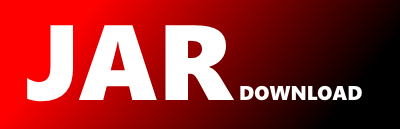
org.omnifaces.persistence.model.BaseEntity Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of omnipersistence Show documentation
Show all versions of omnipersistence Show documentation
Utilities for JPA, JDBC and DataSources
package org.omnifaces.persistence.model;
import static javax.persistence.GenerationType.IDENTITY;
import java.io.Serializable;
import java.util.Objects;
import javax.persistence.EntityListeners;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import javax.persistence.MappedSuperclass;
import org.omnifaces.persistence.listener.BaseEntityListener;
import org.omnifaces.persistence.service.BaseEntityService;
/**
*
* Let all your entities extend from this. Then you can make use of {@link BaseEntityService}. This mapped superclass
* already automatically takes care of the id
column.
*
* There are two more mapped superclasses which may also be of interest.
*
* - {@link TimestampedEntity} - extends {@link BaseEntity} with
created
and lastModified
* columns and automatically takes care of them.
* - {@link VersionedEntity} - extends {@link TimestampedEntity} with a
@Version
column.
*
*
* @param The generic ID type, usually {@link Long}.
* @author Bauke Scholtz
*/
@MappedSuperclass
@EntityListeners(BaseEntityListener.class)
public abstract class BaseEntity & Serializable> implements Comparable>, Identifiable, Serializable {
private static final long serialVersionUID = 1L;
@Id @GeneratedValue(strategy = IDENTITY)
private I id;
@Override
public I getId() {
return id;
}
@Override
public void setId(I id) {
this.id = id;
}
/**
* Hashes by default the classname and ID.
*/
@Override
public int hashCode() {
return (getId() != null)
? Objects.hash(getClass().getSimpleName(), getId())
: super.hashCode();
}
/**
* Compares by default by entity class (proxies taken into account) and ID.
*/
@Override
public boolean equals(Object other) {
return (other != null && getId() != null && other.getClass().isAssignableFrom(getClass()) && getClass().isAssignableFrom(other.getClass()))
? getId().equals(((BaseEntity>) other).getId())
: (other == this);
}
/**
* Orders by default with "nulls last".
*/
@Override
public int compareTo(BaseEntity other) {
return (other == null)
? -1
: (getId() == null)
? (other.getId() == null ? 0 : 1)
: getId().compareTo(other.getId());
}
/**
* The default format is ClassName[id={id}]
.
*/
@Override
public String toString() {
return String.format("%s[id=%d]", getClass().getSimpleName(), getId());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy