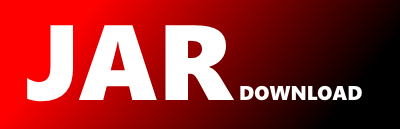
org.omnifaces.persistence.service.RootWrapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of omnipersistence Show documentation
Show all versions of omnipersistence Show documentation
Utilities for JPA, JDBC and DataSources
package org.omnifaces.persistence.service;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Set;
import javax.persistence.criteria.CollectionJoin;
import javax.persistence.criteria.Expression;
import javax.persistence.criteria.Fetch;
import javax.persistence.criteria.From;
import javax.persistence.criteria.Join;
import javax.persistence.criteria.JoinType;
import javax.persistence.criteria.ListJoin;
import javax.persistence.criteria.MapJoin;
import javax.persistence.criteria.Path;
import javax.persistence.criteria.Predicate;
import javax.persistence.criteria.Root;
import javax.persistence.criteria.Selection;
import javax.persistence.criteria.SetJoin;
import javax.persistence.metamodel.CollectionAttribute;
import javax.persistence.metamodel.EntityType;
import javax.persistence.metamodel.ListAttribute;
import javax.persistence.metamodel.MapAttribute;
import javax.persistence.metamodel.PluralAttribute;
import javax.persistence.metamodel.SetAttribute;
import javax.persistence.metamodel.SingularAttribute;
public class RootWrapper implements Root {
private Root wrapped;
public RootWrapper(Root wrapped) {
this.wrapped = wrapped;
}
public Root getWrapped() {
return wrapped;
}
@Override
public Predicate isNull() {
return getWrapped().isNull();
}
@Override
public Class extends X> getJavaType() {
return getWrapped().getJavaType();
}
@Override
public EntityType getModel() {
return getWrapped().getModel();
}
@Override
public Selection alias(String name) {
return getWrapped().alias(name);
}
@Override
public Set> getFetches() {
return getWrapped().getFetches();
}
@Override
public Predicate isNotNull() {
return getWrapped().isNotNull();
}
@Override
public String getAlias() {
return getWrapped().getAlias();
}
@Override
public Predicate in(Object... values) {
return getWrapped().in(values);
}
@Override
public boolean isCompoundSelection() {
return getWrapped().isCompoundSelection();
}
@Override
public Path> getParentPath() {
return getWrapped().getParentPath();
}
@Override
public Fetch fetch(SingularAttribute super X, Y> attribute) {
return getWrapped().fetch(attribute);
}
@Override
public List> getCompoundSelectionItems() {
return getWrapped().getCompoundSelectionItems();
}
@Override
public Path get(SingularAttribute super X, Y> attribute) {
return getWrapped().get(attribute);
}
@Override
public Set> getJoins() {
return getWrapped().getJoins();
}
@Override
public Predicate in(Expression>... values) {
return getWrapped().in(values);
}
@Override
public Fetch fetch(SingularAttribute super X, Y> attribute, JoinType jt) {
return getWrapped().fetch(attribute, jt);
}
@Override
public > Expression get(PluralAttribute collection) {
return getWrapped().get(collection);
}
@Override
public Predicate in(Collection> values) {
return getWrapped().in(values);
}
@Override
public boolean isCorrelated() {
return getWrapped().isCorrelated();
}
@Override
public Fetch fetch(PluralAttribute super X, ?, Y> attribute) {
return getWrapped().fetch(attribute);
}
@Override
public Predicate in(Expression> values) {
return getWrapped().in(values);
}
@Override
public > Expression get(MapAttribute map) {
return getWrapped().get(map);
}
@Override
public From getCorrelationParent() {
return getWrapped().getCorrelationParent();
}
@Override
public Fetch fetch(PluralAttribute super X, ?, Y> attribute, JoinType jt) {
return getWrapped().fetch(attribute, jt);
}
@Override
@SuppressWarnings("hiding")
public Expression as(Class type) {
return getWrapped().as(type);
}
@Override
public Expression> type() {
return getWrapped().type();
}
@Override
@SuppressWarnings("hiding")
public Fetch fetch(String attributeName) {
return getWrapped().fetch(attributeName);
}
@Override
public Join join(SingularAttribute super X, Y> attribute) {
return getWrapped().join(attribute);
}
@Override
public Path get(String attributeName) {
return getWrapped().get(attributeName);
}
@Override
public Join join(SingularAttribute super X, Y> attribute, JoinType jt) {
return getWrapped().join(attribute, jt);
}
@Override
@SuppressWarnings("hiding")
public Fetch fetch(String attributeName, JoinType jt) {
return getWrapped().fetch(attributeName, jt);
}
@Override
public CollectionJoin join(CollectionAttribute super X, Y> collection) {
return getWrapped().join(collection);
}
@Override
public SetJoin join(SetAttribute super X, Y> set) {
return getWrapped().join(set);
}
@Override
public ListJoin join(ListAttribute super X, Y> list) {
return getWrapped().join(list);
}
@Override
public MapJoin join(MapAttribute super X, K, V> map) {
return getWrapped().join(map);
}
@Override
public CollectionJoin join(CollectionAttribute super X, Y> collection, JoinType jt) {
return getWrapped().join(collection, jt);
}
@Override
public SetJoin join(SetAttribute super X, Y> set, JoinType jt) {
return getWrapped().join(set, jt);
}
@Override
public ListJoin join(ListAttribute super X, Y> list, JoinType jt) {
return getWrapped().join(list, jt);
}
@Override
public MapJoin join(MapAttribute super X, K, V> map, JoinType jt) {
return getWrapped().join(map, jt);
}
@Override
@SuppressWarnings("hiding")
public Join join(String attributeName) {
return getWrapped().join(attributeName);
}
@Override
@SuppressWarnings("hiding")
public CollectionJoin joinCollection(String attributeName) {
return getWrapped().joinCollection(attributeName);
}
@Override
@SuppressWarnings("hiding")
public SetJoin joinSet(String attributeName) {
return getWrapped().joinSet(attributeName);
}
@Override
@SuppressWarnings("hiding")
public ListJoin joinList(String attributeName) {
return getWrapped().joinList(attributeName);
}
@Override
@SuppressWarnings("hiding")
public MapJoin joinMap(String attributeName) {
return getWrapped().joinMap(attributeName);
}
@Override
@SuppressWarnings("hiding")
public Join join(String attributeName, JoinType jt) {
return getWrapped().join(attributeName, jt);
}
@Override
@SuppressWarnings("hiding")
public CollectionJoin joinCollection(String attributeName, JoinType jt) {
return getWrapped().joinCollection(attributeName, jt);
}
@Override
@SuppressWarnings("hiding")
public SetJoin joinSet(String attributeName, JoinType jt) {
return getWrapped().joinSet(attributeName, jt);
}
@Override
@SuppressWarnings("hiding")
public ListJoin joinList(String attributeName, JoinType jt) {
return getWrapped().joinList(attributeName, jt);
}
@Override
@SuppressWarnings("hiding")
public MapJoin joinMap(String attributeName, JoinType jt) {
return getWrapped().joinMap(attributeName, jt);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy