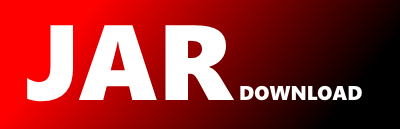
org.gpel.model.GpelInvoke Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gpel-client Show documentation
Show all versions of gpel-client Show documentation
Grid BPEL Engine Client developed by Extreme Computing Lab, Indiana University
The newest version!
/* -*- mode: Java; c-basic-offset: 4; indent-tabs-mode: nil; -*- //------100-columns-wide------>|*/
/*
* Copyright (c) 2004-2005 Extreme! Lab, Indiana University. All rights reserved.
*
* This software is open source. See the bottom of this file for the license.
*
* $Id: GpelInvoke.java,v 1.4 2008/02/26 01:39:24 cherath Exp $
*/
package org.gpel.model;
import javax.xml.namespace.QName;
import org.xmlpull.infoset.XmlElement;
import org.xmlpull.infoset.XmlNamespace;
public class GpelInvoke extends GpelExternalActivity {
public static final String TYPE_NAME = "invoke";
private static final String INPUT_VARIABLE_NAME_ATTR = "inputVariable";
private static final String OUTPUT_VARIABLE_NAME_ATTR = "outputVariable";
public GpelInvoke(XmlElement target) {
super(TYPE_NAME, target);
}
public GpelInvoke(XmlNamespace NS, String partnerLink, QName portTypeQName, String operation) {
super(NS, TYPE_NAME);
setPartnerLinkNcName(partnerLink);
setPortTypeQName(portTypeQName);
setOperationNcName(operation);
}
public GpelInvoke(XmlNamespace NS, String partnerLink, XmlNamespace portTypeNamespace, String portTypeName, String operation) {
this(NS, partnerLink,
new QName(portTypeNamespace.getName(), portTypeName, portTypeNamespace.getPrefix()),
operation);
}
/**
* Set output variable name.
*/
public GpelInvoke setInputVariableName(String gpelInputVariableName) {
xml.setAttributeValue(INPUT_VARIABLE_NAME_ATTR, gpelInputVariableName);
return this;
}
/**
* Return input variable name;
*/
public String getInputVariableName() {
return xml.attributeValue(INPUT_VARIABLE_NAME_ATTR);
}
/**
* Set output variable name.
*/
public GpelInvoke setOutputVariableName(String gpelOutputVariableName) {
xml.setAttributeValue(OUTPUT_VARIABLE_NAME_ATTR, gpelOutputVariableName);
return this;
}
/**
* Return output variable name;
*/
public String gelOutputVariableName() {
return xml.attributeValue(OUTPUT_VARIABLE_NAME_ATTR);
}
}
/*
* Indiana University Extreme! Lab Software License, Version 1.2
*
* Copyright (c) 2004-2005 The Trustees of Indiana University.
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are
* met:
*
* 1) All redistributions of source code must retain the above
* copyright notice, the list of authors in the original source
* code, this list of conditions and the disclaimer listed in this
* license;
*
* 2) All redistributions in binary form must reproduce the above
* copyright notice, this list of conditions and the disclaimer
* listed in this license in the documentation and/or other
* materials provided with the distribution;
*
* 3) Any documentation included with all redistributions must include
* the following acknowledgement:
*
* "This product includes software developed by the Indiana
* University Extreme! Lab. For further information please visit
* http://www.extreme.indiana.edu/"
*
* Alternatively, this acknowledgment may appear in the software
* itself, and wherever such third-party acknowledgments normally
* appear.
*
* 4) The name "Indiana University" or "Indiana University
* Extreme! Lab" shall not be used to endorse or promote
* products derived from this software without prior written
* permission from Indiana University. For written permission,
* please contact http://www.extreme.indiana.edu/.
*
* 5) Products derived from this software may not use "Indiana
* University" name nor may "Indiana University" appear in their name,
* without prior written permission of the Indiana University.
*
* Indiana University provides no reassurances that the source code
* provided does not infringe the patent or any other intellectual
* property rights of any other entity. Indiana University disclaims any
* liability to any recipient for claims brought by any other entity
* based on infringement of intellectual property rights or otherwise.
*
* LICENSEE UNDERSTANDS THAT SOFTWARE IS PROVIDED "AS IS" FOR WHICH
* NO WARRANTIES AS TO CAPABILITIES OR ACCURACY ARE MADE. INDIANA
* UNIVERSITY GIVES NO WARRANTIES AND MAKES NO REPRESENTATION THAT
* SOFTWARE IS FREE OF INFRINGEMENT OF THIRD PARTY PATENT, COPYRIGHT, OR
* OTHER PROPRIETARY RIGHTS. INDIANA UNIVERSITY MAKES NO WARRANTIES THAT
* SOFTWARE IS FREE FROM "BUGS", "VIRUSES", "TROJAN HORSES", "TRAP
* DOORS", "WORMS", OR OTHER HARMFUL CODE. LICENSEE ASSUMES THE ENTIRE
* RISK AS TO THE PERFORMANCE OF SOFTWARE AND/OR ASSOCIATED MATERIALS,
* AND TO THE PERFORMANCE AND VALIDITY OF INFORMATION GENERATED USING
* SOFTWARE.
*/
© 2015 - 2024 Weber Informatics LLC | Privacy Policy