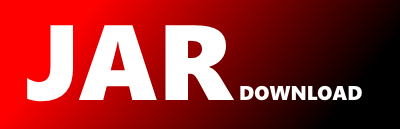
org.onetwo.common.db.filequery.FileBaseNamedQueryInfo Maven / Gradle / Ivy
The newest version!
package org.onetwo.common.db.filequery;
import java.util.List;
import java.util.Map;
import org.onetwo.common.db.DataBase;
import org.onetwo.common.db.spi.FileSqlParserType;
import org.onetwo.common.db.spi.NamedQueryFile;
import org.onetwo.common.db.spi.NamedQueryInfo;
import org.onetwo.common.db.spi.QueryConfigData;
import org.onetwo.common.db.sqlext.ExtQueryUtils;
import org.onetwo.common.propconf.JFishProperties;
import org.onetwo.common.propconf.ResourceAdapter;
import org.onetwo.common.utils.LangUtils;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.Maps;
/****
* 对应每个sql文件里的单个命名查询
* @author way
*
*/
public class FileBaseNamedQueryInfo implements Cloneable, NamedQueryInfo {
public static final char DOT_KEY = '.';
public static final char UNDERLINE_KEY = '_';
public static final String COUNT_POSTFIX = "-count";
public static final String FRAGMENT_KEY = "fragment";
// public static final String MATCHER_KEY = "matcher";
// public static final String MATCHER_SPIT_KEY = "|";
public static final String PROPERTY_KEY = "property";
public static final String NAME_KEY = "name";
public static final String ALIAS_KEY = "alias";
/***
* fragment.
*/
public static final String FRAGMENT_DOT_KEY = FRAGMENT_KEY + DOT_KEY;
public static boolean isCountName(String name){
return name.endsWith(COUNT_POSTFIX);
}
public static String trimCountPostfix(String name){
if(!isCountName(name))
return name;
return name.substring(0, name.length() - COUNT_POSTFIX.length());
}
private String name;
private String value;
private NamedQueryFile dbmNamedQueryFile;
private String namespace;
private JFishProperties config;
private ResourceAdapter> srcfile;
// private DataBase dataBaseType;
// private String mappedEntity;
private String countSql;
private FileSqlParserType parserType = FileSqlParserType.TEMPLATE;
private boolean autoGeneratedCountSql = true;
private Map fragment = LangUtils.newHashMap();
private List aliasList = ImmutableList.of();
// private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy