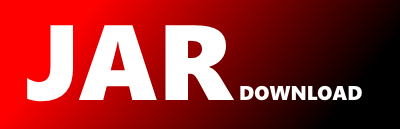
org.xmlpull.infoset.impl.XmlElementWithViewsImpl Maven / Gradle / Ivy
/* -*- c-basic-offset: 4; indent-tabs-mode: nil; -*- //------100-columns-wide------>|*/
// for license please see accompanying LICENSE.txt file (available also at http://www.xmlpull.org/)
package org.xmlpull.infoset.impl;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.List;
import org.xmlpull.infoset.XmlBuilderException;
import org.xmlpull.infoset.XmlElement;
import org.xmlpull.infoset.XmlElementView;
import org.xmlpull.infoset.XmlNamespace;
/**
* This class supoprts for views to XML Infoset Element.
*
* @version $Revision: 1.4 $
* @author Aleksander Slominski
*/
public class XmlElementWithViewsImpl extends XmlElementImpl implements XmlElement {
private List views = null;
public XmlElementWithViewsImpl clone() throws CloneNotSupportedException {
XmlElementWithViewsImpl cloned = (XmlElementWithViewsImpl) super.clone();
cloned.views = null;
return cloned;
}
public XmlElementWithViewsImpl(XmlNamespace namespace, String name) {
super(namespace, name);
}
public XmlElementWithViewsImpl(String namespaceName, String name) {
super(namespaceName, name);
}
public XmlElement newElement(String namespace, String name) {
return new XmlElementWithViewsImpl(namespace, name);
}
public XmlElement newElement(XmlNamespace namespace, String name) {
return new XmlElementWithViewsImpl(namespace, name);
}
public T viewAs(Class someViewClass) throws XmlBuilderException {
//public XmlElementView viewAs(Class someViewClass) throws XmlBuilderException {
if (views != null) {
for (int i = 0; i < views.size(); i++) {
XmlElementView view = views.get(i);
Class viewClass = view.getClass();
if (someViewClass.isAssignableFrom(viewClass)) {
//return (XmlElementView) view;
return (T) view;
}
}
}
try {
XmlElementView view = (XmlElementView)
someViewClass.getConstructor(new Class[]{XmlElement.class}).newInstance(
new Object[]{this});
addView(view);
return (T) view;
} catch (Exception e) {
throw new XmlBuilderException("could not create view to " + someViewClass, e);
}
}
public void addView(XmlElementView newView) throws XmlBuilderException {
Class newViewClass = newView.getClass();
if (views != null) {
for (int i = 0; i < views.size(); i++) {
XmlElementView view = views.get(i);
Class viewClass = view.getClass();
if (newViewClass.isAssignableFrom(viewClass)) {
//return (XmlElementView) view;
if (newView == view) {
return; //view may have added itself in constructor ...
} else {
throw new XmlBuilderException(
"there is already view that is assignable to " + newViewClass
+ "(old: " + view + " new: " + newView + ")");
}
}
}
}
if (views == null) {
views = new LinkedList();
}
views.add(newView);
}
public Iterable elements(XmlNamespace n, String name,
Class someViewClass)
{
final Iterable iterable = elements(n, name);
return new XmlViewIterableAdapter(iterable, someViewClass);
}
}
// thansk to broken generics T.class have to duplicate instead of
class XmlViewIterableAdapter implements Iterable {
private final Iterable iterable;
private final Class xmlViewClass;
XmlViewIterableAdapter(Iterable iterable, Class xmlViewClass) {
this.iterable = iterable;
this.xmlViewClass = xmlViewClass;
}
public T next(Iterator iter) {
//return (T) iter.next();
XmlElement el = (XmlElement) iter.next();
return el.viewAs(xmlViewClass); //BROKENJAVA: T.class would be simpleter ...
}
public Iterator iterator() {
final Iterator iter = iterable.iterator();
return new Iterator() {
public boolean hasNext() {
return iter.hasNext();
}
public void remove() {
iter.remove();
}
public T next() {
return XmlViewIterableAdapter.this.next(iter);
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy