ...
((GcAtomResource)resource).xmlSet(((GcAtomResource)newRes).xml());
}
}
resource.setLocation(resp.getLocation());
}
public GcWebResource loadResource(URI location) throws GcException {
return loadResource(location, null);
}
public GcWebResource loadResource(URI loc, String rel) throws GcException {
if (loc == null) throw new IllegalArgumentException();
URI location = mapIdToLocation(loc);
GcHttpRequest.Method met;
met = GcHttpRequest.Method.GET;
GcHttpRequest req = new GcHttpRequest(GcHttpRequest.Method.GET, location);
//actually do it
GcHttpResponse resp = getTransport().perform(req);
//if (!res.hasContent()) throw new IllegalStateException();
if (!resp.hasContent()) {
throw new GcException("no resource for " + loc + "(mapped to " + location + ")");
}
GcWebResource resource = resFromHttp.createResourceFromHttp(resp, rel);
resource.setLocation(resp.getLocation());
if (rel != null) {
resource.setRel(rel);
}
return resource;
}
private void introspectLocation(URI autoDicoveryLocation) throws GcException {
XmlElement xhtml = getTransport().getXml(autoDicoveryLocation);
//will not use XPATH as Jaxen impl ("xpp5_xpath*jar") would add 200KB to footprint
//XPATH "/html/head/link@rel='gpel.service'"
//XPATH "/html/head/link@rel='gpel.post'"
//XmlElement head = xhtml.requiredElement("html").requiredElement("head");
XmlElement head = xhtml.requiredElement("head");
for (XmlElement link : head.elements(null, "link")) {
String rel = link.attributeValue("rel");
String href = link.attributeValue("href");
if (GcUtil.GPEL_SPACE_POST.equals(rel) && href != null) {
gpelNewPostLocation = URI.create(href);
//FIXME hardcoded
//this.gpelSecureNewPostLocation = URI.create(
//this.gpelNewPostLocation = URI.create(
// "https://tyr13.cs.indiana.edu:7443/gpel/api/gpel/post");
}
if (GcUtil.GPEL_SPACE_GUID.equals(rel) && href != null) {
gpelSpaceGuid = href;
}
if (GcUtil.GPEL_SPACE_LOC.equals(rel) && href != null) {
gpelSpaceLoc = URI.create(href);
// FIXME HARDCODED
//gpelSpaceLoc = URI.create("https://tyr13.cs.indiana.edu:7443/gpel/");
//gpelSecureSpaceLoc = URI.create("https://tyr13.cs.indiana.edu:7443/gpel/");
}
}
}
public void setResourceFromHttpFactory(GcWebResourceFromHttp resFromHttp) {
this.resFromHttp = resFromHttp;
}
public GcWebResourceFromHttp getResourceFromHttpFactory() {
return resFromHttp;
}
public void setResourceToHttpFactory(GcWebResourceToHttp resToHttp) {
this.resToHttp = resToHttp;
}
public GcWebResourceToHttp getResourceToHttpFactory() {
return resToHttp;
}
static class GcSearchListImpl implements GcSearchList {
private List list = new ArrayList();
public void addResult(GcSearchResult hit) {
list.add(hit);
}
public Iterable results() {
return list;
}
public int size() {
return list.size();
}
}
static class GcSearchResultImpl implements GcSearchResult {
private URI id;
private String title;
private long lastModfied;
public GcSearchResultImpl(URI id, String title, long lastModfied) {
this.title = title;
this.id = id;
this.lastModfied = lastModfied;
}
public String getTitle() { return title;}
public URI getId() { return id;}
public long getLastModfied() { return lastModfied; }
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy