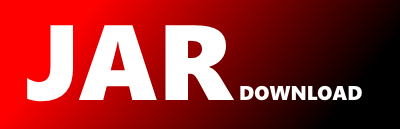
org.onetwo.common.utils.MyUtils Maven / Gradle / Ivy
package org.onetwo.common.utils;
import java.io.File;
import java.io.Serializable;
import java.io.UnsupportedEncodingException;
import java.lang.reflect.Array;
import java.lang.reflect.Field;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Random;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.apache.commons.lang3.BooleanUtils;
import org.onetwo.common.exception.ServiceException;
import org.onetwo.common.log.JFishLoggerFactory;
import org.onetwo.common.reflect.ReflectUtils;
import org.slf4j.Logger;
@SuppressWarnings("unchecked")
public class MyUtils {
protected static Logger logger = JFishLoggerFactory.getLogger(MyUtils.class);
public static final char CHOMP = '\r';
public static final char LINE = '\n';
public static final char SPACE = ' ';
public static final String HTML_BLANK = " ";
public static final String HTML_NEWLINE = "
";
public static final String USER_REGEX = "\\w+([-+.]\\w+)*@\\w+([-.]\\w+)*\\.\\w+([-.]\\w+)*";
public static final Pattern USERNAME_PATTER = Pattern.compile(USER_REGEX);
private MyUtils() {
}
/*
public static T convertValue(Object value, Class toType){
return (T)OgnlOps.convertValue(value, toType);
}*/
public static Object getValue(String expression, Object root){
return getValue(root, expression);
}
public static Object getValue(Object root, String expression){
try {
return ReflectUtils.getExpr(root, expression);
} catch (Exception e){
String msg = "get value error : [expression=" + expression + ", root="+root.getClass()+", msg="+e.getMessage()+"]";
throw new ServiceException(msg, e);
}
}
/*public static Object getValue(Object root, String expression){
try {
return Ognl.getValue(expression, root);
} catch (OgnlException e) {
logger.error("get value error : [expression=" + expression + ", root="+root.getClass()+"]", e);
} catch (Exception e){
String msg = "get value error : [expression=" + expression + ", root="+root.getClass()+", msg="+e.getMessage()+"]";
throw new ServiceException(msg, e);
}
return null;
}*/
/*public static void setValue(Object root, String expression, Object value){
try {
Ognl.setValue(expression, root, value);
} catch (OgnlException e) {
logger.error("set value error : [expression=" + expression + ", root="+root.getClass()+"]", e);
}
}*/
public static void setValue(Object root, String expression, Object value){
try {
ReflectUtils.setExpr(root, expression, value);
} catch (Exception e) {
logger.error("set value error : [expression=" + expression + ", root="+root.getClass()+"]", e);
}
}
public static T simpleConvert(Object val, Class toType){
return simpleConvert(val, toType, null);
}
public static T simpleConvert(Object val, Class toType, T def){
if(val==null)
return def;
Object newValue = null;
if(toType==null)
toType = (Class)String.class;
if(toType.isAssignableFrom(val.getClass()))
return (T)val;
if(val.getClass().isArray()){
if(Array.getLength(val)==0)
return def;
val = Array.get(val, 0);
if(val==null)
return def;
return simpleConvert(val, toType, def);
}
String strVal = val.toString();
if(toType==Long.class || toType==long.class){
newValue = NumberUtils.toLong(strVal);
}else if(toType==Integer.class || toType==int.class){
newValue = NumberUtils.toInt(strVal);
}else if(toType==Double.class || toType==double.class){
newValue = NumberUtils.toDouble(strVal);
}else if(toType==Float.class || toType==float.class){
newValue = NumberUtils.toFloat(strVal);
}else if(toType==Boolean.class || toType==boolean.class){
newValue = BooleanUtils.toBooleanObject(strVal);
}else if(toType==String.class){
newValue = val.toString();
}else{
newValue = val;
}
return (T)newValue;
}
public static boolean checkIdValid(Serializable id){
boolean rs;
if(id==null)
rs = false;
else if(id instanceof Number && ((Number)id).longValue()<1)
rs = false;
else
rs = true;
return rs;
}
public static String getFileExtName(String filepath) {
if(filepath.indexOf('.')!=-1)
return filepath.substring(filepath.lastIndexOf(".") + 1, filepath.length());
return "";
}
public static String getCountSql(String sql){
return getCountSql(sql, "id");
}
public static String getCountSql(String sql, String rep){
String hql = sql;
if(StringUtils.isBlank(rep))
rep = "*";
if(hql.indexOf("{")!=-1 || hql.indexOf("}")!=-1)
hql = hql.replace("{", "").replace("}", "");
if(hql.indexOf(" group by ")!=-1 || hql.indexOf(" distinct ")!=-1){
int index = rep.lastIndexOf('.');
rep = rep.substring(index+1);
hql = "select count(count_entity." + rep + ") from (" + hql + ") count_entity ";
}else{
hql = StringUtils.substringAfter(hql, "from ");
hql = StringUtils.substringBefore(hql, " order by ");
hql = "select count(" + rep + ") from " + hql;
}
return hql;
}
public final static String htmlEncode(String s) {
if (StringUtils.isBlank(s))
return "";
StringBuilder str = new StringBuilder();
for (int j = 0; j < s.length(); j++) {
char c = s.charAt(j);
// encode standard ASCII characters into HTML entities where needed
if (c < '\200') {
switch (c) {
case '"':
str.append(""");
break;
case '&':
str.append("&");
break;
case '<':
str.append("<");
break;
case '>':
str.append(">");
break;
case '\n':
str.append("
");
/*
* case ' ':
* if(Locale.CHINA.getLanguage().equals(StrutsUtils.getCurrentSessionLocale().getLanguage()))
* str.append(" "); else str.append(" "); break;
*/
default:
str.append(c);
}
} else if (c < '\377') {
String hexChars = "0123456789ABCDEF";
int a = c % 16;
int b = (c - a) / 16;
String hex = "" + hexChars.charAt(b) + hexChars.charAt(a);
str.append("" + hex + ";");
} else {
str.append(c);
}
}
return str.toString();
}
public static Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy