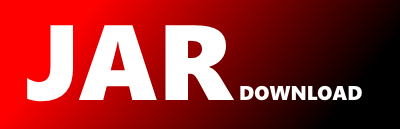
org.onetwo.common.db.sqlext.AbstractSQLSymbolParser Maven / Gradle / Ivy
The newest version!
package org.onetwo.common.db.sqlext;
import java.util.List;
import org.onetwo.common.db.builder.QueryField;
import org.onetwo.common.db.sqlext.ExtQuery.K.IfNull;
import org.onetwo.common.log.JFishLoggerFactory;
import org.onetwo.common.utils.Assert;
import org.onetwo.common.utils.StringUtils;
import org.onetwo.dbm.exception.DbmException;
import org.slf4j.Logger;
/***
* 可用于解释一般的操作符,如=,<,> ……
* @author weishao
*
*/
@SuppressWarnings({"rawtypes"})
abstract public class AbstractSQLSymbolParser implements HqlSymbolParser{
protected Logger logger = JFishLoggerFactory.getLogger(this.getClass());
// protected boolean like;
protected final QueryDSLOps mappedOperator;
protected final String actualOperator;
AbstractSQLSymbolParser(QueryDSLOps symbol){
Assert.notNull(symbol);
this.mappedOperator = symbol;
this.actualOperator = symbol.getActualOperator();
}
AbstractSQLSymbolParser(QueryDSLOps mappedOperator, String actualOperator){
Assert.notNull(mappedOperator);
Assert.hasText(actualOperator, "actual operator must has text!");
this.mappedOperator = mappedOperator;
this.actualOperator = actualOperator;
}
public QueryDSLOps getMappedOperator() {
return mappedOperator;
}
@Override
public String parse(QueryField field) {
return parse(getActualDbOperator(field), field);
}
public String getActualDbOperator(QueryField field){
return actualOperator;
}
/*public String parse(String field, Object value, ParamValues paramValues, IfNull ifNull){
return parse(field, null, value, paramValues, ifNull);
}*/
/*protected void processKey(String field, String symbol, SQLKeys key, StringBuilder hql){
//process SQLKeys
if(SQLKeys.Null==key){
if(FieldOP.eq.equals(symbol)){
hql.append(field).append(" is null ");
}else if(FieldOP.neq.equals(symbol) || FieldOP.neq2.equals(symbol)){
hql.append(field).append(" is not null ");
}else{
LangUtils.throwBaseException("unsupported symbol: " + symbol);
}
}
}*/
final protected IfNull getIfNull(QueryField qfield){
return qfield.getExtQuery().getIfNull();
/*IfNull ifNull = qfield.getIfNull();
if (ifNull==null) {
ifNull = qfield.getExtQuery().getIfNull();
}
return ifNull;*/
}
public String parse(String actualOperator, QueryField qfield){
if(StringUtils.isBlank(actualOperator)) {
throw new DbmException("symbol can not be blank : " + actualOperator);
}
String field = qfield.getActualFieldName();
Object value = qfield.getValue();
ParamValues paramValues = qfield.getExtQuery().getParamsValue();
IfNull ifNull = getIfNull(qfield);
List list = convertValues(field, value, ifNull, true);
field = getFieldName(field);
StringBuilder hql = new StringBuilder();
if(this.subQuery(field, actualOperator, list, paramValues, hql)){
return hql.toString();
}
boolean mutiValue = list.size()>1;
Object v = null;
if(mutiValue) {
hql.append("(");
}
for(int i=0; i convertValues(Object fields, Object values, IfNull ifNull){
return ExtQueryUtils.processValue(fields, values, ifNull, false);
}
protected List convertValues(Object fields, Object values, IfNull ifNull, boolean trimNull){
return ExtQueryUtils.processValue(fields, values, ifNull, trimNull);
}
protected boolean subQuery(String field, String symbol, List paramlist, ParamValues paramValues, StringBuilder hql){
return false;
}
protected String getFieldName(String f){
return f;
}
/*public boolean isLike(){
return like;
}*/
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy