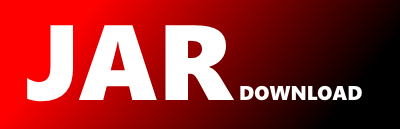
org.gpel.client.http.GcHttpResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gpel-client Show documentation
Show all versions of gpel-client Show documentation
Grid BPEL Engine Client developed by Extreme Computing Lab, Indiana University
The newest version!
/* -*- mode: Java; c-basic-offset: 4; indent-tabs-mode: nil; -*- //------100-columns-wide------>|*/
/* Copyright (c) 2005 Extreme! Lab, Indiana University. All rights reserved.
* This software is open source. See the bottom of this file for the license.
* $Id: GcHttpResponse.java,v 1.4 2006/12/07 04:55:30 aslom Exp $ */
package org.gpel.client.http;
import java.net.URI;
import org.xmlpull.infoset.XmlElement;
public class GcHttpResponse {
//getResponseAsString
private URI location;
private String contentType;
private byte[] binaryContent;
private String textContent;
private XmlElement xmlContent;
public GcHttpResponse(URI location, String contentType, byte[] binaryContent) {
this.location = location;
this.contentType = contentType;
this.binaryContent = binaryContent;
}
public GcHttpResponse(URI location, String contentType, String textContent) {
this.location = location;
this.contentType = contentType;
this.textContent = textContent;
}
public GcHttpResponse(URI location, String contentType, XmlElement xmlContent) {
this.location = location;
this.contentType = contentType;
this.xmlContent = xmlContent;
}
public GcHttpResponse(URI location) {
this.location = location;
}
public boolean hasContent() {
return !(xmlContent == null && textContent == null && binaryContent == null);
}
// public void setXmlContent(XmlElement xmlContent) {
// this.xmlContent = xmlContent;
// }
public XmlElement getXmlContent() {
return xmlContent;
}
// public void setTextContent(String textContent) {
// this.textContent = textContent;
// }
public String getTextContent() {
return textContent;
}
// public void setBinaryContent(byte[] binaryContent) {
// this.binaryContent = binaryContent;
// }
public byte[] getBinaryContent() {
return binaryContent;
}
// public void setContentType(String contentType) {
// this.contentType = contentType;
// }
public String getContentType() {
return contentType;
}
// public void setLocation(URI location) {
// this.location = location;
// }
public URI getLocation() {
return location;
}
public String toString() {
return getClass().getName() + "{"+"location="+location
+" contentType="+contentType
+" binaryContent="+binaryContent
+" textContent="+textContent
+" xmlContent="+xmlContent
+"}";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy