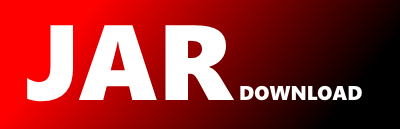
org.onetwo.common.delegate.MutiDelegate Maven / Gradle / Ivy
package org.onetwo.common.delegate;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import org.onetwo.common.utils.CUtils;
import org.onetwo.common.utils.LangUtils;
@SuppressWarnings({"rawtypes"})
public class MutiDelegate {
private Class[] argTypes;
private List delegateMethods;
public MutiDelegate(Class...argTypes){
this.argTypes = argTypes;
this.delegateMethods = new ArrayList();
}
public Class[] getArgTypes() {
return argTypes;
}
public MutiDelegate add(Object target, String method){
DelegateMethodImpl d = DelegateFactory.create(target, method, argTypes);
this.delegateMethods.add(d);
return this;
}
public Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy