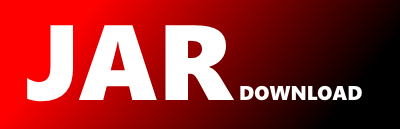
org.onetwo.common.spring.converter.IntStringValueToEnumConverterFactory Maven / Gradle / Ivy
package org.onetwo.common.spring.converter;
import net.jodah.typetools.TypeResolver;
import org.onetwo.common.convert.Types;
import org.springframework.core.convert.converter.Converter;
import org.springframework.core.convert.converter.ConverterFactory;
import org.springframework.util.Assert;
@SuppressWarnings({ "unchecked", "rawtypes" })
public class IntStringValueToEnumConverterFactory implements ConverterFactory {
public Converter getConverter(Class targetType) {
Class> enumType = targetType;
while(enumType != null && !enumType.isEnum()) {
enumType = enumType.getSuperclass();
}
Assert.notNull(enumType, "The target type " + targetType.getName()
+ " does not refer to an enum");
return new StringToEnum(enumType);
}
private class StringToEnum implements Converter {
private final Class enumType;
public StringToEnum(Class enumType) {
this.enumType = enumType;
}
public T convert(String source) {
if (source.length() == 0) {
return null;
}
if(ValueEnum.class.isAssignableFrom(enumType)){
Class genericClass = (Class)TypeResolver.resolveRawArgument(ValueEnum.class, enumType);
T value = Types.convertValue(source, genericClass);
return Types.asValue(value, enumType);
/*Method staticMehtod = ReflectUtils.findMethod(enumType, "valueOf", int.class);
Object val;
try {
val = staticMehtod.invoke(enumType, value);
} catch (Exception e) {
throw new BaseException("convert to int value enum error, does enum type["+enumType+"] has a valueOf(int) method? ", e);
}
return (T) val;*/
}else{
T enumValue = Types.convertValue(source, enumType);
return enumValue;
// try {
// return (T) Enum.valueOf(this.enumType, source.trim());
// } catch (IllegalArgumentException e) {
// try {
// return (T) Enum.valueOf(this.enumType, source.trim().toUpperCase());
// } catch (IllegalArgumentException e2) {
// throw e;
// }
// }
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy