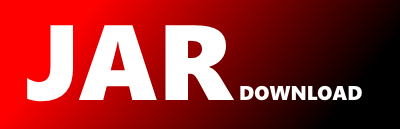
org.onetwo.common.db.sqlext.DateRangeSymbolParser Maven / Gradle / Ivy
package org.onetwo.common.db.sqlext;
import java.util.Date;
import java.util.List;
import org.onetwo.common.date.DateUtils;
import org.onetwo.common.date.NiceDate;
import org.onetwo.common.db.builder.QueryField;
import org.onetwo.common.db.sqlext.ExtQuery.K.IfNull;
import org.onetwo.dbm.exception.DbmException;
/****
* 对in操作符的解释
*
* @author weishao
*
*/
public class DateRangeSymbolParser extends CommonSQLSymbolParser implements HqlSymbolParser {
public DateRangeSymbolParser(SQLSymbolManager sqlSymbolManager, QueryDSLOps symbol){
super(sqlSymbolManager, symbol);
}
@SuppressWarnings("rawtypes")
public String parse(String symbol, QueryField context){
String field = context.getActualFieldName();
Object value = context.getValue();
ParamValues paramValues = context.getExtQuery().getParamsValue();
IfNull ifNull = getIfNull(context);
/*if(value==null || (value instanceof String && StringUtils.isBlank(value.toString())))
return null;
List paramlist = MyUtils.asList(value);
if(paramlist==null || paramlist.isEmpty())
return null;*/
List paramlist = convertValues(field, value, ifNull);
if(paramlist.size()>2)
throw new DbmException("the operator [" + symbol + "] excepted 1 or 2 parameters, acutal: " + paramlist.size());
Date startDate = null;
Date endDate = null;
try {
if(paramlist.size()==1){
Date date = getDate(paramlist.get(0));
if(date!=null){
startDate = DateUtils.setDateStart(date);
// endDate = DateUtil.setDateEnd(date);
endDate = DateUtils.addDay(startDate, 1);
}
}else{
startDate = getDate(paramlist.get(0));
endDate = getDate(paramlist.get(1));
}
} catch (ClassCastException e) {
throw new DbmException("the parameter type of "+symbol+" is error, check it!", e);
}
field = this.getFieldName(field);
StringBuilder hql = new StringBuilder();
hql.append("( ");
if(startDate!=null){
hql.append(field).append(" >= ");
paramValues.addValue(field, startDate, hql);
if(endDate!=null)
hql.append(" and ");
}
if(endDate!=null){
hql.append(field).append(" < ");
paramValues.addValue(field, endDate, hql);
}
hql.append(" ) ");
// if(!this.subQuery(field, symbol, paramlist, paramValues, hql)){
// hql.append("( ");
// if(startDate!=null){
// hql.append(field).append(" >= ");
// paramValues.addValue(field, startDate, hql);
// if(endDate!=null)
// hql.append(" and ");
// }
// if(endDate!=null){
// hql.append(field).append(" < ");
// paramValues.addValue(field, endDate, hql);
// }
// hql.append(" ) ");
// return hql.toString();
// }
return hql.toString();
}
protected Date getDate(Object value){
Date date = null;
if(value instanceof String){
date = DateUtils.date(value.toString());
}else if(value instanceof NiceDate){
date = ((NiceDate)value).getTime();
}else{
date = (Date)value;
}
return date;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy