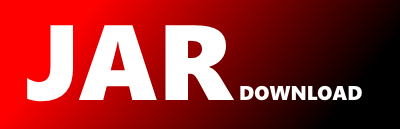
org.onetwo.common.utils.map.ObjectMappingBuilder Maven / Gradle / Ivy
package org.onetwo.common.utils.map;
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
import org.onetwo.common.reflect.ReflectUtils;
import org.onetwo.common.utils.map.MappableMap.MappingInfo;
import org.onetwo.common.utils.map.MappableMap.MappingValueFunc;
public class ObjectMappingBuilder {
public static ObjectMappingBuilder newBuilder(Class srcClass, Class targetClass){
return new ObjectMappingBuilder(srcClass, targetClass);
}
final private List> mappingInfos = new ArrayList<>();
// final private List sourceObjects;
private boolean mapAllFields = true;
@SuppressWarnings("unused")
private Class srcClass;
private Class targetClass;
public ObjectMappingBuilder(Class srcClass, Class targetClass) {
super();
this.srcClass = srcClass;
this.targetClass = targetClass;
}
public ObjectMappingBuilder mapAllFields(){
this.mapAllFields = true;
return this;
}
public ObjectMappingBuilder specifyMappedFields(){
this.mapAllFields = false;
return this;
}
public ObjectMappingBuilder addMapping(String targetFieldName, String srcFieldName){
this.mappingInfos.add(new MappingInfo(targetFieldName, srcFieldName));
return this;
}
public ObjectMappingBuilder addMapping(String targetFieldName, MappingValueFunc valueFunc){
this.mappingInfos.add(new MappingInfo(targetFieldName, valueFunc));
return this;
}
public List bindValues(List sourceObjects){
return sourceObjects.stream().map(obj->bindValue(obj, ReflectUtils.newInstance(targetClass)))
.collect(Collectors.toList());
}
public T bindValue(S sourceObject){
T mappingObject = ReflectUtils.newInstance(targetClass);
return bindValue(sourceObject, mappingObject);
}
public T bindValue(S sourceObject, T mappingObject){
if(mapAllFields){
ReflectUtils.copyExcludes(sourceObject, mappingObject);
}
mappingInfos.stream().forEach(maping->{
if(maping.isMappingValueFunc()){
// mappingObject.put(maping.getJsonFieldName(), maping.getAddMappingValueFunc().mapping(sourceObject));
ReflectUtils.setProperty(mappingObject, maping.getJsonFieldName(), maping.getAddMappingValueFunc().mapping(sourceObject));
}else{
Object value = ReflectUtils.getPropertyValue(sourceObject, maping.getObjectFieldName());
// mappingObject.put(maping.getJsonFieldName(), value);
ReflectUtils.setProperty(mappingObject, maping.getJsonFieldName(), value);
}
});
return mappingObject;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy