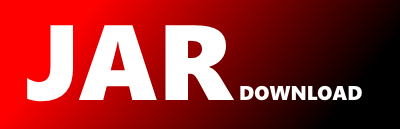
org.onetwo.common.db.BaseCrudEntityManager Maven / Gradle / Ivy
package org.onetwo.common.db;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.stream.Stream;
import org.onetwo.common.db.builder.QueryBuilder;
import org.onetwo.common.db.builder.Querys;
import org.onetwo.common.db.builder.WhereCauseBuilder;
import org.onetwo.common.db.spi.BaseEntityManager;
import org.onetwo.common.db.spi.CrudEntityManager;
import org.onetwo.common.log.JFishLoggerFactory;
import org.onetwo.common.reflect.ReflectUtils;
import org.onetwo.common.spring.Springs;
import org.onetwo.common.utils.Page;
import org.onetwo.dbm.core.spi.DbmEntityManager;
import org.slf4j.Logger;
import org.springframework.transaction.annotation.Transactional;
@SuppressWarnings({"unchecked"})
public class BaseCrudEntityManager implements CrudEntityManager {
protected final Logger logger = JFishLoggerFactory.getLogger(this.getClass());
protected Class entityClass;
protected volatile BaseEntityManager baseEntityManager;
public BaseCrudEntityManager(Class entityClass, BaseEntityManager baseEntityManager){
if(entityClass==null){
this.entityClass = (Class)ReflectUtils.getSuperClassGenricType(this.getClass(), BaseCrudEntityManager.class);
}else{
this.entityClass = entityClass;
}
this.baseEntityManager = baseEntityManager;
}
public BaseCrudEntityManager(BaseEntityManager baseEntityManager){
this((Class)null, baseEntityManager);
}
public BaseEntityManager getBaseEntityManager() {
BaseEntityManager bem = this.baseEntityManager;
if(bem==null){
bem = Springs.getInstance().getBean(BaseEntityManager.class);
Objects.requireNonNull(bem, "BaseEntityManager not found");
if(this.baseEntityManager==null){
this.baseEntityManager = bem;
}
}
return bem;
}
@Transactional
@Override
public int batchInsert(Collection entities) {
return getBaseEntityManager().getSessionFactory().getSession().batchInsert(entities);
}
@Transactional(readOnly=true)
@Override
public T findById(PK id) {
return (T)getBaseEntityManager().findById(entityClass, id);
}
@Transactional(readOnly=true)
@Override
public Optional findOptionalById(PK id) {
return Optional.ofNullable(getBaseEntityManager().findById(entityClass, id));
}
@Transactional(readOnly=true)
@Override
public Number countRecord(Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy