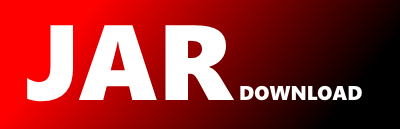
org.onosproject.store.service.AsyncDocumentTree Maven / Gradle / Ivy
/*
* Copyright 2016-present Open Networking Foundation
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.onosproject.store.service;
import org.onosproject.store.primitives.NodeUpdate;
import java.util.Map;
import java.util.concurrent.CompletableFuture;
import javax.annotation.concurrent.NotThreadSafe;
import org.onosproject.store.primitives.DefaultDocumentTree;
/**
* A hierarchical document tree data structure.
*
* @param document tree value type
*/
@NotThreadSafe
public interface AsyncDocumentTree extends DistributedPrimitive, Transactional> {
@Override
default Type primitiveType() {
return Type.DOCUMENT_TREE;
}
/**
* Returns the {@link DocumentPath path} to root of the tree.
*
* @return path to root of the tree
*/
DocumentPath root();
/**
* Returns the children of node at specified path in the form of a mapping from child name to child value.
*
* @param path path to the node
* @return future for mapping from child name to child value
* @throws NoSuchDocumentPathException if the path does not point to a valid node
*/
CompletableFuture
© 2015 - 2025 Weber Informatics LLC | Privacy Policy