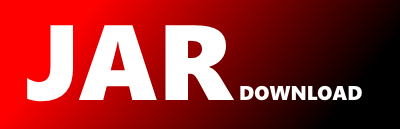
org.onetwo.common.reflect.ReflectUtils Maven / Gradle / Ivy
package org.onetwo.common.reflect;
import java.beans.BeanInfo;
import java.beans.IntrospectionException;
import java.beans.Introspector;
import java.beans.PropertyDescriptor;
import java.lang.annotation.Annotation;
import java.lang.reflect.Constructor;
import java.lang.reflect.Field;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import java.util.ArrayDeque;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.Deque;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.NavigableSet;
import java.util.Queue;
import java.util.Set;
import java.util.SortedSet;
import java.util.TreeSet;
import java.util.function.BiFunction;
import java.util.function.Function;
import org.onetwo.common.annotation.AnnotationUtils;
import org.onetwo.common.convert.Types;
import org.onetwo.common.delegate.DelegateFactory;
import org.onetwo.common.delegate.DelegateMethod;
import org.onetwo.common.exception.BaseException;
import org.onetwo.common.exception.ServiceException;
import org.onetwo.common.expr.Expression;
import org.onetwo.common.expr.ExpressionFacotry;
import org.onetwo.common.expr.ValueProvider;
import org.onetwo.common.log.JFishLoggerFactory;
import org.onetwo.common.reflect.BeanToMapConvertor.BeanToMapBuilder;
import org.onetwo.common.utils.ArrayUtils;
import org.onetwo.common.utils.Assert;
import org.onetwo.common.utils.CUtils;
import org.onetwo.common.utils.ClassUtils;
import org.onetwo.common.utils.CollectionUtils;
import org.onetwo.common.utils.LangUtils;
import org.onetwo.common.utils.StringUtils;
import org.onetwo.common.utils.func.Closure2;
import org.onetwo.common.utils.map.BaseMap;
import org.slf4j.Logger;
@SuppressWarnings( { "rawtypes", "unchecked" })
public class ReflectUtils {
private final static Logger logger = JFishLoggerFactory.getLogger(ReflectUtils.class);
public static interface PropertyDescriptorCallback {
void doWithProperty(PropertyDescriptor propertyDescriptor);
}
public static final CopyConfig IGNORE_BLANK = CopyConfig.create().ignoreIfNoSetMethod(true).ignoreNull().ignoreBlank();
public static final Class>[] EMPTY_CLASSES = new Class[0];
// public static final Class[] EMPTY_CLASS_ARRAY = new Class[0];
// private static WeakHashMap DESCRIPTORS_CACHE = new WeakHashMap();
// private static WeakHashMap> FIELDS_CACHE = new WeakHashMap>();
private static final ClassIntroManager introManager = ClassIntroManager.getInstance();
public static final String READMETHOD_KEY = "get";
public static final String BOOLEAN_READMETHOD_KEY = "is";
public static final String WRITEMETHOD_KEY = "set";
private static final BaseMap, Class>> BASE_TYPE_MAPPER;
private static final BeanToMapConvertor BEAN_TO_MAP_CONVERTOR = new BeanToMapConvertor();
private static final BeanToMapConvertor BEAN_TO_MAP_IGNORE_NULL_CONVERTOR = BeanToMapBuilder.newBuilder()
.propertyAcceptor((p, v)->v!=null)
.build();
static {
BASE_TYPE_MAPPER = new BaseMap, Class>>();
BASE_TYPE_MAPPER.put(boolean.class, Boolean.class);
BASE_TYPE_MAPPER.put(char.class, Character.class);
BASE_TYPE_MAPPER.put(byte.class, Byte.class);
BASE_TYPE_MAPPER.put(short.class, Short.class);
BASE_TYPE_MAPPER.put(int.class, Integer.class);
BASE_TYPE_MAPPER.put(long.class, Long.class);
BASE_TYPE_MAPPER.put(float.class, Float.class);
BASE_TYPE_MAPPER.put(double.class, Double.class);
}
private ReflectUtils() {
}
public static BeanToMapConvertor getBeanToMapConvertor() {
return BEAN_TO_MAP_CONVERTOR;
}
public static void mergeByCheckedIds(
final Collection srcObjects, final Collection checkedIds,
final Class clazz, final String idName) {
Assert.notNull(srcObjects, "srcObjects不能为null");
Assert.hasText(idName, "idName不能为null");
Assert.notNull(clazz, "clazz不能为null");
if (checkedIds == null) {
srcObjects.clear();
return;
}
Iterator srcIterator = srcObjects.iterator();
try {
while (srcIterator.hasNext()) {
T element = srcIterator.next();
Object id;
id = getPropertyValue(element, idName);
if (!checkedIds.contains(id)) {
srcIterator.remove();
} else {
checkedIds.remove(id);
}
}
for (ID id : checkedIds) {
T obj = clazz.newInstance();
setProperty(obj, idName, id);
srcObjects.add(obj);
}
} catch (Exception e) {
handleReflectionException(e);
}
}
public static Collection getProperties(Collection elements, String propName) {
Collection values = new ArrayList(elements.size());
T val = null;
for(Object obj : elements){
val = (T)getPropertyValue(obj, propName);
values.add(val);
}
return values;
}
public static Collection w(Object[] elements, String propName) {
Collection values = new ArrayList(elements.length);
T val = null;
for(Object obj : elements){
val = (T)getPropertyValue(obj, propName);
values.add(val);
}
return values;
}
public static Object getPropertyValue(Object element, String propName) {
return getPropertyValue(element, propName, true);
}
public static Object getPropertyValue(Object element, String propName, boolean throwIfError) {
return getPropertyValue(element, propName, (p, e)->{
logger.error("get ["+element+"] property["+propName+"] error: " + e.getMessage());
if(throwIfError)
throw new BaseException("get ["+element+"] property["+propName+"] error", e);
});
}
public static Object getPropertyValue(Object element, String propName, Closure2 errorHandler) {
if (element instanceof Map) {
return getValue((Map) element, propName);
}
try{
// Intro> info = getIntro(getObjectClass(element));
// PropertyDescriptor pd = info.getProperty(propName);
// return info.getPropertyValue(element, pd);
return getIntro(getObjectClass(element)).getPropertyValue(element, propName);
}catch(Exception e){
/*logger.error("get ["+element+"] property["+propName+"] error: " + e.getMessage());
if(throwIfError)
throw new BaseException("get ["+element+"] property["+propName+"] error", e);*/
errorHandler.execute(propName, e);
}
return null;
}
public static boolean hasProperty(Object element, String propName) {
return ClassIntroManager.getInstance()
.getIntro(getObjectClass(element))
.hasProperty(propName);
}
public static Object getProperty(Object element, PropertyDescriptor prop) {
return invokeMethod(false, ReflectUtils.getReadMethod(element
.getClass(), prop), element);
}
public static void setProperty(Object element, PropertyDescriptor prop, Object val) {
Method wmethod = getWriteMethod(element.getClass(), prop);
if(wmethod==null)
throw new BaseException("not write method: " + prop.getName());
invokeMethod(wmethod, element, LangUtils.tryCastTo(val, prop.getPropertyType()));
}
protected static Object getValue(Map map, String propName) {
return ((Map) map).get(propName);
}
public static void setProperty(Object element, String propName, Object value) {
setProperty(element, propName, value, true);
}
public static void setProperty(Object element, String propName, Object value, boolean throwIfError) {
setProperty(element, propName, value, throwIfError, false);
}
public static void setProperty(Object element, String propName, Object value, boolean throwIfError, boolean skipIfNoSetMethod) {
if (element instanceof Map) {
((Map) element).put(propName, value);
return;
}
PropertyDescriptor prop = getPropertyDescriptor(element, propName);
try {
if (prop == null)
throw new BaseException("can not find the property : " + propName);
if (prop.getPropertyType().isPrimitive() && value == null) {
LangUtils.throwBaseException("the property[" + propName
+ "] type is primitive[" + prop.getPropertyType()
+ "], the value can not be null");
}
if(prop.getWriteMethod()==null){
if(!skipIfNoSetMethod){
throw new NoSuchMethodException("not property[" + propName+"] setter found on class: " + element.getClass());
}else{
return ;
}
}
invokeMethod(prop.getWriteMethod(), element, value);
} catch (Exception e) {
if (throwIfError)
handleReflectionException(e);
}
}
public static void tryToSetProperty(Object element, String propName,
Object value) {
boolean exp = false;
try {
setProperty(element, propName, value, true);
} catch (Exception e) {
exp = true;
}
if (exp) {
exp = false;
try {
String setMethodName = "set"
+ propName.substring(0, 1).toUpperCase()
+ propName.substring(1);
invokeMethod(setMethodName, element, value);
} catch (Exception e) {
exp = true;
}
}
if (exp) {
exp = false;
try {
setFieldValue(propName, element, value);
} catch (Exception e) {
exp = true;
}
}
if (exp)
throw new ServiceException("can not set the property[" + propName
+ "]'s value");
}
public static PropertyDescriptor getPropertyDescriptor(Object element, String propName) {
return getPropertyDescriptor(element.getClass(), propName);
}
public static PropertyDescriptor getPropertyDescriptor(Class> element, String propName) {
/*PropertyDescriptor[] props = desribProperties(element);
for (PropertyDescriptor prop : props) {
if (prop.getName().equals(propName))
return prop;
}*/
return getIntro(element).getProperty(propName);
}
public static boolean isPropertyOf(Object bean, String propName){
if(bean instanceof Map){
return ((Map) bean).containsKey(propName);
}else{
return getPropertyValue(bean, propName)!=null;
}
}
public static List convertElementPropertyToList(
final Collection collection, final String propertyName) {
if (collection == null || collection.isEmpty())
return null;
List list = new ArrayList();
try {
for (Object obj : collection) {
if (obj == null)
continue;
list.add(getPropertyValue(obj, propertyName));
}
} catch (Exception e) {
handleReflectionException(e);
}
return list;
}
public static String convertElementPropertyToString(
final Collection collection, final String propertyName,
final String separator) {
List list = convertElementPropertyToList(collection, propertyName);
return StringUtils.join(list, separator);
}
public static Class> getSuperClassGenricType(final Class> clazz) {
return getSuperClassGenricType(clazz, Object.class);
}
public static Class> getSuperClassGenricType(final Class> clazz,
final Class> stopClass) {
return getSuperClassGenricType(clazz, 0, stopClass);
}
/***************************************************************************
* GenricType handler, copy form spring-side
*
* @param clazz
* @param index
* @param stopClass
* @return
*/
public static Class> getSuperClassGenricType(final Class> clazz,
final int index, final Class> stopClass) {
if(clazz.equals(stopClass))
return clazz;
Type genType = clazz.getGenericSuperclass();
if (!(genType instanceof ParameterizedType)) {
if (stopClass.isAssignableFrom((Class) genType)) {
while (!(genType instanceof ParameterizedType)) {
genType = ((Class) genType).getGenericSuperclass();
if (genType == null)
return Object.class;
}
} else {
logger.warn(clazz.getSimpleName()
+ "'s superclass not ParameterizedType");
return Object.class;
}
}
Type[] params = ((ParameterizedType) genType).getActualTypeArguments();
if (index >= params.length || index < 0) {
logger.warn("Index: " + index + ", Size of "
+ clazz.getSimpleName() + "'s Parameterized Type: "
+ params.length);
return Object.class;
}
if (!(params[index] instanceof Class)) {
logger
.warn(clazz.getSimpleName()
+ " not set the actual class on superclass generic parameter");
return Object.class;
}
return (Class>) params[index];
}
public static Class> getGenricType(final Object obj, final int index) {
return getGenricType(obj, index, Object.class);
}
public static Class> getGenricType(final Object obj, final int index, Class> defaultCLass) {
Class clazz = getObjectClass(obj);
Type genType = null;
if(obj instanceof Type){
genType = (Type) obj;
}else{
genType = (Type) clazz;
}
if (!(genType instanceof ParameterizedType)) {
// logger.warn(clazz.getSimpleName() + "'s class not ParameterizedType");
// return Object.class;
return defaultCLass;
}
Type[] params = ((ParameterizedType) genType).getActualTypeArguments();
if (index >= params.length || index < 0) {
// logger.warn("Index: " + index + ", Size of " + clazz.getSimpleName() + "'s Parameterized Type: " + params.length);
// return Object.class;
return defaultCLass;
}
/*if (!(params[index] instanceof Class)) {
// logger.warn(clazz.getSimpleName() + " not set the actual class on class generic parameter");
return Object.class;
}*/
if(Class.class.isInstance(params[index])){
return (Class) params[index];
}else if(ParameterizedType.class.isInstance(params[index])){
ParameterizedType ptype = (ParameterizedType) params[index];
return (Class)ptype.getRawType();
}else{
// return Object.class;
return defaultCLass;
}
}
public static Class getListGenricType(final Class clazz) {
Class genClass = null;
if (List.class.isAssignableFrom(clazz)) {
Method method = findMethod(clazz, "get", int.class);
if (method != null) {
Type rtype = method.getGenericReturnType();
if (ParameterizedType.class.isAssignableFrom(rtype.getClass())) {
ParameterizedType ptype = (ParameterizedType) rtype;
genClass = (Class) ptype.getActualTypeArguments()[0];
}
}
}
return genClass;
}
public static Method findMethod(Class objClass, String name, Class... paramTypes) {
return findMethod(false, objClass, name, paramTypes);
}
public static Method getReadMethod(Class objClass, PropertyDescriptor pd) {
Method readMethod;
// if (Serializable.class.equals(pd.getPropertyType())) {
if (pd.getReadMethod()==null || pd.getReadMethod().isBridge()) {
readMethod = getReadMethod(objClass, pd.getName(), pd.getPropertyType());
} else {
readMethod = pd.getReadMethod();
}
return readMethod;
}
public static Method getWriteMethod(Class objClass, PropertyDescriptor pd) {
Method writeMethod;
if (pd.getWriteMethod()==null || pd.getWriteMethod().isBridge()) {
writeMethod = getWriteMethod(objClass, pd.getName());
} else {
writeMethod = pd.getWriteMethod();
}
return writeMethod;
}
public static Method getReadMethod(Class objClass, String propName, Class returnType) {
String mName = getReadMethodName(propName, returnType);
return findPublicMethod(objClass, mName);
}
public static Method getWriteMethod(Class objClass, String propName) {
String mName = getWriteMethodName(propName);
return findPublicMethod(objClass, mName);
}
public static String getReadMethodName(String name, Class returnType) {
return Intro.getReadMethodName(name, returnType);
}
public static String getWriteMethodName(String name) {
return Intro.getWriteMethodName(name);
}
public static Method findGetMethod(Class objClass, Field field) {
String mName = StringUtils.capitalize(field.getName());
if (field.getType().equals(Boolean.class)
|| field.getType().equals(boolean.class))
mName = BOOLEAN_READMETHOD_KEY + mName;
else
mName = READMETHOD_KEY + mName;
return findMethod(false, objClass, mName);
}
public static Method findMethod(boolean ignoreIfNotfound, Class objClass, String name, Class... paramTypes) {
Assert.notNull(objClass, "objClass must not be null");
Assert.notNull(name, "Method name must not be null");
try {
Class searchType = objClass;
while (!Object.class.equals(searchType) && searchType != null) {
Method[] methods = (searchType.isInterface() ? searchType
.getMethods() : searchType.getDeclaredMethods());
for (int i = 0; i < methods.length; i++) {
Method method = methods[i];
// System.out.println("====>>>method:"+method+" parent: " +
// method.isBridge());
// if (name.equals(method.getName()) && (paramTypes == null
// || Arrays.equals(paramTypes,
// method.getParameterTypes()))) {
if (!method.isBridge() && name.equals(method.getName()) && (LangUtils.isEmpty(paramTypes) || matchParameterTypes(paramTypes, method.getParameterTypes()))) {
// System.out.println("====>>>method match");
return method;
}
}
searchType = searchType.getSuperclass();
}
} catch (Exception e) {
if (ignoreIfNotfound)
return null;
handleReflectionException(e);
}
if (ignoreIfNotfound)
return null;
throw new BaseException("can not find the method : [class=" + objClass + ", methodName=" + name + ", paramTypes=" + LangUtils.toString(paramTypes) + "]");
}
/***********
* find from declared methods in class or parent class
*
* @param objClass
* @param name
* @return
*/
public static List findMethodsByName(Class objClass, String name, Class... paramTypes) {
Assert.notNull(objClass, "objClass must not be null");
Assert.notNull(name, "Method name must not be null");
List methodList = new ArrayList();
try {
Class searchType = objClass;
while (!Object.class.equals(searchType) && searchType != null) {
Method[] methods = (searchType.isInterface() ? searchType.getMethods() : searchType.getDeclaredMethods());
for (int i = 0; i < methods.length; i++) {
Method method = methods[i];
if (!method.isBridge() && name.equals(method.getName()) && (LangUtils.isEmpty(paramTypes) || matchParameterTypes(paramTypes, method.getParameterTypes())) ) {
methodList.add(method);
}
}
searchType = searchType.getSuperclass();
}
} catch (Exception e) {
logger.error("findMethodsByName ["+name+"] error : "+e.getMessage());
}
return methodList;
}
/***********
*
* find from public methods
*
* @param objClass
* @param name
* @return
*/
public static List findPublicMethods(Class objClass, String name, Class... paramTypes) {
Assert.notNull(objClass, "objClass must not be null");
Assert.notNull(name, "Method name must not be null");
List methodList = new ArrayList();
try {
Method[] methods = objClass.getMethods();
for (int i = 0; i < methods.length; i++) {
Method method = methods[i];
if (!method.isBridge() && name.equals(method.getName()) && (LangUtils.isEmpty(paramTypes) || matchParameterTypes(paramTypes, method.getParameterTypes())) ) {
methodList.add(method);
}
}
} catch (Exception e) {
logger.error("findPublicMethods ["+name+"] method error : "+e.getMessage());
}
return methodList;
}
public static Method findPublicMethod(Class objClass, String name, Class... paramTypes) {
try {
return findPublicMethods(objClass, name, paramTypes).get(0);
} catch (IndexOutOfBoundsException e) {
return null;
}
}
public static List findAnnotationMethods(Class objClass,
Class extends Annotation> annoClasses) {
Assert.notNull(annoClasses);
List methodList = null;
try {
Method[] methods = objClass.getMethods();
for (Method method : methods) {
if (method.getAnnotation(annoClasses) == null)
continue;
if (methodList == null)
methodList = new ArrayList();
methodList.add(method);
}
} catch (Exception e) {
handleReflectionException(e);
}
return methodList == null ? Collections.EMPTY_LIST : methodList;
}
public static Method findUniqueAnnotationMethod(Class objClass,
Class extends Annotation> annoClasses, boolean throwIfMore) {
Assert.notNull(annoClasses);
Method method = null;
try {
Method[] methods = objClass.getMethods();
for (Method m : methods) {
if (m.getAnnotation(annoClasses) == null)
continue;
if (method != null && throwIfMore)
throw new ServiceException("the class[" + objClass
+ "] has more than one method has the annotaiton["
+ annoClasses + "]");
method = m;
}
} catch (Exception e) {
handleReflectionException(e);
}
return method;
}
/***************************************************************************
* reflectionException handle, copy from spring
*
* @param ex
*/
public static void handleReflectionException(Exception ex) {
if (ex instanceof NoSuchMethodException) {
throw new IllegalStateException("Method not found: "
+ ex.getMessage());
}
if (ex instanceof IllegalAccessException) {
throw new IllegalStateException("Could not access method: "
+ ex.getMessage());
}
if (ex instanceof InvocationTargetException) {
handleInvocationTargetException((InvocationTargetException) ex);
}
if (ex instanceof RuntimeException) {
throw (RuntimeException) ex;
}
handleUnexpectedException(ex);
}
public static void handleInvocationTargetException(
InvocationTargetException ex) {
rethrowRuntimeException(ex.getTargetException());
}
public static void rethrowRuntimeException(Throwable ex) {
if (ex instanceof RuntimeException) {
throw (RuntimeException) ex;
}
if (ex instanceof Error) {
throw (Error) ex;
}
handleUnexpectedException(ex);
}
private static void handleUnexpectedException(Throwable ex) {
IllegalStateException isex = new IllegalStateException(
"Unexpected exception thrown");
isex.initCause(ex);
throw isex;
}
public static T newInstance(Class clazz) {
T instance = null;
try {
instance = clazz.newInstance();
} catch (Exception e) {
throw new ServiceException("instantce class error : " + clazz, e);
}
return instance;
}
public static Class> loadClass(String className) {
return loadClass(className, true);
}
public static Class> loadClass(String className, boolean throwIfError) {
Class> clz = null;
try {
// clz = Class.forName(className);
clz = Class.forName(className, true, ClassUtils.getDefaultClassLoader());
} catch (Exception e) {
if(throwIfError)
throw new ServiceException("class not found : " + className, e);
else
logger.warn("class not found : " + className);
}
return clz;
}
public static Class> loadClass(ClassLoader cl, String className) {
Class> clz = null;
try {
clz = cl.loadClass(className);
} catch (ClassNotFoundException e) {
throw new ServiceException("class not found : " + className, e);
}
return clz;
}
public static T newInstance(String className) {
return (T) newInstance(loadClass(className));
}
public static List toInstanceList(String clsNames) {
if (StringUtils.isBlank(clsNames))
return Collections.EMPTY_LIST;
String[] cls = StringUtils.split(clsNames, ",");
T inst = null;
List list = new ArrayList();
for (String c : cls) {
inst = ReflectUtils.newInstance(c);
if (inst != null)
list.add(inst);
}
return list;
}
public static T newInstance(Class clazz, Object... objects) {
T instance = null;
try {
Constructor[] constructs = (Constructor[]) clazz
.getDeclaredConstructors();
boolean appropriateConstractor = false;
for (Constructor constr : constructs) {
if (matchConstructor(constr, objects)) {
constr.setAccessible(true);
instance = constr.newInstance(objects);
appropriateConstractor = true;
break;
}
}
if (appropriateConstractor == false && instance == null) {
StringBuilder sb = new StringBuilder(
"can not find the appropriate constructor, class: ")
.append(clazz.getName()).append(", args: ");
if (objects != null) {
for (Object o : objects)
sb.append(o.getClass().getName()).append(" ");
}
throw new ServiceException(sb.toString());
}
} catch (Exception e) {
throw new ServiceException("instantce class error : " + clazz, e);
}
return instance;
}
public static boolean matchConstructor(Constructor constr,
Object... objects) {
Class[] pclass = constr.getParameterTypes();
if (objects.length != pclass.length)
return false;
int index = 0;
for (Class cls : pclass) {
if (!hasImplements(objects[index], cls))
return false;
index++;
}
return true;
}
public static boolean matchParameterTypes(Class>[] sourceTypes, Class>[] targetTypes) {
if (sourceTypes.length != targetTypes.length)
return false;
int index = 0;
for (Class> cls : targetTypes) {
if (!cls.isAssignableFrom(sourceTypes[index]))
return false;
index++;
}
return true;
}
public static boolean matchParameters(Class[] paramTypes, Object[] params) {
if(LangUtils.isEmpty(params)){
return LangUtils.isEmpty(paramTypes);
}
if (paramTypes.length != params.length)
return false;
int index = 0;
for (Class cls : paramTypes) {
if (!cls.isInstance(params[index])){
if(cls.isPrimitive() && BASE_TYPE_MAPPER.get(cls).isInstance(params[index])){
return true;
}else{
return false;
}
}
index++;
}
return true;
}
public static Class> getBoxingType(Class> primitiveType){
if(!primitiveType.isPrimitive())
return primitiveType;
return BASE_TYPE_MAPPER.get(primitiveType);
}
public static boolean hasImplements(Object obj, Class clazz) {
return clazz.isAssignableFrom(getObjectClass(obj));
}
public static PropertyDescriptor findProperty(Class> clazz,
String propName) {
BeanInfo beanInfo = null;
try {
beanInfo = Introspector.getBeanInfo(clazz, Object.class);
} catch (Exception e) {
throw new ServiceException(e);
}
for (PropertyDescriptor prop : beanInfo.getPropertyDescriptors()) {
if (propName.equals(prop.getName()))
return prop;
}
return null;
}
public static PropertyDescriptor[] desribProperties(Class> clazz) {
/*PropertyDescriptor[] props = DESCRIPTORS_CACHE.get(clazz);
if (props != null)
return props;
BeanInfo beanInfo = null;
try {
beanInfo = Introspector.getBeanInfo(clazz, Object.class);
} catch (Exception e) {
throw new ServiceException(e);
}
props = beanInfo.getPropertyDescriptors();
if (props != null) {
DESCRIPTORS_CACHE.put(clazz, props);
}
return props;*/
return getIntro(clazz).getPropertyArray();
}
public static Map toMap(Object obj) {
return toMap(true, obj);
}
public static Map toMap(boolean ignoreNull, Object obj) {
// return toMap(ignoreNull, obj, null);
return ignoreNull?BEAN_TO_MAP_IGNORE_NULL_CONVERTOR.toMap(obj):BEAN_TO_MAP_CONVERTOR.toMap(obj);
}
public static Map toMap(boolean ignoreNull, Object obj, Function
© 2015 - 2024 Weber Informatics LLC | Privacy Policy