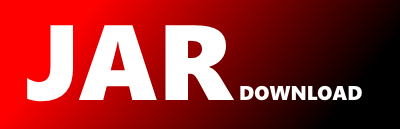
org.onetwo.dbm.richmodel.RichModel Maven / Gradle / Ivy
package org.onetwo.dbm.richmodel;
import java.io.Serializable;
import java.util.Collection;
import java.util.List;
import org.onetwo.common.spring.Springs;
import org.onetwo.common.spring.validator.ValidatorWrapper;
import org.onetwo.common.utils.Page;
import org.onetwo.dbm.core.BaseModel;
import org.onetwo.dbm.exception.NotImplementedDbmOperationException;
abstract public class RichModel extends BaseModel {
protected static Class> obtainStaticEntityClass(){
throw new NotImplementedDbmOperationException();
}
public static int batchInsert(Collection entities){
// return Dbms.obtainCrudManager((Class)obtainStaticEntityClass()).batchInsert(entities);
throw new NotImplementedDbmOperationException();
}
public static Number count(Object...params){
// return Dbms.obtainCrudManager(obtainStaticEntityClass()).countRecord(params);
throw new NotImplementedDbmOperationException();
}
public static List findList(Object... properties){
// return (List)Dbms.obtainCrudManager(obtainStaticEntityClass()).findListByProperties(properties);
throw new NotImplementedDbmOperationException();
}
public static List findListByExample(Object example){
// return Dbms.obtainCrudManager((Class)obtainStaticEntityClass()).findListByExample(example);
throw new NotImplementedDbmOperationException();
}
public static Page findPageByExample(Page page, Object example){
// return Dbms.obtainCrudManager((Class)obtainStaticEntityClass()).findPageByExample(page, example);
// entityManager().findPage(getEntityClass(), page, properties);
throw new NotImplementedDbmOperationException();
}
public static Page findPage(Page page, Object... properties){
// return Dbms.obtainCrudManager((Class)obtainStaticEntityClass()).findPage(page, properties);
// entityManager().findPage(getEntityClass(), page, properties);
throw new NotImplementedDbmOperationException();
}
public static T findById(Serializable id){
// return (T)Dbms.obtainCrudManager(obtainStaticEntityClass()).findById(id);
throw new NotImplementedDbmOperationException();
}
public static T loadById(Serializable id){
// return (T)Dbms.obtainCrudManager(obtainStaticEntityClass()).load(id);
throw new NotImplementedDbmOperationException();
}
public static Collection removeByIds(Serializable[] ids){
// return (Collection)Dbms.obtainCrudManager(obtainStaticEntityClass()).removeByIds(ids);
throw new NotImplementedDbmOperationException();
}
public static T removeById(Serializable id){
// return (T)Dbms.obtainCrudManager(obtainStaticEntityClass()).removeById(id);
throw new NotImplementedDbmOperationException();
}
public static T findOne(Object... properties){
// return (T)Dbms.obtainCrudManager(obtainStaticEntityClass()).findOne(properties);
// return (T)entityManager().findOne(getEntityClass(), properties);
throw new NotImplementedDbmOperationException();
}
protected static ValidatorWrapper getValidator(){
return Springs.getInstance().getValidator();
}
public static int removeAll(){
// return Dbms.obtainCrudManager(obtainStaticEntityClass()).removeAll();
// return entityManager().removeAll(obtainStaticEntityClass());
throw new NotImplementedDbmOperationException();
}
public static boolean exists(Object...params){
Number numb = count(params);
if(numb!=null && numb.longValue()>0)
return true;
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy