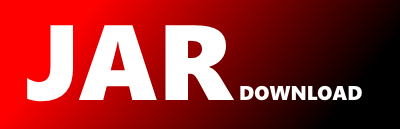
org.openmetadata.schema.services.connections.database.SynapseConnection Maven / Gradle / Ivy
package org.openmetadata.schema.services.connections.database;
import java.util.HashMap;
import java.util.Map;
import javax.annotation.processing.Generated;
import javax.validation.Valid;
import javax.validation.constraints.NotNull;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyDescription;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonValue;
import org.openmetadata.annotations.PasswordField;
/**
* SynapseConnection
*
* Synapse Database Connection Config
*
*/
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"type",
"scheme",
"username",
"password",
"hostPort",
"database",
"driver",
"authenticationMode",
"ingestAllDatabases",
"connectionOptions",
"connectionArguments",
"supportsMetadataExtraction",
"supportsDBTExtraction",
"supportsProfiler",
"supportsDatabase",
"supportsUsageExtraction",
"supportsLineageExtraction",
"sampleDataStorageConfig",
"supportsQueryComment"
})
@Generated("jsonschema2pojo")
public class SynapseConnection {
/**
* Service type.
*
*/
@JsonProperty("type")
@JsonPropertyDescription("Service type.")
private SynapseConnection.SynapseType type = SynapseConnection.SynapseType.fromValue("Synapse");
/**
* SQLAlchemy driver scheme options.
*
*/
@JsonProperty("scheme")
@JsonPropertyDescription("SQLAlchemy driver scheme options.")
private SynapseConnection.SynapseScheme scheme = SynapseConnection.SynapseScheme.fromValue("mssql+pyodbc");
/**
* Username
*
* Username to connect to Azure Synapse. This user should have privileges to read all the metadata in Azure Synapse.
*
*/
@JsonProperty("username")
@JsonPropertyDescription("Username to connect to Azure Synapse. This user should have privileges to read all the metadata in Azure Synapse.")
private String username;
/**
* Password
*
* Password to connect to Azure Synapse.
*
*/
@JsonProperty("password")
@JsonPropertyDescription("Password to connect to Azure Synapse.")
@PasswordField
private String password;
/**
* Host and Port
*
* Host and port of the Azure Synapse service.
* (Required)
*
*/
@JsonProperty("hostPort")
@JsonPropertyDescription("Host and port of the Azure Synapse service.")
@NotNull
private String hostPort;
/**
* Database
*
* Database of the data source. This is optional parameter, if you would like to restrict the metadata reading to a single database. When left blank, OpenMetadata Ingestion attempts to scan all the databases.
* (Required)
*
*/
@JsonProperty("database")
@JsonPropertyDescription("Database of the data source. This is optional parameter, if you would like to restrict the metadata reading to a single database. When left blank, OpenMetadata Ingestion attempts to scan all the databases.")
@NotNull
private String database;
/**
* Driver
*
* ODBC driver version in case of pyodbc connection.
*
*/
@JsonProperty("driver")
@JsonPropertyDescription("ODBC driver version in case of pyodbc connection.")
private String driver = "ODBC Driver 18 for SQL Server";
/**
* Authentication Mode
*
* This parameter determines the mode of authentication for connecting to Azure Synapse using ODBC. If 'Active Directory Password' is selected, you need to provide the password. If 'Active Directory Integrated' is selected, password is not required as it uses the logged-in user's credentials. This mode is useful for establishing secure and seamless connections with Azure Synapse.
*
*/
@JsonProperty("authenticationMode")
@JsonPropertyDescription("This parameter determines the mode of authentication for connecting to Azure Synapse using ODBC. If 'Active Directory Password' is selected, you need to provide the password. If 'Active Directory Integrated' is selected, password is not required as it uses the logged-in user's credentials. This mode is useful for establishing secure and seamless connections with Azure Synapse.")
private AuthenticationMode__1 authenticationMode;
/**
* Ingest All Databases
*
* Ingest data from all databases in Azure Synapse. You can use databaseFilterPattern on top of this.
*
*/
@JsonProperty("ingestAllDatabases")
@JsonPropertyDescription("Ingest data from all databases in Azure Synapse. You can use databaseFilterPattern on top of this.")
private Boolean ingestAllDatabases = false;
/**
* Additional connection options to build the URL that can be sent to service during the connection.
*
*/
@JsonProperty("connectionOptions")
@JsonPropertyDescription("Additional connection options to build the URL that can be sent to service during the connection.")
@Valid
private ConnectionOptions connectionOptions;
/**
* Additional connection arguments such as security or protocol configs that can be sent to service during connection.
*
*/
@JsonProperty("connectionArguments")
@JsonPropertyDescription("Additional connection arguments such as security or protocol configs that can be sent to service during connection.")
@Valid
private ConnectionArguments connectionArguments;
/**
* Supports Metadata Extraction.
*
*/
@JsonProperty("supportsMetadataExtraction")
@JsonPropertyDescription("Supports Metadata Extraction.")
private Boolean supportsMetadataExtraction = true;
/**
* Supports DBT Extraction.
*
*/
@JsonProperty("supportsDBTExtraction")
@JsonPropertyDescription("Supports DBT Extraction.")
private Boolean supportsDBTExtraction = true;
/**
* Supports Profiler
*
*/
@JsonProperty("supportsProfiler")
@JsonPropertyDescription("Supports Profiler")
private Boolean supportsProfiler = true;
/**
* The source service supports the database concept in its hierarchy
*
*/
@JsonProperty("supportsDatabase")
@JsonPropertyDescription("The source service supports the database concept in its hierarchy")
private Boolean supportsDatabase = true;
/**
* Supports Usage Extraction.
*
*/
@JsonProperty("supportsUsageExtraction")
@JsonPropertyDescription("Supports Usage Extraction.")
private Boolean supportsUsageExtraction = true;
/**
* Supports Lineage Extraction.
*
*/
@JsonProperty("supportsLineageExtraction")
@JsonPropertyDescription("Supports Lineage Extraction.")
private Boolean supportsLineageExtraction = true;
/**
* Storage config to store sample data
*
*/
@JsonProperty("sampleDataStorageConfig")
@JsonPropertyDescription("Storage config to store sample data")
@Valid
private SampleDataStorageConfig sampleDataStorageConfig;
/**
* For Database Services using SQLAlchemy, True to enable running a comment for all queries run from OpenMetadata.
*
*/
@JsonProperty("supportsQueryComment")
@JsonPropertyDescription("For Database Services using SQLAlchemy, True to enable running a comment for all queries run from OpenMetadata.")
private Boolean supportsQueryComment = true;
/**
* Service type.
*
*/
@JsonProperty("type")
public SynapseConnection.SynapseType getType() {
return type;
}
/**
* Service type.
*
*/
@JsonProperty("type")
public void setType(SynapseConnection.SynapseType type) {
this.type = type;
}
public SynapseConnection withType(SynapseConnection.SynapseType type) {
this.type = type;
return this;
}
/**
* SQLAlchemy driver scheme options.
*
*/
@JsonProperty("scheme")
public SynapseConnection.SynapseScheme getScheme() {
return scheme;
}
/**
* SQLAlchemy driver scheme options.
*
*/
@JsonProperty("scheme")
public void setScheme(SynapseConnection.SynapseScheme scheme) {
this.scheme = scheme;
}
public SynapseConnection withScheme(SynapseConnection.SynapseScheme scheme) {
this.scheme = scheme;
return this;
}
/**
* Username
*
* Username to connect to Azure Synapse. This user should have privileges to read all the metadata in Azure Synapse.
*
*/
@JsonProperty("username")
public String getUsername() {
return username;
}
/**
* Username
*
* Username to connect to Azure Synapse. This user should have privileges to read all the metadata in Azure Synapse.
*
*/
@JsonProperty("username")
public void setUsername(String username) {
this.username = username;
}
public SynapseConnection withUsername(String username) {
this.username = username;
return this;
}
/**
* Password
*
* Password to connect to Azure Synapse.
*
*/
@JsonProperty("password")
@PasswordField
public String getPassword() {
return password;
}
/**
* Password
*
* Password to connect to Azure Synapse.
*
*/
@JsonProperty("password")
@PasswordField
public void setPassword(String password) {
this.password = password;
}
public SynapseConnection withPassword(String password) {
this.password = password;
return this;
}
/**
* Host and Port
*
* Host and port of the Azure Synapse service.
* (Required)
*
*/
@JsonProperty("hostPort")
public String getHostPort() {
return hostPort;
}
/**
* Host and Port
*
* Host and port of the Azure Synapse service.
* (Required)
*
*/
@JsonProperty("hostPort")
public void setHostPort(String hostPort) {
this.hostPort = hostPort;
}
public SynapseConnection withHostPort(String hostPort) {
this.hostPort = hostPort;
return this;
}
/**
* Database
*
* Database of the data source. This is optional parameter, if you would like to restrict the metadata reading to a single database. When left blank, OpenMetadata Ingestion attempts to scan all the databases.
* (Required)
*
*/
@JsonProperty("database")
public String getDatabase() {
return database;
}
/**
* Database
*
* Database of the data source. This is optional parameter, if you would like to restrict the metadata reading to a single database. When left blank, OpenMetadata Ingestion attempts to scan all the databases.
* (Required)
*
*/
@JsonProperty("database")
public void setDatabase(String database) {
this.database = database;
}
public SynapseConnection withDatabase(String database) {
this.database = database;
return this;
}
/**
* Driver
*
* ODBC driver version in case of pyodbc connection.
*
*/
@JsonProperty("driver")
public String getDriver() {
return driver;
}
/**
* Driver
*
* ODBC driver version in case of pyodbc connection.
*
*/
@JsonProperty("driver")
public void setDriver(String driver) {
this.driver = driver;
}
public SynapseConnection withDriver(String driver) {
this.driver = driver;
return this;
}
/**
* Authentication Mode
*
* This parameter determines the mode of authentication for connecting to Azure Synapse using ODBC. If 'Active Directory Password' is selected, you need to provide the password. If 'Active Directory Integrated' is selected, password is not required as it uses the logged-in user's credentials. This mode is useful for establishing secure and seamless connections with Azure Synapse.
*
*/
@JsonProperty("authenticationMode")
public AuthenticationMode__1 getAuthenticationMode() {
return authenticationMode;
}
/**
* Authentication Mode
*
* This parameter determines the mode of authentication for connecting to Azure Synapse using ODBC. If 'Active Directory Password' is selected, you need to provide the password. If 'Active Directory Integrated' is selected, password is not required as it uses the logged-in user's credentials. This mode is useful for establishing secure and seamless connections with Azure Synapse.
*
*/
@JsonProperty("authenticationMode")
public void setAuthenticationMode(AuthenticationMode__1 authenticationMode) {
this.authenticationMode = authenticationMode;
}
public SynapseConnection withAuthenticationMode(AuthenticationMode__1 authenticationMode) {
this.authenticationMode = authenticationMode;
return this;
}
/**
* Ingest All Databases
*
* Ingest data from all databases in Azure Synapse. You can use databaseFilterPattern on top of this.
*
*/
@JsonProperty("ingestAllDatabases")
public Boolean getIngestAllDatabases() {
return ingestAllDatabases;
}
/**
* Ingest All Databases
*
* Ingest data from all databases in Azure Synapse. You can use databaseFilterPattern on top of this.
*
*/
@JsonProperty("ingestAllDatabases")
public void setIngestAllDatabases(Boolean ingestAllDatabases) {
this.ingestAllDatabases = ingestAllDatabases;
}
public SynapseConnection withIngestAllDatabases(Boolean ingestAllDatabases) {
this.ingestAllDatabases = ingestAllDatabases;
return this;
}
/**
* Additional connection options to build the URL that can be sent to service during the connection.
*
*/
@JsonProperty("connectionOptions")
public ConnectionOptions getConnectionOptions() {
return connectionOptions;
}
/**
* Additional connection options to build the URL that can be sent to service during the connection.
*
*/
@JsonProperty("connectionOptions")
public void setConnectionOptions(ConnectionOptions connectionOptions) {
this.connectionOptions = connectionOptions;
}
public SynapseConnection withConnectionOptions(ConnectionOptions connectionOptions) {
this.connectionOptions = connectionOptions;
return this;
}
/**
* Additional connection arguments such as security or protocol configs that can be sent to service during connection.
*
*/
@JsonProperty("connectionArguments")
public ConnectionArguments getConnectionArguments() {
return connectionArguments;
}
/**
* Additional connection arguments such as security or protocol configs that can be sent to service during connection.
*
*/
@JsonProperty("connectionArguments")
public void setConnectionArguments(ConnectionArguments connectionArguments) {
this.connectionArguments = connectionArguments;
}
public SynapseConnection withConnectionArguments(ConnectionArguments connectionArguments) {
this.connectionArguments = connectionArguments;
return this;
}
/**
* Supports Metadata Extraction.
*
*/
@JsonProperty("supportsMetadataExtraction")
public Boolean getSupportsMetadataExtraction() {
return supportsMetadataExtraction;
}
/**
* Supports Metadata Extraction.
*
*/
@JsonProperty("supportsMetadataExtraction")
public void setSupportsMetadataExtraction(Boolean supportsMetadataExtraction) {
this.supportsMetadataExtraction = supportsMetadataExtraction;
}
public SynapseConnection withSupportsMetadataExtraction(Boolean supportsMetadataExtraction) {
this.supportsMetadataExtraction = supportsMetadataExtraction;
return this;
}
/**
* Supports DBT Extraction.
*
*/
@JsonProperty("supportsDBTExtraction")
public Boolean getSupportsDBTExtraction() {
return supportsDBTExtraction;
}
/**
* Supports DBT Extraction.
*
*/
@JsonProperty("supportsDBTExtraction")
public void setSupportsDBTExtraction(Boolean supportsDBTExtraction) {
this.supportsDBTExtraction = supportsDBTExtraction;
}
public SynapseConnection withSupportsDBTExtraction(Boolean supportsDBTExtraction) {
this.supportsDBTExtraction = supportsDBTExtraction;
return this;
}
/**
* Supports Profiler
*
*/
@JsonProperty("supportsProfiler")
public Boolean getSupportsProfiler() {
return supportsProfiler;
}
/**
* Supports Profiler
*
*/
@JsonProperty("supportsProfiler")
public void setSupportsProfiler(Boolean supportsProfiler) {
this.supportsProfiler = supportsProfiler;
}
public SynapseConnection withSupportsProfiler(Boolean supportsProfiler) {
this.supportsProfiler = supportsProfiler;
return this;
}
/**
* The source service supports the database concept in its hierarchy
*
*/
@JsonProperty("supportsDatabase")
public Boolean getSupportsDatabase() {
return supportsDatabase;
}
/**
* The source service supports the database concept in its hierarchy
*
*/
@JsonProperty("supportsDatabase")
public void setSupportsDatabase(Boolean supportsDatabase) {
this.supportsDatabase = supportsDatabase;
}
public SynapseConnection withSupportsDatabase(Boolean supportsDatabase) {
this.supportsDatabase = supportsDatabase;
return this;
}
/**
* Supports Usage Extraction.
*
*/
@JsonProperty("supportsUsageExtraction")
public Boolean getSupportsUsageExtraction() {
return supportsUsageExtraction;
}
/**
* Supports Usage Extraction.
*
*/
@JsonProperty("supportsUsageExtraction")
public void setSupportsUsageExtraction(Boolean supportsUsageExtraction) {
this.supportsUsageExtraction = supportsUsageExtraction;
}
public SynapseConnection withSupportsUsageExtraction(Boolean supportsUsageExtraction) {
this.supportsUsageExtraction = supportsUsageExtraction;
return this;
}
/**
* Supports Lineage Extraction.
*
*/
@JsonProperty("supportsLineageExtraction")
public Boolean getSupportsLineageExtraction() {
return supportsLineageExtraction;
}
/**
* Supports Lineage Extraction.
*
*/
@JsonProperty("supportsLineageExtraction")
public void setSupportsLineageExtraction(Boolean supportsLineageExtraction) {
this.supportsLineageExtraction = supportsLineageExtraction;
}
public SynapseConnection withSupportsLineageExtraction(Boolean supportsLineageExtraction) {
this.supportsLineageExtraction = supportsLineageExtraction;
return this;
}
/**
* Storage config to store sample data
*
*/
@JsonProperty("sampleDataStorageConfig")
public SampleDataStorageConfig getSampleDataStorageConfig() {
return sampleDataStorageConfig;
}
/**
* Storage config to store sample data
*
*/
@JsonProperty("sampleDataStorageConfig")
public void setSampleDataStorageConfig(SampleDataStorageConfig sampleDataStorageConfig) {
this.sampleDataStorageConfig = sampleDataStorageConfig;
}
public SynapseConnection withSampleDataStorageConfig(SampleDataStorageConfig sampleDataStorageConfig) {
this.sampleDataStorageConfig = sampleDataStorageConfig;
return this;
}
/**
* For Database Services using SQLAlchemy, True to enable running a comment for all queries run from OpenMetadata.
*
*/
@JsonProperty("supportsQueryComment")
public Boolean getSupportsQueryComment() {
return supportsQueryComment;
}
/**
* For Database Services using SQLAlchemy, True to enable running a comment for all queries run from OpenMetadata.
*
*/
@JsonProperty("supportsQueryComment")
public void setSupportsQueryComment(Boolean supportsQueryComment) {
this.supportsQueryComment = supportsQueryComment;
}
public SynapseConnection withSupportsQueryComment(Boolean supportsQueryComment) {
this.supportsQueryComment = supportsQueryComment;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append(SynapseConnection.class.getName()).append('@').append(Integer.toHexString(System.identityHashCode(this))).append('[');
sb.append("type");
sb.append('=');
sb.append(((this.type == null)?"":this.type));
sb.append(',');
sb.append("scheme");
sb.append('=');
sb.append(((this.scheme == null)?"":this.scheme));
sb.append(',');
sb.append("username");
sb.append('=');
sb.append(((this.username == null)?"":this.username));
sb.append(',');
sb.append("password");
sb.append('=');
sb.append(((this.password == null)?"":this.password));
sb.append(',');
sb.append("hostPort");
sb.append('=');
sb.append(((this.hostPort == null)?"":this.hostPort));
sb.append(',');
sb.append("database");
sb.append('=');
sb.append(((this.database == null)?"":this.database));
sb.append(',');
sb.append("driver");
sb.append('=');
sb.append(((this.driver == null)?"":this.driver));
sb.append(',');
sb.append("authenticationMode");
sb.append('=');
sb.append(((this.authenticationMode == null)?"":this.authenticationMode));
sb.append(',');
sb.append("ingestAllDatabases");
sb.append('=');
sb.append(((this.ingestAllDatabases == null)?"":this.ingestAllDatabases));
sb.append(',');
sb.append("connectionOptions");
sb.append('=');
sb.append(((this.connectionOptions == null)?"":this.connectionOptions));
sb.append(',');
sb.append("connectionArguments");
sb.append('=');
sb.append(((this.connectionArguments == null)?"":this.connectionArguments));
sb.append(',');
sb.append("supportsMetadataExtraction");
sb.append('=');
sb.append(((this.supportsMetadataExtraction == null)?"":this.supportsMetadataExtraction));
sb.append(',');
sb.append("supportsDBTExtraction");
sb.append('=');
sb.append(((this.supportsDBTExtraction == null)?"":this.supportsDBTExtraction));
sb.append(',');
sb.append("supportsProfiler");
sb.append('=');
sb.append(((this.supportsProfiler == null)?"":this.supportsProfiler));
sb.append(',');
sb.append("supportsDatabase");
sb.append('=');
sb.append(((this.supportsDatabase == null)?"":this.supportsDatabase));
sb.append(',');
sb.append("supportsUsageExtraction");
sb.append('=');
sb.append(((this.supportsUsageExtraction == null)?"":this.supportsUsageExtraction));
sb.append(',');
sb.append("supportsLineageExtraction");
sb.append('=');
sb.append(((this.supportsLineageExtraction == null)?"":this.supportsLineageExtraction));
sb.append(',');
sb.append("sampleDataStorageConfig");
sb.append('=');
sb.append(((this.sampleDataStorageConfig == null)?"":this.sampleDataStorageConfig));
sb.append(',');
sb.append("supportsQueryComment");
sb.append('=');
sb.append(((this.supportsQueryComment == null)?"":this.supportsQueryComment));
sb.append(',');
if (sb.charAt((sb.length()- 1)) == ',') {
sb.setCharAt((sb.length()- 1), ']');
} else {
sb.append(']');
}
return sb.toString();
}
@Override
public int hashCode() {
int result = 1;
result = ((result* 31)+((this.supportsMetadataExtraction == null)? 0 :this.supportsMetadataExtraction.hashCode()));
result = ((result* 31)+((this.scheme == null)? 0 :this.scheme.hashCode()));
result = ((result* 31)+((this.supportsProfiler == null)? 0 :this.supportsProfiler.hashCode()));
result = ((result* 31)+((this.ingestAllDatabases == null)? 0 :this.ingestAllDatabases.hashCode()));
result = ((result* 31)+((this.sampleDataStorageConfig == null)? 0 :this.sampleDataStorageConfig.hashCode()));
result = ((result* 31)+((this.type == null)? 0 :this.type.hashCode()));
result = ((result* 31)+((this.supportsUsageExtraction == null)? 0 :this.supportsUsageExtraction.hashCode()));
result = ((result* 31)+((this.supportsDBTExtraction == null)? 0 :this.supportsDBTExtraction.hashCode()));
result = ((result* 31)+((this.connectionArguments == null)? 0 :this.connectionArguments.hashCode()));
result = ((result* 31)+((this.supportsDatabase == null)? 0 :this.supportsDatabase.hashCode()));
result = ((result* 31)+((this.supportsLineageExtraction == null)? 0 :this.supportsLineageExtraction.hashCode()));
result = ((result* 31)+((this.password == null)? 0 :this.password.hashCode()));
result = ((result* 31)+((this.database == null)? 0 :this.database.hashCode()));
result = ((result* 31)+((this.driver == null)? 0 :this.driver.hashCode()));
result = ((result* 31)+((this.connectionOptions == null)? 0 :this.connectionOptions.hashCode()));
result = ((result* 31)+((this.hostPort == null)? 0 :this.hostPort.hashCode()));
result = ((result* 31)+((this.supportsQueryComment == null)? 0 :this.supportsQueryComment.hashCode()));
result = ((result* 31)+((this.authenticationMode == null)? 0 :this.authenticationMode.hashCode()));
result = ((result* 31)+((this.username == null)? 0 :this.username.hashCode()));
return result;
}
@Override
public boolean equals(Object other) {
if (other == this) {
return true;
}
if ((other instanceof SynapseConnection) == false) {
return false;
}
SynapseConnection rhs = ((SynapseConnection) other);
return ((((((((((((((((((((this.supportsMetadataExtraction == rhs.supportsMetadataExtraction)||((this.supportsMetadataExtraction!= null)&&this.supportsMetadataExtraction.equals(rhs.supportsMetadataExtraction)))&&((this.scheme == rhs.scheme)||((this.scheme!= null)&&this.scheme.equals(rhs.scheme))))&&((this.supportsProfiler == rhs.supportsProfiler)||((this.supportsProfiler!= null)&&this.supportsProfiler.equals(rhs.supportsProfiler))))&&((this.ingestAllDatabases == rhs.ingestAllDatabases)||((this.ingestAllDatabases!= null)&&this.ingestAllDatabases.equals(rhs.ingestAllDatabases))))&&((this.sampleDataStorageConfig == rhs.sampleDataStorageConfig)||((this.sampleDataStorageConfig!= null)&&this.sampleDataStorageConfig.equals(rhs.sampleDataStorageConfig))))&&((this.type == rhs.type)||((this.type!= null)&&this.type.equals(rhs.type))))&&((this.supportsUsageExtraction == rhs.supportsUsageExtraction)||((this.supportsUsageExtraction!= null)&&this.supportsUsageExtraction.equals(rhs.supportsUsageExtraction))))&&((this.supportsDBTExtraction == rhs.supportsDBTExtraction)||((this.supportsDBTExtraction!= null)&&this.supportsDBTExtraction.equals(rhs.supportsDBTExtraction))))&&((this.connectionArguments == rhs.connectionArguments)||((this.connectionArguments!= null)&&this.connectionArguments.equals(rhs.connectionArguments))))&&((this.supportsDatabase == rhs.supportsDatabase)||((this.supportsDatabase!= null)&&this.supportsDatabase.equals(rhs.supportsDatabase))))&&((this.supportsLineageExtraction == rhs.supportsLineageExtraction)||((this.supportsLineageExtraction!= null)&&this.supportsLineageExtraction.equals(rhs.supportsLineageExtraction))))&&((this.password == rhs.password)||((this.password!= null)&&this.password.equals(rhs.password))))&&((this.database == rhs.database)||((this.database!= null)&&this.database.equals(rhs.database))))&&((this.driver == rhs.driver)||((this.driver!= null)&&this.driver.equals(rhs.driver))))&&((this.connectionOptions == rhs.connectionOptions)||((this.connectionOptions!= null)&&this.connectionOptions.equals(rhs.connectionOptions))))&&((this.hostPort == rhs.hostPort)||((this.hostPort!= null)&&this.hostPort.equals(rhs.hostPort))))&&((this.supportsQueryComment == rhs.supportsQueryComment)||((this.supportsQueryComment!= null)&&this.supportsQueryComment.equals(rhs.supportsQueryComment))))&&((this.authenticationMode == rhs.authenticationMode)||((this.authenticationMode!= null)&&this.authenticationMode.equals(rhs.authenticationMode))))&&((this.username == rhs.username)||((this.username!= null)&&this.username.equals(rhs.username))));
}
/**
* SQLAlchemy driver scheme options.
*
*/
@Generated("jsonschema2pojo")
public enum SynapseScheme {
MSSQL_PYODBC("mssql+pyodbc");
private final String value;
private final static Map CONSTANTS = new HashMap();
static {
for (SynapseConnection.SynapseScheme c: values()) {
CONSTANTS.put(c.value, c);
}
}
SynapseScheme(String value) {
this.value = value;
}
@Override
public String toString() {
return this.value;
}
@JsonValue
public String value() {
return this.value;
}
@JsonCreator
public static SynapseConnection.SynapseScheme fromValue(String value) {
SynapseConnection.SynapseScheme constant = CONSTANTS.get(value);
if (constant == null) {
throw new IllegalArgumentException(value);
} else {
return constant;
}
}
}
/**
* Service type.
*
*/
@Generated("jsonschema2pojo")
public enum SynapseType {
SYNAPSE("Synapse");
private final String value;
private final static Map CONSTANTS = new HashMap();
static {
for (SynapseConnection.SynapseType c: values()) {
CONSTANTS.put(c.value, c);
}
}
SynapseType(String value) {
this.value = value;
}
@Override
public String toString() {
return this.value;
}
@JsonValue
public String value() {
return this.value;
}
@JsonCreator
public static SynapseConnection.SynapseType fromValue(String value) {
SynapseConnection.SynapseType constant = CONSTANTS.get(value);
if (constant == null) {
throw new IllegalArgumentException(value);
} else {
return constant;
}
}
}
}