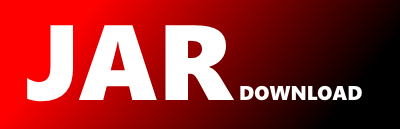
org.onetwo.dbm.jdbc.internal.SimpleArgsPreparedStatementCreator Maven / Gradle / Ivy
package org.onetwo.dbm.jdbc.internal;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import org.onetwo.common.utils.LangUtils;
import org.onetwo.dbm.jdbc.spi.SqlParametersProvider;
import org.springframework.jdbc.core.PreparedStatementCreator;
import org.springframework.jdbc.core.SqlProvider;
import org.springframework.util.Assert;
public class SimpleArgsPreparedStatementCreator implements PreparedStatementCreator, SqlProvider, SqlParametersProvider {
private final String sql;
private final Object[] args;
private String[] columnNames;
private int autoGeneratedKeys = Statement.RETURN_GENERATED_KEYS;
public SimpleArgsPreparedStatementCreator(String sql, Object[] args) {
this(sql, args, (String[])null);
}
public SimpleArgsPreparedStatementCreator(String sql, Object[] args, String... columnNames) {
Assert.notNull(sql, "SQL must not be null");
this.sql = sql;
this.args = args;
this.columnNames = columnNames;
}
public PreparedStatement createPreparedStatement(Connection con) throws SQLException {
if(LangUtils.hasElement(columnNames))
return con.prepareStatement(sql, columnNames);
else
return con.prepareStatement(sql, autoGeneratedKeys);
}
public String getSql() {
return this.sql;
}
public Object[] getSqlParameters() {
return args;
}
public List> getSqlParameterList() {
if(LangUtils.isEmpty(args)){
return Collections.emptyList();
}
return Arrays.asList(args);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy