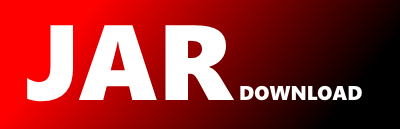
org.gpel.client.http.GcHttpRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gpel-client Show documentation
Show all versions of gpel-client Show documentation
Grid BPEL Engine Client developed by Extreme Computing Lab, Indiana University
The newest version!
/* -*- mode: Java; c-basic-offset: 4; indent-tabs-mode: nil; -*- //------100-columns-wide------>|*/
/* Copyright (c) 2005 Extreme! Lab, Indiana University. All rights reserved.
* This software is open source. See the bottom of this file for the license.
* $Id: GcHttpRequest.java,v 1.7 2007/02/04 22:03:31 aslom Exp $ */
package org.gpel.client.http;
import java.net.URI;
import org.xmlpull.infoset.XmlElement;
public class GcHttpRequest {
public enum Method { GET, PUT, POST, DELETE };
private Method method;
private URI location;
private String contentType;
private XmlElement xmlContent;
private String textContent;
private byte[] binaryContent;
private boolean withAuthz = true;
public GcHttpRequest(Method method, URI location) {
this(method, location, true);
}
public GcHttpRequest(Method method, URI location, boolean secure) {
if (method == null) throw new IllegalArgumentException();
if (!(Method.GET.equals(method) || Method.DELETE.equals(method)) ) {
throw new IllegalArgumentException(
"method must be " + Method.GET + " or " + Method.DELETE + " for no content operation");
}
this.method = method;
this.location = location;
this.withAuthz = secure;
}
public GcHttpRequest(Method method, URI location, String contentType, XmlElement xmlContent) {
if (method == null) throw new IllegalArgumentException();
if (!( (Method.POST.equals(method) || Method.PUT.equals(method)) )) {
throw new IllegalArgumentException(
"method must be " + Method.POST + " or " + Method.PUT + " for content");
}
this.method = method;
this.location = location;
this.contentType = contentType;
this.xmlContent = xmlContent;
}
public GcHttpRequest(Method method, URI location, String contentType, String textContent) {
if (method == null) throw new IllegalArgumentException();
if (!( (Method.POST.equals(method) || Method.PUT.equals(method)) )) {
throw new IllegalArgumentException("method must be POST or PUT for content");
}
this.method = method;
this.location = location;
this.contentType = contentType;
this.textContent = textContent;
}
public GcHttpRequest(Method method, URI location, String contentType, byte[] binaryContent) {
if (method == null) throw new IllegalArgumentException();
if (!( (Method.POST.equals(method) || Method.PUT.equals(method)) )) {
throw new IllegalArgumentException("method must be POST or PUT for content");
}
this.method = method;
this.location = location;
this.contentType = contentType;
this.binaryContent = binaryContent;
}
public boolean hasContent() {
return !(xmlContent == null && textContent == null && binaryContent == null);
}
// public void setXmlContent(XmlElement xmlContent) {
// this.xmlContent = xmlContent;
// }
public XmlElement getXmlContent() {
return xmlContent;
}
// public void setTextContent(String textContent) {
// this.textContent = textContent;
// }
public String getTextContent() {
return textContent;
}
// public void setBinaryContent(byte[] binaryContent) {
// this.binaryContent = binaryContent;
// }
public byte[] getBinaryContent() {
return binaryContent;
}
// public void setContentType(String contentType) {
// this.contentType = contentType;
// }
public String getContentType() {
return contentType;
}
// public void setLocation(URI location) {
// this.location = location;
// }
public URI getLocation() {
return location;
}
// public void setMethod(Method method) {
// this.method = method;
// }
public Method getMethod() {
return method;
}
// public boolean isSecure() {
// return withAuthz;
// }
public boolean useAuthz() {
return withAuthz;
}
public String toString() {
return super.toString()
+"{method="+method
+" location="+location
+" contentType="+contentType
+" xmlContent="+xmlContent
+" textContent="+textContent
+" binaryContent="+binaryContent
+" withAuthz="+withAuthz
+"}";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy