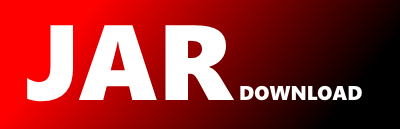
org.onetwo.common.tree.TreeBuilder Maven / Gradle / Ivy
package org.onetwo.common.tree;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.Comparator;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.stream.Collectors;
import org.onetwo.common.utils.CUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.google.common.collect.Lists;
import com.google.common.collect.Sets;
@SuppressWarnings("unchecked")
public class TreeBuilder> {
public static interface ParentNodeNotFoundAction {
Node onParentNodeNotFound(Node currentNode);
}
public static interface RootNodeFunc> {
public boolean isRootNode(T node);
}
public static class RootIdsFunc> implements RootNodeFunc {
final private List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy