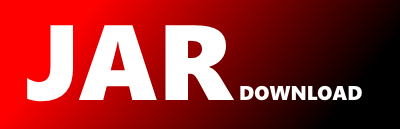
org.omnifaces.utils.text.NameBasedMessageFormat Maven / Gradle / Ivy
/*
* Copyright 2018 OmniFaces
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*/
package org.omnifaces.utils.text;
import static java.util.Collections.unmodifiableList;
import static java.util.Collections.unmodifiableMap;
import static org.omnifaces.utils.text.FormatterUtil.DEFAULT_FORMATTER_FACTORIES;
import java.io.IOException;
import java.io.StringReader;
import java.text.FieldPosition;
import java.text.Format;
import java.text.ParsePosition;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.function.Consumer;
import java.util.function.Function;
public class NameBasedMessageFormat extends Format {
private static final long serialVersionUID = -4520307378273079056L;
private final String pattern;
private final List, String>> segmentFunctions;
private final Map subFormatterFactories;
private final Locale locale;
public NameBasedMessageFormat(String messagePattern) {
this(messagePattern, Locale.getDefault());
}
public NameBasedMessageFormat(String pattern, Locale locale) {
this(pattern, locale, DEFAULT_FORMATTER_FACTORIES);
}
public NameBasedMessageFormat(String pattern, Locale locale, Map subFormatterFactories) {
this.pattern = pattern;
this.segmentFunctions = parsePattern(pattern, locale, subFormatterFactories);
this.subFormatterFactories = copyToUnmodifiableMap(subFormatterFactories);
this.locale = locale;
}
@Override
@SuppressWarnings("unchecked")
public StringBuffer format(Object obj, StringBuffer toAppendTo, FieldPosition pos) {
if (obj instanceof Map) {
format((Map)obj, toAppendTo::append);
return toAppendTo;
}
throw new IllegalArgumentException("Can only format map-based arguments");
}
public String format(Map parameters) {
StringBuilder builder = new StringBuilder();
format(parameters, builder::append);
return builder.toString();
}
public NameBasedMessageFormat withLocale(Locale locale) {
if (this.locale.equals(locale)) {
return this;
}
return new NameBasedMessageFormat(pattern, locale, subFormatterFactories);
}
public NameBasedMessageFormat withPattern(String pattern) {
if (this.pattern.equals(pattern)) {
return this;
}
return new NameBasedMessageFormat(pattern, locale, subFormatterFactories);
}
@Override
public Object parseObject(String source, ParsePosition pos) {
throw new UnsupportedOperationException();
}
public String getPattern() {
return pattern;
}
public Map getSubFormatterFactories() {
return subFormatterFactories;
}
public Locale getLocale() {
return locale;
}
public static String format(String pattern, Map super String, ?> parameters) {
return format(pattern, parameters, Locale.getDefault());
}
public static String format(String pattern, Map super String, ?> parameters, Locale locale) {
return new NameBasedMessageFormat(pattern, locale).format(parameters);
}
public static String format(String pattern, Map super String, ?> parameters, Locale locale, Map formatterFactories) {
return new NameBasedMessageFormat(pattern, locale, formatterFactories).format(parameters);
}
private void format(Map parameters, Consumer toAppendTo) {
segmentFunctions.stream()
.map(function -> function.apply(parameters))
.forEachOrdered(toAppendTo);
}
private static List, String>> parsePattern(String pattern, Locale locale, Map formatterFactories) {
List, String>> segmentFunctions = new ArrayList<>();
try (StringReader reader = new StringReader(pattern)) {
int peek;
while ((peek = peek(reader)) >= 0) {
char nextChar = (char) peek;
if (nextChar == '{') {
segmentFunctions.add(parseFormat(reader, locale, formatterFactories));
}
else {
segmentFunctions.add(readText(reader));
}
}
}
catch (IOException e) {
throw new IllegalArgumentException("Illegal pattern format", e);
}
return unmodifiableList(segmentFunctions);
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy