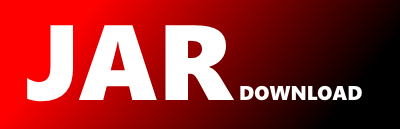
org.onetwo.common.utils.map.CollectionMap Maven / Gradle / Ivy
package org.onetwo.common.utils.map;
import java.util.Collection;
import java.util.HashMap;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.LinkedHashSet;
import java.util.Map;
import java.util.Set;
import java.util.function.Supplier;
import org.onetwo.common.utils.LangUtils;
public class CollectionMap implements Map>{
public static CollectionMap newListMap(){
return new CollectionMap(new HashMap>());
}
public static CollectionMap newListMap(Supplier> collectionCreator){
return new CollectionMap(new HashMap>(), collectionCreator);
}
public static CollectionMap newListMap(int size){
return new CollectionMap(new HashMap>(size));
}
public static CollectionMap newLinkedListMap(){
return new CollectionMap(new LinkedHashMap>());
}
public static CollectionMap newLinkedListMap(Supplier> collectionCreator){
return new CollectionMap(new LinkedHashMap>(), collectionCreator);
}
public static CollectionMap newListMap(Map> map){
return new CollectionMap(map);
}
private Map> map;
private Supplier> collectionCreator = ()->new LinkedHashSet();
protected CollectionMap(){
this(new LinkedHashMap>());
}
protected CollectionMap(Map> map) {
super();
if(map!=null){
this.map = map;
}else{
this.map = new LinkedHashMap<>();
}
}
public CollectionMap(Map> map, Supplier> collectionCreator) {
super();
this.map = map;
this.collectionCreator = collectionCreator;
}
public boolean putElement(K key, V value){
Collection list = get(key);
if(list==null){
list = createCollection();
map.put(key, list);
}
return list.add(value);
}
private Collection createCollection(){
return collectionCreator.get();
}
public V removeFirst(K key){
Collection list = get(key);
if(LangUtils.isEmpty(list)){
return null;
}
Iterator it = list.iterator();
V v = it.next();
it.remove();
/*if(list.isEmpty()){
map.remove(key);
}*/
return v;
}
public Collection putElements(K key, V... values){
Collection list = get(key);
if(list==null){
list = createCollection();
map.put(key, list);
}
for(V v : values)
list.add(v);
return list;
}
public int size() {
return map.size();
}
public boolean isEmpty() {
return map.isEmpty();
}
public boolean containsKey(Object key) {
return map.containsKey(key);
}
public boolean containsValue(Object value) {
return map.containsValue(value);
}
public Collection get(Object key) {
return map.get(key);
}
public V getFirstValue(Object key) {
Collection list = map.get(key);
if(LangUtils.isEmpty(list))
return null;
return list.iterator().next();
}
public Collection put(K key, Collection value) {
return map.put(key, value);
}
public Collection remove(Object key) {
return map.remove(key);
}
public void putAll(Map extends K, ? extends Collection> m) {
map.putAll(m);
}
public void clear() {
map.clear();
}
public Set keySet() {
return map.keySet();
}
public Collection> values() {
return map.values();
}
public Set>> entrySet() {
return map.entrySet();
}
public boolean equals(Object o) {
return map.equals(o);
}
public int hashCode() {
return map.hashCode();
}
public String toString() {
StringBuilder str = new StringBuilder("{");
int index = 0;
for(Map.Entry> entry : (Set>>)entrySet()){
if(index!=0)
str.append(", ");
str.append(entry.getKey()).append("=").append(entry.getValue());
index++;
}
str.append("}");
return str.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy