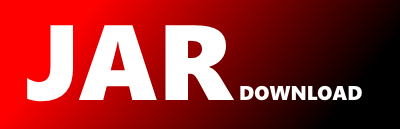
main.org.onflow.protobuf.execution.ExecutionAPIGrpc Maven / Gradle / Ivy
package org.onflow.protobuf.execution;
import static io.grpc.MethodDescriptor.generateFullMethodName;
/**
*
* ExecutionAPI is the API provided by the execution nodes.
*
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.35.0)",
comments = "Source: flow/execution/execution.proto")
public final class ExecutionAPIGrpc {
private ExecutionAPIGrpc() {}
public static final String SERVICE_NAME = "flow.execution.ExecutionAPI";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getPingMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Ping",
requestType = org.onflow.protobuf.execution.Execution.PingRequest.class,
responseType = org.onflow.protobuf.execution.Execution.PingResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getPingMethod() {
io.grpc.MethodDescriptor getPingMethod;
if ((getPingMethod = ExecutionAPIGrpc.getPingMethod) == null) {
synchronized (ExecutionAPIGrpc.class) {
if ((getPingMethod = ExecutionAPIGrpc.getPingMethod) == null) {
ExecutionAPIGrpc.getPingMethod = getPingMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Ping"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.onflow.protobuf.execution.Execution.PingRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.onflow.protobuf.execution.Execution.PingResponse.getDefaultInstance()))
.setSchemaDescriptor(new ExecutionAPIMethodDescriptorSupplier("Ping"))
.build();
}
}
}
return getPingMethod;
}
private static volatile io.grpc.MethodDescriptor getGetAccountAtBlockIDMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetAccountAtBlockID",
requestType = org.onflow.protobuf.execution.Execution.GetAccountAtBlockIDRequest.class,
responseType = org.onflow.protobuf.execution.Execution.GetAccountAtBlockIDResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetAccountAtBlockIDMethod() {
io.grpc.MethodDescriptor getGetAccountAtBlockIDMethod;
if ((getGetAccountAtBlockIDMethod = ExecutionAPIGrpc.getGetAccountAtBlockIDMethod) == null) {
synchronized (ExecutionAPIGrpc.class) {
if ((getGetAccountAtBlockIDMethod = ExecutionAPIGrpc.getGetAccountAtBlockIDMethod) == null) {
ExecutionAPIGrpc.getGetAccountAtBlockIDMethod = getGetAccountAtBlockIDMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetAccountAtBlockID"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.onflow.protobuf.execution.Execution.GetAccountAtBlockIDRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.onflow.protobuf.execution.Execution.GetAccountAtBlockIDResponse.getDefaultInstance()))
.setSchemaDescriptor(new ExecutionAPIMethodDescriptorSupplier("GetAccountAtBlockID"))
.build();
}
}
}
return getGetAccountAtBlockIDMethod;
}
private static volatile io.grpc.MethodDescriptor getExecuteScriptAtBlockIDMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ExecuteScriptAtBlockID",
requestType = org.onflow.protobuf.execution.Execution.ExecuteScriptAtBlockIDRequest.class,
responseType = org.onflow.protobuf.execution.Execution.ExecuteScriptAtBlockIDResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getExecuteScriptAtBlockIDMethod() {
io.grpc.MethodDescriptor getExecuteScriptAtBlockIDMethod;
if ((getExecuteScriptAtBlockIDMethod = ExecutionAPIGrpc.getExecuteScriptAtBlockIDMethod) == null) {
synchronized (ExecutionAPIGrpc.class) {
if ((getExecuteScriptAtBlockIDMethod = ExecutionAPIGrpc.getExecuteScriptAtBlockIDMethod) == null) {
ExecutionAPIGrpc.getExecuteScriptAtBlockIDMethod = getExecuteScriptAtBlockIDMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ExecuteScriptAtBlockID"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.onflow.protobuf.execution.Execution.ExecuteScriptAtBlockIDRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.onflow.protobuf.execution.Execution.ExecuteScriptAtBlockIDResponse.getDefaultInstance()))
.setSchemaDescriptor(new ExecutionAPIMethodDescriptorSupplier("ExecuteScriptAtBlockID"))
.build();
}
}
}
return getExecuteScriptAtBlockIDMethod;
}
private static volatile io.grpc.MethodDescriptor getGetEventsForBlockIDsMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetEventsForBlockIDs",
requestType = org.onflow.protobuf.execution.Execution.GetEventsForBlockIDsRequest.class,
responseType = org.onflow.protobuf.execution.Execution.GetEventsForBlockIDsResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetEventsForBlockIDsMethod() {
io.grpc.MethodDescriptor getGetEventsForBlockIDsMethod;
if ((getGetEventsForBlockIDsMethod = ExecutionAPIGrpc.getGetEventsForBlockIDsMethod) == null) {
synchronized (ExecutionAPIGrpc.class) {
if ((getGetEventsForBlockIDsMethod = ExecutionAPIGrpc.getGetEventsForBlockIDsMethod) == null) {
ExecutionAPIGrpc.getGetEventsForBlockIDsMethod = getGetEventsForBlockIDsMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetEventsForBlockIDs"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.onflow.protobuf.execution.Execution.GetEventsForBlockIDsRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.onflow.protobuf.execution.Execution.GetEventsForBlockIDsResponse.getDefaultInstance()))
.setSchemaDescriptor(new ExecutionAPIMethodDescriptorSupplier("GetEventsForBlockIDs"))
.build();
}
}
}
return getGetEventsForBlockIDsMethod;
}
private static volatile io.grpc.MethodDescriptor getGetTransactionResultMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetTransactionResult",
requestType = org.onflow.protobuf.execution.Execution.GetTransactionResultRequest.class,
responseType = org.onflow.protobuf.execution.Execution.GetTransactionResultResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetTransactionResultMethod() {
io.grpc.MethodDescriptor getGetTransactionResultMethod;
if ((getGetTransactionResultMethod = ExecutionAPIGrpc.getGetTransactionResultMethod) == null) {
synchronized (ExecutionAPIGrpc.class) {
if ((getGetTransactionResultMethod = ExecutionAPIGrpc.getGetTransactionResultMethod) == null) {
ExecutionAPIGrpc.getGetTransactionResultMethod = getGetTransactionResultMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetTransactionResult"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.onflow.protobuf.execution.Execution.GetTransactionResultRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.onflow.protobuf.execution.Execution.GetTransactionResultResponse.getDefaultInstance()))
.setSchemaDescriptor(new ExecutionAPIMethodDescriptorSupplier("GetTransactionResult"))
.build();
}
}
}
return getGetTransactionResultMethod;
}
private static volatile io.grpc.MethodDescriptor getGetTransactionResultByIndexMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetTransactionResultByIndex",
requestType = org.onflow.protobuf.execution.Execution.GetTransactionByIndexRequest.class,
responseType = org.onflow.protobuf.execution.Execution.GetTransactionResultResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetTransactionResultByIndexMethod() {
io.grpc.MethodDescriptor getGetTransactionResultByIndexMethod;
if ((getGetTransactionResultByIndexMethod = ExecutionAPIGrpc.getGetTransactionResultByIndexMethod) == null) {
synchronized (ExecutionAPIGrpc.class) {
if ((getGetTransactionResultByIndexMethod = ExecutionAPIGrpc.getGetTransactionResultByIndexMethod) == null) {
ExecutionAPIGrpc.getGetTransactionResultByIndexMethod = getGetTransactionResultByIndexMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetTransactionResultByIndex"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.onflow.protobuf.execution.Execution.GetTransactionByIndexRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.onflow.protobuf.execution.Execution.GetTransactionResultResponse.getDefaultInstance()))
.setSchemaDescriptor(new ExecutionAPIMethodDescriptorSupplier("GetTransactionResultByIndex"))
.build();
}
}
}
return getGetTransactionResultByIndexMethod;
}
private static volatile io.grpc.MethodDescriptor getGetTransactionResultsByBlockIDMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetTransactionResultsByBlockID",
requestType = org.onflow.protobuf.execution.Execution.GetTransactionsByBlockIDRequest.class,
responseType = org.onflow.protobuf.execution.Execution.GetTransactionResultsResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetTransactionResultsByBlockIDMethod() {
io.grpc.MethodDescriptor getGetTransactionResultsByBlockIDMethod;
if ((getGetTransactionResultsByBlockIDMethod = ExecutionAPIGrpc.getGetTransactionResultsByBlockIDMethod) == null) {
synchronized (ExecutionAPIGrpc.class) {
if ((getGetTransactionResultsByBlockIDMethod = ExecutionAPIGrpc.getGetTransactionResultsByBlockIDMethod) == null) {
ExecutionAPIGrpc.getGetTransactionResultsByBlockIDMethod = getGetTransactionResultsByBlockIDMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetTransactionResultsByBlockID"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.onflow.protobuf.execution.Execution.GetTransactionsByBlockIDRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.onflow.protobuf.execution.Execution.GetTransactionResultsResponse.getDefaultInstance()))
.setSchemaDescriptor(new ExecutionAPIMethodDescriptorSupplier("GetTransactionResultsByBlockID"))
.build();
}
}
}
return getGetTransactionResultsByBlockIDMethod;
}
private static volatile io.grpc.MethodDescriptor getGetTransactionErrorMessageMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetTransactionErrorMessage",
requestType = org.onflow.protobuf.execution.Execution.GetTransactionErrorMessageRequest.class,
responseType = org.onflow.protobuf.execution.Execution.GetTransactionErrorMessageResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetTransactionErrorMessageMethod() {
io.grpc.MethodDescriptor getGetTransactionErrorMessageMethod;
if ((getGetTransactionErrorMessageMethod = ExecutionAPIGrpc.getGetTransactionErrorMessageMethod) == null) {
synchronized (ExecutionAPIGrpc.class) {
if ((getGetTransactionErrorMessageMethod = ExecutionAPIGrpc.getGetTransactionErrorMessageMethod) == null) {
ExecutionAPIGrpc.getGetTransactionErrorMessageMethod = getGetTransactionErrorMessageMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetTransactionErrorMessage"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.onflow.protobuf.execution.Execution.GetTransactionErrorMessageRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.onflow.protobuf.execution.Execution.GetTransactionErrorMessageResponse.getDefaultInstance()))
.setSchemaDescriptor(new ExecutionAPIMethodDescriptorSupplier("GetTransactionErrorMessage"))
.build();
}
}
}
return getGetTransactionErrorMessageMethod;
}
private static volatile io.grpc.MethodDescriptor getGetTransactionErrorMessageByIndexMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetTransactionErrorMessageByIndex",
requestType = org.onflow.protobuf.execution.Execution.GetTransactionErrorMessageByIndexRequest.class,
responseType = org.onflow.protobuf.execution.Execution.GetTransactionErrorMessageResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetTransactionErrorMessageByIndexMethod() {
io.grpc.MethodDescriptor getGetTransactionErrorMessageByIndexMethod;
if ((getGetTransactionErrorMessageByIndexMethod = ExecutionAPIGrpc.getGetTransactionErrorMessageByIndexMethod) == null) {
synchronized (ExecutionAPIGrpc.class) {
if ((getGetTransactionErrorMessageByIndexMethod = ExecutionAPIGrpc.getGetTransactionErrorMessageByIndexMethod) == null) {
ExecutionAPIGrpc.getGetTransactionErrorMessageByIndexMethod = getGetTransactionErrorMessageByIndexMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetTransactionErrorMessageByIndex"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.onflow.protobuf.execution.Execution.GetTransactionErrorMessageByIndexRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.onflow.protobuf.execution.Execution.GetTransactionErrorMessageResponse.getDefaultInstance()))
.setSchemaDescriptor(new ExecutionAPIMethodDescriptorSupplier("GetTransactionErrorMessageByIndex"))
.build();
}
}
}
return getGetTransactionErrorMessageByIndexMethod;
}
private static volatile io.grpc.MethodDescriptor getGetTransactionErrorMessagesByBlockIDMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetTransactionErrorMessagesByBlockID",
requestType = org.onflow.protobuf.execution.Execution.GetTransactionErrorMessagesByBlockIDRequest.class,
responseType = org.onflow.protobuf.execution.Execution.GetTransactionErrorMessagesResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetTransactionErrorMessagesByBlockIDMethod() {
io.grpc.MethodDescriptor getGetTransactionErrorMessagesByBlockIDMethod;
if ((getGetTransactionErrorMessagesByBlockIDMethod = ExecutionAPIGrpc.getGetTransactionErrorMessagesByBlockIDMethod) == null) {
synchronized (ExecutionAPIGrpc.class) {
if ((getGetTransactionErrorMessagesByBlockIDMethod = ExecutionAPIGrpc.getGetTransactionErrorMessagesByBlockIDMethod) == null) {
ExecutionAPIGrpc.getGetTransactionErrorMessagesByBlockIDMethod = getGetTransactionErrorMessagesByBlockIDMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetTransactionErrorMessagesByBlockID"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.onflow.protobuf.execution.Execution.GetTransactionErrorMessagesByBlockIDRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.onflow.protobuf.execution.Execution.GetTransactionErrorMessagesResponse.getDefaultInstance()))
.setSchemaDescriptor(new ExecutionAPIMethodDescriptorSupplier("GetTransactionErrorMessagesByBlockID"))
.build();
}
}
}
return getGetTransactionErrorMessagesByBlockIDMethod;
}
private static volatile io.grpc.MethodDescriptor getGetRegisterAtBlockIDMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetRegisterAtBlockID",
requestType = org.onflow.protobuf.execution.Execution.GetRegisterAtBlockIDRequest.class,
responseType = org.onflow.protobuf.execution.Execution.GetRegisterAtBlockIDResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetRegisterAtBlockIDMethod() {
io.grpc.MethodDescriptor getGetRegisterAtBlockIDMethod;
if ((getGetRegisterAtBlockIDMethod = ExecutionAPIGrpc.getGetRegisterAtBlockIDMethod) == null) {
synchronized (ExecutionAPIGrpc.class) {
if ((getGetRegisterAtBlockIDMethod = ExecutionAPIGrpc.getGetRegisterAtBlockIDMethod) == null) {
ExecutionAPIGrpc.getGetRegisterAtBlockIDMethod = getGetRegisterAtBlockIDMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetRegisterAtBlockID"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.onflow.protobuf.execution.Execution.GetRegisterAtBlockIDRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.onflow.protobuf.execution.Execution.GetRegisterAtBlockIDResponse.getDefaultInstance()))
.setSchemaDescriptor(new ExecutionAPIMethodDescriptorSupplier("GetRegisterAtBlockID"))
.build();
}
}
}
return getGetRegisterAtBlockIDMethod;
}
private static volatile io.grpc.MethodDescriptor getGetLatestBlockHeaderMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetLatestBlockHeader",
requestType = org.onflow.protobuf.execution.Execution.GetLatestBlockHeaderRequest.class,
responseType = org.onflow.protobuf.execution.Execution.BlockHeaderResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetLatestBlockHeaderMethod() {
io.grpc.MethodDescriptor getGetLatestBlockHeaderMethod;
if ((getGetLatestBlockHeaderMethod = ExecutionAPIGrpc.getGetLatestBlockHeaderMethod) == null) {
synchronized (ExecutionAPIGrpc.class) {
if ((getGetLatestBlockHeaderMethod = ExecutionAPIGrpc.getGetLatestBlockHeaderMethod) == null) {
ExecutionAPIGrpc.getGetLatestBlockHeaderMethod = getGetLatestBlockHeaderMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetLatestBlockHeader"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.onflow.protobuf.execution.Execution.GetLatestBlockHeaderRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.onflow.protobuf.execution.Execution.BlockHeaderResponse.getDefaultInstance()))
.setSchemaDescriptor(new ExecutionAPIMethodDescriptorSupplier("GetLatestBlockHeader"))
.build();
}
}
}
return getGetLatestBlockHeaderMethod;
}
private static volatile io.grpc.MethodDescriptor getGetBlockHeaderByIDMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetBlockHeaderByID",
requestType = org.onflow.protobuf.execution.Execution.GetBlockHeaderByIDRequest.class,
responseType = org.onflow.protobuf.execution.Execution.BlockHeaderResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetBlockHeaderByIDMethod() {
io.grpc.MethodDescriptor getGetBlockHeaderByIDMethod;
if ((getGetBlockHeaderByIDMethod = ExecutionAPIGrpc.getGetBlockHeaderByIDMethod) == null) {
synchronized (ExecutionAPIGrpc.class) {
if ((getGetBlockHeaderByIDMethod = ExecutionAPIGrpc.getGetBlockHeaderByIDMethod) == null) {
ExecutionAPIGrpc.getGetBlockHeaderByIDMethod = getGetBlockHeaderByIDMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetBlockHeaderByID"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.onflow.protobuf.execution.Execution.GetBlockHeaderByIDRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.onflow.protobuf.execution.Execution.BlockHeaderResponse.getDefaultInstance()))
.setSchemaDescriptor(new ExecutionAPIMethodDescriptorSupplier("GetBlockHeaderByID"))
.build();
}
}
}
return getGetBlockHeaderByIDMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static ExecutionAPIStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public ExecutionAPIStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new ExecutionAPIStub(channel, callOptions);
}
};
return ExecutionAPIStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static ExecutionAPIBlockingStub newBlockingStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public ExecutionAPIBlockingStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new ExecutionAPIBlockingStub(channel, callOptions);
}
};
return ExecutionAPIBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static ExecutionAPIFutureStub newFutureStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public ExecutionAPIFutureStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new ExecutionAPIFutureStub(channel, callOptions);
}
};
return ExecutionAPIFutureStub.newStub(factory, channel);
}
/**
*
* ExecutionAPI is the API provided by the execution nodes.
*
*/
public static abstract class ExecutionAPIImplBase implements io.grpc.BindableService {
/**
*
* Ping is used to check if the access node is alive and healthy.
*
*/
public void ping(org.onflow.protobuf.execution.Execution.PingRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getPingMethod(), responseObserver);
}
/**
*
* GetAccountAtBlockID gets an account by address at the given block ID
*
*/
public void getAccountAtBlockID(org.onflow.protobuf.execution.Execution.GetAccountAtBlockIDRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetAccountAtBlockIDMethod(), responseObserver);
}
/**
*
* ExecuteScriptAtBlockID executes a ready-only Cadence script against the
* execution state at the block with the given ID.
*
*/
public void executeScriptAtBlockID(org.onflow.protobuf.execution.Execution.ExecuteScriptAtBlockIDRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getExecuteScriptAtBlockIDMethod(), responseObserver);
}
/**
*
* GetEventsForBlockIDs retrieves events for all the specified block IDs that
* have the given type
*
*/
public void getEventsForBlockIDs(org.onflow.protobuf.execution.Execution.GetEventsForBlockIDsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetEventsForBlockIDsMethod(), responseObserver);
}
/**
*
* GetTransactionResult gets the result of a transaction.
*
*/
public void getTransactionResult(org.onflow.protobuf.execution.Execution.GetTransactionResultRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetTransactionResultMethod(), responseObserver);
}
/**
*
* GetTransactionResultByIndex gets the result of a transaction at the index.
*
*/
public void getTransactionResultByIndex(org.onflow.protobuf.execution.Execution.GetTransactionByIndexRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetTransactionResultByIndexMethod(), responseObserver);
}
/**
*
* GetTransactionResultByIndex gets the results of all transactions in the
* block ordered by transaction index.
*
*/
public void getTransactionResultsByBlockID(org.onflow.protobuf.execution.Execution.GetTransactionsByBlockIDRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetTransactionResultsByBlockIDMethod(), responseObserver);
}
/**
*
* GetTransactionErrorMessage gets the error messages of a failed transaction by id.
*
*/
public void getTransactionErrorMessage(org.onflow.protobuf.execution.Execution.GetTransactionErrorMessageRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetTransactionErrorMessageMethod(), responseObserver);
}
/**
*
* GetTransactionErrorMessageByIndex gets the error messages of a failed transaction at the index.
*
*/
public void getTransactionErrorMessageByIndex(org.onflow.protobuf.execution.Execution.GetTransactionErrorMessageByIndexRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetTransactionErrorMessageByIndexMethod(), responseObserver);
}
/**
*
* GetTransactionErrorMessagesByBlockID gets the error messages of all failed transactions in the
* block ordered by transaction index.
*
*/
public void getTransactionErrorMessagesByBlockID(org.onflow.protobuf.execution.Execution.GetTransactionErrorMessagesByBlockIDRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetTransactionErrorMessagesByBlockIDMethod(), responseObserver);
}
/**
*
* GetRegisterAtBlockID collects a register at the block with the given ID (if
* available).
*
*/
public void getRegisterAtBlockID(org.onflow.protobuf.execution.Execution.GetRegisterAtBlockIDRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetRegisterAtBlockIDMethod(), responseObserver);
}
/**
*
* GetLatestBlockHeader gets the latest sealed or unsealed block header.
*
*/
public void getLatestBlockHeader(org.onflow.protobuf.execution.Execution.GetLatestBlockHeaderRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetLatestBlockHeaderMethod(), responseObserver);
}
/**
*
* GetBlockHeaderByID gets a block header by ID.
*
*/
public void getBlockHeaderByID(org.onflow.protobuf.execution.Execution.GetBlockHeaderByIDRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetBlockHeaderByIDMethod(), responseObserver);
}
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getPingMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
org.onflow.protobuf.execution.Execution.PingRequest,
org.onflow.protobuf.execution.Execution.PingResponse>(
this, METHODID_PING)))
.addMethod(
getGetAccountAtBlockIDMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
org.onflow.protobuf.execution.Execution.GetAccountAtBlockIDRequest,
org.onflow.protobuf.execution.Execution.GetAccountAtBlockIDResponse>(
this, METHODID_GET_ACCOUNT_AT_BLOCK_ID)))
.addMethod(
getExecuteScriptAtBlockIDMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
org.onflow.protobuf.execution.Execution.ExecuteScriptAtBlockIDRequest,
org.onflow.protobuf.execution.Execution.ExecuteScriptAtBlockIDResponse>(
this, METHODID_EXECUTE_SCRIPT_AT_BLOCK_ID)))
.addMethod(
getGetEventsForBlockIDsMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
org.onflow.protobuf.execution.Execution.GetEventsForBlockIDsRequest,
org.onflow.protobuf.execution.Execution.GetEventsForBlockIDsResponse>(
this, METHODID_GET_EVENTS_FOR_BLOCK_IDS)))
.addMethod(
getGetTransactionResultMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
org.onflow.protobuf.execution.Execution.GetTransactionResultRequest,
org.onflow.protobuf.execution.Execution.GetTransactionResultResponse>(
this, METHODID_GET_TRANSACTION_RESULT)))
.addMethod(
getGetTransactionResultByIndexMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
org.onflow.protobuf.execution.Execution.GetTransactionByIndexRequest,
org.onflow.protobuf.execution.Execution.GetTransactionResultResponse>(
this, METHODID_GET_TRANSACTION_RESULT_BY_INDEX)))
.addMethod(
getGetTransactionResultsByBlockIDMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
org.onflow.protobuf.execution.Execution.GetTransactionsByBlockIDRequest,
org.onflow.protobuf.execution.Execution.GetTransactionResultsResponse>(
this, METHODID_GET_TRANSACTION_RESULTS_BY_BLOCK_ID)))
.addMethod(
getGetTransactionErrorMessageMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
org.onflow.protobuf.execution.Execution.GetTransactionErrorMessageRequest,
org.onflow.protobuf.execution.Execution.GetTransactionErrorMessageResponse>(
this, METHODID_GET_TRANSACTION_ERROR_MESSAGE)))
.addMethod(
getGetTransactionErrorMessageByIndexMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
org.onflow.protobuf.execution.Execution.GetTransactionErrorMessageByIndexRequest,
org.onflow.protobuf.execution.Execution.GetTransactionErrorMessageResponse>(
this, METHODID_GET_TRANSACTION_ERROR_MESSAGE_BY_INDEX)))
.addMethod(
getGetTransactionErrorMessagesByBlockIDMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
org.onflow.protobuf.execution.Execution.GetTransactionErrorMessagesByBlockIDRequest,
org.onflow.protobuf.execution.Execution.GetTransactionErrorMessagesResponse>(
this, METHODID_GET_TRANSACTION_ERROR_MESSAGES_BY_BLOCK_ID)))
.addMethod(
getGetRegisterAtBlockIDMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
org.onflow.protobuf.execution.Execution.GetRegisterAtBlockIDRequest,
org.onflow.protobuf.execution.Execution.GetRegisterAtBlockIDResponse>(
this, METHODID_GET_REGISTER_AT_BLOCK_ID)))
.addMethod(
getGetLatestBlockHeaderMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
org.onflow.protobuf.execution.Execution.GetLatestBlockHeaderRequest,
org.onflow.protobuf.execution.Execution.BlockHeaderResponse>(
this, METHODID_GET_LATEST_BLOCK_HEADER)))
.addMethod(
getGetBlockHeaderByIDMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
org.onflow.protobuf.execution.Execution.GetBlockHeaderByIDRequest,
org.onflow.protobuf.execution.Execution.BlockHeaderResponse>(
this, METHODID_GET_BLOCK_HEADER_BY_ID)))
.build();
}
}
/**
*
* ExecutionAPI is the API provided by the execution nodes.
*
*/
public static final class ExecutionAPIStub extends io.grpc.stub.AbstractAsyncStub {
private ExecutionAPIStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected ExecutionAPIStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new ExecutionAPIStub(channel, callOptions);
}
/**
*
* Ping is used to check if the access node is alive and healthy.
*
*/
public void ping(org.onflow.protobuf.execution.Execution.PingRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getPingMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* GetAccountAtBlockID gets an account by address at the given block ID
*
*/
public void getAccountAtBlockID(org.onflow.protobuf.execution.Execution.GetAccountAtBlockIDRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetAccountAtBlockIDMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* ExecuteScriptAtBlockID executes a ready-only Cadence script against the
* execution state at the block with the given ID.
*
*/
public void executeScriptAtBlockID(org.onflow.protobuf.execution.Execution.ExecuteScriptAtBlockIDRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getExecuteScriptAtBlockIDMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* GetEventsForBlockIDs retrieves events for all the specified block IDs that
* have the given type
*
*/
public void getEventsForBlockIDs(org.onflow.protobuf.execution.Execution.GetEventsForBlockIDsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetEventsForBlockIDsMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* GetTransactionResult gets the result of a transaction.
*
*/
public void getTransactionResult(org.onflow.protobuf.execution.Execution.GetTransactionResultRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetTransactionResultMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* GetTransactionResultByIndex gets the result of a transaction at the index.
*
*/
public void getTransactionResultByIndex(org.onflow.protobuf.execution.Execution.GetTransactionByIndexRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetTransactionResultByIndexMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* GetTransactionResultByIndex gets the results of all transactions in the
* block ordered by transaction index.
*
*/
public void getTransactionResultsByBlockID(org.onflow.protobuf.execution.Execution.GetTransactionsByBlockIDRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetTransactionResultsByBlockIDMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* GetTransactionErrorMessage gets the error messages of a failed transaction by id.
*
*/
public void getTransactionErrorMessage(org.onflow.protobuf.execution.Execution.GetTransactionErrorMessageRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetTransactionErrorMessageMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* GetTransactionErrorMessageByIndex gets the error messages of a failed transaction at the index.
*
*/
public void getTransactionErrorMessageByIndex(org.onflow.protobuf.execution.Execution.GetTransactionErrorMessageByIndexRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetTransactionErrorMessageByIndexMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* GetTransactionErrorMessagesByBlockID gets the error messages of all failed transactions in the
* block ordered by transaction index.
*
*/
public void getTransactionErrorMessagesByBlockID(org.onflow.protobuf.execution.Execution.GetTransactionErrorMessagesByBlockIDRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetTransactionErrorMessagesByBlockIDMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* GetRegisterAtBlockID collects a register at the block with the given ID (if
* available).
*
*/
public void getRegisterAtBlockID(org.onflow.protobuf.execution.Execution.GetRegisterAtBlockIDRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetRegisterAtBlockIDMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* GetLatestBlockHeader gets the latest sealed or unsealed block header.
*
*/
public void getLatestBlockHeader(org.onflow.protobuf.execution.Execution.GetLatestBlockHeaderRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetLatestBlockHeaderMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* GetBlockHeaderByID gets a block header by ID.
*
*/
public void getBlockHeaderByID(org.onflow.protobuf.execution.Execution.GetBlockHeaderByIDRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetBlockHeaderByIDMethod(), getCallOptions()), request, responseObserver);
}
}
/**
*
* ExecutionAPI is the API provided by the execution nodes.
*
*/
public static final class ExecutionAPIBlockingStub extends io.grpc.stub.AbstractBlockingStub {
private ExecutionAPIBlockingStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected ExecutionAPIBlockingStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new ExecutionAPIBlockingStub(channel, callOptions);
}
/**
*
* Ping is used to check if the access node is alive and healthy.
*
*/
public org.onflow.protobuf.execution.Execution.PingResponse ping(org.onflow.protobuf.execution.Execution.PingRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getPingMethod(), getCallOptions(), request);
}
/**
*
* GetAccountAtBlockID gets an account by address at the given block ID
*
*/
public org.onflow.protobuf.execution.Execution.GetAccountAtBlockIDResponse getAccountAtBlockID(org.onflow.protobuf.execution.Execution.GetAccountAtBlockIDRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetAccountAtBlockIDMethod(), getCallOptions(), request);
}
/**
*
* ExecuteScriptAtBlockID executes a ready-only Cadence script against the
* execution state at the block with the given ID.
*
*/
public org.onflow.protobuf.execution.Execution.ExecuteScriptAtBlockIDResponse executeScriptAtBlockID(org.onflow.protobuf.execution.Execution.ExecuteScriptAtBlockIDRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getExecuteScriptAtBlockIDMethod(), getCallOptions(), request);
}
/**
*
* GetEventsForBlockIDs retrieves events for all the specified block IDs that
* have the given type
*
*/
public org.onflow.protobuf.execution.Execution.GetEventsForBlockIDsResponse getEventsForBlockIDs(org.onflow.protobuf.execution.Execution.GetEventsForBlockIDsRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetEventsForBlockIDsMethod(), getCallOptions(), request);
}
/**
*
* GetTransactionResult gets the result of a transaction.
*
*/
public org.onflow.protobuf.execution.Execution.GetTransactionResultResponse getTransactionResult(org.onflow.protobuf.execution.Execution.GetTransactionResultRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetTransactionResultMethod(), getCallOptions(), request);
}
/**
*
* GetTransactionResultByIndex gets the result of a transaction at the index.
*
*/
public org.onflow.protobuf.execution.Execution.GetTransactionResultResponse getTransactionResultByIndex(org.onflow.protobuf.execution.Execution.GetTransactionByIndexRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetTransactionResultByIndexMethod(), getCallOptions(), request);
}
/**
*
* GetTransactionResultByIndex gets the results of all transactions in the
* block ordered by transaction index.
*
*/
public org.onflow.protobuf.execution.Execution.GetTransactionResultsResponse getTransactionResultsByBlockID(org.onflow.protobuf.execution.Execution.GetTransactionsByBlockIDRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetTransactionResultsByBlockIDMethod(), getCallOptions(), request);
}
/**
*
* GetTransactionErrorMessage gets the error messages of a failed transaction by id.
*
*/
public org.onflow.protobuf.execution.Execution.GetTransactionErrorMessageResponse getTransactionErrorMessage(org.onflow.protobuf.execution.Execution.GetTransactionErrorMessageRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetTransactionErrorMessageMethod(), getCallOptions(), request);
}
/**
*
* GetTransactionErrorMessageByIndex gets the error messages of a failed transaction at the index.
*
*/
public org.onflow.protobuf.execution.Execution.GetTransactionErrorMessageResponse getTransactionErrorMessageByIndex(org.onflow.protobuf.execution.Execution.GetTransactionErrorMessageByIndexRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetTransactionErrorMessageByIndexMethod(), getCallOptions(), request);
}
/**
*
* GetTransactionErrorMessagesByBlockID gets the error messages of all failed transactions in the
* block ordered by transaction index.
*
*/
public org.onflow.protobuf.execution.Execution.GetTransactionErrorMessagesResponse getTransactionErrorMessagesByBlockID(org.onflow.protobuf.execution.Execution.GetTransactionErrorMessagesByBlockIDRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetTransactionErrorMessagesByBlockIDMethod(), getCallOptions(), request);
}
/**
*
* GetRegisterAtBlockID collects a register at the block with the given ID (if
* available).
*
*/
public org.onflow.protobuf.execution.Execution.GetRegisterAtBlockIDResponse getRegisterAtBlockID(org.onflow.protobuf.execution.Execution.GetRegisterAtBlockIDRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetRegisterAtBlockIDMethod(), getCallOptions(), request);
}
/**
*
* GetLatestBlockHeader gets the latest sealed or unsealed block header.
*
*/
public org.onflow.protobuf.execution.Execution.BlockHeaderResponse getLatestBlockHeader(org.onflow.protobuf.execution.Execution.GetLatestBlockHeaderRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetLatestBlockHeaderMethod(), getCallOptions(), request);
}
/**
*
* GetBlockHeaderByID gets a block header by ID.
*
*/
public org.onflow.protobuf.execution.Execution.BlockHeaderResponse getBlockHeaderByID(org.onflow.protobuf.execution.Execution.GetBlockHeaderByIDRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetBlockHeaderByIDMethod(), getCallOptions(), request);
}
}
/**
*
* ExecutionAPI is the API provided by the execution nodes.
*
*/
public static final class ExecutionAPIFutureStub extends io.grpc.stub.AbstractFutureStub {
private ExecutionAPIFutureStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected ExecutionAPIFutureStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new ExecutionAPIFutureStub(channel, callOptions);
}
/**
*
* Ping is used to check if the access node is alive and healthy.
*
*/
public com.google.common.util.concurrent.ListenableFuture ping(
org.onflow.protobuf.execution.Execution.PingRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getPingMethod(), getCallOptions()), request);
}
/**
*
* GetAccountAtBlockID gets an account by address at the given block ID
*
*/
public com.google.common.util.concurrent.ListenableFuture getAccountAtBlockID(
org.onflow.protobuf.execution.Execution.GetAccountAtBlockIDRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGetAccountAtBlockIDMethod(), getCallOptions()), request);
}
/**
*
* ExecuteScriptAtBlockID executes a ready-only Cadence script against the
* execution state at the block with the given ID.
*
*/
public com.google.common.util.concurrent.ListenableFuture executeScriptAtBlockID(
org.onflow.protobuf.execution.Execution.ExecuteScriptAtBlockIDRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getExecuteScriptAtBlockIDMethod(), getCallOptions()), request);
}
/**
*
* GetEventsForBlockIDs retrieves events for all the specified block IDs that
* have the given type
*
*/
public com.google.common.util.concurrent.ListenableFuture getEventsForBlockIDs(
org.onflow.protobuf.execution.Execution.GetEventsForBlockIDsRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGetEventsForBlockIDsMethod(), getCallOptions()), request);
}
/**
*
* GetTransactionResult gets the result of a transaction.
*
*/
public com.google.common.util.concurrent.ListenableFuture getTransactionResult(
org.onflow.protobuf.execution.Execution.GetTransactionResultRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGetTransactionResultMethod(), getCallOptions()), request);
}
/**
*
* GetTransactionResultByIndex gets the result of a transaction at the index.
*
*/
public com.google.common.util.concurrent.ListenableFuture getTransactionResultByIndex(
org.onflow.protobuf.execution.Execution.GetTransactionByIndexRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGetTransactionResultByIndexMethod(), getCallOptions()), request);
}
/**
*
* GetTransactionResultByIndex gets the results of all transactions in the
* block ordered by transaction index.
*
*/
public com.google.common.util.concurrent.ListenableFuture getTransactionResultsByBlockID(
org.onflow.protobuf.execution.Execution.GetTransactionsByBlockIDRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGetTransactionResultsByBlockIDMethod(), getCallOptions()), request);
}
/**
*
* GetTransactionErrorMessage gets the error messages of a failed transaction by id.
*
*/
public com.google.common.util.concurrent.ListenableFuture getTransactionErrorMessage(
org.onflow.protobuf.execution.Execution.GetTransactionErrorMessageRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGetTransactionErrorMessageMethod(), getCallOptions()), request);
}
/**
*
* GetTransactionErrorMessageByIndex gets the error messages of a failed transaction at the index.
*
*/
public com.google.common.util.concurrent.ListenableFuture getTransactionErrorMessageByIndex(
org.onflow.protobuf.execution.Execution.GetTransactionErrorMessageByIndexRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGetTransactionErrorMessageByIndexMethod(), getCallOptions()), request);
}
/**
*
* GetTransactionErrorMessagesByBlockID gets the error messages of all failed transactions in the
* block ordered by transaction index.
*
*/
public com.google.common.util.concurrent.ListenableFuture getTransactionErrorMessagesByBlockID(
org.onflow.protobuf.execution.Execution.GetTransactionErrorMessagesByBlockIDRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGetTransactionErrorMessagesByBlockIDMethod(), getCallOptions()), request);
}
/**
*
* GetRegisterAtBlockID collects a register at the block with the given ID (if
* available).
*
*/
public com.google.common.util.concurrent.ListenableFuture getRegisterAtBlockID(
org.onflow.protobuf.execution.Execution.GetRegisterAtBlockIDRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGetRegisterAtBlockIDMethod(), getCallOptions()), request);
}
/**
*
* GetLatestBlockHeader gets the latest sealed or unsealed block header.
*
*/
public com.google.common.util.concurrent.ListenableFuture getLatestBlockHeader(
org.onflow.protobuf.execution.Execution.GetLatestBlockHeaderRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGetLatestBlockHeaderMethod(), getCallOptions()), request);
}
/**
*
* GetBlockHeaderByID gets a block header by ID.
*
*/
public com.google.common.util.concurrent.ListenableFuture getBlockHeaderByID(
org.onflow.protobuf.execution.Execution.GetBlockHeaderByIDRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGetBlockHeaderByIDMethod(), getCallOptions()), request);
}
}
private static final int METHODID_PING = 0;
private static final int METHODID_GET_ACCOUNT_AT_BLOCK_ID = 1;
private static final int METHODID_EXECUTE_SCRIPT_AT_BLOCK_ID = 2;
private static final int METHODID_GET_EVENTS_FOR_BLOCK_IDS = 3;
private static final int METHODID_GET_TRANSACTION_RESULT = 4;
private static final int METHODID_GET_TRANSACTION_RESULT_BY_INDEX = 5;
private static final int METHODID_GET_TRANSACTION_RESULTS_BY_BLOCK_ID = 6;
private static final int METHODID_GET_TRANSACTION_ERROR_MESSAGE = 7;
private static final int METHODID_GET_TRANSACTION_ERROR_MESSAGE_BY_INDEX = 8;
private static final int METHODID_GET_TRANSACTION_ERROR_MESSAGES_BY_BLOCK_ID = 9;
private static final int METHODID_GET_REGISTER_AT_BLOCK_ID = 10;
private static final int METHODID_GET_LATEST_BLOCK_HEADER = 11;
private static final int METHODID_GET_BLOCK_HEADER_BY_ID = 12;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final ExecutionAPIImplBase serviceImpl;
private final int methodId;
MethodHandlers(ExecutionAPIImplBase serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_PING:
serviceImpl.ping((org.onflow.protobuf.execution.Execution.PingRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_ACCOUNT_AT_BLOCK_ID:
serviceImpl.getAccountAtBlockID((org.onflow.protobuf.execution.Execution.GetAccountAtBlockIDRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_EXECUTE_SCRIPT_AT_BLOCK_ID:
serviceImpl.executeScriptAtBlockID((org.onflow.protobuf.execution.Execution.ExecuteScriptAtBlockIDRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_EVENTS_FOR_BLOCK_IDS:
serviceImpl.getEventsForBlockIDs((org.onflow.protobuf.execution.Execution.GetEventsForBlockIDsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_TRANSACTION_RESULT:
serviceImpl.getTransactionResult((org.onflow.protobuf.execution.Execution.GetTransactionResultRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_TRANSACTION_RESULT_BY_INDEX:
serviceImpl.getTransactionResultByIndex((org.onflow.protobuf.execution.Execution.GetTransactionByIndexRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_TRANSACTION_RESULTS_BY_BLOCK_ID:
serviceImpl.getTransactionResultsByBlockID((org.onflow.protobuf.execution.Execution.GetTransactionsByBlockIDRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_TRANSACTION_ERROR_MESSAGE:
serviceImpl.getTransactionErrorMessage((org.onflow.protobuf.execution.Execution.GetTransactionErrorMessageRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_TRANSACTION_ERROR_MESSAGE_BY_INDEX:
serviceImpl.getTransactionErrorMessageByIndex((org.onflow.protobuf.execution.Execution.GetTransactionErrorMessageByIndexRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_TRANSACTION_ERROR_MESSAGES_BY_BLOCK_ID:
serviceImpl.getTransactionErrorMessagesByBlockID((org.onflow.protobuf.execution.Execution.GetTransactionErrorMessagesByBlockIDRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_REGISTER_AT_BLOCK_ID:
serviceImpl.getRegisterAtBlockID((org.onflow.protobuf.execution.Execution.GetRegisterAtBlockIDRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_LATEST_BLOCK_HEADER:
serviceImpl.getLatestBlockHeader((org.onflow.protobuf.execution.Execution.GetLatestBlockHeaderRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_BLOCK_HEADER_BY_ID:
serviceImpl.getBlockHeaderByID((org.onflow.protobuf.execution.Execution.GetBlockHeaderByIDRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
private static abstract class ExecutionAPIBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
ExecutionAPIBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return org.onflow.protobuf.execution.Execution.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("ExecutionAPI");
}
}
private static final class ExecutionAPIFileDescriptorSupplier
extends ExecutionAPIBaseDescriptorSupplier {
ExecutionAPIFileDescriptorSupplier() {}
}
private static final class ExecutionAPIMethodDescriptorSupplier
extends ExecutionAPIBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final String methodName;
ExecutionAPIMethodDescriptorSupplier(String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (ExecutionAPIGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new ExecutionAPIFileDescriptorSupplier())
.addMethod(getPingMethod())
.addMethod(getGetAccountAtBlockIDMethod())
.addMethod(getExecuteScriptAtBlockIDMethod())
.addMethod(getGetEventsForBlockIDsMethod())
.addMethod(getGetTransactionResultMethod())
.addMethod(getGetTransactionResultByIndexMethod())
.addMethod(getGetTransactionResultsByBlockIDMethod())
.addMethod(getGetTransactionErrorMessageMethod())
.addMethod(getGetTransactionErrorMessageByIndexMethod())
.addMethod(getGetTransactionErrorMessagesByBlockIDMethod())
.addMethod(getGetRegisterAtBlockIDMethod())
.addMethod(getGetLatestBlockHeaderMethod())
.addMethod(getGetBlockHeaderByIDMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy