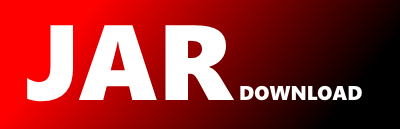
org.onetwo.easyui.EasyModel Maven / Gradle / Ivy
package org.onetwo.easyui;
import java.util.List;
import org.onetwo.common.tree.TreeBuilder;
import org.onetwo.common.utils.map.MappableMap.MappingValueFunc;
import org.onetwo.common.utils.map.ObjectMappingBuilder;
import org.onetwo.easyui.EasyBuilder.SimpleEasyBuilder;
final public class EasyModel {
public static SimpleEasyBuilder newSimpleBuilder(Class clazz){
return new SimpleEasyBuilder();
}
public static EasyTreeBuilder newTreeBuilder(Class clazz){
return new EasyTreeBuilder();
}
public static EasyChildrenTreeModelBuilder newChildrenTreeBuilder(Class clazz){
return new EasyChildrenTreeModelBuilder(ObjectMappingBuilder.newBuilder(clazz, EasyChildrenTreeModel.class));
}
public static EasyComboBoxBuilder newComboBoxBuilder(Class clazz){
return new EasyComboBoxBuilder();
}
public static class EasyChildrenTreeModelBuilder {
private ObjectMappingBuilder builder;
public EasyChildrenTreeModelBuilder(
ObjectMappingBuilder builder) {
super();
this.builder = builder;
}
public EasyChildrenTreeModelBuilder mapId(String fieldName){
builder.addMapping("id", fieldName);
return this;
}
public EasyChildrenTreeModelBuilder mapText(String fieldName){
builder.addMapping("text", fieldName);
return this;
}
public EasyChildrenTreeModelBuilder mapParentId(String fieldName){
builder.addMapping("parentId", fieldName);
return this;
}
public EasyChildrenTreeModelBuilder mapState(String fieldName){
builder.addMapping("state", fieldName);
return this;
}
public EasyChildrenTreeModelBuilder mapIsStateOpen(MappingValueFunc stateFunc){
builder.addMapping("state", src->stateFunc.mapping(src)?"open":"closed");
return this;
}
public EasyChildrenTreeModelBuilder mapChecked(String fieldName){
builder.addMapping("checked", fieldName);
return this;
}
public EasyChildrenTreeModelBuilder mapChecked(MappingValueFunc stateFunc){
builder.addMapping("checked", stateFunc);
return this;
}
public EasyChildrenTreeModel build(List sourceObjects, String rootNode){
List modelList = builder.bindValues(sourceObjects);
List rootTree = new TreeBuilder<>(modelList)
.rootIds(rootNode)
.buidTree();
return rootTree.get(0);
}
}
public static class EasyTreeBuilder extends EasyBuilder, E>{
public EasyTreeBuilder mapId(String fieldName){
addMapping("id", fieldName);
return this;
}
public EasyTreeBuilder mapState(String fieldName){
addMapping("state", fieldName);
return this;
}
public EasyTreeBuilder mapText(String fieldName){
addMapping("text", fieldName);
return this;
}
/***
* true: open
* false: closed
* @param stateFunc
* @return
*/
public EasyTreeBuilder mapIsStateOpen(MappingValueFunc stateFunc){
addMapping("state", src->stateFunc.mapping(src)?"open":"closed");
// addMapping("state", new StateMappingValueFunc(stateFunc));
return this;
}
}
public static class EasyComboBoxBuilder extends EasyBuilder, E>{
public EasyComboBoxBuilder mapValue(String fieldName){
addMapping("value", fieldName);
return this;
}
public EasyComboBoxBuilder mapText(String fieldName){
addMapping("text", fieldName);
return this;
}
public EasyComboBoxBuilder mapSelected(MappingValueFunc selected){
addMapping("selected", selected);
return this;
}
}
private EasyModel(){}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy