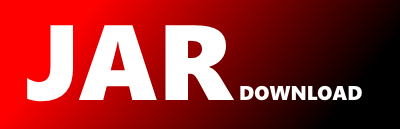
org.gpel.client.security.GpelUserX509Credential Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gpel-client Show documentation
Show all versions of gpel-client Show documentation
Grid BPEL Engine Client developed by Extreme Computing Lab, Indiana University
The newest version!
///* -*- mode: Java; c-basic-offset: 4; indent-tabs-mode: nil; -*- //------100-columns-wide------>|*/
///* Copyright (c) 2005 Extreme! Lab, Indiana University. All rights reserved.
// * This software is open source. See the bottom of this file for the license.
// * $Id: GpelUserX509Credential.java,v 1.7 2007/02/08 15:36:06 aslom Exp $ */
///**
// SSLContext.java
//
// Copyright (C) 1999, Claymore Systems, Inc.
// All Rights Reserved.
//
// [email protected] Tue May 18 09:43:47 1999
//
// This package is a SSLv3/TLS implementation written by Eric Rescorla
// and licensed by Claymore Systems, Inc.
//
// Redistribution and use in source and binary forms, with or without
// modification, are permitted provided that the following conditions
// are met:
// 1. Redistributions of source code must retain the above copyright
// notice, this list of conditions and the following disclaimer.
// 2. Redistributions in binary form must reproduce the above copyright
// notice, this list of conditions and the following disclaimer in the
// documentation and/or other materials provided with the distribution.
// 3. All advertising materials mentioning features or use of this software
// must display the following acknowledgement:
// This product includes software developed by Claymore Systems, Inc.
// 4. Neither the name of Claymore Systems, Inc. nor the name of Eric
// Rescorla may be used to endorse or promote products derived from this
// software without specific prior written permission.
//
// THIS SOFTWARE IS PROVIDED BY THE REGENTS AND CONTRIBUTORS ``AS IS'' AND
// ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
// IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
// ARE DISCLAIMED. IN NO EVENT SHALL THE REGENTS OR CONTRIBUTORS BE LIABLE
// FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
// DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS
// OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION)
// HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT
// LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY
// OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF
// SUCH DAMAGE.
//
// $Id: GpelUserX509Credential.java,v 1.7 2007/02/08 15:36:06 aslom Exp $
//
// */
//package org.gpel.client.security;
//
//import COM.claymoresystems.cert.WrappedObject;
//import COM.claymoresystems.ptls.SSLDebug;
//import java.io.BufferedReader;
//import java.io.ByteArrayInputStream;
//import java.io.ByteArrayOutputStream;
//import java.io.EOFException;
//import java.io.FileInputStream;
//import java.io.IOException;
//import java.io.InputStream;
//import java.io.InputStreamReader;
//import java.security.GeneralSecurityException;
//import java.security.PrivateKey;
//import java.security.cert.X509Certificate;
//import org.globus.gsi.CertUtil;
//import org.globus.gsi.GlobusCredential;
//import org.globus.gsi.OpenSSLKey;
//import org.globus.gsi.bc.BouncyCastleOpenSSLKey;
//import org.globus.gsi.gssapi.GlobusGSSCredentialImpl;
//import org.globus.util.Base64;
//import org.gpel.client.GcException;
//import org.gpel.logger.GLogger;
//import org.ietf.jgss.GSSCredential;
//
//
///**
// * Represent X509 user credentials (user private key, and certificate chain) used to authenticate
// * and authorize user to GPEL engine.
// */
//public class GpelUserX509Credential {
// private final static GLogger logger = GLogger.getLogger();
// private X509Certificate[] userCertChain;
// private PrivateKey userPrivateKey;
// private X509Certificate[] certificatesTrustedByUser;
//
// public GpelUserX509Credential(String certKeyFile, String trustedCertsFile) {
// try {
// this.userPrivateKey = GpelUserX509Credential.loadPrivateKey(certKeyFile, "");
// logger.info("userPrivateKey=" + userPrivateKey);
// } catch (Exception e) {
// String message = "Failed to read private key from "+certKeyFile;
// throw new GcException(message, e);
// }
// try {
// this.userCertChain = CertUtil.loadCertificates(certKeyFile);
// logger.info("userCertChain.length=" + userCertChain.length);
// logger.info("userCertChain[0]=" + userCertChain);
// //userCertChain[0].checkValidity();
// } catch (Exception e) {
// String message = "Failed to read user certificates from "+certKeyFile;
// throw new GcException(message, e);
// }
// try {
// this.certificatesTrustedByUser = CertUtil.loadCertificates(trustedCertsFile);
// logger.info("certificatesTrustedByUser.length=" + certificatesTrustedByUser.length);
// logger.info("userCercertificatesTrustedByUsertChain[0]=" + certificatesTrustedByUser);
// } catch (Exception e) {
// String message = "Failed to read trusted certificates from "+trustedCertsFile;
// throw new GcException(message, e);
// }
// init(userCertChain, userPrivateKey, certificatesTrustedByUser);
// }
//
//
//
// public GpelUserX509Credential(
// GSSCredential proxy,
// X509Certificate[] certificatesTrustedByUser) {
// //if(proxy == null) throw new IllegalArgumentException();
// logger.config("proxy: " + proxy);
// if(proxy != null) {
// GlobusCredential globusCred =
// ( (GlobusGSSCredentialImpl) proxy).getGlobusCredential();
// init(globusCred.getCertificateChain(), globusCred.getPrivateKey(),
// certificatesTrustedByUser);
// } else {
// init(null, null, certificatesTrustedByUser);
// }
// }
//
// public GpelUserX509Credential(
// X509Certificate[] userCertChain,
// PrivateKey userPrivateKey,
// X509Certificate[] certificatesTrustedByUser) {
// init(userCertChain, userPrivateKey, certificatesTrustedByUser);
// }
//
//// public GpelUserX509Credential(GSSCredential cred) throws XsulException {
//// this(cred, null);
//// }
//
//// public GpelUserX509Credential(GSSCredential cred, X509Certificate[] trustedCerts) {
//// if (! (cred instanceof GlobusGSSCredentialImpl)) {
//// String error = "The credential is not a Globus credential. ";
//// logger.severe(error);
//// throw new XsulException(error);
//// }
//
//// GlobusCredential globusCred = ( (GlobusGSSCredentialImpl) cred).getGlobusCredential();
//// if (trustedCerts == null) {
//// // Load from the default Globus location, if trustedCerts are not passed.
//// TrustedCertificates tc = TrustedCertificates.getDefaultTrustedCertificates();
//// if (tc == null) {
//// String error = "Trusted certificates is null. ";
//// logger.severe(error);
//// throw new DynamicInfosetInvokerException(error);
//// }
//// trustedCerts = tc.getCertificates();
//// }
//
//// }
//
// private void init(X509Certificate[] userCertChain, PrivateKey userPrivateKey,
// X509Certificate[] certificatesTrustedByUser) {
//
// //if(userCertChain == null || userCertChain.length == 0) throw new IllegalArgumentException();
// this.userCertChain = userCertChain;
// if(logger.isConfigEnabled() && userCertChain != null) {
// for (int i = 0; i < userCertChain.length; i++) {
// X509Certificate certificate = userCertChain[i];
// logger.config("userCertChain["+i+"]: " + certificate);
// }
// }
// //if(userPrivateKey == null) throw new IllegalArgumentException();
// this.userPrivateKey = userPrivateKey;
// if(userPrivateKey != null) {
// logger.config("userPrivateKey algo: " + userPrivateKey.getAlgorithm());
// }
// if(certificatesTrustedByUser == null || certificatesTrustedByUser.length == 0) throw new IllegalArgumentException();
// this.certificatesTrustedByUser = certificatesTrustedByUser;
// if(logger.isConfigEnabled()) {
// for (int i = 0; i < certificatesTrustedByUser.length; i++) {
// X509Certificate certificate = certificatesTrustedByUser[i];
// logger.config("certificatesTrustedByUser["+i+"]: " + certificate);
// }
// }
// }
//
// public X509Certificate[] getCertificatesTrustedByUser() {
// return certificatesTrustedByUser;
// }
//
//// public void setCertificatesTrustedByUser(X509Certificate[] certificatesTrustedByUser) {
//// this.certificatesTrustedByUser = certificatesTrustedByUser;
//// }
//
// public X509Certificate[] getUserCertChain() {
// return userCertChain;
// }
//
//// public void setUserCertChain(X509Certificate[] userCertChain) {
//// this.userCertChain = userCertChain;
//// }
//
// public PrivateKey getUserPrivateKey() {
// return userPrivateKey;
// }
//
//// public void setUserPrivateKey(PrivateKey userPrivateKey) {
//// this.userPrivateKey = userPrivateKey;
//// }
//
// public static PrivateKey loadPrivateKey(String fileName, String passphrase)
// throws IOException {
// FileInputStream fis=new FileInputStream(fileName);
// return loadPrivateKey(fis,passphrase);
// }
//
// // copied from PureTLS SSLContext as unfortunately getPrivateKey() is not public ...
// public static PrivateKey loadPrivateKey(InputStream is, String passphrase)
// throws IOException {
//
// // first, we copy this into a byte array so we can open it twice
// byte[] blk=new byte[1024];
// int r;
// ByteArrayOutputStream tos=new ByteArrayOutputStream();
//
// while((r=is.read(blk))>0){
// tos.write(blk,0,r);
// }
// byte[] tmp=tos.toByteArray();
//
// ByteArrayInputStream tis=new ByteArrayInputStream(tmp);
//
// // Now read it
// BufferedReader br=new BufferedReader(new InputStreamReader(tis));
//
// PrivateKey tmpPrivateKey;
// StringBuffer keyType=new StringBuffer();
//
// SSLDebug.debug(SSLDebug.DEBUG_INIT,"Loading key file");
//
// if(!WrappedObject.findObject(br,"PRIVATE KEY",keyType)) {
// throw new IOException("Couldn't find private key in this file");
// }
//
// byte [] data = getDecodedPEMObject(br);
// OpenSSLKey k;
// try {
// k = new BouncyCastleOpenSSLKey("RSA", data);
// } catch (GeneralSecurityException e) {
// // TODO Auto-generated catch block
// throw new IOException("could not load private key");
// }
// PrivateKey key = k.getPrivateKey();
//
// return key;
//// try {
//// tmpPrivateKey=EAYEncryptedPrivateKey.createPrivateKey(br,
//// keyType.toString(),passphrase.getBytes());
//// } catch (IllegalArgumentException e){
//// throw new IOException(e.toString());
//// }
//
//// return tmpPrivateKey;
//
//// // Now reopen the file to get certs
//// tis=new ByteArrayInputStream(tmp);
//// br=new BufferedReader(new InputStreamReader(tis));
//
//// Vector certs=new Vector();
//
//// for(;;){
//// byte[] cert=WrappedObject.loadObject(br,"CERTIFICATE",null);
//
//// if(cert==null)
//// break;
//// SSLDebug.debug(SSLDebug.DEBUG_INIT,"Loading certificate",cert);
//// certs.insertElementAt((Object)cert,0);
//// }
//
//// // Enforce a minimum of one certificate
//// if(certs.size()==0)
//// throw new IOException("Need at least one certificate");
//
//// // Now extract the public key
//// X509Cert cert=new X509Cert((byte[])certs.elementAt(0));
//
//// userPublicKey=cert.getPublicKey(); // Some genius made private keys
//// // not contain the public key
//// userPrivateKey=tmpPrivateKey;
//// userCertChain=certs;
// }
//
// private static final byte[] getDecodedPEMObject(BufferedReader reader)
// throws IOException {
// String line;
// StringBuffer buf = new StringBuffer();
// while( (line = reader.readLine()) != null ) {
// if (line.indexOf("--END") != -1) { // found end
// return Base64.decode(buf.toString().getBytes());
// } else {
// buf.append(line);
// }
// }
// throw new EOFException("PEM footer");
// }
//
//
//}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy