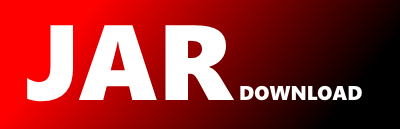
org.gpel.model.GpelProcess Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gpel-client Show documentation
Show all versions of gpel-client Show documentation
Grid BPEL Engine Client developed by Extreme Computing Lab, Indiana University
The newest version!
/* -*- mode: Java; c-basic-offset: 4; indent-tabs-mode: nil; -*- //------100-columns-wide------>|*/
/*
* Copyright (c) 2004-2005 Extreme! Lab, Indiana University. All rights reserved.
*
* This software is open source. See the bottom of this file for the license.
*
* $Id: GpelProcess.java,v 1.7 2008/02/26 01:39:24 cherath Exp $
*/
package org.gpel.model;
import javax.xml.namespace.QName;
import org.gpel.GpelConstants;
import org.gpel.logger.GLogger;
import org.xmlpull.infoset.XmlElement;
import org.xmlpull.infoset.XmlNamespace;
import org.xmlpull.infoset.view.XmlValidationException;
public class GpelProcess extends GpelModelBase {
private final static GLogger logger = GLogger.getLogger();
public static final String TYPE_NAME = "process";
private static final String TARGET_NAMESPACE = "targetNamespace";
public GpelProcess(XmlElement target) {
super(TYPE_NAME, target);
}
public GpelProcess(XmlNamespace docNS, String targetNamespaceUri) {
super(docNS, TYPE_NAME);
GpelConstants.GPEL_NS =docNS;
xml().declareNamespace(GpelConstants.GPEL_NS);
setTargetNamespace(targetNamespaceUri);
}
public GpelVariablesContainer getVariables() throws GpelModelValidationException {
XmlElement el = xml().element(xml().getNamespace(), GpelVariablesContainer.TYPE_NAME);
if(el == null) {
el = xml().addElement(builder.newFragment(NS, GpelVariablesContainer.TYPE_NAME));
}
GpelVariablesContainer variables = el.viewAs(GpelVariablesContainer.class);
return variables;
}
public void setVariables(GpelVariablesContainer variables) throws GpelModelValidationException {
XmlElement el = xml().element(null, GpelVariablesContainer.TYPE_NAME);
if(el != null) {
xml().removeChild(el);
}
xml().addElement(variables.xml());
}
public void addMessageVariable(String varName, XmlNamespace msgTypeNs, String msgTypeLocalName) {
GpelVariable var = new GpelVariable(NS, varName);
//todo: check that names is not duplicated
getVariables().xml().addElement(var.xml());
var.setMessageTypeQName(new QName(msgTypeNs.getName(), msgTypeLocalName, msgTypeNs.getPrefix()));
}
public GpelPartnerLinksContainer getPartnerLinks() throws GpelModelValidationException {
XmlElement el = xml().element(xml().getNamespace(), GpelPartnerLinksContainer.TYPE_NAME);
if(el == null) {
el = xml().addElement(builder.newFragment(NS, GpelPartnerLinksContainer.TYPE_NAME));
}
GpelPartnerLinksContainer partnerLinks = el.viewAs(GpelPartnerLinksContainer.class);
return partnerLinks;
}
public void addPartnerLink(String linkName, XmlNamespace partnerLinkTypeNs, String partnerLinkTypeName) {
addPartnerLink(linkName, partnerLinkTypeNs, partnerLinkTypeName, null, null);
}
public void addPartnerLink(String linkName, XmlNamespace partnerLinkTypeNs, String partnerLinkTypeName,
String myRole, String partnerRole) {
GpelPartnerLink pl = new GpelPartnerLink(NS, linkName, partnerLinkTypeNs, partnerLinkTypeName);
//todo: check that names is not duplicated
getPartnerLinks().xml().addElement(pl.xml());
if(myRole != null) {
pl.setMyRole(myRole);
}
if(partnerRole != null) {
pl.setPartnerRole(partnerRole);
}
}
public String getTargetNamespace() {
return xml.attributeValue(TARGET_NAMESPACE);
}
public void setTargetNamespace(String targetNamespaceUri) {
xml.setAttributeValue(TARGET_NAMESPACE, targetNamespaceUri);
}
private int counter = 1; // GpelProces is not multi-thread safe!
public GpelActivity lookupActivity(String pos) throws GpelModelValidationException {
GpelActivity topActivity = getActivity();
GpelActivity result = lookupActivity(pos, topActivity);
return result;
}
public GpelActivity lookupActivity(String pos, GpelActivity parent) throws GpelModelValidationException {
String p = parent.getInternalStatementId();
if(p == null) {
p = "" + counter++;
parent.setInternalStatementId(p);
}
if(pos.equals(p)) {
return parent;
}
for(XmlElement el : parent.xml().requiredElementContent()) {
GpelActivity childActivity = GpelActivity.convertElementToActivityIfPossible(el);
if(childActivity != null) {
GpelActivity result = lookupActivity(pos, childActivity);
if(result != null) {
return result;
}
}
}
return null;
}
public GpelActivity getActivity() throws GpelModelValidationException {
for(XmlElement el : xml().requiredElementContent()) {
XmlNamespace elNs = el.getNamespace();
if(elNs.equals(GpelConstants.BPEL_NS) || elNs.equals(GpelConstants.GPEL_NS)) {
String elName = el.getName();
//logger.finest("checking for activity "+el);
if(elName.equals(GpelSequence.TYPE_NAME)) {
GpelSequence seq = el.viewAs(GpelSequence.class);
return seq;
}
}
}
return null;
// throw new GpelModelValidationException(
// ""+GpelSequence.TYPE_NAME+" is the only supported activity");
}
public void setActivity(GpelActivity activity) throws GpelModelValidationException {
if(!( activity instanceof GpelSequence)) {
throw new IllegalArgumentException(
"only "+GpelSequence.TYPE_NAME+" currently supported as main process activity");
}
// if(getActivity() != null) {
// throw GpelModelValidationException
// }
if(getActivity() != null) {
xml().removeChild(getActivity().xml());
}
assert getActivity() == null;
xml().addElement(activity.xml());
}
public void xmlValidate() throws XmlValidationException {
GpelActivity activity = getActivity();
if(activity == null) {
throw new XmlValidationException("missing required activity in "+xmlString());
}
activity.xmlValidate();
}
}
/*
* Indiana University Extreme! Lab Software License, Version 1.2
*
* Copyright (c) 2004-2005 The Trustees of Indiana University.
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are
* met:
*
* 1) All redistributions of source code must retain the above
* copyright notice, the list of authors in the original source
* code, this list of conditions and the disclaimer listed in this
* license;
*
* 2) All redistributions in binary form must reproduce the above
* copyright notice, this list of conditions and the disclaimer
* listed in this license in the documentation and/or other
* materials provided with the distribution;
*
* 3) Any documentation included with all redistributions must include
* the following acknowledgement:
*
* "This product includes software developed by the Indiana
* University Extreme! Lab. For further information please visit
* http://www.extreme.indiana.edu/"
*
* Alternatively, this acknowledgment may appear in the software
* itself, and wherever such third-party acknowledgments normally
* appear.
*
* 4) The name "Indiana University" or "Indiana University
* Extreme! Lab" shall not be used to endorse or promote
* products derived from this software without prior written
* permission from Indiana University. For written permission,
* please contact http://www.extreme.indiana.edu/.
*
* 5) Products derived from this software may not use "Indiana
* University" name nor may "Indiana University" appear in their name,
* without prior written permission of the Indiana University.
*
* Indiana University provides no reassurances that the source code
* provided does not infringe the patent or any other intellectual
* property rights of any other entity. Indiana University disclaims any
* liability to any recipient for claims brought by any other entity
* based on infringement of intellectual property rights or otherwise.
*
* LICENSEE UNDERSTANDS THAT SOFTWARE IS PROVIDED "AS IS" FOR WHICH
* NO WARRANTIES AS TO CAPABILITIES OR ACCURACY ARE MADE. INDIANA
* UNIVERSITY GIVES NO WARRANTIES AND MAKES NO REPRESENTATION THAT
* SOFTWARE IS FREE OF INFRINGEMENT OF THIRD PARTY PATENT, COPYRIGHT, OR
* OTHER PROPRIETARY RIGHTS. INDIANA UNIVERSITY MAKES NO WARRANTIES THAT
* SOFTWARE IS FREE FROM "BUGS", "VIRUSES", "TROJAN HORSES", "TRAP
* DOORS", "WORMS", OR OTHER HARMFUL CODE. LICENSEE ASSUMES THE ENTIRE
* RISK AS TO THE PERFORMANCE OF SOFTWARE AND/OR ASSOCIATED MATERIALS,
* AND TO THE PERFORMANCE AND VALIDITY OF INFORMATION GENERATED USING
* SOFTWARE.
*/
© 2015 - 2024 Weber Informatics LLC | Privacy Policy