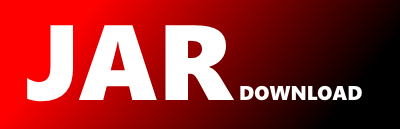
org.onetwo.common.jackson.serializer.BooleanWithLabelSerializer Maven / Gradle / Ivy
The newest version!
package org.onetwo.common.jackson.serializer;
import java.io.IOException;
import org.onetwo.common.exception.BaseException;
import com.fasterxml.jackson.core.JsonGenerator;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.JsonSerializer;
import com.fasterxml.jackson.databind.SerializerProvider;
public class BooleanWithLabelSerializer extends JsonSerializer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy