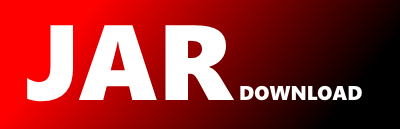
org.openmetadata.client.api.WorkflowInstancesApi Maven / Gradle / Ivy
package org.openmetadata.client.api;
import org.openmetadata.client.ApiClient;
import org.openmetadata.client.EncodingUtils;
import org.openmetadata.client.model.ApiResponse;
import java.math.BigDecimal;
import org.openmetadata.client.model.WorkflowInstanceResultList;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import feign.*;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-11-20T14:35:21.920766473Z[Etc/UTC]")
public interface WorkflowInstancesApi extends ApiClient.Api {
/**
* List the Workflow Instances
* Get a list of all the workflow instances, filtered by `startTs` and `endTs` of the creation, and Workflow Definition FQN. Use cursor-based pagination to limit the number of entries in the list using `limit` and `before` or `after` query params.
* @param limit Limit the number of Workflow Instances returned. (1 to 1000000, default = 10) (optional, default to 10)
* @param offset Returns list of Workflow Instances at the offset (optional)
* @param startTs Filter Workflow Instances after the given start timestamp (optional)
* @param endTs Filter Workflow Instances before the given end timestamp (optional)
* @param latest Only list the latest Workflow Instance (optional, default to false)
* @param workflowDefinitionName Workflow Definition Name (optional)
* @return WorkflowInstanceResultList
*/
@RequestLine("GET /v1/governance/workflowInstances?limit={limit}&offset={offset}&startTs={startTs}&endTs={endTs}&latest={latest}&workflowDefinitionName={workflowDefinitionName}")
@Headers({
"Accept: application/json",
})
WorkflowInstanceResultList listWorkflowInstances(@Param("limit") Integer limit, @Param("offset") String offset, @Param("startTs") BigDecimal startTs, @Param("endTs") BigDecimal endTs, @Param("latest") Boolean latest, @Param("workflowDefinitionName") String workflowDefinitionName);
/**
* List the Workflow Instances
* Similar to listWorkflowInstances
but it also returns the http response headers .
* Get a list of all the workflow instances, filtered by `startTs` and `endTs` of the creation, and Workflow Definition FQN. Use cursor-based pagination to limit the number of entries in the list using `limit` and `before` or `after` query params.
* @param limit Limit the number of Workflow Instances returned. (1 to 1000000, default = 10) (optional, default to 10)
* @param offset Returns list of Workflow Instances at the offset (optional)
* @param startTs Filter Workflow Instances after the given start timestamp (optional)
* @param endTs Filter Workflow Instances before the given end timestamp (optional)
* @param latest Only list the latest Workflow Instance (optional, default to false)
* @param workflowDefinitionName Workflow Definition Name (optional)
* @return A ApiResponse that wraps the response boyd and the http headers.
*/
@RequestLine("GET /v1/governance/workflowInstances?limit={limit}&offset={offset}&startTs={startTs}&endTs={endTs}&latest={latest}&workflowDefinitionName={workflowDefinitionName}")
@Headers({
"Accept: application/json",
})
ApiResponse listWorkflowInstancesWithHttpInfo(@Param("limit") Integer limit, @Param("offset") String offset, @Param("startTs") BigDecimal startTs, @Param("endTs") BigDecimal endTs, @Param("latest") Boolean latest, @Param("workflowDefinitionName") String workflowDefinitionName);
/**
* List the Workflow Instances
* Get a list of all the workflow instances, filtered by `startTs` and `endTs` of the creation, and Workflow Definition FQN. Use cursor-based pagination to limit the number of entries in the list using `limit` and `before` or `after` query params.
* Note, this is equivalent to the other listWorkflowInstances
method,
* but with the query parameters collected into a single Map parameter. This
* is convenient for services with optional query parameters, especially when
* used with the {@link ListWorkflowInstancesQueryParams} class that allows for
* building up this map in a fluent style.
* @param queryParams Map of query parameters as name-value pairs
* The following elements may be specified in the query map:
*
* - limit - Limit the number of Workflow Instances returned. (1 to 1000000, default = 10) (optional, default to 10)
* - offset - Returns list of Workflow Instances at the offset (optional)
* - startTs - Filter Workflow Instances after the given start timestamp (optional)
* - endTs - Filter Workflow Instances before the given end timestamp (optional)
* - latest - Only list the latest Workflow Instance (optional, default to false)
* - workflowDefinitionName - Workflow Definition Name (optional)
*
* @return WorkflowInstanceResultList
*/
@RequestLine("GET /v1/governance/workflowInstances?limit={limit}&offset={offset}&startTs={startTs}&endTs={endTs}&latest={latest}&workflowDefinitionName={workflowDefinitionName}")
@Headers({
"Accept: application/json",
})
WorkflowInstanceResultList listWorkflowInstances(@QueryMap(encoded=true) ListWorkflowInstancesQueryParams queryParams);
/**
* List the Workflow Instances
* Get a list of all the workflow instances, filtered by `startTs` and `endTs` of the creation, and Workflow Definition FQN. Use cursor-based pagination to limit the number of entries in the list using `limit` and `before` or `after` query params.
* Note, this is equivalent to the other listWorkflowInstances
that receives the query parameters as a map,
* but this one also exposes the Http response headers
* @param queryParams Map of query parameters as name-value pairs
* The following elements may be specified in the query map:
*
* - limit - Limit the number of Workflow Instances returned. (1 to 1000000, default = 10) (optional, default to 10)
* - offset - Returns list of Workflow Instances at the offset (optional)
* - startTs - Filter Workflow Instances after the given start timestamp (optional)
* - endTs - Filter Workflow Instances before the given end timestamp (optional)
* - latest - Only list the latest Workflow Instance (optional, default to false)
* - workflowDefinitionName - Workflow Definition Name (optional)
*
* @return WorkflowInstanceResultList
*/
@RequestLine("GET /v1/governance/workflowInstances?limit={limit}&offset={offset}&startTs={startTs}&endTs={endTs}&latest={latest}&workflowDefinitionName={workflowDefinitionName}")
@Headers({
"Accept: application/json",
})
ApiResponse listWorkflowInstancesWithHttpInfo(@QueryMap(encoded=true) ListWorkflowInstancesQueryParams queryParams);
/**
* A convenience class for generating query parameters for the
* listWorkflowInstances
method in a fluent style.
*/
public static class ListWorkflowInstancesQueryParams extends HashMap {
public ListWorkflowInstancesQueryParams limit(final Integer value) {
put("limit", EncodingUtils.encode(value));
return this;
}
public ListWorkflowInstancesQueryParams offset(final String value) {
put("offset", EncodingUtils.encode(value));
return this;
}
public ListWorkflowInstancesQueryParams startTs(final BigDecimal value) {
put("startTs", EncodingUtils.encode(value));
return this;
}
public ListWorkflowInstancesQueryParams endTs(final BigDecimal value) {
put("endTs", EncodingUtils.encode(value));
return this;
}
public ListWorkflowInstancesQueryParams latest(final Boolean value) {
put("latest", EncodingUtils.encode(value));
return this;
}
public ListWorkflowInstancesQueryParams workflowDefinitionName(final String value) {
put("workflowDefinitionName", EncodingUtils.encode(value));
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy