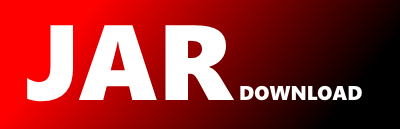
org.ojalgo.function.FunctionSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ojalgo Show documentation
Show all versions of ojalgo Show documentation
oj! Algorithms - ojAlgo - is Open Source Java code that has to do with mathematics, linear algebra and optimisation.
/*
* Copyright 1997-2021 Optimatika
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
package org.ojalgo.function;
import org.ojalgo.function.aggregator.AggregatorSet;
import org.ojalgo.type.context.NumberContext;
/**
* A predefined/standard set of functions.
*
* @author apete
*/
public abstract class FunctionSet> {
protected FunctionSet() {
super();
}
/**
* @see Math#abs(double)
*/
public abstract UnaryFunction abs();
/**
* @see Math#acos(double)
*/
public abstract UnaryFunction acos();
public abstract UnaryFunction acosh();
/**
* +
*/
public abstract BinaryFunction add();
public abstract AggregatorSet aggregator();
/**
* @see Math#asin(double)
*/
public abstract UnaryFunction asin();
public abstract UnaryFunction asinh();
/**
* @see Math#atan(double)
*/
public abstract UnaryFunction atan();
/**
* @see Math#atan2(double,double)
*/
public abstract BinaryFunction atan2();
public abstract UnaryFunction atanh();
public abstract UnaryFunction cardinality();
/**
* @see Math#cbrt(double)
*/
public abstract UnaryFunction cbrt();
/**
* @see Math#ceil(double)
*/
public abstract UnaryFunction ceil();
public abstract UnaryFunction conjugate();
/**
* @see Math#cos(double)
*/
public abstract UnaryFunction cos();
/**
* @see Math#cosh(double)
*/
public abstract UnaryFunction cosh();
/**
* /
*/
public abstract BinaryFunction divide();
public abstract UnaryFunction enforce(NumberContext context);
/**
* @see Math#exp(double)
*/
public abstract UnaryFunction exp();
/**
* @see Math#expm1(double)
*/
public abstract UnaryFunction expm1();
/**
* @see Math#floor(double)
*/
public abstract UnaryFunction floor();
/**
* @see Math#hypot(double, double)
*/
public abstract BinaryFunction hypot();
public abstract UnaryFunction invert();
/**
* @see Math#log(double)
*/
public abstract UnaryFunction log();
/**
* @see Math#log10(double)
*/
public abstract UnaryFunction log10();
/**
* @see Math#log1p(double)
*/
public abstract UnaryFunction log1p();
/**
* Standard logistic sigmoid function
*/
public abstract UnaryFunction logistic();
public abstract UnaryFunction logit();
/**
* @see Math#max(double, double)
*/
public abstract BinaryFunction max();
/**
* @see Math#min(double, double)
*/
public abstract BinaryFunction min();
/**
* *
*/
public abstract BinaryFunction multiply();
public abstract UnaryFunction negate();
/**
* @see Math#pow(double, double)
*/
public abstract BinaryFunction pow();
public abstract ParameterFunction power();
/**
* @see Math#rint(double)
*/
public abstract UnaryFunction rint();
public abstract ParameterFunction root();
public abstract ParameterFunction scale();
/**
* @see Math#signum(double)
*/
public abstract UnaryFunction signum();
/**
* @see Math#sin(double)
*/
public abstract UnaryFunction sin();
/**
* @see Math#sinh(double)
*/
public abstract UnaryFunction sinh();
/**
* @see Math#sqrt(double)
*/
public abstract UnaryFunction sqrt();
/**
* @return sqrt(1.0 + x2)
*/
public abstract UnaryFunction sqrt1px2();
/**
* -
*/
public abstract BinaryFunction subtract();
/**
* @see Math#tan(double)
*/
public abstract UnaryFunction tan();
/**
* @see Math#tanh(double)
*/
public abstract UnaryFunction tanh();
public abstract UnaryFunction value();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy