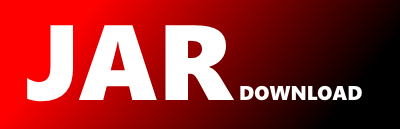
org.onetwo.common.jackson.serializer.UrlJsonSerializer Maven / Gradle / Ivy
package org.onetwo.common.jackson.serializer;
import java.io.IOException;
import java.util.Collection;
import java.util.zip.CRC32;
import com.fasterxml.jackson.core.JsonGenerator;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.JsonToken;
import com.fasterxml.jackson.core.type.WritableTypeId;
import com.fasterxml.jackson.databind.JsonSerializer;
import com.fasterxml.jackson.databind.SerializerProvider;
import com.fasterxml.jackson.databind.jsontype.TypeSerializer;
public class UrlJsonSerializer extends JsonSerializer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy