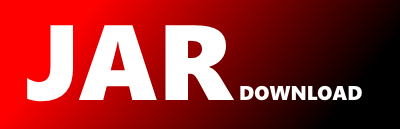
org.onetwo.common.db.BaseEntityManagerAdapter Maven / Gradle / Ivy
The newest version!
package org.onetwo.common.db;
import java.io.Serializable;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import org.onetwo.common.db.builder.QueryBuilder;
import org.onetwo.common.db.spi.QueryWrapper;
import org.onetwo.common.db.spi.SqlParamterPostfixFunctionRegistry;
import org.onetwo.common.db.sql.SequenceNameManager;
import org.onetwo.common.db.sqlext.DeleteExtQuery;
import org.onetwo.common.db.sqlext.ExtQuery.K;
import org.onetwo.common.db.sqlext.SelectExtQuery;
import org.onetwo.common.exception.BaseException;
import org.onetwo.common.exception.ServiceException;
import org.onetwo.common.log.JFishLoggerFactory;
import org.onetwo.common.utils.CUtils;
import org.onetwo.common.utils.LangUtils;
import org.onetwo.common.utils.Page;
import org.slf4j.Logger;
import com.google.common.collect.Lists;
@SuppressWarnings("unchecked")
public abstract class BaseEntityManagerAdapter implements InnerBaseEntityManager {
protected final Logger logger = JFishLoggerFactory.getLogger(this.getClass());
/*@Override
public DataQuery createMappingSQLQuery(String sqlString, String resultSetMapping) {
throw new UnsupportedOperationException("not support operation!");
}*/
public SqlParamterPostfixFunctionRegistry getSqlParamterPostfixFunctionRegistry(){
throw new UnsupportedOperationException("no SqlParamterPostfixFunctionRegistry found!");
}
abstract public SequenceNameManager getSequenceNameManager();
protected Long createSequence(Class> entityClass){
String seqName = getSequenceNameManager().getSequenceName(entityClass);
return createSequence(seqName);
}
/*****
* 创建序列,for oracle
* @param sequenceName
*/
protected Long createSequence(String sequenceName){
String sql = getSequenceNameManager().getSequenceSql(sequenceName, null);
Long id = null;
try {
QueryWrapper dq = this.createQuery(getSequenceNameManager().getCreateSequence(sequenceName), null);
dq.executeUpdate();
dq = this.createQuery(sql, null);
id = ((Number)dq.getSingleResult()).longValue();
} catch (Exception ne) {
throw new ServiceException("createSequences error: " + ne.getMessage(), ne);
}
return id;
}
public Number countRecord(Class> entityClass, Object... params) {
return countRecordByProperties(entityClass, CUtils.asLinkedMap(params));
}
public List findList(QueryBuilder squery) {
return findListByProperties((Class)squery.getEntityClass(), squery.getParams());
}
@Override
public Page findPage(final Page page, QueryBuilder squery){
findPageByProperties((Class)squery.getEntityClass(), page, squery.getParams());
return page;
}
@Override
public List selectFields(Class> entityClass, Object[] selectFields, Object... properties) {
throw new UnsupportedOperationException();
}
@Override
public List selectFieldsToEntity(Class> entityClass, Object[] selectFields, Object... properties){
throw new UnsupportedOperationException();
}
public Number count(SelectExtQuery extQuery) {
extQuery.build();
Number countNumber = (Number)this.findUnique(extQuery.getCountSql(), extQuery.getParamsValue().asMap());
if (countNumber==null) {
countNumber = 0;
}
return countNumber;
}
@Override
public List select(SelectExtQuery extQuery) {
extQuery.build();
return createQuery(extQuery).getResultList();
}
@Override
public int remove(DeleteExtQuery deleteQuery) {
deleteQuery.build();
QueryWrapper q = this.createQuery(deleteQuery.getSql(), deleteQuery.getParamsValue().asMap());
return q.executeUpdate();
}
// @Override
// public T selectOne(SelectExtQuery extQuery) {
// // 限制返回一条
// extQuery.limit(0, 1);
// List list = select(extQuery);
// T entity = null;
// if(LangUtils.hasElement(list))
// entity = list.get(0);
// return entity;
// }
//
// /****
// * 检测数据是否存在,只select id即可
// * @author weishao zeng
// * @param extQuery
// * @return
// */
// @Override
// public boolean exist(SelectExtQuery extQuery) {
// extQuery.selectId();
// Object dataWithId = selectOne(extQuery);
// return dataWithId!=null;
// }
/****
* 查找唯一结果,如果找不到则返回null,找到多个则抛异常 IncorrectResultSizeDataAccessException,详见:DataAccessUtils.requiredSingleResult
*/
@Override
public T selectUnique(SelectExtQuery extQuery) {
extQuery.build();
return (T)createQuery((SelectExtQuery)extQuery).getSingleResult();
}
protected T findUnique(final String sql, final Map values){
T entity = null;
try {
entity = (T)this.createQuery(sql, values).getSingleResult();
}catch(Exception e){
throw new BaseException("find the unique result error : " + sql, e);
}
return entity;
}
public void selectPage(Page page, SelectExtQuery extQuery){
//add
extQuery.build();
if (page.isAutoCount()) {
// Long totalCount = (Long)this.findUnique(extQuery.getCountSql(), (Map)extQuery.getParamsValue().getValues());
Long totalCount = 0l;
Number countNumber = (Number)this.findUnique(extQuery.getCountSql(), extQuery.getParamsValue().asMap());
totalCount = countNumber.longValue();
page.setTotalCount(totalCount);
if(page.getTotalCount()<1){
return ;
}
}
if (page.getPageSize()<=0) {
// 若设置了页数为0,则直接返回
page.setResult(Lists.newArrayList());
return ;
}
List datalist = createQuery(extQuery).setPageParameter(page).getResultList();
if(!page.isAutoCount()){
page.setTotalCount(datalist.size());
}
page.setResult(datalist);
}
protected QueryWrapper createQuery(SelectExtQuery extQuery){
QueryWrapper q = null;
q = this.createQuery(extQuery.getSql(), extQuery.getParamsValue().asMap());
if(extQuery.needSetRange()){
q.setLimited(extQuery.getFirstResult(), extQuery.getMaxResults());
}
q.setQueryConfig(extQuery.getQueryConfig());
q.setLockInfo(extQuery.getLockInfo());
return q;
}
/*@Override
public List select(Class> entityClass, Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy