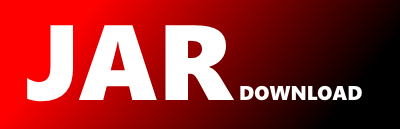
org.onetwo.common.db.spi.BaseEntityManager Maven / Gradle / Ivy
The newest version!
package org.onetwo.common.db.spi;
import java.io.Serializable;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import org.onetwo.common.db.DbmQueryValue;
import org.onetwo.common.db.EntityManagerProvider;
import org.onetwo.common.db.builder.QueryBuilder;
import org.onetwo.common.db.sqlext.SQLSymbolManager;
import org.onetwo.common.utils.Page;
import org.onetwo.dbm.core.spi.DbmSessionFactory;
import org.onetwo.dbm.dialet.DBDialect.LockInfo;
import org.onetwo.dbm.utils.DbmLock;
/****
* 通用的实体查询接口
* ByProperties后缀的方法名一般以Map为参数,其作用和没有ByProperties后缀的一样
* @author way
*
*/
public interface BaseEntityManager {
public T load(Class entityClass, Serializable id);
public T findById(Class entityClass, Serializable id);
/***
* @see org.onetwo.dbm.dialet.DBDialect$LockInfo
* @author wayshall
* @param entityClass
* @param id
* @param lock
* @param timeoutInMillis lock forevaer if null, support: oracle, not support: mysql
* @return return the target or null if not found
*/
public T lock(Class entityClass, Serializable id, DbmLock lock, Integer timeoutInMillis);
default T lockWrite(Class entityClass, Serializable id) {
return lock(entityClass, id, DbmLock.PESSIMISTIC_WRITE, LockInfo.WAIT_FOREVER);
}
default T lockRead(Class entityClass, Serializable id) {
return lock(entityClass, id, DbmLock.PESSIMISTIC_READ, LockInfo.WAIT_FOREVER);
}
public T save(T entity);
public Collection saves(Collection entities);
public void persist(T entity);
/****
* 根据id把实体的所有字段更新到数据库
* @param entity
*/
public void update(Object entity);
/***
* 根据id把实体的非null字段更新到数据库
* @author wayshall
* @param entity
*/
public void dymanicUpdate(Object entity);
/****
* 执行此方法时,若实体实现了逻辑删除接口ILogicDeleteEntity,则只是更新状态
* @param entity
*/
public int remove(Object entity);
/***
* 执行此方法时,若实体实现了逻辑删除接口ILogicDeleteEntity,则只是更新状态
*
* 返回updateCount
* 注意:某些数据库或版本的jdbc driver,批量删除时,updateCount并不正确
* @author wayshall
* @param entities
* @return
*/
public int removes(Collection entities);
/***
* 实际上是removeById的批量操作,如果removeById返回了null,则不会被放到返回的list里
* 可以根据实际返回的list数量和传入的集合数量是否相等来判断是否全部删除
* @author wayshall
* @param entityClass
* @param id
* @return
*/
public Collection removeByIds(Class entityClass, Serializable[] id);
/***
* 执行此方法时,若实体实现了逻辑删除接口ILogicDeleteEntity,则只是更新状态
* 若找不到数据,则抛错
* @author wayshall
* @param entityClass
* @param id
* @return
*/
public T removeById(Class entityClass, Serializable id);
/***
* 物理删除,不判断实体是否实现 ILogicDeleteEntity 接口
* 返回updateCount
* @author wayshall
* @param entityClass
* @return
*/
public int removeAll(Class> entityClass);
/***
* 逻辑删除
* @author wayshall
* @param entity
*/
// public void delete(ILogicDeleteEntity entity);
/***
* 逻辑删除
* @author wayshall
* @param entityClass
* @param id
* @return
*/
// public T deleteById(Class entityClass, Serializable id);
public List findAll(Class entityClass);
public Number countRecordByProperties(Class> entityClass, Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy