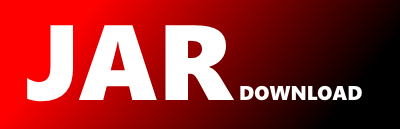
org.onetwo.common.db.builder.DefaultWhereCauseBuilderField Maven / Gradle / Ivy
The newest version!
package org.onetwo.common.db.builder;
import java.util.Collection;
import java.util.Date;
import java.util.Optional;
import java.util.function.Supplier;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import javax.persistence.metamodel.SingularAttribute;
import org.apache.commons.lang3.ArrayUtils;
import org.onetwo.common.db.sqlext.ExtQueryUtils;
import org.onetwo.common.db.sqlext.QueryDSLOps;
import org.onetwo.common.utils.CUtils;
import org.onetwo.common.utils.StringUtils;
import org.onetwo.common.utils.func.Closure;
public class DefaultWhereCauseBuilderField extends WhereCauseBuilderField> {
private String[] fields;
private QueryDSLOps op;
private QueryDSLOps[] ops;
private Object values;
protected Supplier whenPredicate;
// 是否已添加到queryBuilder
// private boolean added;
private boolean autoAddField = true;
public DefaultWhereCauseBuilderField(WhereCauseBuilder squery, String... fields) {
super(squery);
this.fields = fields;
}
public DefaultWhereCauseBuilderField(WhereCauseBuilder squery, SingularAttribute, ?>... fields) {
super(squery);
this.fields = Stream.of(fields)
.map(f->f.getName())
.collect(Collectors.toList())
.toArray(new String[0]);
}
public DefaultWhereCauseBuilderField when(Supplier predicate) {
this.whenPredicate = predicate;
return this;
}
/***
* like '%value'
* @author weishao zeng
* @param values
* @return
*/
public WhereCauseBuilder prelike(String... values) {
return this.doWhenPredicate(()-> {
this.op = QueryDSLOps.LIKE;
this.values = Stream.of(values)
.map(val -> StringUtils.appendStartWith(val, "%"))
.collect(Collectors.toList())
.toArray(new String[0]);
});
// this.op = FieldOP.like;
// this.values = Stream.of(values)
// .map(val -> StringUtils.appendStartWith(val, "%"))
// .collect(Collectors.toList())
// .toArray(new String[0]);
// this.queryBuilder.addField(this);
// return queryBuilder;
}
/***
* like 'value%'
* @author weishao zeng
* @param values
* @return
*/
public WhereCauseBuilder postlike(String... values) {
return this.doWhenPredicate(()-> {
this.op = QueryDSLOps.LIKE;
this.values = Stream.of(values)
.map(val -> StringUtils.appendEndWith(val, "%"))
.collect(Collectors.toList())
.toArray(new String[0]);
});
// this.op = FieldOP.like;
// this.values = Stream.of(values)
// .map(val -> StringUtils.appendEndWith(val, "%"))
// .collect(Collectors.toList())
// .toArray(new String[0]);
// this.queryBuilder.addField(this);
// return queryBuilder;
}
public WhereCauseBuilder notLike(String... values) {
return this.doWhenPredicate(()-> {
this.op = QueryDSLOps.NOT_LIKE;
this.values = values;
});
// this.op = FieldOP.not_like;
// this.values = values;
// this.queryBuilder.addField(this);
// return queryBuilder;
}
public WhereCauseBuilder like(String... values) {
return this.doWhenPredicate(()-> {
this.op = QueryDSLOps.LIKE;
this.values = values;
});
// this.op = FieldOP.like;
// this.values = values;
// this.queryBuilder.addField(this);
// return queryBuilder;
}
/***
* 等于
* @param values
* @return
*/
@SuppressWarnings("unchecked")
public WhereCauseBuilder equalTo(T... values) {
return this.doWhenPredicate(()->{
// this.op = QueryDSLOps.EQ;
this.values = values;
setOp(QueryDSLOps.EQ);
});
}
public WhereCauseBuilder value(QueryDSLOps sqlOp, Supplier
© 2015 - 2025 Weber Informatics LLC | Privacy Policy