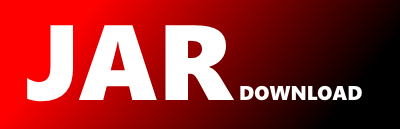
org.onosproject.net.topology.TopologyStore Maven / Gradle / Ivy
/*
* Copyright 2014 Open Networking Laboratory
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.onosproject.net.topology;
import org.onosproject.event.Event;
import org.onosproject.net.ConnectPoint;
import org.onosproject.net.DeviceId;
import org.onosproject.net.Link;
import org.onosproject.net.Path;
import org.onosproject.net.DisjointPath;
import org.onosproject.net.provider.ProviderId;
import org.onosproject.store.Store;
import java.util.List;
import java.util.Set;
import java.util.Map;
/**
* Manages inventory of topology snapshots; not intended for direct use.
*/
public interface TopologyStore extends Store {
/**
* Returns the current topology snapshot.
*
* @return current topology descriptor
*/
Topology currentTopology();
/**
* Indicates whether the topology is the latest.
*
* @param topology topology descriptor
* @return true if topology is the most recent one
*/
boolean isLatest(Topology topology);
/**
* Returns the immutable graph view of the current topology.
*
* @param topology topology descriptor
* @return graph view
*/
TopologyGraph getGraph(Topology topology);
/**
* Returns the set of topology SCC clusters.
*
* @param topology topology descriptor
* @return set of clusters
*/
Set getClusters(Topology topology);
/**
* Returns the cluster of the specified topology.
*
* @param topology topology descriptor
* @param clusterId cluster identity
* @return topology cluster
*/
TopologyCluster getCluster(Topology topology, ClusterId clusterId);
/**
* Returns the cluster of the specified topology.
*
* @param topology topology descriptor
* @param cluster topology cluster
* @return set of cluster links
*/
Set getClusterDevices(Topology topology, TopologyCluster cluster);
/**
* Returns the cluster of the specified topology.
*
* @param topology topology descriptor
* @param cluster topology cluster
* @return set of cluster links
*/
Set getClusterLinks(Topology topology, TopologyCluster cluster);
/**
* Returns the set of pre-computed shortest paths between src and dest.
*
* @param topology topology descriptor
* @param src source device
* @param dst destination device
* @return set of shortest paths
*/
Set getPaths(Topology topology, DeviceId src, DeviceId dst);
/**
* Computes and returns the set of shortest paths between src and dest.
*
* @param topology topology descriptor
* @param src source device
* @param dst destination device
* @param weight link weight function
* @return set of shortest paths
*/
Set getPaths(Topology topology, DeviceId src, DeviceId dst,
LinkWeight weight);
/**
* Computes and returns the set of disjoint shortest path pairs
* between src and dst.
*
* @param topology topology descriptor
* @param src source device
* @param dst destination device
* @param weight link weight function
* @return set of shortest paths
*/
Set getDisjointPaths(Topology topology, DeviceId src, DeviceId dst,
LinkWeight weight);
/**
* Computes and returns the set of disjoint shortest path pairs
* between src and dst.
*
* @param topology topology descriptor
* @param src source device
* @param dst destination device
* @return set of shortest paths
*/
Set getDisjointPaths(Topology topology, DeviceId src, DeviceId dst);
/**
* Computes and returns the set of SRLG disjoint shortest path pairs between source
* and dst, given a mapping of edges to SRLG risk groups.
*
* @param topology topology descriptor
* @param src source device
* @param dst destination device
* @param weight link weight function
* @param riskProfile map of edges to objects. Edges that map to the same object will
* be treated as if they were in the same risk group.
* @return set of shortest paths
*/
Set getDisjointPaths(Topology topology, DeviceId src, DeviceId dst,
LinkWeight weight, Map riskProfile);
/**
* Returns the set of pre-computed SRLG shortest paths between src and dest.
*
* @param topology topology descriptor
* @param src source device
* @param dst destination device
* @param riskProfile map of edges to objects. Edges that map to the same object will
* be treated as if they were in the same risk group.
* @return set of shortest paths
*/
Set getDisjointPaths(Topology topology, DeviceId src, DeviceId dst,
Map riskProfile);
/**
* Indicates whether the given connect point is part of the network fabric.
*
* @param topology topology descriptor
* @param connectPoint connection point
* @return true if infrastructure; false otherwise
*/
boolean isInfrastructure(Topology topology, ConnectPoint connectPoint);
/**
* Indicates whether broadcast is allowed for traffic received on the
* given connection point.
*
* @param topology topology descriptor
* @param connectPoint connection point
* @return true if broadcast allowed; false otherwise
*/
boolean isBroadcastPoint(Topology topology, ConnectPoint connectPoint);
/**
* Generates a new topology snapshot from the specified description.
*
* @param providerId provider identification
* @param graphDescription topology graph description
* @param reasons list of events that triggered the update
* @return topology update event or null if the description is old
*/
TopologyEvent updateTopology(ProviderId providerId,
GraphDescription graphDescription,
List reasons);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy