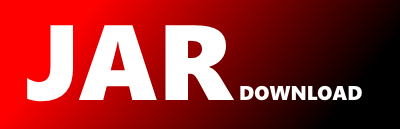
org.onetwo.common.utils.map.KVEntry Maven / Gradle / Ivy
package org.onetwo.common.utils.map;
import java.util.Collections;
import java.util.Map;
import java.util.Map.Entry;
public class KVEntry implements Map.Entry {
public static class ConstantKVEntry extends KVEntry {
public ConstantKVEntry(K key, V val) {
super(key, val);
}
@Override
public void setKey(K key) {
throw new UnsupportedOperationException("read only!");
}
@Override
public V setValue(V value) {
throw new UnsupportedOperationException("read only!");
}
}
public static KVEntry create(Entry entry){
return create(entry.getKey(), entry.getValue(), null);
}
public static KVEntry create(K k, V v){
return create(k, v, null);
}
public static KVEntry create(K k, V v, Map map){
KVEntry entry = new KVEntry(k, v);
if(map!=null)
map.put(k, v);
return entry;
}
public static KVEntry constEntry(K k, V v, Map map){
KVEntry entry = new ConstantKVEntry(k, v);
if(map!=null)
map.put(k, v);
return entry;
}
public static Map freezeMap(Map map){
return Collections.unmodifiableMap(map);
}
private K key;
private V value;
public KVEntry(K key, V val) {
super();
this.key = key;
this.value = val;
}
public K getKey() {
return key;
}
public void setKey(K key) {
this.key = key;
}
public V getValue() {
return value;
}
public V setValue(V value) {
V t = this.value;
this.value = value;
return t;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy