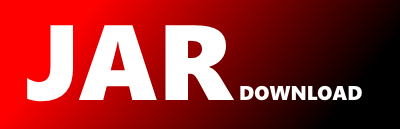
main.org.onflow.protobuf.entities.ExecutionResultOuterClass Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: flow/entities/execution_result.proto
package org.onflow.protobuf.entities;
public final class ExecutionResultOuterClass {
private ExecutionResultOuterClass() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface ExecutionResultOrBuilder extends
// @@protoc_insertion_point(interface_extends:flow.entities.ExecutionResult)
com.google.protobuf.MessageOrBuilder {
/**
* bytes previous_result_id = 1;
* @return The previousResultId.
*/
com.google.protobuf.ByteString getPreviousResultId();
/**
* bytes block_id = 2;
* @return The blockId.
*/
com.google.protobuf.ByteString getBlockId();
/**
* repeated .flow.entities.Chunk chunks = 3;
*/
java.util.List
getChunksList();
/**
* repeated .flow.entities.Chunk chunks = 3;
*/
org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk getChunks(int index);
/**
* repeated .flow.entities.Chunk chunks = 3;
*/
int getChunksCount();
/**
* repeated .flow.entities.Chunk chunks = 3;
*/
java.util.List extends org.onflow.protobuf.entities.ExecutionResultOuterClass.ChunkOrBuilder>
getChunksOrBuilderList();
/**
* repeated .flow.entities.Chunk chunks = 3;
*/
org.onflow.protobuf.entities.ExecutionResultOuterClass.ChunkOrBuilder getChunksOrBuilder(
int index);
/**
* repeated .flow.entities.ServiceEvent service_events = 4;
*/
java.util.List
getServiceEventsList();
/**
* repeated .flow.entities.ServiceEvent service_events = 4;
*/
org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent getServiceEvents(int index);
/**
* repeated .flow.entities.ServiceEvent service_events = 4;
*/
int getServiceEventsCount();
/**
* repeated .flow.entities.ServiceEvent service_events = 4;
*/
java.util.List extends org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEventOrBuilder>
getServiceEventsOrBuilderList();
/**
* repeated .flow.entities.ServiceEvent service_events = 4;
*/
org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEventOrBuilder getServiceEventsOrBuilder(
int index);
/**
* bytes execution_data_id = 5 [deprecated = true];
* @return The executionDataId.
*/
@java.lang.Deprecated com.google.protobuf.ByteString getExecutionDataId();
}
/**
* Protobuf type {@code flow.entities.ExecutionResult}
*/
public static final class ExecutionResult extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:flow.entities.ExecutionResult)
ExecutionResultOrBuilder {
private static final long serialVersionUID = 0L;
// Use ExecutionResult.newBuilder() to construct.
private ExecutionResult(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ExecutionResult() {
previousResultId_ = com.google.protobuf.ByteString.EMPTY;
blockId_ = com.google.protobuf.ByteString.EMPTY;
chunks_ = java.util.Collections.emptyList();
serviceEvents_ = java.util.Collections.emptyList();
executionDataId_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ExecutionResult();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ExecutionResult(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
previousResultId_ = input.readBytes();
break;
}
case 18: {
blockId_ = input.readBytes();
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
chunks_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
chunks_.add(
input.readMessage(org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk.parser(), extensionRegistry));
break;
}
case 34: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
serviceEvents_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
serviceEvents_.add(
input.readMessage(org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent.parser(), extensionRegistry));
break;
}
case 42: {
executionDataId_ = input.readBytes();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
chunks_ = java.util.Collections.unmodifiableList(chunks_);
}
if (((mutable_bitField0_ & 0x00000002) != 0)) {
serviceEvents_ = java.util.Collections.unmodifiableList(serviceEvents_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.onflow.protobuf.entities.ExecutionResultOuterClass.internal_static_flow_entities_ExecutionResult_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.onflow.protobuf.entities.ExecutionResultOuterClass.internal_static_flow_entities_ExecutionResult_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult.class, org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult.Builder.class);
}
public static final int PREVIOUS_RESULT_ID_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString previousResultId_;
/**
* bytes previous_result_id = 1;
* @return The previousResultId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getPreviousResultId() {
return previousResultId_;
}
public static final int BLOCK_ID_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString blockId_;
/**
* bytes block_id = 2;
* @return The blockId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBlockId() {
return blockId_;
}
public static final int CHUNKS_FIELD_NUMBER = 3;
private java.util.List chunks_;
/**
* repeated .flow.entities.Chunk chunks = 3;
*/
@java.lang.Override
public java.util.List getChunksList() {
return chunks_;
}
/**
* repeated .flow.entities.Chunk chunks = 3;
*/
@java.lang.Override
public java.util.List extends org.onflow.protobuf.entities.ExecutionResultOuterClass.ChunkOrBuilder>
getChunksOrBuilderList() {
return chunks_;
}
/**
* repeated .flow.entities.Chunk chunks = 3;
*/
@java.lang.Override
public int getChunksCount() {
return chunks_.size();
}
/**
* repeated .flow.entities.Chunk chunks = 3;
*/
@java.lang.Override
public org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk getChunks(int index) {
return chunks_.get(index);
}
/**
* repeated .flow.entities.Chunk chunks = 3;
*/
@java.lang.Override
public org.onflow.protobuf.entities.ExecutionResultOuterClass.ChunkOrBuilder getChunksOrBuilder(
int index) {
return chunks_.get(index);
}
public static final int SERVICE_EVENTS_FIELD_NUMBER = 4;
private java.util.List serviceEvents_;
/**
* repeated .flow.entities.ServiceEvent service_events = 4;
*/
@java.lang.Override
public java.util.List getServiceEventsList() {
return serviceEvents_;
}
/**
* repeated .flow.entities.ServiceEvent service_events = 4;
*/
@java.lang.Override
public java.util.List extends org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEventOrBuilder>
getServiceEventsOrBuilderList() {
return serviceEvents_;
}
/**
* repeated .flow.entities.ServiceEvent service_events = 4;
*/
@java.lang.Override
public int getServiceEventsCount() {
return serviceEvents_.size();
}
/**
* repeated .flow.entities.ServiceEvent service_events = 4;
*/
@java.lang.Override
public org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent getServiceEvents(int index) {
return serviceEvents_.get(index);
}
/**
* repeated .flow.entities.ServiceEvent service_events = 4;
*/
@java.lang.Override
public org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEventOrBuilder getServiceEventsOrBuilder(
int index) {
return serviceEvents_.get(index);
}
public static final int EXECUTION_DATA_ID_FIELD_NUMBER = 5;
private com.google.protobuf.ByteString executionDataId_;
/**
* bytes execution_data_id = 5 [deprecated = true];
* @return The executionDataId.
*/
@java.lang.Override
@java.lang.Deprecated public com.google.protobuf.ByteString getExecutionDataId() {
return executionDataId_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!previousResultId_.isEmpty()) {
output.writeBytes(1, previousResultId_);
}
if (!blockId_.isEmpty()) {
output.writeBytes(2, blockId_);
}
for (int i = 0; i < chunks_.size(); i++) {
output.writeMessage(3, chunks_.get(i));
}
for (int i = 0; i < serviceEvents_.size(); i++) {
output.writeMessage(4, serviceEvents_.get(i));
}
if (!executionDataId_.isEmpty()) {
output.writeBytes(5, executionDataId_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!previousResultId_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, previousResultId_);
}
if (!blockId_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, blockId_);
}
for (int i = 0; i < chunks_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, chunks_.get(i));
}
for (int i = 0; i < serviceEvents_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, serviceEvents_.get(i));
}
if (!executionDataId_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, executionDataId_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult)) {
return super.equals(obj);
}
org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult other = (org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult) obj;
if (!getPreviousResultId()
.equals(other.getPreviousResultId())) return false;
if (!getBlockId()
.equals(other.getBlockId())) return false;
if (!getChunksList()
.equals(other.getChunksList())) return false;
if (!getServiceEventsList()
.equals(other.getServiceEventsList())) return false;
if (!getExecutionDataId()
.equals(other.getExecutionDataId())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PREVIOUS_RESULT_ID_FIELD_NUMBER;
hash = (53 * hash) + getPreviousResultId().hashCode();
hash = (37 * hash) + BLOCK_ID_FIELD_NUMBER;
hash = (53 * hash) + getBlockId().hashCode();
if (getChunksCount() > 0) {
hash = (37 * hash) + CHUNKS_FIELD_NUMBER;
hash = (53 * hash) + getChunksList().hashCode();
}
if (getServiceEventsCount() > 0) {
hash = (37 * hash) + SERVICE_EVENTS_FIELD_NUMBER;
hash = (53 * hash) + getServiceEventsList().hashCode();
}
hash = (37 * hash) + EXECUTION_DATA_ID_FIELD_NUMBER;
hash = (53 * hash) + getExecutionDataId().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code flow.entities.ExecutionResult}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:flow.entities.ExecutionResult)
org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResultOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.onflow.protobuf.entities.ExecutionResultOuterClass.internal_static_flow_entities_ExecutionResult_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.onflow.protobuf.entities.ExecutionResultOuterClass.internal_static_flow_entities_ExecutionResult_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult.class, org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult.Builder.class);
}
// Construct using org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getChunksFieldBuilder();
getServiceEventsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
previousResultId_ = com.google.protobuf.ByteString.EMPTY;
blockId_ = com.google.protobuf.ByteString.EMPTY;
if (chunksBuilder_ == null) {
chunks_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
chunksBuilder_.clear();
}
if (serviceEventsBuilder_ == null) {
serviceEvents_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
serviceEventsBuilder_.clear();
}
executionDataId_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.onflow.protobuf.entities.ExecutionResultOuterClass.internal_static_flow_entities_ExecutionResult_descriptor;
}
@java.lang.Override
public org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult getDefaultInstanceForType() {
return org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult.getDefaultInstance();
}
@java.lang.Override
public org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult build() {
org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult buildPartial() {
org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult result = new org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult(this);
int from_bitField0_ = bitField0_;
result.previousResultId_ = previousResultId_;
result.blockId_ = blockId_;
if (chunksBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
chunks_ = java.util.Collections.unmodifiableList(chunks_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.chunks_ = chunks_;
} else {
result.chunks_ = chunksBuilder_.build();
}
if (serviceEventsBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
serviceEvents_ = java.util.Collections.unmodifiableList(serviceEvents_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.serviceEvents_ = serviceEvents_;
} else {
result.serviceEvents_ = serviceEventsBuilder_.build();
}
result.executionDataId_ = executionDataId_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult) {
return mergeFrom((org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult other) {
if (other == org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult.getDefaultInstance()) return this;
if (other.getPreviousResultId() != com.google.protobuf.ByteString.EMPTY) {
setPreviousResultId(other.getPreviousResultId());
}
if (other.getBlockId() != com.google.protobuf.ByteString.EMPTY) {
setBlockId(other.getBlockId());
}
if (chunksBuilder_ == null) {
if (!other.chunks_.isEmpty()) {
if (chunks_.isEmpty()) {
chunks_ = other.chunks_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureChunksIsMutable();
chunks_.addAll(other.chunks_);
}
onChanged();
}
} else {
if (!other.chunks_.isEmpty()) {
if (chunksBuilder_.isEmpty()) {
chunksBuilder_.dispose();
chunksBuilder_ = null;
chunks_ = other.chunks_;
bitField0_ = (bitField0_ & ~0x00000001);
chunksBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getChunksFieldBuilder() : null;
} else {
chunksBuilder_.addAllMessages(other.chunks_);
}
}
}
if (serviceEventsBuilder_ == null) {
if (!other.serviceEvents_.isEmpty()) {
if (serviceEvents_.isEmpty()) {
serviceEvents_ = other.serviceEvents_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureServiceEventsIsMutable();
serviceEvents_.addAll(other.serviceEvents_);
}
onChanged();
}
} else {
if (!other.serviceEvents_.isEmpty()) {
if (serviceEventsBuilder_.isEmpty()) {
serviceEventsBuilder_.dispose();
serviceEventsBuilder_ = null;
serviceEvents_ = other.serviceEvents_;
bitField0_ = (bitField0_ & ~0x00000002);
serviceEventsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getServiceEventsFieldBuilder() : null;
} else {
serviceEventsBuilder_.addAllMessages(other.serviceEvents_);
}
}
}
if (other.getExecutionDataId() != com.google.protobuf.ByteString.EMPTY) {
setExecutionDataId(other.getExecutionDataId());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString previousResultId_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes previous_result_id = 1;
* @return The previousResultId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getPreviousResultId() {
return previousResultId_;
}
/**
* bytes previous_result_id = 1;
* @param value The previousResultId to set.
* @return This builder for chaining.
*/
public Builder setPreviousResultId(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
previousResultId_ = value;
onChanged();
return this;
}
/**
* bytes previous_result_id = 1;
* @return This builder for chaining.
*/
public Builder clearPreviousResultId() {
previousResultId_ = getDefaultInstance().getPreviousResultId();
onChanged();
return this;
}
private com.google.protobuf.ByteString blockId_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes block_id = 2;
* @return The blockId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBlockId() {
return blockId_;
}
/**
* bytes block_id = 2;
* @param value The blockId to set.
* @return This builder for chaining.
*/
public Builder setBlockId(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
blockId_ = value;
onChanged();
return this;
}
/**
* bytes block_id = 2;
* @return This builder for chaining.
*/
public Builder clearBlockId() {
blockId_ = getDefaultInstance().getBlockId();
onChanged();
return this;
}
private java.util.List chunks_ =
java.util.Collections.emptyList();
private void ensureChunksIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
chunks_ = new java.util.ArrayList(chunks_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk, org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk.Builder, org.onflow.protobuf.entities.ExecutionResultOuterClass.ChunkOrBuilder> chunksBuilder_;
/**
* repeated .flow.entities.Chunk chunks = 3;
*/
public java.util.List getChunksList() {
if (chunksBuilder_ == null) {
return java.util.Collections.unmodifiableList(chunks_);
} else {
return chunksBuilder_.getMessageList();
}
}
/**
* repeated .flow.entities.Chunk chunks = 3;
*/
public int getChunksCount() {
if (chunksBuilder_ == null) {
return chunks_.size();
} else {
return chunksBuilder_.getCount();
}
}
/**
* repeated .flow.entities.Chunk chunks = 3;
*/
public org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk getChunks(int index) {
if (chunksBuilder_ == null) {
return chunks_.get(index);
} else {
return chunksBuilder_.getMessage(index);
}
}
/**
* repeated .flow.entities.Chunk chunks = 3;
*/
public Builder setChunks(
int index, org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk value) {
if (chunksBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureChunksIsMutable();
chunks_.set(index, value);
onChanged();
} else {
chunksBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .flow.entities.Chunk chunks = 3;
*/
public Builder setChunks(
int index, org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk.Builder builderForValue) {
if (chunksBuilder_ == null) {
ensureChunksIsMutable();
chunks_.set(index, builderForValue.build());
onChanged();
} else {
chunksBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .flow.entities.Chunk chunks = 3;
*/
public Builder addChunks(org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk value) {
if (chunksBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureChunksIsMutable();
chunks_.add(value);
onChanged();
} else {
chunksBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .flow.entities.Chunk chunks = 3;
*/
public Builder addChunks(
int index, org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk value) {
if (chunksBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureChunksIsMutable();
chunks_.add(index, value);
onChanged();
} else {
chunksBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .flow.entities.Chunk chunks = 3;
*/
public Builder addChunks(
org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk.Builder builderForValue) {
if (chunksBuilder_ == null) {
ensureChunksIsMutable();
chunks_.add(builderForValue.build());
onChanged();
} else {
chunksBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .flow.entities.Chunk chunks = 3;
*/
public Builder addChunks(
int index, org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk.Builder builderForValue) {
if (chunksBuilder_ == null) {
ensureChunksIsMutable();
chunks_.add(index, builderForValue.build());
onChanged();
} else {
chunksBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .flow.entities.Chunk chunks = 3;
*/
public Builder addAllChunks(
java.lang.Iterable extends org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk> values) {
if (chunksBuilder_ == null) {
ensureChunksIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, chunks_);
onChanged();
} else {
chunksBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .flow.entities.Chunk chunks = 3;
*/
public Builder clearChunks() {
if (chunksBuilder_ == null) {
chunks_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
chunksBuilder_.clear();
}
return this;
}
/**
* repeated .flow.entities.Chunk chunks = 3;
*/
public Builder removeChunks(int index) {
if (chunksBuilder_ == null) {
ensureChunksIsMutable();
chunks_.remove(index);
onChanged();
} else {
chunksBuilder_.remove(index);
}
return this;
}
/**
* repeated .flow.entities.Chunk chunks = 3;
*/
public org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk.Builder getChunksBuilder(
int index) {
return getChunksFieldBuilder().getBuilder(index);
}
/**
* repeated .flow.entities.Chunk chunks = 3;
*/
public org.onflow.protobuf.entities.ExecutionResultOuterClass.ChunkOrBuilder getChunksOrBuilder(
int index) {
if (chunksBuilder_ == null) {
return chunks_.get(index); } else {
return chunksBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .flow.entities.Chunk chunks = 3;
*/
public java.util.List extends org.onflow.protobuf.entities.ExecutionResultOuterClass.ChunkOrBuilder>
getChunksOrBuilderList() {
if (chunksBuilder_ != null) {
return chunksBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(chunks_);
}
}
/**
* repeated .flow.entities.Chunk chunks = 3;
*/
public org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk.Builder addChunksBuilder() {
return getChunksFieldBuilder().addBuilder(
org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk.getDefaultInstance());
}
/**
* repeated .flow.entities.Chunk chunks = 3;
*/
public org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk.Builder addChunksBuilder(
int index) {
return getChunksFieldBuilder().addBuilder(
index, org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk.getDefaultInstance());
}
/**
* repeated .flow.entities.Chunk chunks = 3;
*/
public java.util.List
getChunksBuilderList() {
return getChunksFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk, org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk.Builder, org.onflow.protobuf.entities.ExecutionResultOuterClass.ChunkOrBuilder>
getChunksFieldBuilder() {
if (chunksBuilder_ == null) {
chunksBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk, org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk.Builder, org.onflow.protobuf.entities.ExecutionResultOuterClass.ChunkOrBuilder>(
chunks_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
chunks_ = null;
}
return chunksBuilder_;
}
private java.util.List serviceEvents_ =
java.util.Collections.emptyList();
private void ensureServiceEventsIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
serviceEvents_ = new java.util.ArrayList(serviceEvents_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent, org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent.Builder, org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEventOrBuilder> serviceEventsBuilder_;
/**
* repeated .flow.entities.ServiceEvent service_events = 4;
*/
public java.util.List getServiceEventsList() {
if (serviceEventsBuilder_ == null) {
return java.util.Collections.unmodifiableList(serviceEvents_);
} else {
return serviceEventsBuilder_.getMessageList();
}
}
/**
* repeated .flow.entities.ServiceEvent service_events = 4;
*/
public int getServiceEventsCount() {
if (serviceEventsBuilder_ == null) {
return serviceEvents_.size();
} else {
return serviceEventsBuilder_.getCount();
}
}
/**
* repeated .flow.entities.ServiceEvent service_events = 4;
*/
public org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent getServiceEvents(int index) {
if (serviceEventsBuilder_ == null) {
return serviceEvents_.get(index);
} else {
return serviceEventsBuilder_.getMessage(index);
}
}
/**
* repeated .flow.entities.ServiceEvent service_events = 4;
*/
public Builder setServiceEvents(
int index, org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent value) {
if (serviceEventsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureServiceEventsIsMutable();
serviceEvents_.set(index, value);
onChanged();
} else {
serviceEventsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .flow.entities.ServiceEvent service_events = 4;
*/
public Builder setServiceEvents(
int index, org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent.Builder builderForValue) {
if (serviceEventsBuilder_ == null) {
ensureServiceEventsIsMutable();
serviceEvents_.set(index, builderForValue.build());
onChanged();
} else {
serviceEventsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .flow.entities.ServiceEvent service_events = 4;
*/
public Builder addServiceEvents(org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent value) {
if (serviceEventsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureServiceEventsIsMutable();
serviceEvents_.add(value);
onChanged();
} else {
serviceEventsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .flow.entities.ServiceEvent service_events = 4;
*/
public Builder addServiceEvents(
int index, org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent value) {
if (serviceEventsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureServiceEventsIsMutable();
serviceEvents_.add(index, value);
onChanged();
} else {
serviceEventsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .flow.entities.ServiceEvent service_events = 4;
*/
public Builder addServiceEvents(
org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent.Builder builderForValue) {
if (serviceEventsBuilder_ == null) {
ensureServiceEventsIsMutable();
serviceEvents_.add(builderForValue.build());
onChanged();
} else {
serviceEventsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .flow.entities.ServiceEvent service_events = 4;
*/
public Builder addServiceEvents(
int index, org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent.Builder builderForValue) {
if (serviceEventsBuilder_ == null) {
ensureServiceEventsIsMutable();
serviceEvents_.add(index, builderForValue.build());
onChanged();
} else {
serviceEventsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .flow.entities.ServiceEvent service_events = 4;
*/
public Builder addAllServiceEvents(
java.lang.Iterable extends org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent> values) {
if (serviceEventsBuilder_ == null) {
ensureServiceEventsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, serviceEvents_);
onChanged();
} else {
serviceEventsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .flow.entities.ServiceEvent service_events = 4;
*/
public Builder clearServiceEvents() {
if (serviceEventsBuilder_ == null) {
serviceEvents_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
serviceEventsBuilder_.clear();
}
return this;
}
/**
* repeated .flow.entities.ServiceEvent service_events = 4;
*/
public Builder removeServiceEvents(int index) {
if (serviceEventsBuilder_ == null) {
ensureServiceEventsIsMutable();
serviceEvents_.remove(index);
onChanged();
} else {
serviceEventsBuilder_.remove(index);
}
return this;
}
/**
* repeated .flow.entities.ServiceEvent service_events = 4;
*/
public org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent.Builder getServiceEventsBuilder(
int index) {
return getServiceEventsFieldBuilder().getBuilder(index);
}
/**
* repeated .flow.entities.ServiceEvent service_events = 4;
*/
public org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEventOrBuilder getServiceEventsOrBuilder(
int index) {
if (serviceEventsBuilder_ == null) {
return serviceEvents_.get(index); } else {
return serviceEventsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .flow.entities.ServiceEvent service_events = 4;
*/
public java.util.List extends org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEventOrBuilder>
getServiceEventsOrBuilderList() {
if (serviceEventsBuilder_ != null) {
return serviceEventsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(serviceEvents_);
}
}
/**
* repeated .flow.entities.ServiceEvent service_events = 4;
*/
public org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent.Builder addServiceEventsBuilder() {
return getServiceEventsFieldBuilder().addBuilder(
org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent.getDefaultInstance());
}
/**
* repeated .flow.entities.ServiceEvent service_events = 4;
*/
public org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent.Builder addServiceEventsBuilder(
int index) {
return getServiceEventsFieldBuilder().addBuilder(
index, org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent.getDefaultInstance());
}
/**
* repeated .flow.entities.ServiceEvent service_events = 4;
*/
public java.util.List
getServiceEventsBuilderList() {
return getServiceEventsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent, org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent.Builder, org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEventOrBuilder>
getServiceEventsFieldBuilder() {
if (serviceEventsBuilder_ == null) {
serviceEventsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent, org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent.Builder, org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEventOrBuilder>(
serviceEvents_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
serviceEvents_ = null;
}
return serviceEventsBuilder_;
}
private com.google.protobuf.ByteString executionDataId_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes execution_data_id = 5 [deprecated = true];
* @return The executionDataId.
*/
@java.lang.Override
@java.lang.Deprecated public com.google.protobuf.ByteString getExecutionDataId() {
return executionDataId_;
}
/**
* bytes execution_data_id = 5 [deprecated = true];
* @param value The executionDataId to set.
* @return This builder for chaining.
*/
@java.lang.Deprecated public Builder setExecutionDataId(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
executionDataId_ = value;
onChanged();
return this;
}
/**
* bytes execution_data_id = 5 [deprecated = true];
* @return This builder for chaining.
*/
@java.lang.Deprecated public Builder clearExecutionDataId() {
executionDataId_ = getDefaultInstance().getExecutionDataId();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:flow.entities.ExecutionResult)
}
// @@protoc_insertion_point(class_scope:flow.entities.ExecutionResult)
private static final org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult();
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ExecutionResult parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ExecutionResult(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionResult getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ChunkOrBuilder extends
// @@protoc_insertion_point(interface_extends:flow.entities.Chunk)
com.google.protobuf.MessageOrBuilder {
/**
* uint32 CollectionIndex = 1;
* @return The collectionIndex.
*/
int getCollectionIndex();
/**
*
* state when starting executing this chunk
*
*
* bytes start_state = 2;
* @return The startState.
*/
com.google.protobuf.ByteString getStartState();
/**
*
* Events generated by executing results
*
*
* bytes event_collection = 3;
* @return The eventCollection.
*/
com.google.protobuf.ByteString getEventCollection();
/**
*
* Block id of the execution result this chunk belongs to
*
*
* bytes block_id = 4;
* @return The blockId.
*/
com.google.protobuf.ByteString getBlockId();
/**
*
* total amount of computation used by running all txs in this chunk
*
*
* uint64 total_computation_used = 5;
* @return The totalComputationUsed.
*/
long getTotalComputationUsed();
/**
*
* number of transactions inside the collection
*
*
* uint32 number_of_transactions = 6;
* @return The numberOfTransactions.
*/
int getNumberOfTransactions();
/**
*
* chunk index inside the ER (starts from zero)
*
*
* uint64 index = 7;
* @return The index.
*/
long getIndex();
/**
*
* EndState inferred from next chunk or from the ER
*
*
* bytes end_state = 8;
* @return The endState.
*/
com.google.protobuf.ByteString getEndState();
/**
* bytes execution_data_id = 9;
* @return The executionDataId.
*/
com.google.protobuf.ByteString getExecutionDataId();
/**
*
* a commitment over sorted list of register changes
*
*
* bytes state_delta_commitment = 10;
* @return The stateDeltaCommitment.
*/
com.google.protobuf.ByteString getStateDeltaCommitment();
}
/**
* Protobuf type {@code flow.entities.Chunk}
*/
public static final class Chunk extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:flow.entities.Chunk)
ChunkOrBuilder {
private static final long serialVersionUID = 0L;
// Use Chunk.newBuilder() to construct.
private Chunk(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Chunk() {
startState_ = com.google.protobuf.ByteString.EMPTY;
eventCollection_ = com.google.protobuf.ByteString.EMPTY;
blockId_ = com.google.protobuf.ByteString.EMPTY;
endState_ = com.google.protobuf.ByteString.EMPTY;
executionDataId_ = com.google.protobuf.ByteString.EMPTY;
stateDeltaCommitment_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Chunk();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Chunk(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
collectionIndex_ = input.readUInt32();
break;
}
case 18: {
startState_ = input.readBytes();
break;
}
case 26: {
eventCollection_ = input.readBytes();
break;
}
case 34: {
blockId_ = input.readBytes();
break;
}
case 40: {
totalComputationUsed_ = input.readUInt64();
break;
}
case 48: {
numberOfTransactions_ = input.readUInt32();
break;
}
case 56: {
index_ = input.readUInt64();
break;
}
case 66: {
endState_ = input.readBytes();
break;
}
case 74: {
executionDataId_ = input.readBytes();
break;
}
case 82: {
stateDeltaCommitment_ = input.readBytes();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.onflow.protobuf.entities.ExecutionResultOuterClass.internal_static_flow_entities_Chunk_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.onflow.protobuf.entities.ExecutionResultOuterClass.internal_static_flow_entities_Chunk_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk.class, org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk.Builder.class);
}
public static final int COLLECTIONINDEX_FIELD_NUMBER = 1;
private int collectionIndex_;
/**
* uint32 CollectionIndex = 1;
* @return The collectionIndex.
*/
@java.lang.Override
public int getCollectionIndex() {
return collectionIndex_;
}
public static final int START_STATE_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString startState_;
/**
*
* state when starting executing this chunk
*
*
* bytes start_state = 2;
* @return The startState.
*/
@java.lang.Override
public com.google.protobuf.ByteString getStartState() {
return startState_;
}
public static final int EVENT_COLLECTION_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString eventCollection_;
/**
*
* Events generated by executing results
*
*
* bytes event_collection = 3;
* @return The eventCollection.
*/
@java.lang.Override
public com.google.protobuf.ByteString getEventCollection() {
return eventCollection_;
}
public static final int BLOCK_ID_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString blockId_;
/**
*
* Block id of the execution result this chunk belongs to
*
*
* bytes block_id = 4;
* @return The blockId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBlockId() {
return blockId_;
}
public static final int TOTAL_COMPUTATION_USED_FIELD_NUMBER = 5;
private long totalComputationUsed_;
/**
*
* total amount of computation used by running all txs in this chunk
*
*
* uint64 total_computation_used = 5;
* @return The totalComputationUsed.
*/
@java.lang.Override
public long getTotalComputationUsed() {
return totalComputationUsed_;
}
public static final int NUMBER_OF_TRANSACTIONS_FIELD_NUMBER = 6;
private int numberOfTransactions_;
/**
*
* number of transactions inside the collection
*
*
* uint32 number_of_transactions = 6;
* @return The numberOfTransactions.
*/
@java.lang.Override
public int getNumberOfTransactions() {
return numberOfTransactions_;
}
public static final int INDEX_FIELD_NUMBER = 7;
private long index_;
/**
*
* chunk index inside the ER (starts from zero)
*
*
* uint64 index = 7;
* @return The index.
*/
@java.lang.Override
public long getIndex() {
return index_;
}
public static final int END_STATE_FIELD_NUMBER = 8;
private com.google.protobuf.ByteString endState_;
/**
*
* EndState inferred from next chunk or from the ER
*
*
* bytes end_state = 8;
* @return The endState.
*/
@java.lang.Override
public com.google.protobuf.ByteString getEndState() {
return endState_;
}
public static final int EXECUTION_DATA_ID_FIELD_NUMBER = 9;
private com.google.protobuf.ByteString executionDataId_;
/**
* bytes execution_data_id = 9;
* @return The executionDataId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getExecutionDataId() {
return executionDataId_;
}
public static final int STATE_DELTA_COMMITMENT_FIELD_NUMBER = 10;
private com.google.protobuf.ByteString stateDeltaCommitment_;
/**
*
* a commitment over sorted list of register changes
*
*
* bytes state_delta_commitment = 10;
* @return The stateDeltaCommitment.
*/
@java.lang.Override
public com.google.protobuf.ByteString getStateDeltaCommitment() {
return stateDeltaCommitment_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (collectionIndex_ != 0) {
output.writeUInt32(1, collectionIndex_);
}
if (!startState_.isEmpty()) {
output.writeBytes(2, startState_);
}
if (!eventCollection_.isEmpty()) {
output.writeBytes(3, eventCollection_);
}
if (!blockId_.isEmpty()) {
output.writeBytes(4, blockId_);
}
if (totalComputationUsed_ != 0L) {
output.writeUInt64(5, totalComputationUsed_);
}
if (numberOfTransactions_ != 0) {
output.writeUInt32(6, numberOfTransactions_);
}
if (index_ != 0L) {
output.writeUInt64(7, index_);
}
if (!endState_.isEmpty()) {
output.writeBytes(8, endState_);
}
if (!executionDataId_.isEmpty()) {
output.writeBytes(9, executionDataId_);
}
if (!stateDeltaCommitment_.isEmpty()) {
output.writeBytes(10, stateDeltaCommitment_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (collectionIndex_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, collectionIndex_);
}
if (!startState_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, startState_);
}
if (!eventCollection_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, eventCollection_);
}
if (!blockId_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, blockId_);
}
if (totalComputationUsed_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(5, totalComputationUsed_);
}
if (numberOfTransactions_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(6, numberOfTransactions_);
}
if (index_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(7, index_);
}
if (!endState_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(8, endState_);
}
if (!executionDataId_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(9, executionDataId_);
}
if (!stateDeltaCommitment_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(10, stateDeltaCommitment_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk)) {
return super.equals(obj);
}
org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk other = (org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk) obj;
if (getCollectionIndex()
!= other.getCollectionIndex()) return false;
if (!getStartState()
.equals(other.getStartState())) return false;
if (!getEventCollection()
.equals(other.getEventCollection())) return false;
if (!getBlockId()
.equals(other.getBlockId())) return false;
if (getTotalComputationUsed()
!= other.getTotalComputationUsed()) return false;
if (getNumberOfTransactions()
!= other.getNumberOfTransactions()) return false;
if (getIndex()
!= other.getIndex()) return false;
if (!getEndState()
.equals(other.getEndState())) return false;
if (!getExecutionDataId()
.equals(other.getExecutionDataId())) return false;
if (!getStateDeltaCommitment()
.equals(other.getStateDeltaCommitment())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + COLLECTIONINDEX_FIELD_NUMBER;
hash = (53 * hash) + getCollectionIndex();
hash = (37 * hash) + START_STATE_FIELD_NUMBER;
hash = (53 * hash) + getStartState().hashCode();
hash = (37 * hash) + EVENT_COLLECTION_FIELD_NUMBER;
hash = (53 * hash) + getEventCollection().hashCode();
hash = (37 * hash) + BLOCK_ID_FIELD_NUMBER;
hash = (53 * hash) + getBlockId().hashCode();
hash = (37 * hash) + TOTAL_COMPUTATION_USED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTotalComputationUsed());
hash = (37 * hash) + NUMBER_OF_TRANSACTIONS_FIELD_NUMBER;
hash = (53 * hash) + getNumberOfTransactions();
hash = (37 * hash) + INDEX_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getIndex());
hash = (37 * hash) + END_STATE_FIELD_NUMBER;
hash = (53 * hash) + getEndState().hashCode();
hash = (37 * hash) + EXECUTION_DATA_ID_FIELD_NUMBER;
hash = (53 * hash) + getExecutionDataId().hashCode();
hash = (37 * hash) + STATE_DELTA_COMMITMENT_FIELD_NUMBER;
hash = (53 * hash) + getStateDeltaCommitment().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code flow.entities.Chunk}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:flow.entities.Chunk)
org.onflow.protobuf.entities.ExecutionResultOuterClass.ChunkOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.onflow.protobuf.entities.ExecutionResultOuterClass.internal_static_flow_entities_Chunk_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.onflow.protobuf.entities.ExecutionResultOuterClass.internal_static_flow_entities_Chunk_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk.class, org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk.Builder.class);
}
// Construct using org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
collectionIndex_ = 0;
startState_ = com.google.protobuf.ByteString.EMPTY;
eventCollection_ = com.google.protobuf.ByteString.EMPTY;
blockId_ = com.google.protobuf.ByteString.EMPTY;
totalComputationUsed_ = 0L;
numberOfTransactions_ = 0;
index_ = 0L;
endState_ = com.google.protobuf.ByteString.EMPTY;
executionDataId_ = com.google.protobuf.ByteString.EMPTY;
stateDeltaCommitment_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.onflow.protobuf.entities.ExecutionResultOuterClass.internal_static_flow_entities_Chunk_descriptor;
}
@java.lang.Override
public org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk getDefaultInstanceForType() {
return org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk.getDefaultInstance();
}
@java.lang.Override
public org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk build() {
org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk buildPartial() {
org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk result = new org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk(this);
result.collectionIndex_ = collectionIndex_;
result.startState_ = startState_;
result.eventCollection_ = eventCollection_;
result.blockId_ = blockId_;
result.totalComputationUsed_ = totalComputationUsed_;
result.numberOfTransactions_ = numberOfTransactions_;
result.index_ = index_;
result.endState_ = endState_;
result.executionDataId_ = executionDataId_;
result.stateDeltaCommitment_ = stateDeltaCommitment_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk) {
return mergeFrom((org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk other) {
if (other == org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk.getDefaultInstance()) return this;
if (other.getCollectionIndex() != 0) {
setCollectionIndex(other.getCollectionIndex());
}
if (other.getStartState() != com.google.protobuf.ByteString.EMPTY) {
setStartState(other.getStartState());
}
if (other.getEventCollection() != com.google.protobuf.ByteString.EMPTY) {
setEventCollection(other.getEventCollection());
}
if (other.getBlockId() != com.google.protobuf.ByteString.EMPTY) {
setBlockId(other.getBlockId());
}
if (other.getTotalComputationUsed() != 0L) {
setTotalComputationUsed(other.getTotalComputationUsed());
}
if (other.getNumberOfTransactions() != 0) {
setNumberOfTransactions(other.getNumberOfTransactions());
}
if (other.getIndex() != 0L) {
setIndex(other.getIndex());
}
if (other.getEndState() != com.google.protobuf.ByteString.EMPTY) {
setEndState(other.getEndState());
}
if (other.getExecutionDataId() != com.google.protobuf.ByteString.EMPTY) {
setExecutionDataId(other.getExecutionDataId());
}
if (other.getStateDeltaCommitment() != com.google.protobuf.ByteString.EMPTY) {
setStateDeltaCommitment(other.getStateDeltaCommitment());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int collectionIndex_ ;
/**
* uint32 CollectionIndex = 1;
* @return The collectionIndex.
*/
@java.lang.Override
public int getCollectionIndex() {
return collectionIndex_;
}
/**
* uint32 CollectionIndex = 1;
* @param value The collectionIndex to set.
* @return This builder for chaining.
*/
public Builder setCollectionIndex(int value) {
collectionIndex_ = value;
onChanged();
return this;
}
/**
* uint32 CollectionIndex = 1;
* @return This builder for chaining.
*/
public Builder clearCollectionIndex() {
collectionIndex_ = 0;
onChanged();
return this;
}
private com.google.protobuf.ByteString startState_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* state when starting executing this chunk
*
*
* bytes start_state = 2;
* @return The startState.
*/
@java.lang.Override
public com.google.protobuf.ByteString getStartState() {
return startState_;
}
/**
*
* state when starting executing this chunk
*
*
* bytes start_state = 2;
* @param value The startState to set.
* @return This builder for chaining.
*/
public Builder setStartState(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
startState_ = value;
onChanged();
return this;
}
/**
*
* state when starting executing this chunk
*
*
* bytes start_state = 2;
* @return This builder for chaining.
*/
public Builder clearStartState() {
startState_ = getDefaultInstance().getStartState();
onChanged();
return this;
}
private com.google.protobuf.ByteString eventCollection_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Events generated by executing results
*
*
* bytes event_collection = 3;
* @return The eventCollection.
*/
@java.lang.Override
public com.google.protobuf.ByteString getEventCollection() {
return eventCollection_;
}
/**
*
* Events generated by executing results
*
*
* bytes event_collection = 3;
* @param value The eventCollection to set.
* @return This builder for chaining.
*/
public Builder setEventCollection(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
eventCollection_ = value;
onChanged();
return this;
}
/**
*
* Events generated by executing results
*
*
* bytes event_collection = 3;
* @return This builder for chaining.
*/
public Builder clearEventCollection() {
eventCollection_ = getDefaultInstance().getEventCollection();
onChanged();
return this;
}
private com.google.protobuf.ByteString blockId_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Block id of the execution result this chunk belongs to
*
*
* bytes block_id = 4;
* @return The blockId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBlockId() {
return blockId_;
}
/**
*
* Block id of the execution result this chunk belongs to
*
*
* bytes block_id = 4;
* @param value The blockId to set.
* @return This builder for chaining.
*/
public Builder setBlockId(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
blockId_ = value;
onChanged();
return this;
}
/**
*
* Block id of the execution result this chunk belongs to
*
*
* bytes block_id = 4;
* @return This builder for chaining.
*/
public Builder clearBlockId() {
blockId_ = getDefaultInstance().getBlockId();
onChanged();
return this;
}
private long totalComputationUsed_ ;
/**
*
* total amount of computation used by running all txs in this chunk
*
*
* uint64 total_computation_used = 5;
* @return The totalComputationUsed.
*/
@java.lang.Override
public long getTotalComputationUsed() {
return totalComputationUsed_;
}
/**
*
* total amount of computation used by running all txs in this chunk
*
*
* uint64 total_computation_used = 5;
* @param value The totalComputationUsed to set.
* @return This builder for chaining.
*/
public Builder setTotalComputationUsed(long value) {
totalComputationUsed_ = value;
onChanged();
return this;
}
/**
*
* total amount of computation used by running all txs in this chunk
*
*
* uint64 total_computation_used = 5;
* @return This builder for chaining.
*/
public Builder clearTotalComputationUsed() {
totalComputationUsed_ = 0L;
onChanged();
return this;
}
private int numberOfTransactions_ ;
/**
*
* number of transactions inside the collection
*
*
* uint32 number_of_transactions = 6;
* @return The numberOfTransactions.
*/
@java.lang.Override
public int getNumberOfTransactions() {
return numberOfTransactions_;
}
/**
*
* number of transactions inside the collection
*
*
* uint32 number_of_transactions = 6;
* @param value The numberOfTransactions to set.
* @return This builder for chaining.
*/
public Builder setNumberOfTransactions(int value) {
numberOfTransactions_ = value;
onChanged();
return this;
}
/**
*
* number of transactions inside the collection
*
*
* uint32 number_of_transactions = 6;
* @return This builder for chaining.
*/
public Builder clearNumberOfTransactions() {
numberOfTransactions_ = 0;
onChanged();
return this;
}
private long index_ ;
/**
*
* chunk index inside the ER (starts from zero)
*
*
* uint64 index = 7;
* @return The index.
*/
@java.lang.Override
public long getIndex() {
return index_;
}
/**
*
* chunk index inside the ER (starts from zero)
*
*
* uint64 index = 7;
* @param value The index to set.
* @return This builder for chaining.
*/
public Builder setIndex(long value) {
index_ = value;
onChanged();
return this;
}
/**
*
* chunk index inside the ER (starts from zero)
*
*
* uint64 index = 7;
* @return This builder for chaining.
*/
public Builder clearIndex() {
index_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.ByteString endState_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* EndState inferred from next chunk or from the ER
*
*
* bytes end_state = 8;
* @return The endState.
*/
@java.lang.Override
public com.google.protobuf.ByteString getEndState() {
return endState_;
}
/**
*
* EndState inferred from next chunk or from the ER
*
*
* bytes end_state = 8;
* @param value The endState to set.
* @return This builder for chaining.
*/
public Builder setEndState(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
endState_ = value;
onChanged();
return this;
}
/**
*
* EndState inferred from next chunk or from the ER
*
*
* bytes end_state = 8;
* @return This builder for chaining.
*/
public Builder clearEndState() {
endState_ = getDefaultInstance().getEndState();
onChanged();
return this;
}
private com.google.protobuf.ByteString executionDataId_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes execution_data_id = 9;
* @return The executionDataId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getExecutionDataId() {
return executionDataId_;
}
/**
* bytes execution_data_id = 9;
* @param value The executionDataId to set.
* @return This builder for chaining.
*/
public Builder setExecutionDataId(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
executionDataId_ = value;
onChanged();
return this;
}
/**
* bytes execution_data_id = 9;
* @return This builder for chaining.
*/
public Builder clearExecutionDataId() {
executionDataId_ = getDefaultInstance().getExecutionDataId();
onChanged();
return this;
}
private com.google.protobuf.ByteString stateDeltaCommitment_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* a commitment over sorted list of register changes
*
*
* bytes state_delta_commitment = 10;
* @return The stateDeltaCommitment.
*/
@java.lang.Override
public com.google.protobuf.ByteString getStateDeltaCommitment() {
return stateDeltaCommitment_;
}
/**
*
* a commitment over sorted list of register changes
*
*
* bytes state_delta_commitment = 10;
* @param value The stateDeltaCommitment to set.
* @return This builder for chaining.
*/
public Builder setStateDeltaCommitment(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
stateDeltaCommitment_ = value;
onChanged();
return this;
}
/**
*
* a commitment over sorted list of register changes
*
*
* bytes state_delta_commitment = 10;
* @return This builder for chaining.
*/
public Builder clearStateDeltaCommitment() {
stateDeltaCommitment_ = getDefaultInstance().getStateDeltaCommitment();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:flow.entities.Chunk)
}
// @@protoc_insertion_point(class_scope:flow.entities.Chunk)
private static final org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk();
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Chunk parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Chunk(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.onflow.protobuf.entities.ExecutionResultOuterClass.Chunk getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ServiceEventOrBuilder extends
// @@protoc_insertion_point(interface_extends:flow.entities.ServiceEvent)
com.google.protobuf.MessageOrBuilder {
/**
* string type = 1;
* @return The type.
*/
java.lang.String getType();
/**
* string type = 1;
* @return The bytes for type.
*/
com.google.protobuf.ByteString
getTypeBytes();
/**
* bytes payload = 2;
* @return The payload.
*/
com.google.protobuf.ByteString getPayload();
}
/**
* Protobuf type {@code flow.entities.ServiceEvent}
*/
public static final class ServiceEvent extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:flow.entities.ServiceEvent)
ServiceEventOrBuilder {
private static final long serialVersionUID = 0L;
// Use ServiceEvent.newBuilder() to construct.
private ServiceEvent(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ServiceEvent() {
type_ = "";
payload_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ServiceEvent();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ServiceEvent(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
type_ = s;
break;
}
case 18: {
payload_ = input.readBytes();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.onflow.protobuf.entities.ExecutionResultOuterClass.internal_static_flow_entities_ServiceEvent_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.onflow.protobuf.entities.ExecutionResultOuterClass.internal_static_flow_entities_ServiceEvent_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent.class, org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent.Builder.class);
}
public static final int TYPE_FIELD_NUMBER = 1;
private volatile java.lang.Object type_;
/**
* string type = 1;
* @return The type.
*/
@java.lang.Override
public java.lang.String getType() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
type_ = s;
return s;
}
}
/**
* string type = 1;
* @return The bytes for type.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PAYLOAD_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString payload_;
/**
* bytes payload = 2;
* @return The payload.
*/
@java.lang.Override
public com.google.protobuf.ByteString getPayload() {
return payload_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getTypeBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, type_);
}
if (!payload_.isEmpty()) {
output.writeBytes(2, payload_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getTypeBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, type_);
}
if (!payload_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, payload_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent)) {
return super.equals(obj);
}
org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent other = (org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent) obj;
if (!getType()
.equals(other.getType())) return false;
if (!getPayload()
.equals(other.getPayload())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + getType().hashCode();
hash = (37 * hash) + PAYLOAD_FIELD_NUMBER;
hash = (53 * hash) + getPayload().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code flow.entities.ServiceEvent}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:flow.entities.ServiceEvent)
org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEventOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.onflow.protobuf.entities.ExecutionResultOuterClass.internal_static_flow_entities_ServiceEvent_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.onflow.protobuf.entities.ExecutionResultOuterClass.internal_static_flow_entities_ServiceEvent_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent.class, org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent.Builder.class);
}
// Construct using org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
type_ = "";
payload_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.onflow.protobuf.entities.ExecutionResultOuterClass.internal_static_flow_entities_ServiceEvent_descriptor;
}
@java.lang.Override
public org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent getDefaultInstanceForType() {
return org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent.getDefaultInstance();
}
@java.lang.Override
public org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent build() {
org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent buildPartial() {
org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent result = new org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent(this);
result.type_ = type_;
result.payload_ = payload_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent) {
return mergeFrom((org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent other) {
if (other == org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent.getDefaultInstance()) return this;
if (!other.getType().isEmpty()) {
type_ = other.type_;
onChanged();
}
if (other.getPayload() != com.google.protobuf.ByteString.EMPTY) {
setPayload(other.getPayload());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object type_ = "";
/**
* string type = 1;
* @return The type.
*/
public java.lang.String getType() {
java.lang.Object ref = type_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
type_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string type = 1;
* @return The bytes for type.
*/
public com.google.protobuf.ByteString
getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string type = 1;
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value;
onChanged();
return this;
}
/**
* string type = 1;
* @return This builder for chaining.
*/
public Builder clearType() {
type_ = getDefaultInstance().getType();
onChanged();
return this;
}
/**
* string type = 1;
* @param value The bytes for type to set.
* @return This builder for chaining.
*/
public Builder setTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
type_ = value;
onChanged();
return this;
}
private com.google.protobuf.ByteString payload_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes payload = 2;
* @return The payload.
*/
@java.lang.Override
public com.google.protobuf.ByteString getPayload() {
return payload_;
}
/**
* bytes payload = 2;
* @param value The payload to set.
* @return This builder for chaining.
*/
public Builder setPayload(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
payload_ = value;
onChanged();
return this;
}
/**
* bytes payload = 2;
* @return This builder for chaining.
*/
public Builder clearPayload() {
payload_ = getDefaultInstance().getPayload();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:flow.entities.ServiceEvent)
}
// @@protoc_insertion_point(class_scope:flow.entities.ServiceEvent)
private static final org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent();
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ServiceEvent parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ServiceEvent(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.onflow.protobuf.entities.ExecutionResultOuterClass.ServiceEvent getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ExecutionReceiptMetaOrBuilder extends
// @@protoc_insertion_point(interface_extends:flow.entities.ExecutionReceiptMeta)
com.google.protobuf.MessageOrBuilder {
/**
* bytes executor_id = 1;
* @return The executorId.
*/
com.google.protobuf.ByteString getExecutorId();
/**
* bytes result_id = 2;
* @return The resultId.
*/
com.google.protobuf.ByteString getResultId();
/**
* repeated bytes spocks = 3;
* @return A list containing the spocks.
*/
java.util.List getSpocksList();
/**
* repeated bytes spocks = 3;
* @return The count of spocks.
*/
int getSpocksCount();
/**
* repeated bytes spocks = 3;
* @param index The index of the element to return.
* @return The spocks at the given index.
*/
com.google.protobuf.ByteString getSpocks(int index);
/**
* bytes executor_signature = 4;
* @return The executorSignature.
*/
com.google.protobuf.ByteString getExecutorSignature();
}
/**
* Protobuf type {@code flow.entities.ExecutionReceiptMeta}
*/
public static final class ExecutionReceiptMeta extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:flow.entities.ExecutionReceiptMeta)
ExecutionReceiptMetaOrBuilder {
private static final long serialVersionUID = 0L;
// Use ExecutionReceiptMeta.newBuilder() to construct.
private ExecutionReceiptMeta(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ExecutionReceiptMeta() {
executorId_ = com.google.protobuf.ByteString.EMPTY;
resultId_ = com.google.protobuf.ByteString.EMPTY;
spocks_ = java.util.Collections.emptyList();
executorSignature_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ExecutionReceiptMeta();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ExecutionReceiptMeta(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
executorId_ = input.readBytes();
break;
}
case 18: {
resultId_ = input.readBytes();
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
spocks_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
spocks_.add(input.readBytes());
break;
}
case 34: {
executorSignature_ = input.readBytes();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
spocks_ = java.util.Collections.unmodifiableList(spocks_); // C
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.onflow.protobuf.entities.ExecutionResultOuterClass.internal_static_flow_entities_ExecutionReceiptMeta_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.onflow.protobuf.entities.ExecutionResultOuterClass.internal_static_flow_entities_ExecutionReceiptMeta_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta.class, org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta.Builder.class);
}
public static final int EXECUTOR_ID_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString executorId_;
/**
* bytes executor_id = 1;
* @return The executorId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getExecutorId() {
return executorId_;
}
public static final int RESULT_ID_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString resultId_;
/**
* bytes result_id = 2;
* @return The resultId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getResultId() {
return resultId_;
}
public static final int SPOCKS_FIELD_NUMBER = 3;
private java.util.List spocks_;
/**
* repeated bytes spocks = 3;
* @return A list containing the spocks.
*/
@java.lang.Override
public java.util.List
getSpocksList() {
return spocks_;
}
/**
* repeated bytes spocks = 3;
* @return The count of spocks.
*/
public int getSpocksCount() {
return spocks_.size();
}
/**
* repeated bytes spocks = 3;
* @param index The index of the element to return.
* @return The spocks at the given index.
*/
public com.google.protobuf.ByteString getSpocks(int index) {
return spocks_.get(index);
}
public static final int EXECUTOR_SIGNATURE_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString executorSignature_;
/**
* bytes executor_signature = 4;
* @return The executorSignature.
*/
@java.lang.Override
public com.google.protobuf.ByteString getExecutorSignature() {
return executorSignature_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!executorId_.isEmpty()) {
output.writeBytes(1, executorId_);
}
if (!resultId_.isEmpty()) {
output.writeBytes(2, resultId_);
}
for (int i = 0; i < spocks_.size(); i++) {
output.writeBytes(3, spocks_.get(i));
}
if (!executorSignature_.isEmpty()) {
output.writeBytes(4, executorSignature_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!executorId_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, executorId_);
}
if (!resultId_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, resultId_);
}
{
int dataSize = 0;
for (int i = 0; i < spocks_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(spocks_.get(i));
}
size += dataSize;
size += 1 * getSpocksList().size();
}
if (!executorSignature_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, executorSignature_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta)) {
return super.equals(obj);
}
org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta other = (org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta) obj;
if (!getExecutorId()
.equals(other.getExecutorId())) return false;
if (!getResultId()
.equals(other.getResultId())) return false;
if (!getSpocksList()
.equals(other.getSpocksList())) return false;
if (!getExecutorSignature()
.equals(other.getExecutorSignature())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + EXECUTOR_ID_FIELD_NUMBER;
hash = (53 * hash) + getExecutorId().hashCode();
hash = (37 * hash) + RESULT_ID_FIELD_NUMBER;
hash = (53 * hash) + getResultId().hashCode();
if (getSpocksCount() > 0) {
hash = (37 * hash) + SPOCKS_FIELD_NUMBER;
hash = (53 * hash) + getSpocksList().hashCode();
}
hash = (37 * hash) + EXECUTOR_SIGNATURE_FIELD_NUMBER;
hash = (53 * hash) + getExecutorSignature().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code flow.entities.ExecutionReceiptMeta}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:flow.entities.ExecutionReceiptMeta)
org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMetaOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.onflow.protobuf.entities.ExecutionResultOuterClass.internal_static_flow_entities_ExecutionReceiptMeta_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.onflow.protobuf.entities.ExecutionResultOuterClass.internal_static_flow_entities_ExecutionReceiptMeta_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta.class, org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta.Builder.class);
}
// Construct using org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
executorId_ = com.google.protobuf.ByteString.EMPTY;
resultId_ = com.google.protobuf.ByteString.EMPTY;
spocks_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
executorSignature_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.onflow.protobuf.entities.ExecutionResultOuterClass.internal_static_flow_entities_ExecutionReceiptMeta_descriptor;
}
@java.lang.Override
public org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta getDefaultInstanceForType() {
return org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta.getDefaultInstance();
}
@java.lang.Override
public org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta build() {
org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta buildPartial() {
org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta result = new org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta(this);
int from_bitField0_ = bitField0_;
result.executorId_ = executorId_;
result.resultId_ = resultId_;
if (((bitField0_ & 0x00000001) != 0)) {
spocks_ = java.util.Collections.unmodifiableList(spocks_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.spocks_ = spocks_;
result.executorSignature_ = executorSignature_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta) {
return mergeFrom((org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta other) {
if (other == org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta.getDefaultInstance()) return this;
if (other.getExecutorId() != com.google.protobuf.ByteString.EMPTY) {
setExecutorId(other.getExecutorId());
}
if (other.getResultId() != com.google.protobuf.ByteString.EMPTY) {
setResultId(other.getResultId());
}
if (!other.spocks_.isEmpty()) {
if (spocks_.isEmpty()) {
spocks_ = other.spocks_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureSpocksIsMutable();
spocks_.addAll(other.spocks_);
}
onChanged();
}
if (other.getExecutorSignature() != com.google.protobuf.ByteString.EMPTY) {
setExecutorSignature(other.getExecutorSignature());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString executorId_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes executor_id = 1;
* @return The executorId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getExecutorId() {
return executorId_;
}
/**
* bytes executor_id = 1;
* @param value The executorId to set.
* @return This builder for chaining.
*/
public Builder setExecutorId(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
executorId_ = value;
onChanged();
return this;
}
/**
* bytes executor_id = 1;
* @return This builder for chaining.
*/
public Builder clearExecutorId() {
executorId_ = getDefaultInstance().getExecutorId();
onChanged();
return this;
}
private com.google.protobuf.ByteString resultId_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes result_id = 2;
* @return The resultId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getResultId() {
return resultId_;
}
/**
* bytes result_id = 2;
* @param value The resultId to set.
* @return This builder for chaining.
*/
public Builder setResultId(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
resultId_ = value;
onChanged();
return this;
}
/**
* bytes result_id = 2;
* @return This builder for chaining.
*/
public Builder clearResultId() {
resultId_ = getDefaultInstance().getResultId();
onChanged();
return this;
}
private java.util.List spocks_ = java.util.Collections.emptyList();
private void ensureSpocksIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
spocks_ = new java.util.ArrayList(spocks_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated bytes spocks = 3;
* @return A list containing the spocks.
*/
public java.util.List
getSpocksList() {
return ((bitField0_ & 0x00000001) != 0) ?
java.util.Collections.unmodifiableList(spocks_) : spocks_;
}
/**
* repeated bytes spocks = 3;
* @return The count of spocks.
*/
public int getSpocksCount() {
return spocks_.size();
}
/**
* repeated bytes spocks = 3;
* @param index The index of the element to return.
* @return The spocks at the given index.
*/
public com.google.protobuf.ByteString getSpocks(int index) {
return spocks_.get(index);
}
/**
* repeated bytes spocks = 3;
* @param index The index to set the value at.
* @param value The spocks to set.
* @return This builder for chaining.
*/
public Builder setSpocks(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureSpocksIsMutable();
spocks_.set(index, value);
onChanged();
return this;
}
/**
* repeated bytes spocks = 3;
* @param value The spocks to add.
* @return This builder for chaining.
*/
public Builder addSpocks(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureSpocksIsMutable();
spocks_.add(value);
onChanged();
return this;
}
/**
* repeated bytes spocks = 3;
* @param values The spocks to add.
* @return This builder for chaining.
*/
public Builder addAllSpocks(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureSpocksIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, spocks_);
onChanged();
return this;
}
/**
* repeated bytes spocks = 3;
* @return This builder for chaining.
*/
public Builder clearSpocks() {
spocks_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
private com.google.protobuf.ByteString executorSignature_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes executor_signature = 4;
* @return The executorSignature.
*/
@java.lang.Override
public com.google.protobuf.ByteString getExecutorSignature() {
return executorSignature_;
}
/**
* bytes executor_signature = 4;
* @param value The executorSignature to set.
* @return This builder for chaining.
*/
public Builder setExecutorSignature(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
executorSignature_ = value;
onChanged();
return this;
}
/**
* bytes executor_signature = 4;
* @return This builder for chaining.
*/
public Builder clearExecutorSignature() {
executorSignature_ = getDefaultInstance().getExecutorSignature();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:flow.entities.ExecutionReceiptMeta)
}
// @@protoc_insertion_point(class_scope:flow.entities.ExecutionReceiptMeta)
private static final org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta();
}
public static org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ExecutionReceiptMeta parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ExecutionReceiptMeta(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.onflow.protobuf.entities.ExecutionResultOuterClass.ExecutionReceiptMeta getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_flow_entities_ExecutionResult_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_flow_entities_ExecutionResult_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_flow_entities_Chunk_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_flow_entities_Chunk_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_flow_entities_ServiceEvent_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_flow_entities_ServiceEvent_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_flow_entities_ExecutionReceiptMeta_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_flow_entities_ExecutionReceiptMeta_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n$flow/entities/execution_result.proto\022\r" +
"flow.entities\"\271\001\n\017ExecutionResult\022\032\n\022pre" +
"vious_result_id\030\001 \001(\014\022\020\n\010block_id\030\002 \001(\014\022" +
"$\n\006chunks\030\003 \003(\0132\024.flow.entities.Chunk\0223\n" +
"\016service_events\030\004 \003(\0132\033.flow.entities.Se" +
"rviceEvent\022\035\n\021execution_data_id\030\005 \001(\014B\002\030" +
"\001\"\376\001\n\005Chunk\022\027\n\017CollectionIndex\030\001 \001(\r\022\023\n\013" +
"start_state\030\002 \001(\014\022\030\n\020event_collection\030\003 " +
"\001(\014\022\020\n\010block_id\030\004 \001(\014\022\036\n\026total_computati" +
"on_used\030\005 \001(\004\022\036\n\026number_of_transactions\030" +
"\006 \001(\r\022\r\n\005index\030\007 \001(\004\022\021\n\tend_state\030\010 \001(\014\022" +
"\031\n\021execution_data_id\030\t \001(\014\022\036\n\026state_delt" +
"a_commitment\030\n \001(\014\"-\n\014ServiceEvent\022\014\n\004ty" +
"pe\030\001 \001(\t\022\017\n\007payload\030\002 \001(\014\"j\n\024ExecutionRe" +
"ceiptMeta\022\023\n\013executor_id\030\001 \001(\014\022\021\n\tresult" +
"_id\030\002 \001(\014\022\016\n\006spocks\030\003 \003(\014\022\032\n\022executor_si" +
"gnature\030\004 \001(\014BP\n\034org.onflow.protobuf.ent" +
"itiesZ0github.com/onflow/flow/protobuf/g" +
"o/flow/entitiesb\006proto3"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
});
internal_static_flow_entities_ExecutionResult_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_flow_entities_ExecutionResult_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_flow_entities_ExecutionResult_descriptor,
new java.lang.String[] { "PreviousResultId", "BlockId", "Chunks", "ServiceEvents", "ExecutionDataId", });
internal_static_flow_entities_Chunk_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_flow_entities_Chunk_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_flow_entities_Chunk_descriptor,
new java.lang.String[] { "CollectionIndex", "StartState", "EventCollection", "BlockId", "TotalComputationUsed", "NumberOfTransactions", "Index", "EndState", "ExecutionDataId", "StateDeltaCommitment", });
internal_static_flow_entities_ServiceEvent_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_flow_entities_ServiceEvent_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_flow_entities_ServiceEvent_descriptor,
new java.lang.String[] { "Type", "Payload", });
internal_static_flow_entities_ExecutionReceiptMeta_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_flow_entities_ExecutionReceiptMeta_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_flow_entities_ExecutionReceiptMeta_descriptor,
new java.lang.String[] { "ExecutorId", "ResultId", "Spocks", "ExecutorSignature", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy