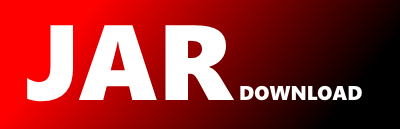
org.onetwo.common.tree.AbstractTreeModel Maven / Gradle / Ivy
package org.onetwo.common.tree;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.onetwo.common.utils.Assert;
@SuppressWarnings({ "unchecked", "serial" })
@XmlType(name="TreeModel")
@XmlAccessorType(XmlAccessType.FIELD)
abstract public class AbstractTreeModel> implements Serializable, TreeModel {
protected Object id;
protected String name;
protected List children = new ArrayList();
protected Object parentId;
protected T parent;
protected Comparable> sort;
protected int level = -1;
protected int index;
public AbstractTreeModel(){
}
public AbstractTreeModel(Object id, String name) {
this(id, name, null);
}
public AbstractTreeModel(Object id, String name, Object parentId) {
Assert.notNull(id, "id must not be null!");
this.id = id;
this.name = name;
this.parentId = parentId;
if(id instanceof Comparable)
this.sort = (Comparable
© 2015 - 2024 Weber Informatics LLC | Privacy Policy