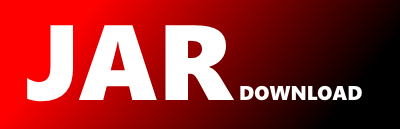
org.projectfloodlight.openflow.protocol.match.MatchField Maven / Gradle / Ivy
package org.projectfloodlight.openflow.protocol.match;
import org.projectfloodlight.openflow.types.ArpOpcode;
import org.projectfloodlight.openflow.types.ClassId;
import org.projectfloodlight.openflow.types.EthType;
import org.projectfloodlight.openflow.types.ICMPv4Code;
import org.projectfloodlight.openflow.types.ICMPv4Type;
import org.projectfloodlight.openflow.types.IPv4Address;
import org.projectfloodlight.openflow.types.IPv6Address;
import org.projectfloodlight.openflow.types.IPv6FlowLabel;
import org.projectfloodlight.openflow.types.IpDscp;
import org.projectfloodlight.openflow.types.IpEcn;
import org.projectfloodlight.openflow.types.IpProtocol;
import org.projectfloodlight.openflow.types.LagId;
import org.projectfloodlight.openflow.types.MacAddress;
import org.projectfloodlight.openflow.types.OFBitMask128;
import org.projectfloodlight.openflow.types.OFBitMask512;
import org.projectfloodlight.openflow.types.OFBooleanValue;
import org.projectfloodlight.openflow.types.OFMetadata;
import org.projectfloodlight.openflow.types.OFPort;
import org.projectfloodlight.openflow.types.OFValueType;
import org.projectfloodlight.openflow.types.OFVlanVidMatch;
import org.projectfloodlight.openflow.types.TransportPort;
import org.projectfloodlight.openflow.types.U16;
import org.projectfloodlight.openflow.types.U32;
import org.projectfloodlight.openflow.types.U64;
import org.projectfloodlight.openflow.types.U8;
import org.projectfloodlight.openflow.types.UDF;
import org.projectfloodlight.openflow.types.VRF;
import org.projectfloodlight.openflow.types.VlanPcp;
import org.projectfloodlight.openflow.types.CircuitSignalID;
import org.projectfloodlight.openflow.types.OduSignalID;
public class MatchField> {
private final String name;
public final MatchFields id;
private final Prerequisite>[] prerequisites;
private MatchField(final String name, final MatchFields id, Prerequisite>... prerequisites) {
this.name = name;
this.id = id;
this.prerequisites = prerequisites;
}
public final static MatchField IN_PORT =
new MatchField("in_port", MatchFields.IN_PORT);
public final static MatchField IN_PHY_PORT =
new MatchField("in_phy_port", MatchFields.IN_PHY_PORT,
new Prerequisite(MatchField.IN_PORT));
public final static MatchField METADATA =
new MatchField("metadata", MatchFields.METADATA);
public final static MatchField ETH_DST =
new MatchField("eth_dst", MatchFields.ETH_DST);
public final static MatchField ETH_SRC =
new MatchField("eth_src", MatchFields.ETH_SRC);
public final static MatchField ETH_TYPE =
new MatchField("eth_type", MatchFields.ETH_TYPE);
public final static MatchField VLAN_VID =
new MatchField("vlan_vid", MatchFields.VLAN_VID);
public final static MatchField VLAN_PCP =
new MatchField("vlan_pcp", MatchFields.VLAN_PCP,
new Prerequisite(MatchField.VLAN_VID));
public final static MatchField IP_DSCP =
new MatchField("ip_dscp", MatchFields.IP_DSCP,
new Prerequisite(MatchField.ETH_TYPE, EthType.IPv4, EthType.IPv6));
public final static MatchField IP_ECN =
new MatchField("ip_ecn", MatchFields.IP_ECN,
new Prerequisite(MatchField.ETH_TYPE, EthType.IPv4, EthType.IPv6));
public final static MatchField IP_PROTO =
new MatchField("ip_proto", MatchFields.IP_PROTO,
new Prerequisite(MatchField.ETH_TYPE, EthType.IPv4, EthType.IPv6));
public final static MatchField IPV4_SRC =
new MatchField("ipv4_src", MatchFields.IPV4_SRC,
new Prerequisite(MatchField.ETH_TYPE, EthType.IPv4));
public final static MatchField IPV4_DST =
new MatchField("ipv4_dst", MatchFields.IPV4_DST,
new Prerequisite(MatchField.ETH_TYPE, EthType.IPv4));
public final static MatchField TCP_SRC = new MatchField(
"tcp_src", MatchFields.TCP_SRC,
new Prerequisite(MatchField.IP_PROTO, IpProtocol.TCP));
public final static MatchField TCP_DST = new MatchField(
"tcp_dst", MatchFields.TCP_DST,
new Prerequisite(MatchField.IP_PROTO, IpProtocol.TCP));
public final static MatchField UDP_SRC = new MatchField(
"udp_src", MatchFields.UDP_SRC,
new Prerequisite(MatchField.IP_PROTO, IpProtocol.UDP));
public final static MatchField UDP_DST = new MatchField(
"udp_dst", MatchFields.UDP_DST,
new Prerequisite(MatchField.IP_PROTO, IpProtocol.UDP));
public final static MatchField SCTP_SRC = new MatchField(
"sctp_src", MatchFields.SCTP_SRC,
new Prerequisite(MatchField.IP_PROTO, IpProtocol.SCTP));
public final static MatchField SCTP_DST = new MatchField(
"sctp_dst", MatchFields.SCTP_DST,
new Prerequisite(MatchField.IP_PROTO, IpProtocol.SCTP));
public final static MatchField ICMPV4_TYPE = new MatchField(
"icmpv4_type", MatchFields.ICMPV4_TYPE,
new Prerequisite(MatchField.IP_PROTO, IpProtocol.ICMP));
public final static MatchField ICMPV4_CODE = new MatchField(
"icmpv4_code", MatchFields.ICMPV4_CODE,
new Prerequisite(MatchField.IP_PROTO, IpProtocol.ICMP));
public final static MatchField ARP_OP = new MatchField(
"arp_op", MatchFields.ARP_OP,
new Prerequisite(MatchField.ETH_TYPE, EthType.ARP));
public final static MatchField ARP_SPA =
new MatchField("arp_spa", MatchFields.ARP_SPA,
new Prerequisite(MatchField.ETH_TYPE, EthType.ARP));
public final static MatchField ARP_TPA =
new MatchField("arp_tpa", MatchFields.ARP_TPA,
new Prerequisite(MatchField.ETH_TYPE, EthType.ARP));
public final static MatchField ARP_SHA =
new MatchField("arp_sha", MatchFields.ARP_SHA,
new Prerequisite(MatchField.ETH_TYPE, EthType.ARP));
public final static MatchField ARP_THA =
new MatchField("arp_tha", MatchFields.ARP_THA,
new Prerequisite(MatchField.ETH_TYPE, EthType.ARP));
public final static MatchField IPV6_SRC =
new MatchField("ipv6_src", MatchFields.IPV6_SRC,
new Prerequisite(MatchField.ETH_TYPE, EthType.IPv6));
public final static MatchField IPV6_DST =
new MatchField("ipv6_dst", MatchFields.IPV6_DST,
new Prerequisite(MatchField.ETH_TYPE, EthType.IPv6));
public final static MatchField IPV6_FLABEL =
new MatchField("ipv6_flabel", MatchFields.IPV6_FLABEL,
new Prerequisite(MatchField.ETH_TYPE, EthType.IPv6));
public final static MatchField ICMPV6_TYPE =
new MatchField("icmpv6_type", MatchFields.ICMPV6_TYPE,
new Prerequisite(MatchField.IP_PROTO, IpProtocol.IPv6_ICMP));
public final static MatchField ICMPV6_CODE =
new MatchField("icmpv6_code", MatchFields.ICMPV6_CODE,
new Prerequisite(MatchField.IP_PROTO, IpProtocol.IPv6_ICMP));
public final static MatchField IPV6_ND_TARGET =
new MatchField("ipv6_nd_target", MatchFields.IPV6_ND_TARGET,
new Prerequisite(MatchField.ICMPV6_TYPE, U8.of((short)135), U8.of((short)136)));
public final static MatchField IPV6_ND_SLL =
new MatchField("ipv6_nd_sll", MatchFields.IPV6_ND_SLL,
new Prerequisite(MatchField.ICMPV6_TYPE, U8.of((short)135)));
public final static MatchField IPV6_ND_TLL =
new MatchField("ipv6_nd_tll", MatchFields.IPV6_ND_TLL,
new Prerequisite(MatchField.ICMPV6_TYPE, U8.of((short)136)));
public final static MatchField MPLS_LABEL =
new MatchField("mpls_label", MatchFields.MPLS_LABEL,
new Prerequisite(MatchField.ETH_TYPE, EthType.MPLS_UNICAST, EthType.MPLS_MULTICAST));
public final static MatchField MPLS_TC =
new MatchField("mpls_tc", MatchFields.MPLS_TC,
new Prerequisite(MatchField.ETH_TYPE, EthType.MPLS_UNICAST, EthType.MPLS_MULTICAST));
public final static MatchField MPLS_BOS =
new MatchField("mpls_bos", MatchFields.MPLS_BOS,
new Prerequisite(MatchField.ETH_TYPE, EthType.MPLS_UNICAST, EthType.MPLS_MULTICAST));
public final static MatchField TUNNEL_ID =
new MatchField("tunnel_id", MatchFields.TUNNEL_ID);
public final static MatchField IPV6_EXTHDR =
new MatchField("ipv6_exthdr", MatchFields.IPV6_EXTHDR);
public final static MatchField PBB_UCA =
new MatchField("pbb_uca", MatchFields.PBB_UCA);
public final static MatchField TUNNEL_IPV4_SRC =
new MatchField("tunnel_ipv4_src", MatchFields.TUNNEL_IPV4_SRC,
new Prerequisite(MatchField.ETH_TYPE, EthType.IPv4));
public final static MatchField TUNNEL_IPV4_DST =
new MatchField("tunnel_ipv4_dst", MatchFields.TUNNEL_IPV4_DST,
new Prerequisite(MatchField.ETH_TYPE, EthType.IPv4));
public final static MatchField BSN_IN_PORTS_128 =
new MatchField("bsn_in_ports_128", MatchFields.BSN_IN_PORTS_128);
public final static MatchField BSN_IN_PORTS_512 =
new MatchField("bsn_in_ports_512", MatchFields.BSN_IN_PORTS_512);
public final static MatchField BSN_LAG_ID =
new MatchField("bsn_lag_id", MatchFields.BSN_LAG_ID);
public final static MatchField BSN_VRF =
new MatchField("bsn_vrf", MatchFields.BSN_VRF);
public final static MatchField BSN_GLOBAL_VRF_ALLOWED =
new MatchField("bsn_global_vrf_allowed", MatchFields.BSN_GLOBAL_VRF_ALLOWED);
public final static MatchField BSN_L3_INTERFACE_CLASS_ID =
new MatchField("bsn_l3_interface_class_id", MatchFields.BSN_L3_INTERFACE_CLASS_ID);
public final static MatchField BSN_L3_SRC_CLASS_ID =
new MatchField("bsn_l3_src_class_id", MatchFields.BSN_L3_SRC_CLASS_ID);
public final static MatchField BSN_L3_DST_CLASS_ID =
new MatchField("bsn_l3_dst_class_id", MatchFields.BSN_L3_DST_CLASS_ID);
public final static MatchField BSN_EGR_PORT_GROUP_ID =
new MatchField("bsn_egr_port_group_id", MatchFields.BSN_EGR_PORT_GROUP_ID);
public final static MatchField BSN_INGRESS_PORT_GROUP_ID =
new MatchField("bsn_ingress_port_group_id", MatchFields.BSN_INGRESS_PORT_GROUP_ID);
public final static MatchField BSN_UDF0 =
new MatchField("bsn_udf", MatchFields.BSN_UDF0);
public final static MatchField BSN_UDF1 =
new MatchField("bsn_udf", MatchFields.BSN_UDF1);
public final static MatchField BSN_UDF2 =
new MatchField("bsn_udf", MatchFields.BSN_UDF2);
public final static MatchField BSN_UDF3 =
new MatchField("bsn_udf", MatchFields.BSN_UDF3);
public final static MatchField BSN_UDF4 =
new MatchField("bsn_udf", MatchFields.BSN_UDF4);
public final static MatchField BSN_UDF5 =
new MatchField("bsn_udf", MatchFields.BSN_UDF5);
public final static MatchField BSN_UDF6 =
new MatchField("bsn_udf", MatchFields.BSN_UDF6);
public final static MatchField BSN_UDF7 =
new MatchField("bsn_udf", MatchFields.BSN_UDF7);
public final static MatchField BSN_TCP_FLAGS =
new MatchField("bsn_tcp_flags", MatchFields.BSN_TCP_FLAGS);
public final static MatchField BSN_VLAN_XLATE_PORT_GROUP_ID =
new MatchField("bsn_vlan_xlate_port_group_id", MatchFields.BSN_VLAN_XLATE_PORT_GROUP_ID);
public final static MatchField OCH_SIGTYPE =
new MatchField("och_sigtype",
MatchFields.OCH_SIGTYPE);
public final static MatchField OCH_SIGTYPE_BASIC =
new MatchField("och_sigtype_basic",
MatchFields.OCH_SIGTYPE_BASIC);
public final static MatchField OCH_SIGID =
new MatchField("och_sigid",
MatchFields.OCH_SIGID);
public final static MatchField OCH_SIGID_BASIC =
new MatchField("och_sigid_basic",
MatchFields.OCH_SIGID);
public final static MatchField OCH_SIGATT =
new MatchField("och_sigatt",
MatchFields.OCH_SIGATT);
public final static MatchField OCH_SIGATT_BASIC =
new MatchField("och_sigatt_basic",
MatchFields.OCH_SIGATT_BASIC);
public final static MatchField BSN_L2_CACHE_HIT =
new MatchField("bsn_l2_cache_hit", MatchFields.BSN_L2_CACHE_HIT);
public final static MatchField BSN_VXLAN_NETWORK_ID =
new MatchField("bsn_vxlan_network_id", MatchFields.BSN_VXLAN_NETWORK_ID);
public final static MatchField BSN_INNER_ETH_DST =
new MatchField("bsn_inner_eth_dst", MatchFields.BSN_INNER_ETH_DST);
public final static MatchField BSN_INNER_ETH_SRC =
new MatchField("bsn_inner_eth_src", MatchFields.BSN_INNER_ETH_SRC);
public final static MatchField BSN_INNER_VLAN_VID =
new MatchField("bsn_inner_vlan_vid", MatchFields.BSN_INNER_VLAN_VID);
public final static MatchField EXP_ODU_SIG_ID =
new MatchField("exp_odu_sig_id", MatchFields.EXP_ODU_SIG_ID);
public final static MatchField EXP_ODU_SIGTYPE =
new MatchField("exp_odu_sigtype", MatchFields.EXP_ODU_SIGTYPE);
public final static MatchField EXP_OCH_SIG_ID =
new MatchField("exp_och_sig_id", MatchFields.EXP_OCH_SIG_ID);
public final static MatchField EXP_OCH_SIGTYPE =
new MatchField("exp_och_sigtype", MatchFields.EXP_OCH_SIGTYPE);
public final static MatchField REG0 =
new MatchField("reg0", MatchFields.REG0);
public final static MatchField REG1 =
new MatchField("reg1", MatchFields.REG1);
public final static MatchField REG2 =
new MatchField("reg2", MatchFields.REG2);
public final static MatchField REG3 =
new MatchField("reg3", MatchFields.REG3);
public final static MatchField REG4 =
new MatchField("reg4", MatchFields.REG4);
public final static MatchField REG5 =
new MatchField("reg5", MatchFields.REG5);
public final static MatchField REG6 =
new MatchField("reg6", MatchFields.REG6);
public final static MatchField REG7 =
new MatchField("reg7", MatchFields.REG7);
public final static MatchField NSP =
new MatchField("nsp", MatchFields.NSP);
public final static MatchField NSI =
new MatchField("nsi", MatchFields.NSI);
public final static MatchField NSH_C1 =
new MatchField("nshc1", MatchFields.NSH_C1);
public final static MatchField NSH_C2 =
new MatchField("nshc2", MatchFields.NSH_C2);
public final static MatchField NSH_C3 =
new MatchField("nshc3", MatchFields.NSH_C3);
public final static MatchField NSH_C4 =
new MatchField("nshc4", MatchFields.NSH_C4);
public final static MatchField NSH_MDTYPE =
new MatchField("nsh_mdtype", MatchFields.NSH_MDTYPE);
public final static MatchField NSH_NP =
new MatchField("nsh_np", MatchFields.NSH_NP);
public final static MatchField ENCAP_ETH_SRC =
new MatchField("encap_eth_src", MatchFields.ENCAP_ETH_SRC);
public final static MatchField ENCAP_ETH_DST =
new MatchField("encap_eth_dst", MatchFields.ENCAP_ETH_DST);
public final static MatchField ENCAP_ETH_TYPE =
new MatchField("encap_eth_type", MatchFields.ENCAP_ETH_TYPE);
public final static MatchField TUN_FLAGS =
new MatchField("tun_flags", MatchFields.TUN_FLAGS);
public final static MatchField TUN_GBP_ID =
new MatchField("tun_gbp_id", MatchFields.TUN_GBP_ID);
public final static MatchField TUN_GBP_FLAGS =
new MatchField("tun_gbp_flags", MatchFields.TUN_GBP_FLAGS);
public final static MatchField TUN_GPE_NP =
new MatchField("tun_gpe_np", MatchFields.TUN_GPE_NP);
public final static MatchField TUN_GPE_FLAGS =
new MatchField("tun_gpe_flags", MatchFields.TUN_GPE_NP);
public String getName() {
return name;
}
public boolean arePrerequisitesOK(Match match) {
for (Prerequisite> p : this.prerequisites) {
if (!p.isSatisfied(match)) {
return false;
}
}
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy